文章目录
- 前言
- 本节内容
- 1、`v-text` 和 `v-html`
- 代码
- 效果
- 2、`v-if ` 和 `v-show`
- 代码
- 效果
- 3、`v-bind`
- 3.1、用法:`v-bind:` + `属性` ,简写 `:`
- 3.2、动态 attribute 名
- 效果
- 3.3、内联字符串拼接
- 效果
- 3.4、绑定 class
- 效果
- 3.5、style 绑定
- 3.6、绑定一个全是 attribute 的对象
- 效果
- 其它用法
- 4、附录
- html 标签属性大全
前言
上节,我们学习了
- Vue的起步 和 插值表达式
本节内容
- Vue指令之
v-text
和v-html
- Vue指令之
v-if
和v-show
- Vue指令之
v-bind
绑定 - Vue指令之
v-on
事件处理
1、v-text
和 v-html
{{}}
和v-text
的作用是一样的 都是插入值,直接渲染 ≈innerText
v-html
既能插入值 又能插入标签 ≈innerHTML
代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>指令之v-text和v-html</title>
</head>
<body>
<div id='app'>
<h1>{{ msg }}</h1>
<h2 v-text='msg'></h2>
<div v-html='htmlMsg'></div>
</div>
<script src="./vue.js"></script>
<script>
// {{}}和v-text的作用是一样的 都是插入值 直接渲染 ≈ innerText
// v-html既能插入值 又能插入标签 ≈ innerHTML
new Vue({
el:'#app',
data:{
msg:"插入标签",
htmlMsg:'<h3>金榜探云手</h3>'
}
})
</script>
</body>
</html>
效果
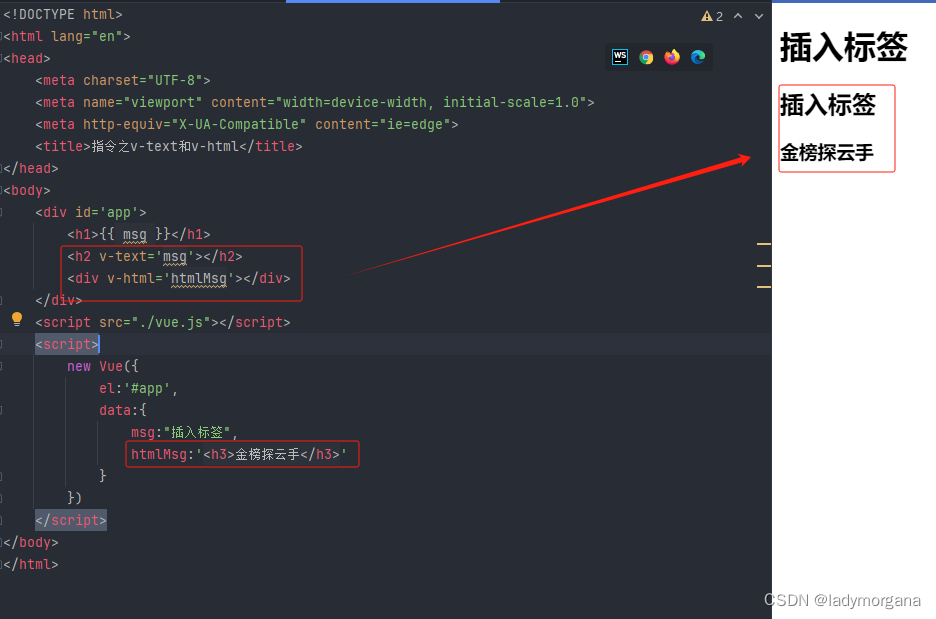
2、v-if
和 v-show
代码
<body>
<div id='app'>
<div v-if = "isShow">
显示
</div>
<div v-else>
隐藏
</div>
<h3 v-show = 'show'>金榜探云手</h3>
</div>
<script src="./vue.js"></script>
<script>
// v-if v-else-if v-else v-show
new Vue({
el: '#app',
data: {
isShow:Math.random() > 0.5,
show:false
}
})
</script>
</body>
效果
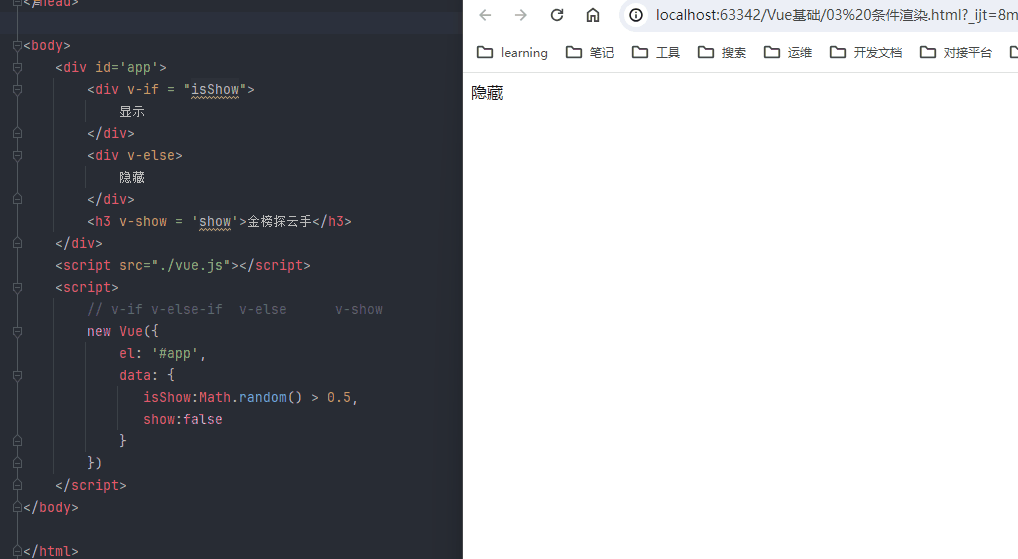
3、v-bind
3.1、用法:v-bind:
+ 属性
,简写 :
<!-- imageSrc 为`data`中属性-->
<img v-bind:src="imageSrc">
<img :src="imageSrc"> <!-- 缩写-->
<script>
new Vue({
el: '#app',
data: {
imageSrc: '../images/logo.png'
}
})
</script>
3.2、动态 attribute 名
<!-- 如:key 为 href ,value = www.baidu.com ,name = 百度一下 -->
<button v-bind:[key]="value">{{name}}</button>
<button :[key]="value">{{name}}</button> <!-- 缩写-->
<script>
new Vue({
el: '#app',
data: {
key: 'href',
value: 'https://www.baidu.com',
name: '百度一下'
}
})
</script>
效果
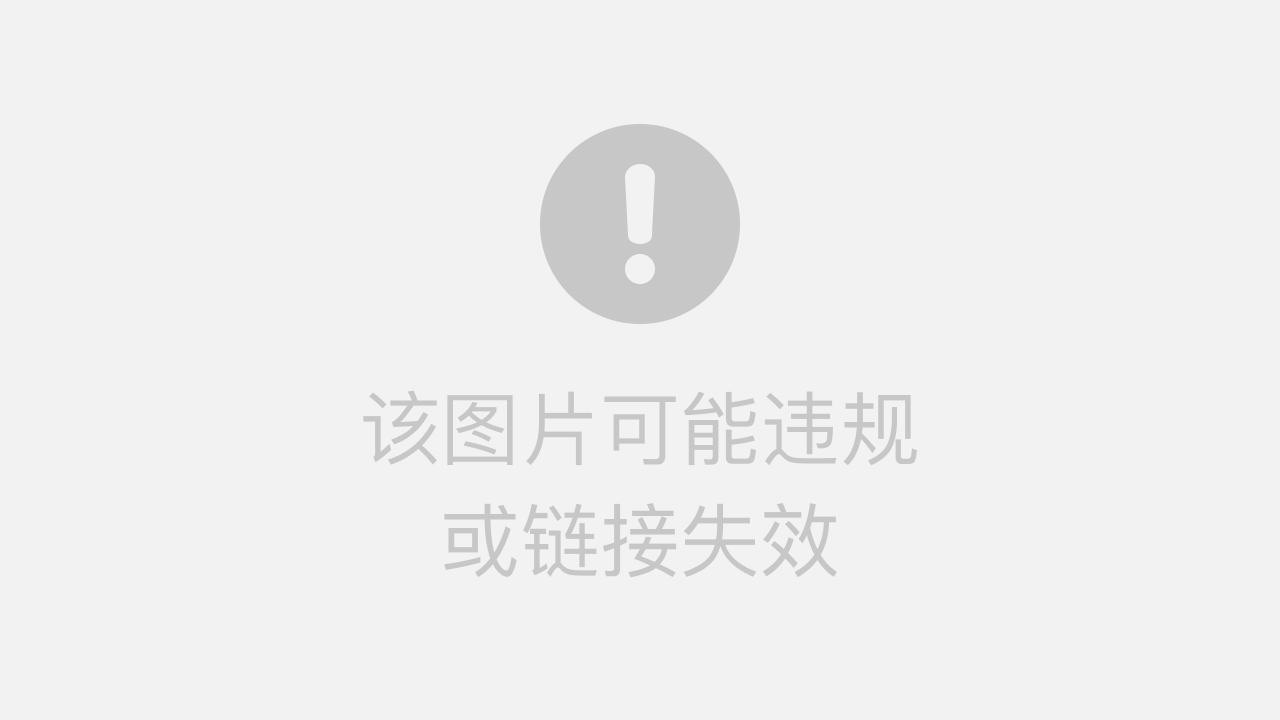
3.3、内联字符串拼接
<!-- 如:key 为 href ,value = www.baidu.com ,name = 百度一下 -->
<img :src="'../images/' + fileName">
<script>
new Vue({
el: '#app',
data: {
fileName: 'logo.png'
}
})
</script>
效果
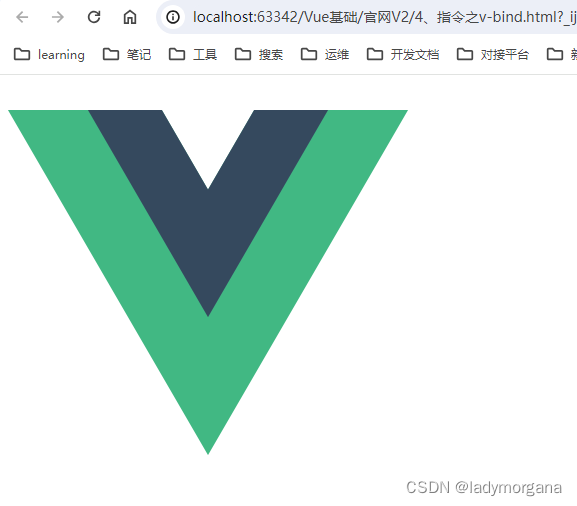
3.4、绑定 class
- 语法一:
:class
=“{style中类名
:布尔值
}”。如::class=“{ red: isRed }” - 语法二:
:class
=“[data属性指向类名
:布尔值
]”。如::class=“[classA, classB]” - 语法三:
{} 和 [] 混合
。如::class=“[classA, { classBd: isB, classCd: isC }]”
<style>
.red {
color: red;
}
.class-A {
color: red;
}
.class-B {
font-size: 30px;
}
.classBd {
font-size: 60px;
}
.classCd {
color: green;
}
</style>
<!-- 语法一: `:class`="{`style中类名`:`布尔值`}" -->
<div :class="{ red: isRed }"></div>
<!-- 语法二: `:class`="[`data属性指向类名`:`布尔值`]" -->
<div :class="[classA, classB]"></div>
<script>
new Vue({
el: '#app',
data: {
isRed: true,
classA: 'class-A',
classB: 'class-B',
classC: 'class-C',
isB: true,
isC: true
}
})
</script>
<div :class="[classA, { classB: isB, classC: isC }]"></div>
效果
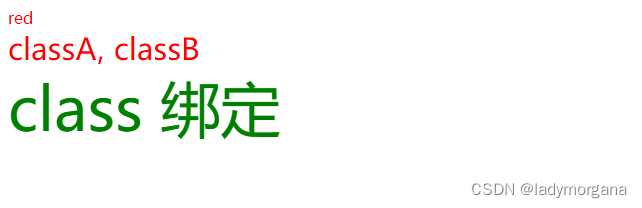
3.5、style 绑定
- 类同 绑定 class
<div :style="{ fontSize: size + 'px' }"></div>
<div :style="[styleObjectA, styleObjectB]"></div>
3.6、绑定一个全是 attribute 的对象
- 语法:
v-bind="{ id: someProp, 'other-attr': otherProp }"
- 作用:定义动态id和attribute(属性)
- 说明
- id 为属性
- ‘other-attr’ 为自定义属性,需要用引号
- 这里不可以用缩写
:
<div id="app">
<!-- 这里表示被vue控制的区域 -->
<div v-bind="{ id: someProp, 'other-attr': otherProp }"></div>
</div>
<script>
const vm = new Vue({
el: '#app', // 控制id为app的元素
data: {
// 存放所需要的数据
someProp: 'demo',
otherProp: 'aaa'
},
methods: {
// 存放所需要调用的方法
},
components: {
// 注册的组件
},
})
</script>
效果
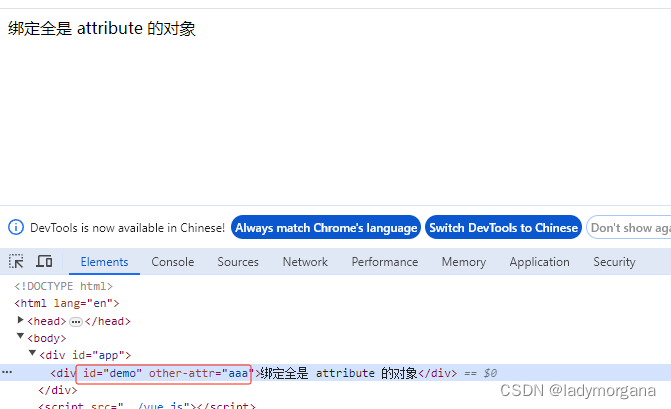
其它用法
<!-- 绑定一个全是 attribute 的对象 -->
<div v-bind="{ id: someProp, 'other-attr': otherProp }"></div>
<!-- 通过 prop 修饰符绑定 DOM attribute -->
<div v-bind:text-content.prop="text"></div>
<!-- prop 绑定。“prop”必须在 my-component 中声明。-->
<my-component :prop="someThing"></my-component>
<!-- 通过 $props 将父组件的 props 一起传给子组件 -->
<child-component v-bind="$props"></child-component>
<!-- XLink -->
<svg><a :xlink:special="foo"></a></svg>
.camel 修饰符允许在使用 DOM 模板时将 v-bind property 名称驼峰化,例如 SVG 的 viewBox property:
<svg :view-box.camel="viewBox"></svg>
4、附录
html 标签属性大全
HTML 标签属性非常多,但是最常用的属性包括:
-
id
: 定义元素的唯一标识符
。 -
class
: 定义元素的一个或多个类名
。 -
style
: 定义元素的行内样式
。 -
href
: 在链接标签 中定义目标URL
。 -
src
: 在资源引用标签<img>, <script>, <iframe>
中定义资源的路径
。 -
alt
: 在图片标签<img>
中定义图片的替代文本。 -
title
: 定义元素的额外信息,通常用作工具提示文本。 -
width
&height
: 在图片或视频
标签中定义尺寸
。 -
type
: 在<script>
和<style>
标签中定义资源的MIME 类型
。 -
placeholder
: 在可输入标签中定义预期输入的提示文本
。