太长时间没更新了,然后,理由是什么呢?是因为阳了,真没想到,吃嘛嘛香的我忽然阳了,果然阳的初期症状就是嘴硬。然后,开始我们连续剧的第二集。
一、进行mysql的安装
学习第一步,从安装开始,别管会不会,先管有没有,你可以说我不会,但不能说我没有。
下载链接: 百度网盘下载链接
里面有详细的安装步骤,按照步骤安装即可。
安装完成后,通过打开MySQL Workbench 8.0 CE 登录我们的账户进入到数据库界面。
二、创建数据库和表
创建数据库
创建数据表
向表内写入数据
右键表选择第一个进行数据的录入
sql语言
通过sql语言实现数据的增删改查
查询数据 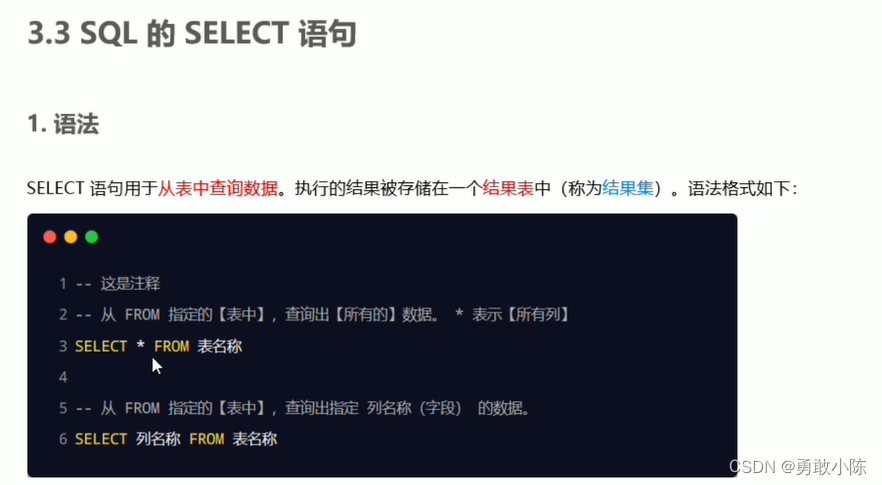
查询代码
-- 通过 *把表中的所有数据查询出来
select * from users
-- 从users表中把username和password查询出来
select username,password from users
添加数据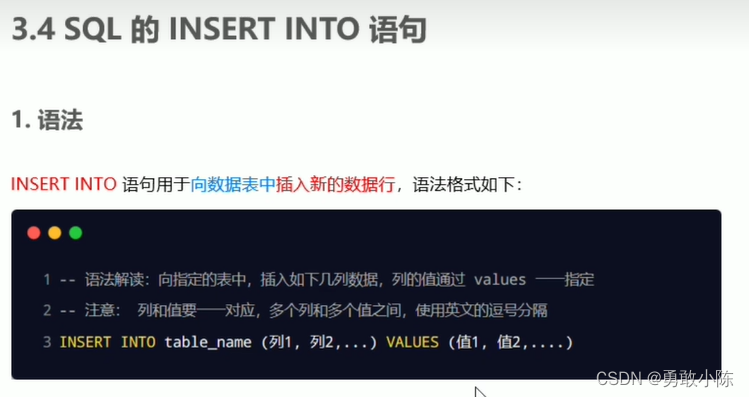
向表中添加数据
-- 向表中添加数据,值与表中的值一一对应
insert into users (username,password) values ('we','456789')
修改数据
更新数据代码
-- 将id为3的用户的密码更新为888888
update users set password='888888' where id=3
更新多个
-- 将id为2的用户密码改为admin123status改为1
update users set password='admin123',status=1 where id=2
删除数据
具体代码
-- 在users中删掉id为3的用户信息
delete from users where id=3
where子句
and与or运算符
and代码
-- 使用and来查询状态为0且id<2的数据
select * from users where status=0 and id<2
or代码
-- 使用or查询username为zs或状态为1的数据
select * from users where username='zs' or status=1
order by子句
进行升序
-- 按照状态进行升序排序
select * from users order by status
进行降序
-- 按照id进行降序排序
select * from users order by id desc
多重排序
-- 对users表中的数据,先按照status进行降序再按照username进行升序
select * from users order by status desc , username
count(*)语法
count代码
-- 使用count(*)来统计users表中,状态为0的数据条数
select count(*) from users where status=0
as设置别名
代码
-- 通过as关键字给列起别名
select count(*) as total from users where status=0
三、在node中去操作数据库
1.安装第三方的mysql模块--mysql提供了在nodejs中操作数据库的能力
yarn add mysql
2.配置mysql模块
新建db.js文件用于数据库的配置
//1.导入mysql模块
const mysql = require('mysql')
//2.建立与mysql数据库的连接关系
const db = mysql.createPool(
{
host: '127.0.0.1',//数据库ip
user: 'root',//登录数据库的账号
password: 'admin123',//登录数据库密码
database: 'csdnyanshi'//指定操作按个表
}
)
//测试mysql模块能否正常工作
db.query('select 1', (err, res) => {
//mysql模块工作期间报错了
if (err) return console.log(err.message);
//能够正常执行mysql模块
console.log(res);
})
输出[ RowDataPacket { '1': 1 } ]表示连接成功,host对应是数据库的ip地址,不是电脑的
3.使用mysql去操作数据---就是在nodejs中执行对应的SQL语句
查询数据
//1.导入mysql模块
const mysql = require('mysql')
//2.建立与mysql数据库的连接关系
const db = mysql.createPool(
{
host: '127.0.0.1',//数据库ip
user: 'root',//登录数据库的账号
password: 'admin123',//登录数据库密码
database: 'csdnyanshi'//指定操作按个表
}
)
//测试mysql模块能否正常工作
const sqlStr = 'select * from users'
db.query(sqlStr, (err, res) => {
//mysql模块工作期间报错了
if (err) return console.log(err.message);
//能够正常执行mysql模块
console.log(res);
})
插入数据
//需要插入的数据
const user = {username:'mz',password:'123456'}
//执行的SQL语句,?表示占位符
const sqlStr = 'insert into users (username,password) values (?,?)'
//执行
db.query(sqlStr,[user.username,user.password],(err,res)=>{
if(err) return err.message
console.log(res);
})
快速的插入数据
//快速插入数据
const user = {username:'mz2222',password:'123456'}
//执行的SQL语句,?表示占位符
const sqlStr = 'insert into users set ?'
//执行
db.query(sqlStr,user,(err,res)=>{
if(err) return console.log(err.message);
if(res.affectedRows=1) console.log(res);
})
更新数据
//更新表中的数据
const user = {username :'111',password:'222',id:6}
const sqlStr= 'update users set username=?,password=? where id=?'
db.query(sqlStr,[user.username,user.password,user.id],(err,res)=>{
if(err) return console.log(err.message);
if(res.affectedRows=1) console.log(res);
})
快速更新数据
//快速更新数据
const user = {username :'11122',password:'222',id:6}
const sqlStr= 'update users set ? where id=?'
db.query(sqlStr,[user,user.id],(err,res)=>{
if(err) return console.log(err.message);
if(res.affectedRows=1) console.log(res);
})
删除数据
//删除数据
const sqlStr = 'delete from users where id=?'
db.query(sqlStr,6,(err,res)=>{
if(err) return console.log(err.message);
if(res.affectedRows=1) console.log(res);
})
标记删除-----在数据中用字段进行标识并不是真正的删除,不然没法恢复