C++之内建函数对象
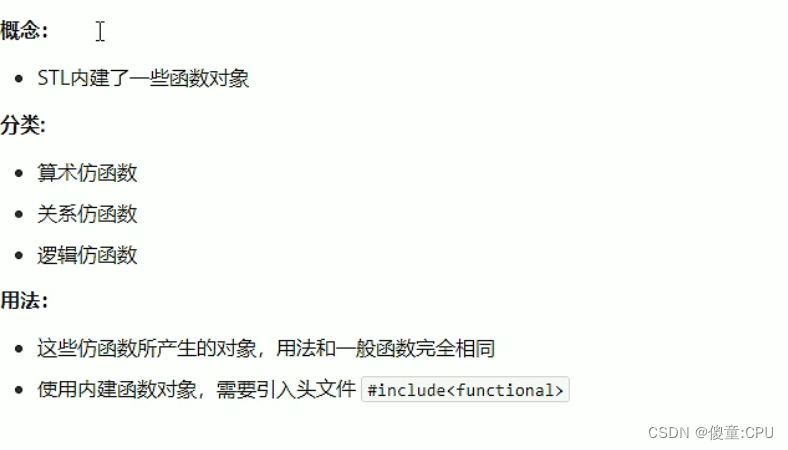
算术仿函数
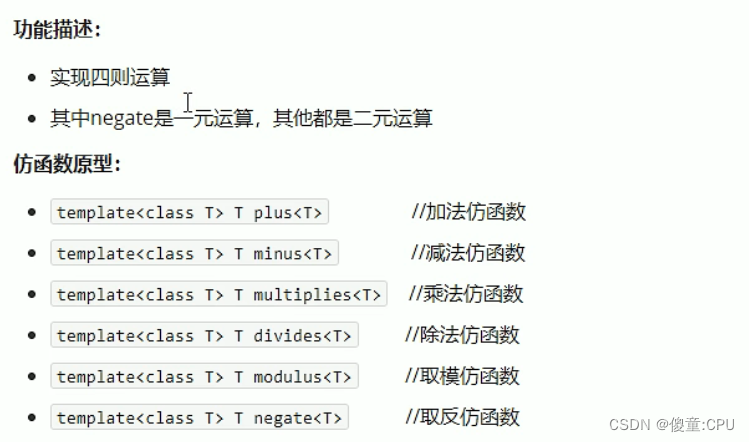
#include<iostream>
using namespace std;
#include<functional>//内建函数对象头文件
//内建函数对象 算术仿函数
void test()
{
// negate 一元仿函数 取反仿函数
negate<int>n;
cout << n(100) << endl;
//plus 二元仿函数 加法
plus<int>p;
cout << p(20, 30) << endl;
}
int main()
{
test();
system("pause");
return 0;
}
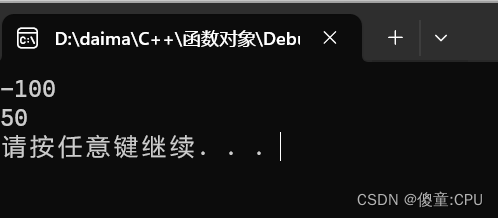
关系仿函数
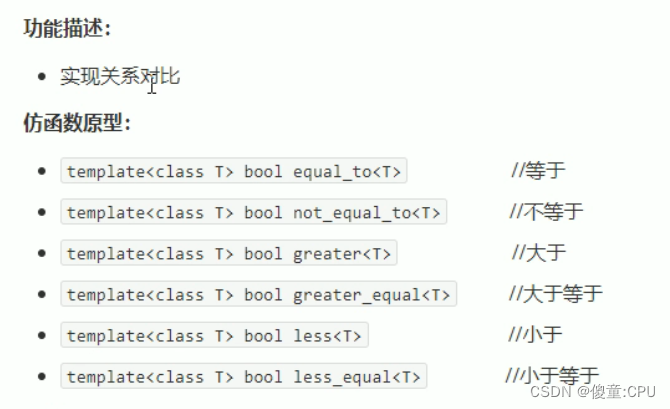
#include<iostream>
using namespace std;
#include<vector>
#include<algorithm>
#include<functional>//内建函数对象头文件
//内建函数对象 关系仿函数
//大于greater
class MyCompare
{
public:
bool operator()(int v1, int v2)
{
return v1 > v2;
}
};
void test()
{
vector<int>v;
v.push_back(10);
v.push_back(50);
v.push_back(20);
v.push_back(30);
v.push_back(40);
for (vector<int>::iterator it = v.begin();it != v.end();it++)
{
cout << *it << " ";
}
cout << endl;
//降序
//sort(v.begin(), v.end(), MyCompare());
sort(v.begin(), v.end(), greater<int>());
for (vector<int>::iterator it = v.begin();it != v.end();it++)
{
cout << *it << " ";
}
cout << endl;
}
int main()
{
test();
system("pause");
return 0;
}
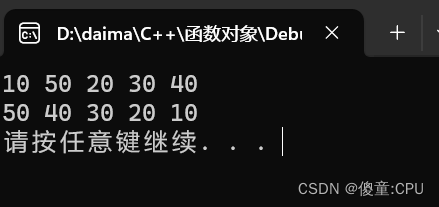
逻辑仿函数
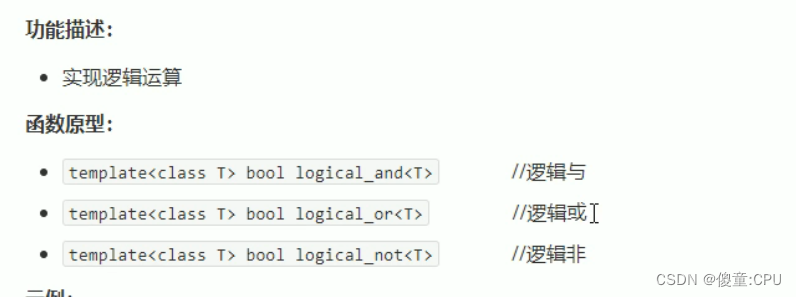
#include<iostream>
using namespace std;
#include<vector>
#include<algorithm>
#include<functional>//内建函数对象头文件
//内建函数对象 逻辑仿函数
//逻辑非logical_not
void test()
{
vector<int>v;
v.push_back(true);
v.push_back(false);
v.push_back(true);
v.push_back(false);
v.push_back(true);
for (vector<int>::iterator it = v.begin();it != v.end();it++)
{
cout << *it << " ";
}
cout << endl;
//利用逻辑非 将容器v 搬运到 容器v2中, 并执行取反操作
vector<int>v2;
v2.resize(v.size());
transform(v.begin(), v.end(), v2.begin(), logical_not<bool>());
for (vector<int>::iterator it = v2.begin();it != v2.end();it++)
{
cout << *it << " ";
}
cout << endl;
}
int main()
{
test();
system("pause");
return 0;
}
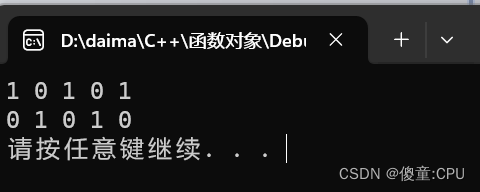