前言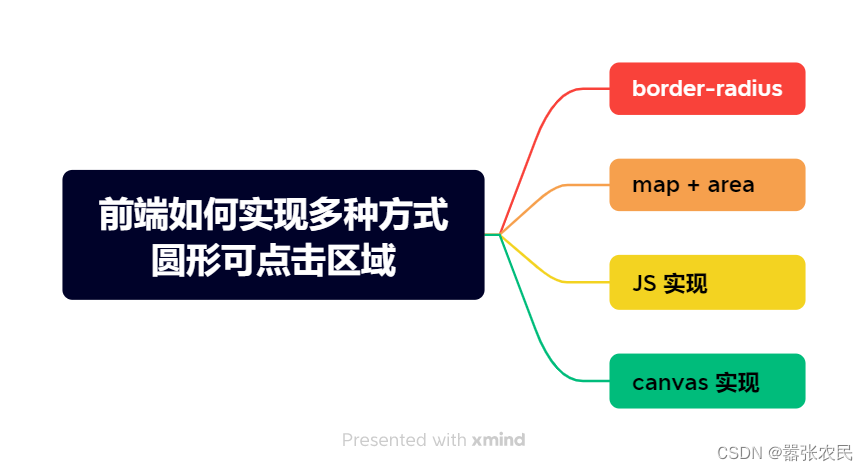
四种方式都可以实现在圆形区域内添加点击事件,选择哪种方式可以根据具体情况选择。其中使用canvas实现的方式可以更好地适用于需要绘制复杂图形的情况下。
方式一:border-radius
使用CSS的border-radius属性创建圆形区域,然后通过绑定点击事件实现可点击效果。
<style> #circle { background: red; width: 100px; height: 100px; border-radius: 50%; } </style> <div id="circle"></div> <script> document.querySelector("#circle").onclick = function () { alert("ok"); }; </script>
方式二:map + area
- map 标签用来定义一个客户端图像映射(带有可点击区域的一副图像);
- area 标签用来定义图像映射中的区域,area 元素永远嵌套在 map 元素内部;
<img src="xxxxx.png" usemap="#Map" /> <map name="Map" id="Map"> <area shape="circle" coords="100,100,50" href="https://www.yidengxuetang.com/" rel="external nofollow" target="_blank" /> </map>
area 属性
- shape:表示热点区域的形状,支持 rect(矩形),circle(圆形),poly(多边形)
- coords:表示热点区域的坐标,(0,0)表示图片左上角。rect 四个值分别表示左上角坐标和右下角坐标。circle 三个值分别表示圆心坐标和半径。poly 有若干个值,每两个表示一个坐标点。
- href:表示链接到某个地址,和
<a>
标签差不多 - alt:对该区域描述,类似于
<img>
的 alt
方式三: JS 实现
获取鼠标点击位置坐标,判断其到圆点的距离是否不大于圆的半径,来判断点击位置是否在圆内。
document.onclick = function (e) { let [x, y, r] = [100, 100, 100], // x,y 坐标原点,r半径 x1 = e.clientX, // 获取x坐标 y1 = e.clientY, // 获取y坐标 dis = Math.abs(Math.sqrt(Math.pow(x - x1, 2) + Math.pow(y - y1, 2))); if (dis < r) { alert("ok"); } };
方式四:canvas 实现
canvas 是 HTML5 提供的,用来在浏览器中创建图形,它可以通过 JavaScript 绘制 2D 图形。
因此我们可以通过用 canvas 在浏览器中绘制一个圆形,然后给这个圆形添加区域内点击事件即可实现。
<canvas id="drawing" width="400" height="400"></canvas> <script> window.onload = () => { let drawing = document.querySelector("#drawing"), ctx = drawing.getContext("2d"); circle = new Shape(100, 100, 50); circle.click = () => { alert("ok"); }; drawing.onmousedown = function (event) { let { clientX, clientY } = event || window.event, point = { x: clientX - drawing.offsetLeft, y: clientY - drawing.offsetTop, }; circle.reDraw(point); }; function Shape(x, y, r) { this.x = x; this.y = y; this.r = r; ctx.fillStyle = "red"; ctx.beginPath(); ctx.arc(this.x, this.y, this.r, 2 * Math.PI, false); ctx.closePath(); ctx.fill(); } Shape.prototype.reDraw = function (point) { ctx.fillStyle = "red"; ctx.beginPath(); ctx.arc(this.x, this.y, this.r, 2 * Math.PI, false); ctx.closePath(); ctx.fill(); // 判断点击是否在圆形区域内 if (ctx.isPointInPath(point.x, point.y)) { this.click(); } }; }; </script>