lesson5:
一、类的6个默认成员函数(2:29:10)
1.什么是空类?(2:29:40)
a.空类中真的什么都没有吗?(2:29:50)
①什么是默认成员函数?(2:30:0)
2.6个默认成员函数分别是谁?(2:30:15)
3.明明写了初始化函数,但经常忘记调用,如果忘记调用,如以下程序,就很可能会出现随机值或崩溃。
using namespace std;
class Date
{
public:
void Init(int year,int month,int day)
{
_year = year;
_month = month;
_day = day;
}
void Print()
{
cout << _year << "-" << _month << "-" << _day;
}
private:
int _year;
int _month;
int _day;
};
int main()
{
Date d1;
Date d2;
d1.Print();
d2.Print();
}
a.如果我想在调试的时候观察成员变量,方式是XX(2种)(2:35:50)
b.能不能保证对象一定被初始化?(2:38:50)
二、构造函数(2:40:0)
1.构造函数本质上是一个XX(2:40:0)
2.构造函数是开空间创建对象吗?(2:40:14)
3.构造函数的特征
a.构造函数的函数名和XX相同(2:41:35)
b.有返回值吗?(2:41:45)
①需要在函数名前面加个void吗?(2:41:55)
c.会在什么时候被自动调用?(2:42:10)
d.可以构成函数重载吗?(2:42:15)
e.有了构造函数,还需要Init()函数吗?(2:42:23)
4.构造函数如何传参?(2:43:35)
5.我定义对象时,不想使用构造函数传参,他能不能默认给我初始值?(2:43:55)
a.这个问题是如何被解决的?(2:44:5)
b.为什么它能默认把值给我的对象?(2:44:30)
4.如何理解构造函数的"特殊"?(2:53:35)
5.这种全缺省构造函数写法,构造函数会在对象实例化时自动调用吗?
class Date
{
public:
Date(int year=1, int month=1, int day=1)
{
_year = year;
_month = month;
_day = day;
}
private:
int _year;
int _month;
int _day;
};
int main()
{
Date d1;
}
a.无参调用时,它会和无参构造函数产生歧义吗?(2:56:10)
6.用构造函数完成栈的初始化(2:59:30)
7.如果类中没有显式定义构造函数,则C++编译器会做什么?(3:2:10)
a.不是说默认生成么,为什么一调试是这个结果?(3:6:0)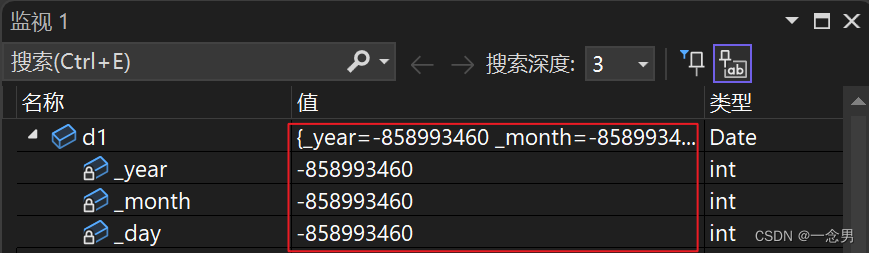
①默认生成的构造函数将C++类型分为什么?(3:7:0)
Ⅰ.哪些是内置类型?
Ⅱ.哪些是自定义类型?(3:7:30)
②默认生成的构造函数,它对自定义类型不做处理吗?(3:8:5)
③默认构造函数在什么时候会发挥作用?(3:10:30)
b.针对默认构造函数的缺陷,C++11打了一个补丁,这个补丁是XX(3:16:10)
①在类里面给成员变量赋值是初始化吗?(3:16:40)
②为什么?(3:22:30)
d.如果自定义类型成员对应的类没有写构造函数,那么会发生什么?(3:24:20)
7.默认构造函数就是我们不写,编译器自动生成的那个吗?(2:26:30)
a.默认构造的特点是什么?(3:27:45)
①下面的这个构造函数属于默认构造函数吗?(3:29:40)
typedef int DataType;
class Stack
{
public:
Stack(int capacity)
{
_array = (DataType*)malloc(sizeof(DataType) * capacity);
if (nullptr == _array)
{
perror("malloc申请空间失败!!!");
return;
}
_size = 0;
_capacity = capacity;
}
private:
DataType* _array;
int _capacity;
int _size;
};
lesson6:
一、
1.从汇编代码角度理解实例化时自动调用构造函数(0:15:50)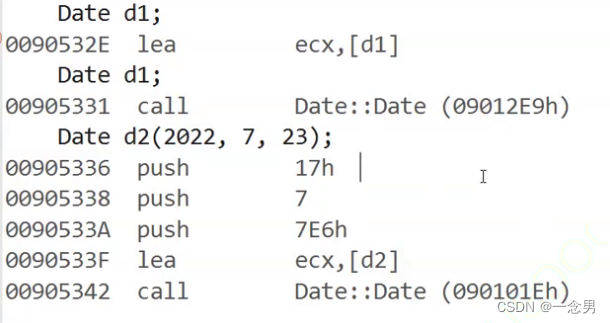
2.指针是内置类型吗?(0:31:25)
3. 一般的类都会让编译器默认生成构造函数吗?(0:37:30)
二、两个栈实现一个队列(0:39:0)(没看,以后补)
三、析构函数
1.析构函数的功能(0:45:30)
a.析构函数是对类对象本身的销毁吗?(0:46:0)
b.析构函数什么时候被调用?(0:49:10)
a.对象什么时候被销毁(0:49:37)
2.析构函数的特点(6点)(看课件)(0:50:25)
a.!是啥意思?(0:51:28)
b.~是啥意思?(0:51:40)
c.析构函数有形参吗?(0:52:0)
d.析构函数有返回值吗?(0:52:0)
e.一个类可以有多个析构函数吗?(0:52:10)
f.类Date有显式写析构函数的必要吗?为什么?(0:53:0)
class Date
{
private:
// 基本类型(内置类型)
int _year = 1970;
int _month = 1;
int _day = 1;
};
int main()
{
Date d;
return 0;
}
①如果不显式写析构函数,那么编译器会默认生成吗?(0:55:15)
Ⅰ.类Date不显式写析构函数时,默认生成的析构函数做了什么?(1:47:7)
g.类Stack有显式写析构函数的必要吗?(0:57:25)
typedef int DataType;
class Stack
{
public:
Stack(size_t capacity = 3)
{
_array = (DataType*)malloc(sizeof(DataType) * capacity);
if (NULL == _array)
{
perror("malloc申请空间失败!!!");
return;
}
_capacity = capacity;
_size = 0;
}
void Push(DataType data)
{
// CheckCapacity();
_array[_size] = data;
_size++;
}
// 其他方法...
~Stack()
{
if (_array)
{
free(_array);
_array = NULL;
_capacity = 0;
_size = 0;
}
}
private:
DataType* _array;
int _capacity;
int _size;
};
void TestStack()
{
Stack s;
s.Push(1);
s.Push(2);
}
int main()
{
TestStack();
}
①free后面的那两步赋0和置空有必要写吗?(0:57:53~0:58:30)
~Stack()
{
free(_array);
_capacity = _size = 0;
_array = nullptr;
}
h.有效的括号(1:2:0)(没看,以后看)
i.默认生成的析构函数的特点(1:11:25~1:15:10)
j.类Date默认生成的析构函数做了什么?(1:47:50)
class Time
{
public:
~Time()
{
cout << "~Time()" << endl;
}
private:
int _hour;
int _minute;
int _second;
};
class Date
{
private:
// 基本类型(内置类型)
int _year = 1970;
int _month = 1;
int _day = 1;
// 自定义类型
Time _t;
};
int main()
{
Date d;
return 0;
}
四、拷贝构造函数
1.我想让d2拷贝d1,怎么写?(2种)(1:55:18)
Date d1(2022, 7, 23);
Date d2 ?
a.为什么会有第二种写法?(1:55:45)
2.拷贝构造函数的特征(1:57:5)(2个)
a.拷贝构造是不是构造函数?(1:57:25)
b.它的形参怎么写?(1:58:10)
c.为什么会报这种错误?(2:0:0)
Date(Date d)
{
_year = d._year;
_month = d._month;
_day = d._day;
}

①为什么会引发无穷递归调用?(2:6: 50~2:10:0)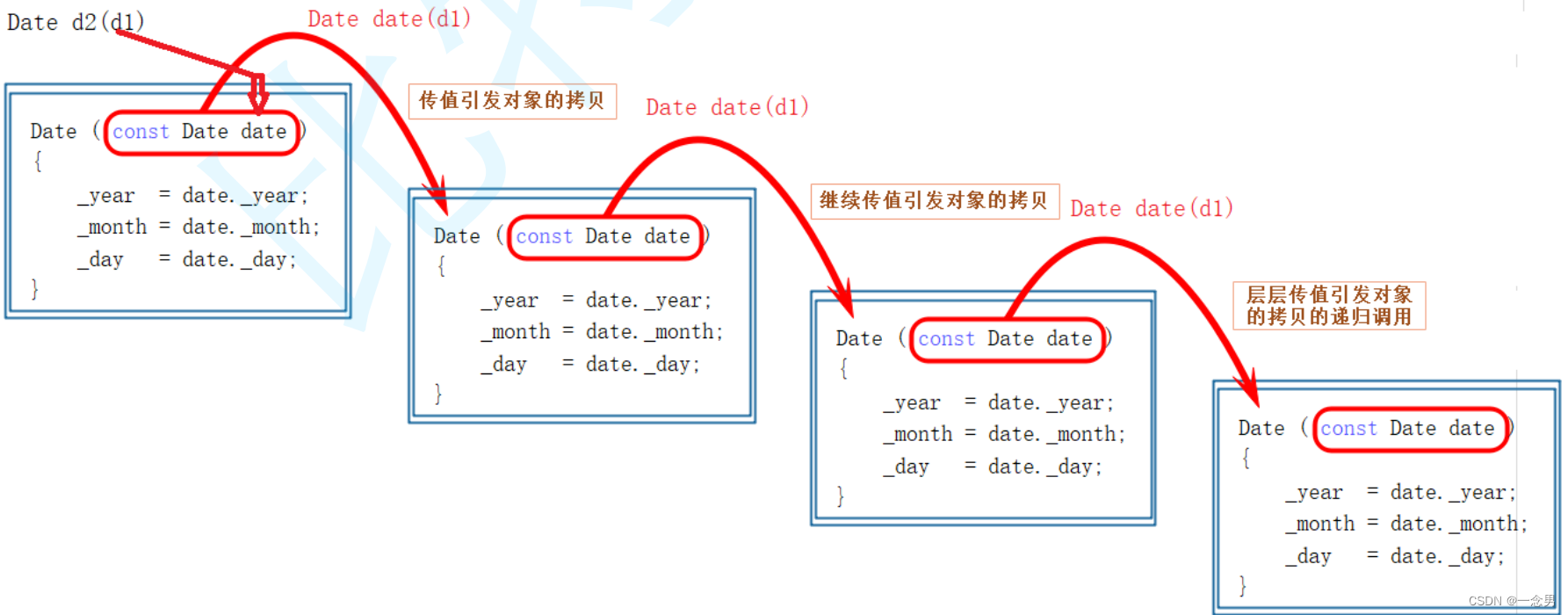
Ⅰ.调用拷贝构造前,要先XX(2:6:55)
Ⅱ.传参也是一个XX(2:7:2)
Ⅲ.在形参前面加const对刚刚的逻辑有影响吗?(2:7:28)
Ⅳ.为什么传参会引发拷贝构造?(2:7:57)
d.传值传参和传引用传参的区别?(2:2:30)
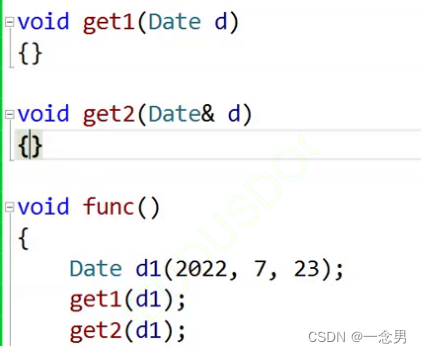
①get1函数的形参是Date d,Date d实例化时会XX(2:4:20)
②get2函数调拷贝构造了吗?(2:5:27)
e.可以用指针*代替引用&吗?(2:11:30)
①被代替后还叫拷贝构造函数吗?(2:11:45)
②被代替后初始化部分怎么写?(2:12:10)
Ⅰ.可以写成=吗?(2:13:50)
f.为什么要在形参前面加const?(2:22:30)
①权限是怎么变的?(2:24:15)
3.拷贝构造的应用场景(2:25:35 ~ 2:30:0)
4.若未显式定义,编译器会生成默认的拷贝构造函数吗?(2:41:10)
a.默认构造函数会处理内置类型吗?(2:43:0)
①什么是按照字节方式字节拷贝?(2:43:20)
b.默认拷贝构造函数怎么处理自定义类型?(2:43:40)
c.处理内置类型是浅拷贝还是深拷贝?(2:46:45)
①浅拷贝引发的问题(2个)(2:49:40)
五、运算符重载
1.下面日期类的运算都能运行成功吗?(2:56:25)
class Date
{
public:
Date(int year = 1, int month = 1, int day = 1)
{
_year = year;
_month = month;
_day = day;
}
private:
int _year;
int _month;
int _day;
};
int main()
{
Date d1(2022, 7, 23);
Date d2(2022, 7, 23);
//日期类的运算
d1 == d2;
d1 < d2;
d1++;
d1 + 100;
Date d3(2022, 10, 1);
d3-d2;
}
a.语言原生支持内置类型的运算符运算吗?(2:56:37)
①为什么?(2:56:55)
b.语言原生支持自定义类型运算符运算吗?(2:57:20)
①自己将其实现的过程叫做XX(2:58:27)
2.运算符重载是函数吗?(2:59:0)
a.函数名是什么?(2:59:17)
b.返回值类型由什么决定?(2:59:55)
c.形参的个数由什么决定?(3:0:34)
①谁是左/右操作数?(3:0:50)
3.下面实现d1==d2的运算符重载正确吗?(3:3:40)
class Date
{
public:
Date(int year = 1, int month = 1, int day = 1)
{
_year = year;
_month = month;
_day = day;
}
private:
int _year;
int _month;
int _day;
};
bool operator==(Date x1, Date x2)
{
return x1._year == x2._year
&& x1._month == x2._month
&& x1._day == x2._day;
}
a.解决方案(4种)(3:4:12)
①为什么不建议用友元(3:4:45)
b.为什么cout << d1==d2 << endl 会报错?(3:5:27)
c.d1==d2可以等价写成XX(3:6:48)
d.这种写法会调用拷贝构造吗?(3:10:19)
bool operator==(Date x1, Date x2)
{
return x1._year == x2._year
&& x1._month == x2._month
&& x1._day == x2._day;
}
①怎么优化?(3:10:30~3:11:0)
4.为什么我把这个运算符重载写到类里面,让它成为成员函数,居然会报错?(3:13:40~3:14:20)

a.调用时可以写成这样吗?(3:15:10)
d1.operator==(&d1, d2);
5.可不可以由对象找成员变量改成用域作用限定符找成员变量?(3:16:47)
bool operator==(Date x1, Date x2)
{
return x1._year == x2._year
&& x1._month == x2._month
&& x1._day == x2._day;
}
6.实现日期类的'+天数'(3:22:0)
a.日期+天数会涉及进位,但进位的前提是我得知道当前进位的第n月有多少天。怎么知道第n月有多少天?(3:25:45)
①为什么光给一个月份不能保证获得的天数一定是正确的?(3:26:17)
b.如何将switch替换掉,更简便的知道第几个月有几天?(3:27:5)
c.if的判断条件只是判断是否是闰年吗?(3:28:5)
①闰年的判断条件
d.为什么要将数组用static修饰?(3:28:45)
e.进位只考虑进月份吗?(3:34:45)
f.返回在类型是Date,return 返回什么才符合要求?(3:35:55)
g.d+50就把对象d给变了,合理吗?(3:38:20)
h.如果我要拿d+50的返回值,应该怎么做?(3:38:50~3:39:25)
i.实际上我们刚刚写的函数是XX(3:39:35)
j.怎么写+的运算符重载?
lesson7:
一、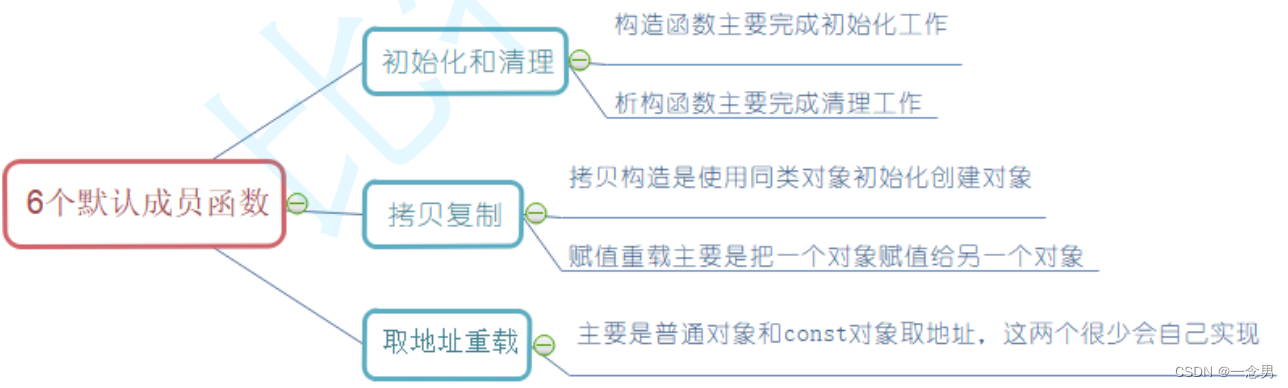
1.构造函数(3点)
a.大部分的类都需要自己写构造函数吗?
b.只有像XX这样的类不需要显式写构造函数
c.为什么每个类最好都要提供默认构造函数?(0:7:25)
2.析构函数(3点)
a.如何理解'清理'(0:9:30)
b.所有类都需要显式写析构吗?(0:11:20 )
c.构造和析构的顺序是先定义的先构造先析构吗?(0:15:45)
#include <iostream>
using namespace std;
class A
{
public:
A(int a = 0)
:_a(a)
{
cout << "A()->" << _a << endl;
}
~A()
{
cout << "~A()->" << _a << endl;
}
private:
int _a;
};
int main()
{
A a1(1);
A a2(2);
}
①为什么是这个顺序?(0:16:28)
Ⅰ.main函数调用函数f1,f1调用函数f2后销毁的顺序(0:18:52)
Ⅱ.栈区和栈结构的性质是一样的吗?(0:20:35)
②析构是销毁类对象的空间吗?(0:25:35)
Ⅰ.对象空间的创建和销毁是随着XX走的(0:28:30)
Ⅱ.如果类对象随函数结束而销毁了,但是销毁前没有用析构函数清理指向堆区的空间,会发生什么后果?(0:28:45)
Ⅲ.为什么日期类不需要析构函数清理?(0:31:10)
d.构造和析构的顺序(0:3)
#include <iostream>
using namespace std;
class A
{
public:
A(int a = 0)
:_a(a)
{
cout << "A(int a=0)->" << _a << endl;
}
~A()
{
cout << "~A()->" << _a << endl;
}
private:
int _a;
};
A a3(3);
int main()
{
static A a0(0);
A a1(1);
A a2(2);
static A a4(4);
}
①为什么全局对象a3在调用main函数之前就要调用构造?(0:37:0)
②为什么是a1和a2最先析构?(0:39:15)
③除了栈区以外,其它区也符合先定义的后析构吗?(0:40:30)
e.构造和析构的顺序(0:45:0)
class A
{
public:
A(int a = 0)
:_a(a)
{
cout << "A(int a=0)->" << _a << endl;
}
~A()
{
cout << "~A()->" << _a << endl;
}
private:
int _a;
};
A a3(3);
void f()
{
static A a0(0);
A a1(1);
A a2(2);
static A a4(4);
}
int main()
{
f();
f();
}
3.拷贝构造
a. C++将&符号带进函数名修饰规则里了吗?(即C++能区分两个f()函数吗?)(1:1:40)
class A
{
public:
A(int a = 0)
:_a(a)
{
cout << "A(int a=0)->" << _a << endl;
}
~A()
{
cout << "~A()->" << _a << endl;
}
private:
int _a;
};
void f(A aa)
{}
void f(A& aa)
{}
int main()
{
A a1(1);
f(a1);
}
①f(A& aa)里面的形参构成引用了吗?(1:2:25)
b.构造和析构的顺序
#include<iostream>
using namespace std;
class A
{
public:
A(int a = 0)
:_a(a)
{
cout << "A(int a=0)->" << _a << endl;
}
A(const A& aa)
{
_a = aa._a;
cout << "A(const A& aa)->" << _a << endl;
}
~A()
{
cout << "~A()->" << _a << endl;
}
private:
int _a;
};
A func3()
{
static A aa(3);
return aa;
}
A& func4()
{
static A aa(4);
return aa;
}
int main()
{
func3();
cout << endl << endl;
func4();
}
①cout << endl << endl的作用(1:5:40)
②为什么运行结果第二行是A(const A& aa)->3?(1:6:45)
③第三行~A()->3析构的是谁?(1:7:25)
Ⅰ.它的生命周期呢?
④对于func4()函数,如果aa出了作用域就销毁,那么引用返回有问题吗?(1:13:30)
4.运算符重载
a.所有的类都需要写拷贝构造和赋值运算符重载吗?(2:18:30)
二、Date类的实现
1.为什么要将构造函数的定义写在.h文件里面?(1:34:30)
#pragma once
class Date
{
public:
Date(int year = 1900, int month = 1, int day = 1)
{
_year = year;
_month = month;
_day = day;
}
private:
int _year;
int _month;
int _day;
};
2.我在类里面没有写拷贝构造,会发生什么?(1:35:45)
Date d1(2022, 7, 24);
Date d2(d1);
a.对于在栈区的类,会发生什么?(1:36:10)
3.如何实现将d3的值赋给d1?(1:38:30)
Date d1(2022, 7, 24);
Date d3(2022, 8, 24);
d1 = d3;
a.赋值运算符支持连续赋值吗?(1:43:10)
①i=j=10,j=10的返回值是什么?(1:44:35)
b.为什么返回值类型要写成Date ?(1:45:40)
①出了operator函数的作用域,*this还在吗?(1:46:30)
Ⅰ.为什么还要将返回值类型改成Date& ?(1:47:5)
Ⅱ.返回值类型和形参类型Date有无&运行的区别(1:49:30~1:50:10)
c.如果写了d2=d2函数怎么修改?(1:51:45)
Ⅰ.为什么不能写成(*this) != d ?(1:52:15)
4.赋值运算符的重载格式(4条)(1:54:10)
5.赋值运算符能将函数重载成全局函数吗?(1:55:45~1:56:30)
6.我们没有显式写赋值运算符的重载时,编译器会默认生成吗?(1:56:52)
a.内置类型和自定义类型分别怎么处理?(1:57:20)
①没有写=的运算符重载,下面能实现连续赋值吗?(1:57:50)
class Date
{
public:
Date(int year = 1900, int month = 1, int day = 1)
{
_year = year;
_month = month;
_day = day;
}
private:
int _year;
int _month;
int _day;
};
int main()
{
Date d1(2023, 8, 29);
Date d2;
Date d3;
d3 = d2 = d1;
}
②自定义类型的_t会赋值成功吗?(1:59:30)
class Time
{
public:
Time()
{
_hour = 1;
_minute = 1;
_second = 1;
}
Time& operator=(const Time& t)
{
if (this != &t)
{
_hour = t._hour;
_minute = t._minute;
_second = t._second;
}
return *this;
}
private:
int _hour;
int _minute;
int _second;
};
class Date
{
public:
Date(int year = 1900, int month = 1, int day = 1)
{
_year = year;
_month = month;
_day = day;
}
private:
int _year;
int _month;
int _day;
//自定义类型
Time _t;
};
int main()
{
Date d1(2023, 8, 29);
Date d2;
Date d3;
d3 = d2 = d1;
}
③为什么说MyQueue是一个躺赢的类?(2:3:40)
class MyQueue
{
private:
Stack _st1;
Stack _st2;
};
7.写一个>运算符的重载(2:29:10)
a.还能如何改进?(2:30:15)
8.任何一个类,只需要写XX,剩下的比较运算符重载复用即可(2:34:20)
9.>=是>&&=还是>||=?(2:35:35)
10.<是!>还是! >= ?(2:36:55)
11.一个类可以重载哪些运算符?(2:41:25)
12.这两种写法从效率的角度看,有没有区别?(2:50:10)
1.month == 2 && ((year % 4 == 0 && year % 100 != 0) || (year%400 == 0))
2.(year % 4 == 0 && year % 100 != 0) || (year%400 == 0 && month == 2)
a.哪种效率更高?(2:51:10)
13.先写谁用来复用更好?(2:52:25)
Date operator+(int day)
{}
Date operator+=(int day)
{}
a.+=会出现连续+=吗?(2:53:8)
14.这种写法是赋值还是拷贝构造?(2:57:50)
Date ret = *this;
a.什么情况下才是赋值?(2:58:16)
15.类中的+复用了+=,但是+=在+函数的下面,需要将它们调换顺序吗?(2:59:40~3:0:45)
class Date
{
Date operator+(int day)
{
//复用了+=
}
Date& operator+=(int day)
{
//实现
}
};
16.更简便的等价写法是什么?(3:8:0)
Date d2 = d1 + 4;
d2.Print();
17.这是前置++还是后置++?(3:18:40~3:18:35)
Date operator++()
a.C++为了处理这个问题,做了什么?(3:19:50)
①默认是前置还是后置?(3:20:0)
②后置的这个参数需要形参接收吗?(3:20:20)
Ⅰ.这个参数的作用?(3:22:25)
b.编译器在调用的地方是怎么处理的?(3:21:15)
c.前置和后置--也是这样吗?(3:26:40)
18.他俩构成运算符重载了吗?(3:29:10)
//d1 - 100
Date& operator-(int day)
{}
//d1 - d2
int operator(const Date& d)
{}
lesson8:
一、
1.假如我在调用Date类的构造函数时,初始化了一个不合理的年月份数据,那么如何解决?(0:40:0)
a.在类里面调用成员函数时,默认会在前面加this->吗?(0:40:30)
b.更粗暴的方案?(0:42:30)
2.如果出现了以下情况,等价于什么?(0:57:45)
Date d(2023, 8, 29);
d -= -100;
3.cin和cout本质上是什么?(1:1:40)
a.cout << 是如何自动识别类型的?(1:2:55)
①cout << i等价于什么?(1:3:20~1:4:50)
b.能够直接使用的原因?(1:6:40)
4.运算符重载和函数重载
①运算符重载(1:7:50)
②函数重载(1:8:40)
③运算符重载和函数重载名字里都有重载,那么他们有关系吗?(1:9:40)
5.我想让a的功能等价b,怎么写它的运算符重载?(1:13:20)
a.d1 + 4.Print();
b.cout<< (d1+4);
a.cout是ostream类的对象,我们可以直接去修改库的类,将ostream&operator<< (Date val)添加到类ostream里面作为成员函数吗?(1:13:55)
①添加不进去的话,应该怎么办?(1:14:23)
b.这里的参数应该是Date还是ostream?(1:37:40)
operator<<(?)
①out是什么?(1:39:20)
c.为什么会报错?
void Date::operator<<(ostream& out)
{
cout << _year << "-" << _month << "-" << _day << endl;
}
cout << (d1 - 100);
①只要涉及XX,C++的报错就会很多很长(1:40:35)
②我们不是已经写了>>运算符重载了吗,为什么调用的时候会找不到?(1:41:0)
Ⅰ.d1.operator<<(cout)能调到吗?(1:41:35)
Ⅱ.运算符有多个操作数的时候,第一个参数是谁?(1:42:22)
Ⅲ.d1.operator<<(cout)等价于XX(1:42:40)
d.如何修改,使其对应cout << d1?(1:45:10)
e.全局函数无法访问私有,怎么办?(2种)(1:46:30)
①什么是友元函数?(1:47:50)
Ⅰ.友元函数写在任意位置都可以吗?(1:47:30)
f.如何让它支持连续提取?(1:53:0)
cout << d1 << d2;
g.流提取重载中,Date& d前面应不应该加const修饰?(1:57:10)
h.cin>>流提取时,我们输入空格和换行等价吗?(2:0:30)
i.cin>>d1>>day 其中两次>>都是调用我们写的自定义运算符重载吗?(2:5:40)
二、运算符重载的规则
1.operator@可以重载吗?(2:50:0)
2.重载操作符必须有一个类类型参数吗?(2:50:30)
3.可以改变内置类型的运算符含义吗?(2:50:35)
4.作为类成员函数重载时,其形参看起来比操作数数目少1,是因为XX
5.哪几个运算符不能重载?(5个)(2:51:5)
三、const成员函数
1.这种写法有问题吗?(2:55:40)
const Date d1(2022, 7, 25);
d1.Print();
a.Date* 能传给Date* const 吗?(2:58:30)
b.&d1的类型是什么?(2:59:15)
c.const Date* 能传给Date* const 吗?(2:59:39)
2.为什么d1 < d2就可以,d2 < d1就报错?(3:0:50)
Date d1(2022, 7, 25);
const Date d2(2022, 7, 25);
d1 < d2;
d2 < d1;
3.如何将隐含的this指针由Date* const类型变成const Date* const类型?(3:3:15~3:4:40)
a.const修饰的是指针本身吗?(3:6:35)
b.const Date* const this不能修改this指针指向的内容,那么能修改this指针本身吗?(3:7:5)
①const放在*之前和之后的作用(3:7:45)
c.只要XX与XX之间才存在权限的放大和缩小(3:8:45)
①这是权限的放大吗?(3:9:10~3:9:45)
int i = 10;
d.这里的const修饰的是什么?(3:12:15)
void Date::Print()const
{
//...
}
①作用是什么?(3:12:40)
e.能运行成功吗?(3:16:30)
class A
{
public:
A Print()const
{
//...
}
};
A d1;
const A d2;
d1.Print();
d2.Print();
f.他俩构成函数重载吗?(3:17:28)
A Print()const
{
//...
}
A Print()
{
//...
}
①编译器在调用的时候,优先调用哪个?(3:17:45)
g.什么情况下需要他俩同时存在?(3:18:7)
A* operator&()
{
return this;
}
const A* operator&()const
{
return this;
}
①只写第二个重载,有问题吗?(3:18:25)
②他俩是默认成员函数吗?(3:20:25)
四、求1+2+3+...+n_牛客题霸_牛客网 (nowcoder.com)

1.for、while对应什么解决方案?(3:25:0)
2.if、else对应什么解决方案?(3:25:10)
3.乘除法对应什么解决方案?(3:25:40)
4.乘除法可以被XX代替(3:25:58)
5.牛客上面支持变长数组吗?(3:28:0)
6.对象实例化的时候,会自动调用它的构造函数,以下对象实例化会调用几次?(3:28:35)
class sum
{
sum()
{
//...
}
};
int main()
{
sum a[n]
}
7.为确保每次调用构造函数时,sum都不是从0开始,而是存储的上次累加结果,那么该如何处理sum?(3:29:0)
class Sum
{
public:
Sum()
{
static int count=0;
count++;
sum+=count;
}
};