一、新建节点
1.在Start场景中新建Music节点,绑定canvas
2.在Game场景中新建Music节点
3.新建节点
4.新建Music脚本,绑定Canvas
Music.ts
const { ccclass, property } = cc._decorator;
@ccclass
export default class NewClass extends cc.Component {
@property({ type: cc.AudioSource, displayName: "背景音乐", tooltip: "背景音乐" })
bg_music: cc.AudioSource = null;
@property({ type: cc.AudioSource, displayName: "碰撞音效", tooltip: "碰撞音效" })
collision: cc.AudioSource = null;
@property({ type: cc.AudioSource, displayName: "buff音效", tooltip: "buff音效" })
buff: cc.AudioSource = null;
@property({ type: cc.AudioSource, displayName: "按钮音效", tooltip: "按钮音效" })
btn: cc.AudioSource = null;
//播放碰撞音效
play_music_collision(){
this.collision.play();
}
//播放buff音效
play_music_buff(){
this.buff.play();
}
//播放点击按钮音效
play_music_btn(){
this.btn.play();
}
}
二、引用
1.Barrier.ts
//碰撞回调
//当碰撞产生的时候调用other产生碰撞的另一个组件 self产生碰撞的自身的碰撞组件
onCollisionEnter(other, self) {
if (other.node.group == "bullet") {
//获取GameController脚本
let gc = cc.find("/Canvas").getComponent("GameController") as GameController;
//获取Bullet脚本
let c = other.getComponent("Bullet");
//从脚本获取攻击力较少自身生命值
this.reduce_num(c.ATK);
// 如果可以加双倍分数
if (gc.is_double == true) {
//加分
gc.add_score((c.ATK) * 2)
}
//如果不可以加双倍分数
if (gc.is_double == false) {
gc.add_score(c.ATK);
}
//销毁子弹
other.node.destroy();
}
//发生了碰撞,无敌
if (other.node.group == "invincible") {
//获取GameController脚本
let gc = cc.find("/Canvas").getComponent("GameController") as GameController;
//如果可以加双倍分数
if (gc.is_double == true) {
//加双倍分
gc.add_score(this.num * 2);
}
//如果不可以加双倍分
else if (gc.is_double == false) {
gc.add_score(this.num);
}
//自身销毁
this.node.destroy()
}
//播放碰撞音效
let music = cc.find("Canvas").getComponent("Music");
music.play_music_collision();
//如果自身生命值小于0
if (this.num <= 0) {
//自身销毁
this.node.destroy();
}
}
2.Buff.ts
//碰撞回调
onCollisionEnter(other,self){
//获取GameController脚本
let gc = cc.find("Canvas").getComponent("GameController") as GameController;
//如果子弹射速满了,就不增加射速了
if(gc.bullet_speed<10000){
//随机数0-10
let n = this.randomNumber(0,10);
//有一半几率执行子弹加速函数
//也有一半几率执行子弹加攻击函数
if(n>5){
//加速buff
gc.enhance_speed();
}else{
//加攻击buff
gc.enhance_ATK();
}
}else{
gc.enhance_ATK()
}
//自身销毁
this.node.destroy()
//播放音效
let music = cc.find("Canvas").getComponent("Music");
music.play_music_buff();
}
三、菜单修改音乐音量
1.新建容器节点sound0
2.新建滑动器节点
Game_Menu.ts
@property({ type: music, displayName: "Music脚本所在节点", tooltip: "Music脚本所在节点" })
music: music = null;
@property({type: cc.Slider, displayName: "背景音乐音量滑动器", tooltip: "背景音乐音量滑动器"})
sound_sd0: cc.Slider = null;
//滑动背景音乐滑动器时调用的函数
set_sound0() {
//把滑动器的值赋给音乐
this.music.bg_music.volume = this.sound_sd0.progress;
}
3.绑定canvas
四、菜单修改音效音量
1.新建容器节点sound1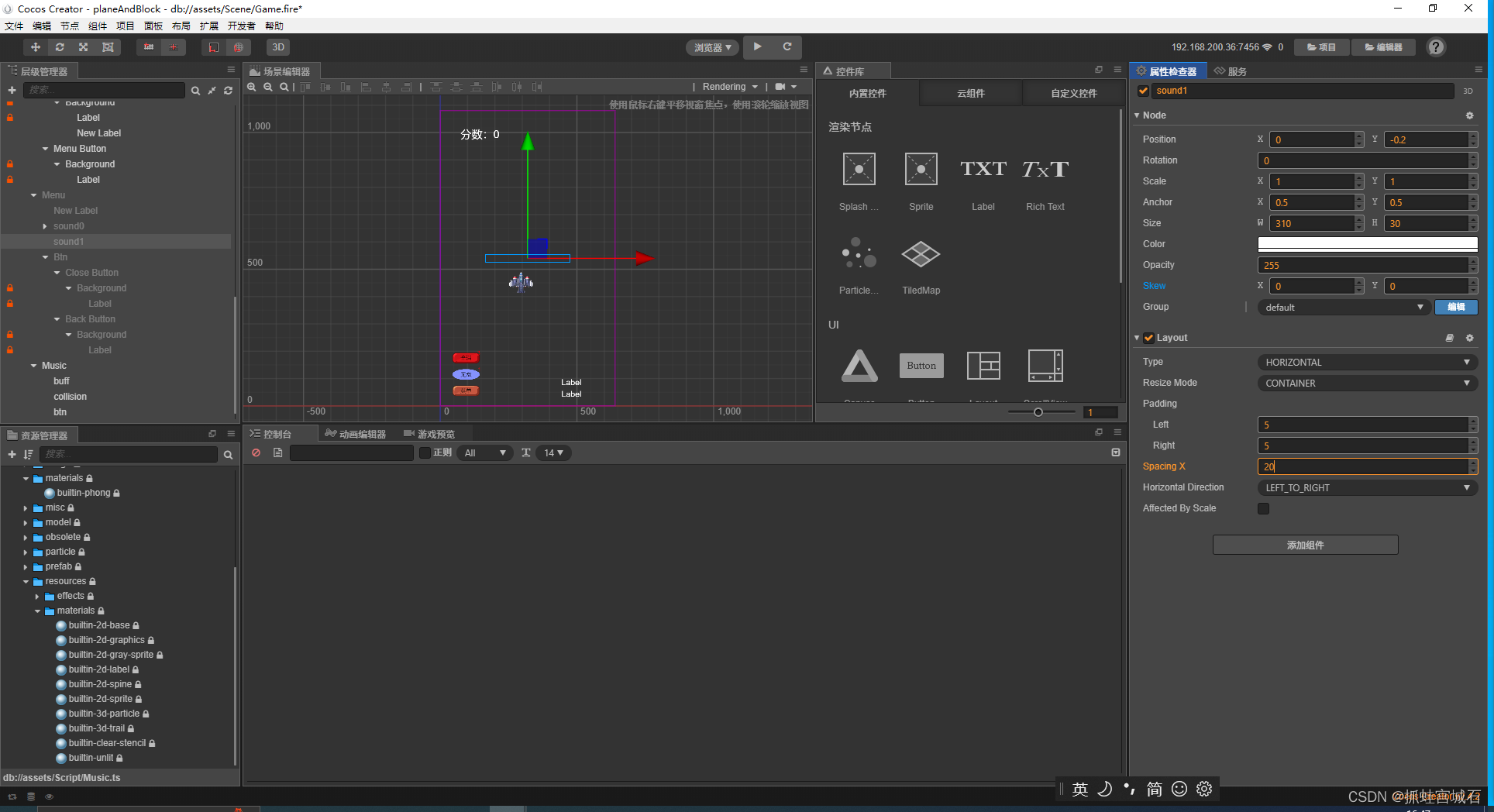
2.新建滑动器节点
Game_Menu.ts
@property({ type: cc.Slider, displayName: "音效音量滑动器", tooltip: "音效音量滑动器" })
sound_sd1: cc.Slider = null;
//滑动音效滑动器时调用的函数
set_sound1() {
//把滑动器的值赋给音乐
this.music.collision.volume = this.sound_sd1.progress;
this.music.buff.volume = this.sound_sd1.progress;
this.music.btn.volume = this.sound_sd1.progress;
}