目录
- vue3+h5实现虚拟列表
- component / List.vue
- component / itemComponent.vue
- 使用组件
- 效果
vue3+h5实现虚拟列表
- 安装:
npm i vue3-virtual-scroll-list
- package.json
"dependencies": {
"vue": "^3.2.36",
"vue3-virtual-scroll-list": "^0.2.1"
},
"devDependencies": {
"vite": "^4.4.6",
"vite-plugin-mock": "^3.0.0",
"vite-plugin-style-import": "^2.0.0",
"vite-plugin-vconsole": "^2.0.0",
}
component / List.vue
<template>
<div class="container">
<vue-virtual-scroll-list
class="scroll-list"
style="height: 100%; overflow-y: scroll;"
:size="list.length"
:keeps="20"
:data-key="'id'"
:data-sources="list"
:data-component="itemComponent"
:extra-props="{
address,
basisInfo,
}"
@tobottom="listToBottom"
>
</vue-virtual-scroll-list>
</div>
</template>
<script>
import { defineComponent, ref } from 'vue';
import VueVirtualScrollList from 'vue3-virtual-scroll-list';
import itemComponent from "./itemComponent.vue"
export default defineComponent({
components: {
VueVirtualScrollList,
},
setup() {
const list = ref([]);
const listToBottom = () => {
console.log('到底了哦');
}
const content = ()=> {
for(let i = 0; i < 100; i++){
const obj = {id: i, name: `人员${i}`, sex: i % 2 === 0 ? '男' : '女'};
list.value.push(obj)
}
}
onMounted(()=>{
content()
})
return {
list,
itemComponent,
address:{ province:'江苏',city:'南京' },
basisInfo:[{age:20}],
listToBottom
};
},
});
</script>
<style>
.container {
height: 500px;
}
.scroll-list {
width: 100%;
}
.list-item {
height: 50px;
line-height: 50px;
border-bottom: 1px solid #ccc;
}
.container ::-webkit-scrollbar {
width: 0px;
background-color: transparent;
}
.container ::-webkit-scrollbar-thumb {
background-color: transparent;
}
.container ::-webkit-scrollbar-track {
background-color: transparent;
}
.container {
scrollbar-width: thin;
scrollbar-color: transparent transparent;
}
</style>
component / itemComponent.vue
<template>
<div>
编号:{{ index }} <br/>
姓名:{{ source.name }}<br/>
年龄:{{basisInfo[0].age}}<br/>
地址:{{address.province}}-{{address.city}} <br />
<div v-if="source.sex === '男'">
{{ source.sex }}
</div>
<hr/>
</div>
</template>
<script>
export default {
name: 'item-component',
props: {
index: {
type: Number
},
source: {
type: Object,
default () {
return {}
}
},
address:{
type: Object,
default () {
return {}
}
},
basisInfo:{
type: Array,
default () {
return []
}
}
},
mounted(){}
}
</script>
使用组件
<List></List>
import List from "./component/List.vue"
export default {
name: "AboutPage",
components: {
List
},
}
效果
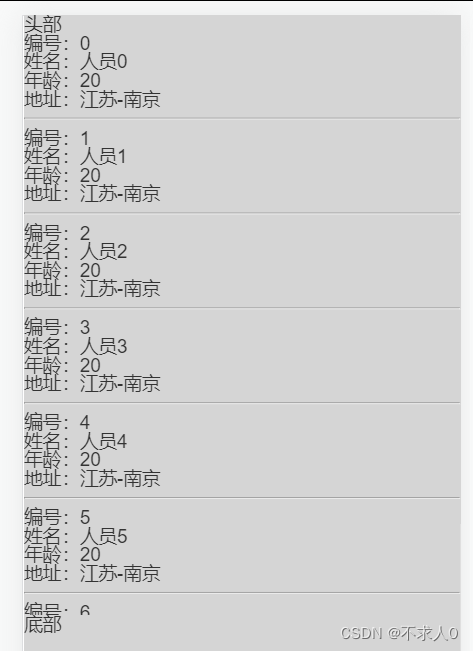