Vue中想用组件总共分几步:
1.创建组件
2.注册组件
3.使用组件
1.创建组件
//1.创建school组件,这里的school并不是组件名,只是一个中转变量名
const school = Vue.extend({
// el:'#root', //组件定时,一定不要写el配置项,因为最终所有的组件都要被一个vm管理,由vm决定服务于哪个容器
data(){
return{
schoolName:'山河大学',
address:'山东济南',
}
}
})
//创建student组件
const student = Vue.extend({
data(){
return{
studentName:'张三',
age:19,
}
}
})
2.注册组件
//创建vm
new Vue({
el:'#root',
components:{
//第二步,注册组件(局部注册)
//key:value 其中key是最终组件名,value是之前定义的中转变量名字
xueiao:school,
xuesheng:student,
}
})
3.使用组件
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<script type="text/javascript" src="js/vue/vue.js"></script>
</head>
<body>
<div id="root">
<xuexiao></xuexiao>
<hr/>
<xuesheng></xuesheng>
</div>
</body>
<script>
//1.创建school组件,这里的school并不是组件名,只是一个中转变量名
const school = Vue.extend({
template:`
<div>
<h2>学校名称:{{schoolName}}</h2>
<h2>学校地址:{{address}}</h2>
</div>
`,
// el:'#root', //组件定时,一定不要写el配置项,因为最终所有的组件都要被一个vm管理,由vm决定服务于哪个容器
data(){
return{
schoolName:'山河大学',
address:'山东济南',
}
}
})
//创建student组件
const student = Vue.extend({
template: `
<div>
<h2>学生姓名:{{studentName}}</h2>
<h2>学生年龄:{{age}}</h2>
</div>
`,
data(){
return{
studentName:'张三',
age:19,
}
}
})
//创建vm
new Vue({
el:'#root',
components:{
//第二步,注册组件(局部注册)
//key:value 其中key是最终组件名,value是之前定义的中转变量名字
xuexiao:school,
xuesheng:student,
}
})
</script>
</html>
全局组件:
<body>
<div id="root">
<hello></hello>
<xuexiao></xuexiao>
<hr/>
<xuesheng></xuesheng>
</div>
<div id="root2">
<hr/>
<hello> </hello>
</div>
</body>
<script>
//第一步,创建school组件,这里的school并不是组件名,只是一个中转变量名
const school = Vue.extend({
template:`
<div>
<h2>学校名称:{{schoolName}}</h2>
<h2>学校地址:{{address}}</h2>
<button @click="showName">点我提示学校名</button>
</div>
`,
// el:'#root', //组件定时,一定不要写el配置项,因为最终所有的组件都要被一个vm管理,由vm决定服务于哪个容器
data(){
return{
schoolName:'山河大学',
address:'山东济南',
}
},
methods:{
showName(){
alert(this.schoolName)
}
}
})
//创建student组件
const student = Vue.extend({
template: `
<div>
<h2>学生姓名:{{studentName}}</h2>
<h2>学生年龄:{{age}}</h2>
</div>
`,
data(){
return{
studentName:'张三',
age:19,
}
}
})
//创建hello组件
const hello = Vue.extend({
template:`
<div>
<h2>你好啊!{{name}}</h2>
</div>
`,
data(){
return {
name:'tom'
}
}
})
//第二步:全局注册组件
Vue.component('hello',hello)
//创建vm
new Vue({
el:'#root',
components:{
//第二步,注册组件(局部注册)
//key:value 其中key是最终组件名,value是之前定义的中转变量名字
xuexiao:school,
xuesheng:student,
}
})
new Vue({
el:'#root2'
})
</script>
总结: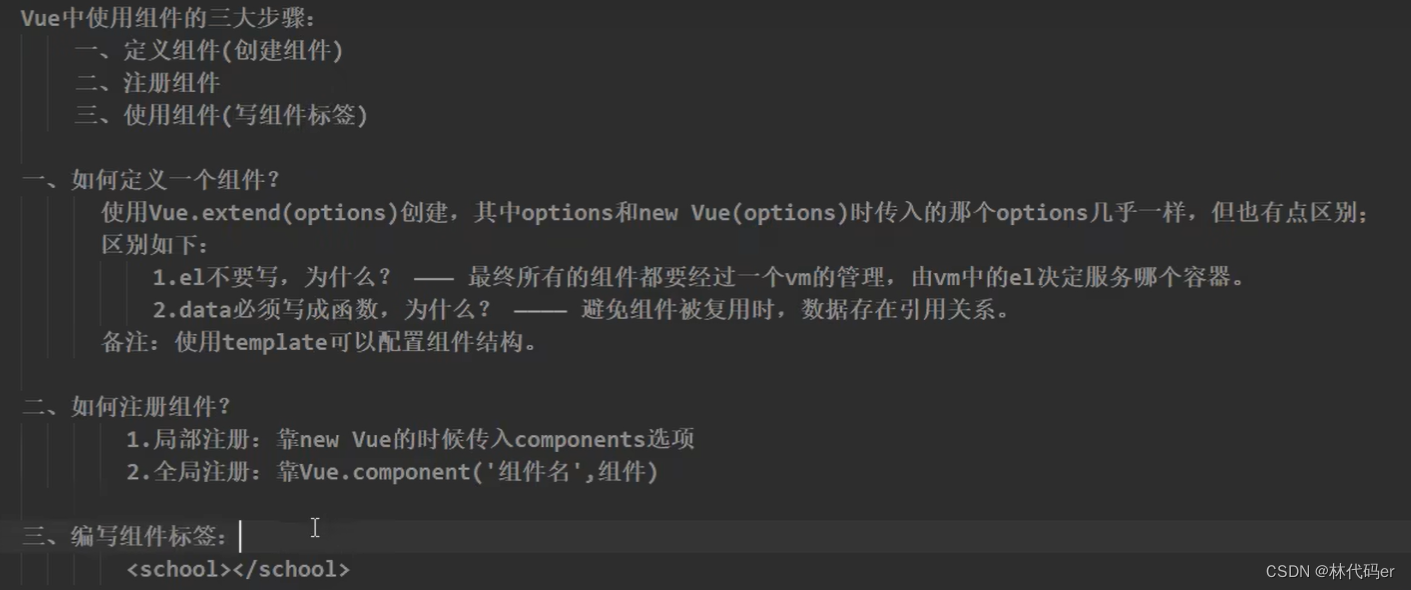