目录
1、边缘检测原理
2、Sobel算子边缘检测
3、Scharr算子边缘检测编辑
4、算子生成函数
5、Scharr、Sobel的使用
6、Laplacian算子边缘检测
7、Canny算子边缘检测
8、Laplacian、Canny的使用
1、边缘检测原理
2、Sobel算子边缘检测
3、Scharr算子边缘检测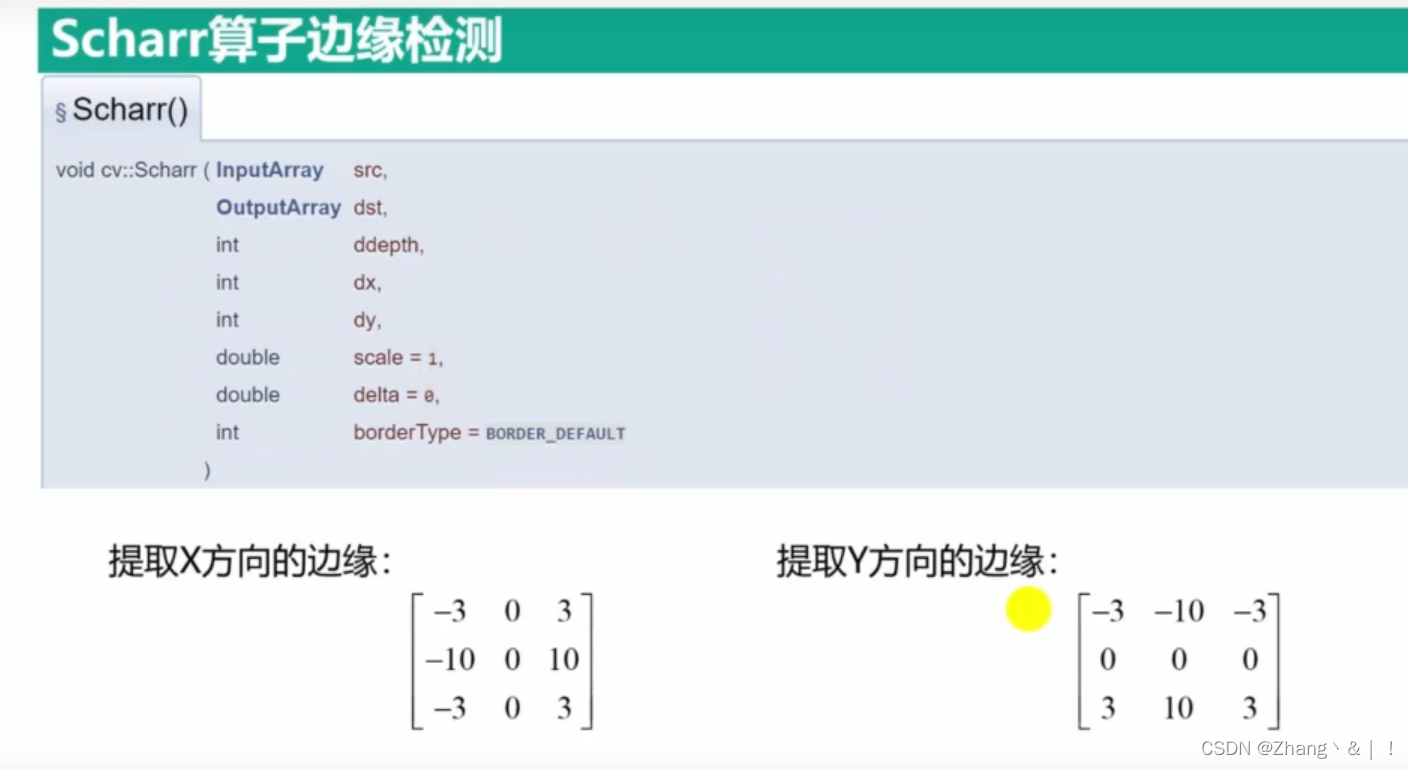
4、算子生成函数
5、Scharr、Sobel的使用
//边缘检测一
int test1()
{
//读取图像,黑白图像边缘检测结果较为明显
Mat img = imread("F:/testMap/equalLena.png", IMREAD_ANYCOLOR);
if (img.empty())
{
cout << "请确认图像文件名称是否正确" << endl;
return -1;
}
Mat resultX, resultY, resultXY;
//X方向一阶边缘
Sobel(img, resultX, CV_16S,2,0,1);
convertScaleAbs(resultX, resultX);
//Y方向一阶边缘
Sobel(img,resultY, CV_16S,0,1,3);
convertScaleAbs(resultY, resultY);
//整幅图像的一阶边缘
resultXY = resultX + resultY;
//显示图像
imshow("resultx", resultX);
imshow("resultY", resultY);
imshow("resultXY", resultXY);
cout << "接下来进行Scharr边缘的检测" << endl;
waitKey(0);
//X方向一阶边缘
Scharr(img,resultX, CV_16S,1,0);
convertScaleAbs(resultX, resultX);
//Y方向一阶边缘I
Scharr(img,resultY, CV_16S,0,1);
convertScaleAbs(resultY,resultY);
//整幅图像的一阶边缘
resultXY = resultX + resultY;
//显示图像
imshow("resultX", resultX);
imshow("resultY", resultY);
imshow("resultXY", resultXY);
cout << "接下来生成边缘检测器"<< endl;
waitKey(0);
Mat sobel_x1,sobel_y1;//存放分离的Sobel算子
Mat scharr_x, scharr_y;//存放分离的Scharr算子
Mat sobelX1,scharrX;//存放最终算子
//一阶交方向Sobel算子
getDerivKernels(sobel_x1, sobel_y1, 1,0, 3);
sobel_x1 = sobel_x1.reshape(CV_8U,1);
sobelX1 = sobel_y1*sobel_x1; //计算滤波器
//X方向Scharr算子
getDerivKernels(scharr_x,scharr_y,1,0,FILTER_SCHARR);
scharr_x = scharr_x.reshape(CV_8U,1);
scharrX = scharr_y*scharr_x;//计算滤波器
//输出结果
cout << "X方向一阶Sobel算子:"<<endl << sobelX1 << endl ;
cout << "X方向Scharr算子:" << endl << scharrX << endl;
waitKey(0);
}
6、Laplacian算子边缘检测
X Y方向均可良好检测
7、Canny算子边缘检测
对Sobel算子优化,去除虚假边缘,保留强边缘,去除弱边缘
8、Laplacian、Canny的使用
//边缘检测二:Laplacian、Canny
int test2()
{
//读取图像,黑白图像边缘检测结果较为明显
Mat img = imread("F:/testMap/equalLena.png", IMREAD_ANYDEPTH);
if (img.empty())
{
cout << "请确认图像文件名称是否正确" << endl;
return -1;
}
Mat result, result_g, result_G;
//未滤波提取边缘
Laplacian(img, result, CV_16S, 3, 1, 0);
convertScaleAbs(result, result);
//滤波后提取Laplacian边缘
GaussianBlur(img, result_g, Size(3, 3), 5, 0);//高斯滤波
Laplacian(result_g, result_G, CV_16S, 3, 1, 0);
convertScaleAbs(result_G, result_G);
//显示图像
imshow("result", result);
imshow("result_G", result_G);
cout << "接下来进行Canny边缘检测" << endl;
waitKey(0);
Mat resultHigh, resultLow, resultG;
//大阈值检测图像边缘
Canny(img, resultHigh, 100, 200, 3);
//小阈值检测图像边缘
Canny(img, resultLow, 20, 40, 3);
//高斯模糊后大阈值检测图像边缘
GaussianBlur(img, resultG, Size(3, 3), 5);
Canny(resultG, resultG, 100, 200, 3);
//显示图像
imshow("resultHigh", resultHigh);
imshow("resultLow", resultLow);
imshow("resultG", resultG);
waitKey(0);
return 0;
}