一. 前置条件
- 接入支付首先得需要有企业资质,并开通企业对公户
- 注册微信支付并进行对公户打款认证
二. 开始接入
1. 下载微信支付的AP证书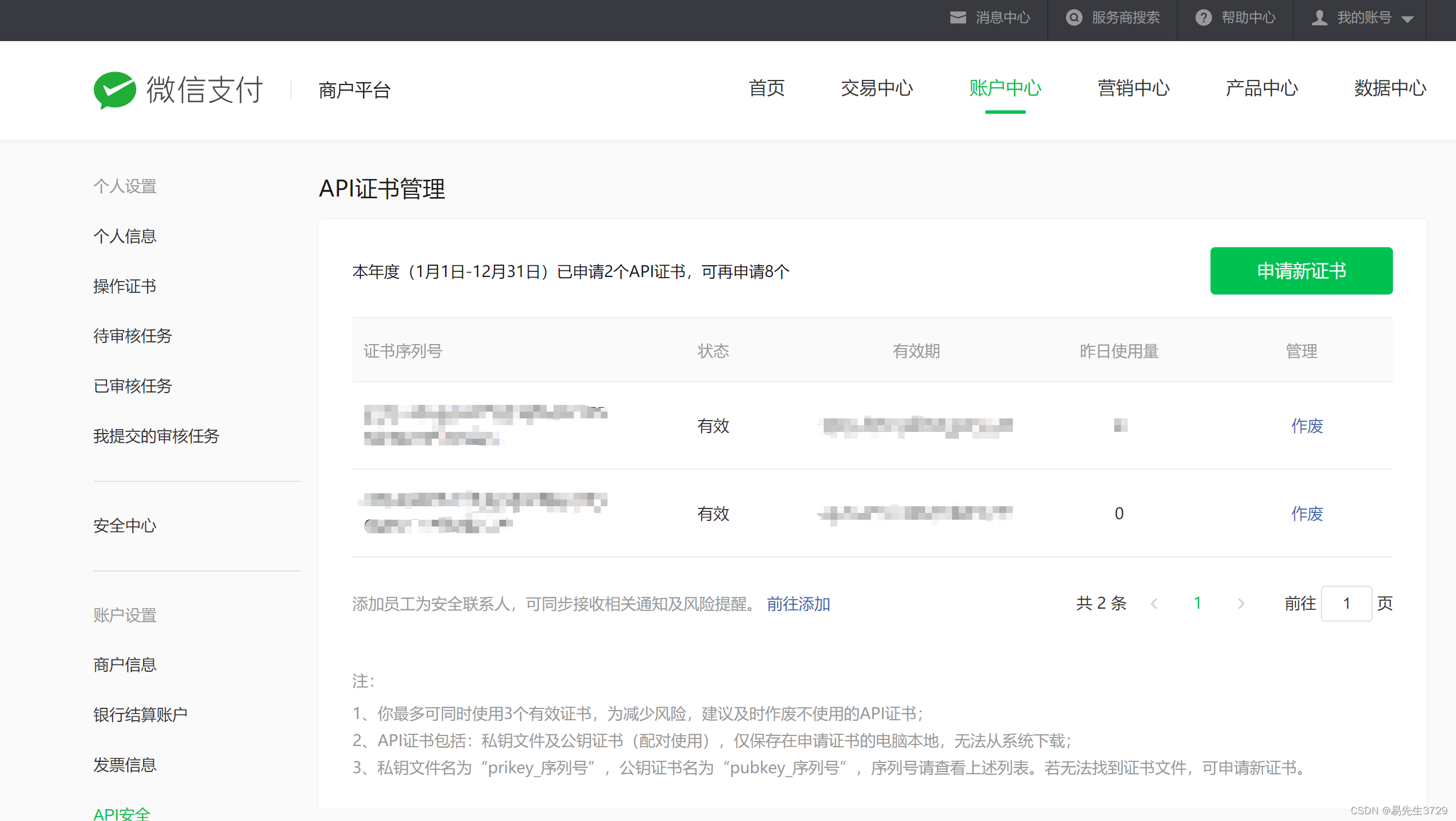
2. 服务端接入微信支付
2.1 引入相关maven配置
<dependency>
<groupId>com.github.binarywang</groupId>
<artifactId>weixin-java-miniapp</artifactId>
<version>${wechat.sdk.version}</version>
</dependency>
<dependency>
<groupId>com.github.binarywang</groupId>
<artifactId>weixin-java-pay</artifactId>
<version>${wechat.sdk.version}</version>
</dependency>
2.2 配置小程序相关信息
#微信支付配置
wx.pay.appId=xxxxxxxxxxxxxxxx
wx.pay.mchId=xxxxx
wx.pay.mchKey=xxxxxxxxxxxxxxxxx
wx.pay.keyPath=classpath:xxxxxxx.p12
2.3 相关配置类文件代码编写
WxPayConfiguration.java
package com.xxxxx;
import com.github.binarywang.wxpay.config.WxPayConfig;
import com.github.binarywang.wxpay.service.WxPayService;
import com.github.binarywang.wxpay.service.impl.WxPayServiceImpl;
import lombok.AllArgsConstructor;
import org.apache.commons.lang3.StringUtils;
import org.springframework.boot.autoconfigure.condition.ConditionalOnClass;
import org.springframework.boot.autoconfigure.condition.ConditionalOnMissingBean;
import org.springframework.boot.context.properties.EnableConfigurationProperties;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
@ConditionalOnClass(WxPayService.class)
@EnableConfigurationProperties(WxPayProperties.class)
@AllArgsConstructor
public class WxPayConfiguration {
private WxPayProperties properties;
@Bean
@ConditionalOnMissingBean
public WxPayService wxService() {
WxPayConfig payConfig = new WxPayConfig();
payConfig.setAppId(StringUtils.trimToNull(this.properties.getAppId()));
payConfig.setMchId(StringUtils.trimToNull(this.properties.getMchId()));
payConfig.setMchKey(StringUtils.trimToNull(this.properties.getMchKey()));
payConfig.setKeyPath(StringUtils.trimToNull(this.properties.getKeyPath()));
// 可以指定是否使用沙箱环境
payConfig.setUseSandboxEnv(false);
WxPayService wxPayService = new WxPayServiceImpl();
wxPayService.setConfig(payConfig);
return wxPayService;
}
}
WxPayProperties.java
package com.xxxxx
import lombok.Data;
import org.springframework.boot.context.properties.ConfigurationProperties;
@Data
@ConfigurationProperties(prefix = "wx.pay")
public class WxPayProperties {
/**
* 设置微信公众号或者小程序等的appid
*/
private String appId;
/**
* 微信支付商户号
*/
private String mchId;
/**
* 微信支付商户密钥
*/
private String mchKey;
/**
* apiclient_cert.p12文件的绝对路径,或者如果放在项目中,请以classpath:开头指定
*/
private String keyPath;
/**
* 回调地址
*/
private String notifyUrl;
}
2.4 下单退款API接入
package com.xxxxx
import com.alibaba.fastjson.JSON;
import com.github.binarywang.wxpay.bean.notify.WxPayOrderNotifyResult;
import com.github.binarywang.wxpay.bean.order.WxPayMpOrderResult;
import com.github.binarywang.wxpay.bean.request.WxPayRefundRequest;
import com.github.binarywang.wxpay.bean.request.WxPayUnifiedOrderRequest;
import com.github.binarywang.wxpay.bean.result.WxPayRefundResult;
import com.github.binarywang.wxpay.exception.WxPayException;
import com.github.binarywang.wxpay.service.WxPayService;
import com.xxxxx.CustomException;
import com.xxxxx.ResultCode;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
@Component
@Slf4j
public class WechatPayRepository {
@Autowired
private WxPayService wxPayService;
/**
* 统一下单 发起微信支付
* @param request
* @return
*/
public WxPayMpOrderResult createOrder(WxPayUnifiedOrderRequest request) {
try {
return wxPayService.createOrder(request);
} catch (WxPayException e) {
log.error("微信发起支付失败: {}", e);
throw new CustomException(ResultCode.WECHAT_PAY_CREATE_ORDER_ERROR);
}
}
/**
* 解析支付结果通知
* @param xmlData
* @return
*/
public WxPayOrderNotifyResult parseOrderNotifyResult(String xmlData) {
try {
return wxPayService.parseOrderNotifyResult(xmlData);
} catch (WxPayException e) {
log.error("微信支付回调解析失败: {}", e);
throw new CustomException(ResultCode.WECHAT_PAY_PARSE_ERROR);
}
}
/**
* 退款
* @param request
* @return
*/
public WxPayRefundResult refund(WxPayRefundRequest request) {
try {
log.info("微信发起退款: {}", JSON.toJSONString(request));
return wxPayService.refund(request);
} catch (WxPayException e) {
if("INVALID_REQUEST".equals(e.getErrCode()) && "订单已全额退款".equals(e.getErrCodeDes())) {
return null;
}
log.error("微信发起退款失败: {}", e);
throw new CustomException(ResultCode.WECHAT_PAY_REFUND_ERROR);
}
}
}
3. 小程序端接入支付
wx.requestPayment({
timeStamp: timeStamp,
nonceStr: nonceStr,
package: packageValue,
signType: signType,
paySign: paySign,
success(res) {
wx.reLaunch({
url: '/xxx/xxx'
});
},
fail(res) { }
})
- 贴上每个参数的说明
4. 至此,小程序的微信支付接入完成了,是不是so easy。
三. 体验更多
想体验更多小程序的功能,欢迎扫以下的小程序码,博主自研产品,捧个场,感谢Thanks♪(・ω・)ノ
下一期想讲解哪一部分,欢迎评论区留言 ~