使用函数判断完全平方数
没有加(int)过不了
int IsSquare(int n){
if((int)sqrt(n) * sqrt(n) != n) return 0;
else return 1;
}
使用函数求余弦函数的近似值
double funcos(double e, double x){
double sum = 1, item = 1;
for(int i = 0; fabs(item) >= e; i += 2){
item = (-1) * item * x * x / ((i + 1) * (i + 2));
sum += item;
}
return sum;
}
6-13 使用函数输出水仙花数
int narcissistic(int number){
int n = number;
int item = 0, sum = 0, cnt = 0;
while(n){
n /= 10;
cnt ++;
}
item = number;
while(item){
int lastnumber = item % 10;
sum += pow(lastnumber, cnt);
item /= 10;
}
if(sum == number) return 1;
return 0;
}
void PrintN(int m, int n){
for(int i = m + 1; i < n; i ++ ){
if(narcissistic(i)) printf("%d\n", i);
}
}
6-18 使用函数输出指定范围内的完数
在一些高级语言当中,为了能够完成更好的逻辑判断,因此就有了bool类型,bool类型的变量值只有true和false两种。
而在C语言中,一般认为0为假,非0为真。
这是因为c99之前,c90是没有bool类型的的。但是c99引入了_Bool类型(_Bool就是一个类型,不过在新增头文件stdbool.h中,被重新用宏写成了 bool,为了保证C/C++兼容性)。
目前为止大部分C语言书籍采用的标准还是c90标准,因此我们很少用bool类型。
factorsum函数中如果是
int factorsum( int number ){
int sum = 1;
for(int i = 2; i < number; i ++ ){
if(number % i == 0) sum += i;
}
return sum;
}
过不了 最大范围 的测试点
int factorsum( int number ){
int sum = 0;
for(int i = 1; i < number; i ++ ){
if(number % i == 0) sum += i;
}
return sum;
}
void PrintPN( int m, int n ){
int flag = 0;
for(int i = m; i <= n; i ++ ){
if(factorsum(i) == i){
flag = 1;
printf("%d = 1", i);
for(int j = 2; j < i; j ++ ){
if(i % j == 0) printf(" + %d", j);
}
printf("\n");
}
}
if(!flag) printf("No perfect number");
}
6-19 使用函数输出指定范围内的Fibonacci数
一开始的代码一直出现 [PTA报错]warning: ignoring return value of ‘scanf’, declared with attribute warn_unused_result 的问题
下面的ac代码反向使用cnt,和我平时的用法感觉妙了点(?
int fib( int n ){
if(n == 1 || n == 2) return 1;
return fib(n - 1) + fib(n - 2);
}
void PrintFN( int m, int n ){
int cnt = 1, flag = 0;
for(int i = 1; fib(i) <= n; i ++ ){
if(fib(i) >= m && fib(i) <= n){
if(cnt){
printf("%d", fib(i));
cnt = 0;
}
else printf(" %d", fib(i));
flag = 1;
}
}
if(!flag) printf("No Fibonacci number");
}
6-30 删除字符
void delchar( char *str, char c ){
int j = 0;
for(int i = 0; *(str + i) !='\0'; i ++ ){
if(*(str + i) != c){
*(str + j) = *(str + i);
j ++;
}
}
*(str + j) = '\0';
}
6-31 字符串的连接
char *str_cat( char *s, char *t ){
int len_s = strlen(s);
int len_t = strlen(t);
for(int i = 0; i < len_t; i ++ ){
*(s + len_s + i) = *(t + i);
}
return &s[0];
}
6-46 指定位置输出字符串
char *match( char *s, char ch1, char ch2 ){
char *p;
int i;
for(i = 0; i < strlen(s); i ++ ){
if(s[i] == ch1) break;
}
p = &s[i];
for(int j = i; j < strlen(s); j ++ ){
printf("%c", s[j]);
if(s[j] == ch2) break;
}
printf("\n");
return p;
}
6-47 查找子串
char *search( char *s, char *t ){
int flag = 0;
for(int i = 0; s[i] != '\0'; i ++ ){
for(int j = 0; t[j] != '\0'; j ++ ){
flag = 0;
if(s[i + j] != t[j]) break;
else flag = 1;
}
if(flag) return &s[i];
}
return NULL;
}
6-49 建立学生信息链表
void input(){
struct stud_node *p;
p = (struct stud_node *)malloc(sizeof(struct stud_node));
scanf("%d", &p->num);
while(p->num != 0){
scanf("%s %d", p->name, &p->score);
if(head == NULL){
head = p;
head->next = NULL;
}
if(tail != NULL) tail->next = p;
tail = p;
tail->next = NULL;
p = (struct stud_node *)malloc(sizeof(struct stud_node));
scanf("%d", &p->num);
}
}
6-50 学生成绩链表处理
struct stud_node *createlist(){
struct stud_node *head, *tail, *s;
head = (struct stud_node *)malloc(sizeof(struct stud_node));
head->next = NULL;
tail = head;
s = (struct stud_node *)malloc(sizeof(struct stud_node));
while(1){
s=(struct stud_node*)malloc(sizeof(struct stud_node));
scanf("%d",&s->num);
if(s->num==0)break;
scanf("%s",s->name);
scanf("%d",&s->score);
s->next=NULL;
tail->next=s;
tail=s;
}
return head;
}
struct stud_node *deletelist( struct stud_node *head, int min_score ){
struct stud_node *p, *n;
p = head->next;
while(p != NULL){
if(p->score < min_score){
n = head;
while(n->next != p) n = n->next;
n->next = p->next;
free(p);
}
p = p->next;
}
return head->next;
}
6-51 逆序数据建立链表
struct ListNode *createlist(){
struct ListNode *head, *tail, *p;
head = tail = NULL;
int num;
while(scanf("%d", &num) && num != -1){
p = (struct ListNode *)malloc(sizeof(struct ListNode));
p->data = num;
p->next = head;
head = p;
}
return head;
}
6-52 链表拼接
struct ListNode *mergelists(struct ListNode *list1, struct ListNode *list2){
struct ListNode *head;
head = (struct ListNode *)malloc(sizeof(struct ListNode));
struct ListNode *p = head, *p1 = list1, *p2 = list2;
while(p1 && p2){
if(p1->data < p2->data){
p->next = p1;
p1 = p1->next;
p = p->next;
}
else{
p->next = p2;
p2 = p2->next;
p = p->next;
}
}
p->next = p1 ? p1 : p2;
return head->next;
}
6-53 奇数值结点链表
struct ListNode *readlist(){
struct ListNode *head = NULL;
struct ListNode *current, *prev;
int n;
while(scanf("%d", &n) == 1 && n != -1){
current = (struct ListNode *)malloc(sizeof(struct ListNode));
if(head == NULL) head = current;
else prev->next = current;
current->next = NULL;
current->data = n;
prev = current;
}
return head;
}
struct ListNode *getodd( struct ListNode **L ){
struct ListNode *t = *L;
struct ListNode *head_even = NULL, *head_odd = NULL;
struct ListNode *current_even, *current_odd, *prev_even, *prev_odd;
while(t){
if((t->data % 2) == 0){
current_even = (struct ListNode *)malloc(sizeof(struct ListNode));
if(head_even == NULL) head_even = current_even;
else prev_even->next = current_even;
current_even->next = NULL;
current_even->data = t->data;
prev_even = current_even;
}
else{
current_odd = (struct ListNode *)malloc(sizeof(struct ListNode));
if(head_odd == NULL) head_odd = current_odd;
else prev_odd->next = current_odd;
current_odd->next = NULL;
current_odd->data = t->data;
prev_odd = current_odd;
}
t = t->next;
}
*L = head_even;
return head_odd;
}
6-54 单链表结点删除
hhhhh,仿照上面的自己敲出来了红红火火恍恍惚惚哈哈哈(已疯
struct ListNode *readlist(){
struct ListNode *head = NULL;
struct ListNode *prev, *current;
int n;
while(scanf("%d", &n) == 1 && n != -1){
current = (struct ListNode *)malloc(sizeof(struct ListNode));
if(head == NULL) head = current;
else prev->next = current;
current->next = NULL;
current->data = n;
prev = current;
}
return head;
}
struct ListNode *deletem( struct ListNode *L, int m ){
struct ListNode *t = L;
struct ListNode *head = NULL;
struct ListNode *current, *prev;
while(t){
if(t->data != m){
current = (struct ListNode *)malloc(sizeof(struct ListNode));
if(head == NULL) head = current;
else prev->next = current;
current->next = NULL;
current->data = t->data;
prev = current;
}
t = t->next;
}
return head;
}
6-55 链表逆置 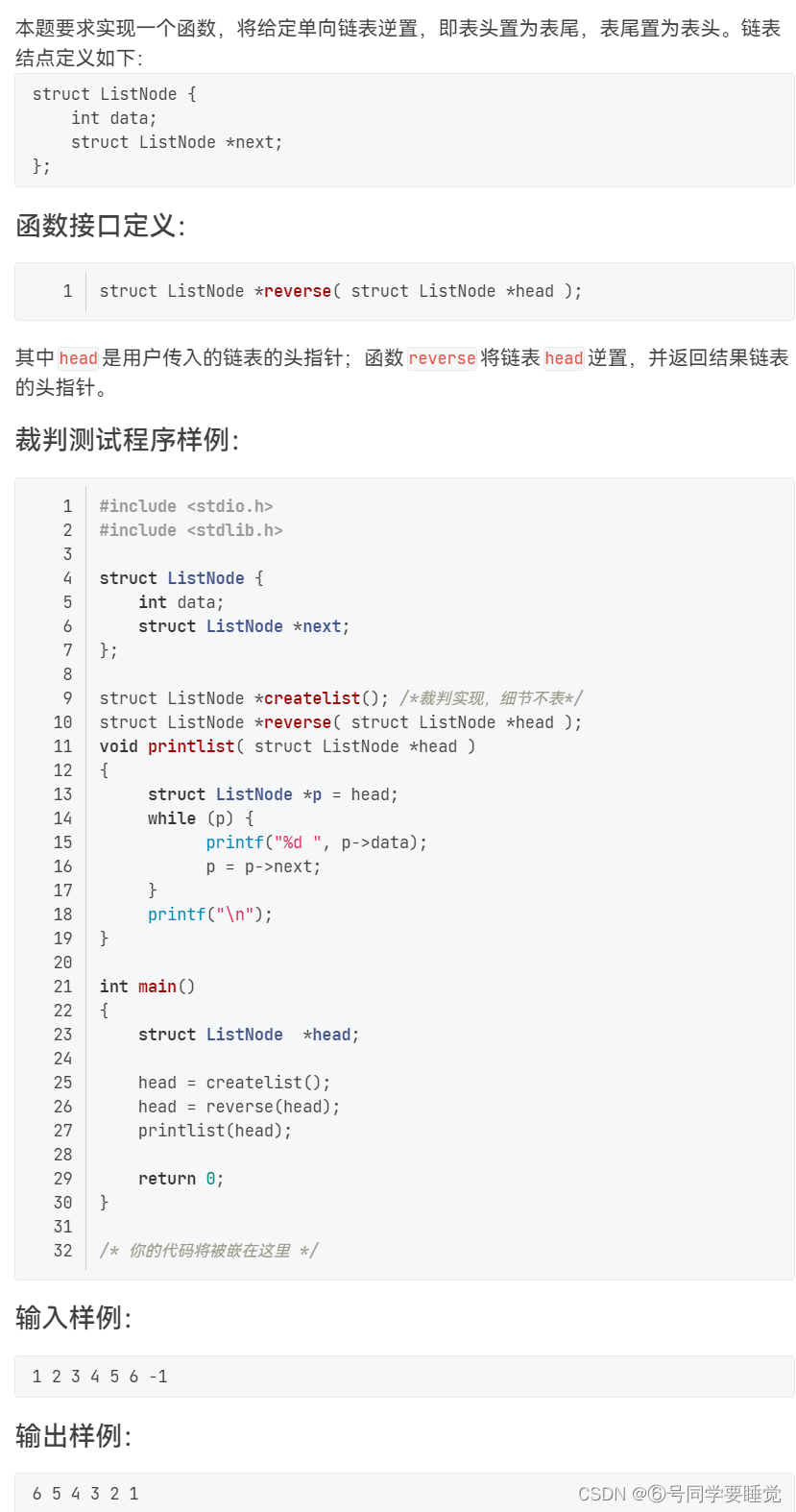
struct ListNode *reverse( struct ListNode *head ){
struct ListNode *Head, *p, *q;
p = head;
Head = NULL;
while(p){
q = p->next;
p->next = Head;
Head = p;
p = q;
}
return Head;
}
6-56 统计专业人数
int countcs( struct ListNode *head ){
int cnt = 0;
if(head == NULL) return cnt;
while(head){
char code[8];
strcpy(code, head->code);
if(code[1] == '0' && code[2] == '2') cnt ++;
head = head->next;
}
return cnt;
}
6-57 删除单链表偶数节点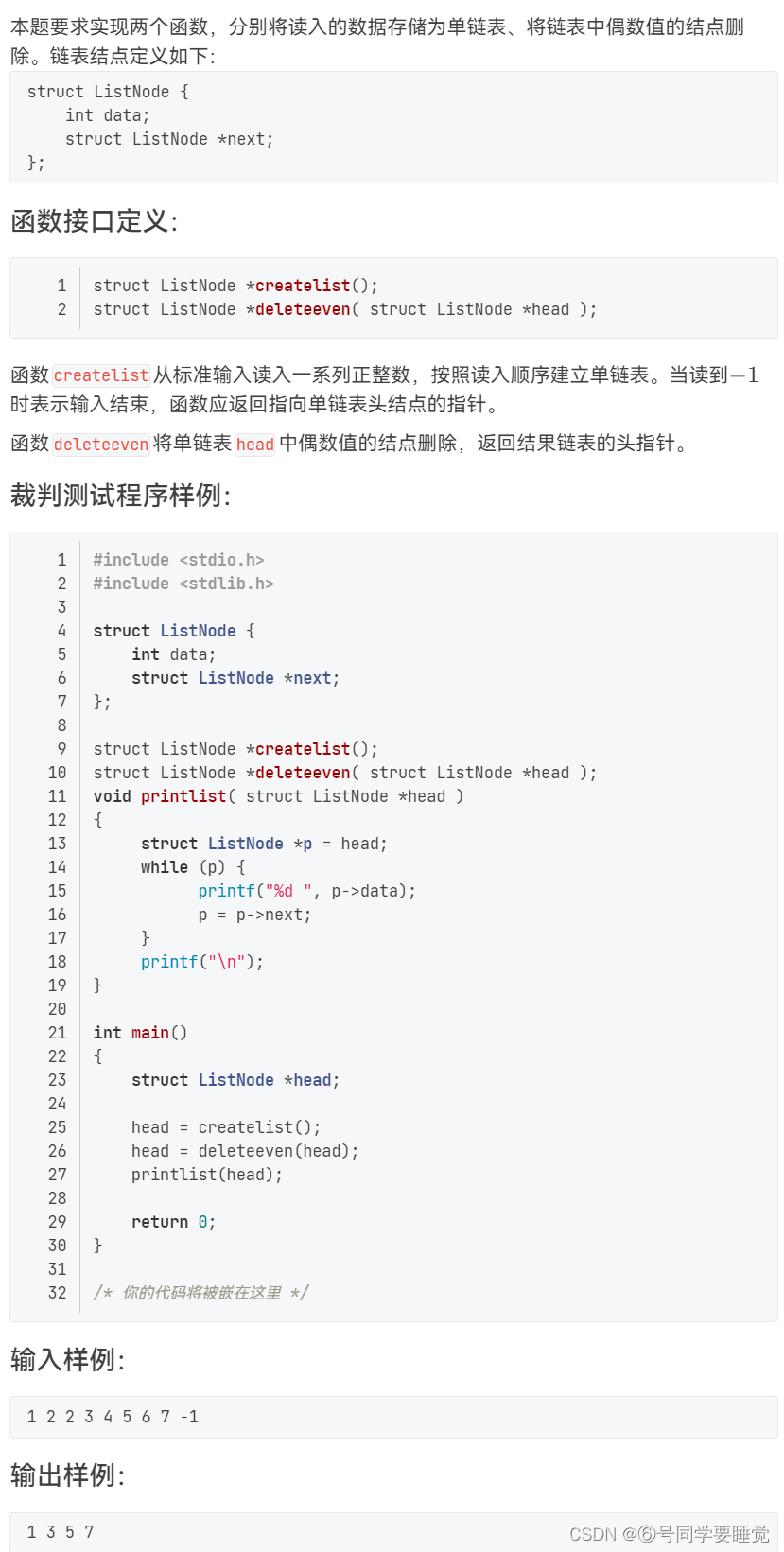
这道题就是前面单链表结点删除直接复制粘贴改几个就ac了(
struct ListNode *createlist(){
struct ListNode *head = NULL;
struct ListNode *prev, *current;
int n;
while(scanf("%d", &n) == 1 && n != -1){
current = (struct ListNode *)malloc(sizeof(struct ListNode));
if(head == NULL) head = current;
else prev->next = current;
current->next = NULL;
current->data = n;
prev = current;
}
return head;
}
struct ListNode *deleteeven( struct ListNode *head ){
struct ListNode *t = head;
struct ListNode *Head = NULL;
struct ListNode *current, *prev;
while(t){
if(t->data % 2 != 0){
current = (struct ListNode *)malloc(sizeof(struct ListNode));
if(Head == NULL) Head = current;
else prev->next = current;
current->next = NULL;
current->data = t->data;
prev = current;
}
t = t->next;
}
return Head;
}
7-45 高速公路超速处罚
打%号 %%
#include<bits/stdc++.h>
using namespace std;
#define INF 0x3f3f3f3f
typedef long long LL;
const int N = 40;
int main(){
int v, lv;
cin >> v >> lv;
double x = 100.0 * (v - lv) / lv;
if(x < 10) cout << "OK";
else if(x < 50) printf("Exceed %.lf%%. Ticket 200\n", x);
else printf("Exceed %.lf%%. License Revoked\n", x);
return 0;
}
7-54 猜数字游戏
if else 过不了,要用if if if,因为后面还有一个循环外的条件不能都过??就是感觉这题还是不太会wwwww
#include<bits/stdc++.h>
using namespace std;
#define INF 0x3f3f3f3f
typedef long long LL;
const int N = 40;
int main(){
int sn, n;
cin >> sn >> n;
int cnt = 0;
for(int i = 1; i <= n; i ++ ){
int a;
cin >> a;
cnt ++ ;
if(a == sn && cnt == 1){
cout << "Bingo!" << endl;
break;
}
else if(a == sn && cnt <= 3 && cnt >= 1){
cout << "Lucky you!" << endl;
break;
}
else{
if(a < 0){
cout << "Game over" << endl;
break;
}
if(a > sn){
cout << "Too big" << endl;
}
if(a < sn){
cout << "Too small" << endl;
}
if(a == sn){
cout << "Good Guess!" << endl;
break;
}
}
if(a != sn && cnt == n){
cout << "Game over" << endl;
}
}
return 0;
}
7-56 高空坠球
#include<bits/stdc++.h>
using namespace std;
#define INF 0x3f3f3f3f
typedef long long LL;
const int N = 40;
int main(){
double H, n;
cin >> H >> n;
if(n == 0){
printf("0.0 0.0");
return 0;
}
double sum = 0, h = H;
for(int i = 1; i <= n; i ++ ){
sum += h;
h /= 2;
sum += h;
}
printf("%.1lf %.1lf", sum - h, h);
return 0;
}
7-57 求分数序列前N项和 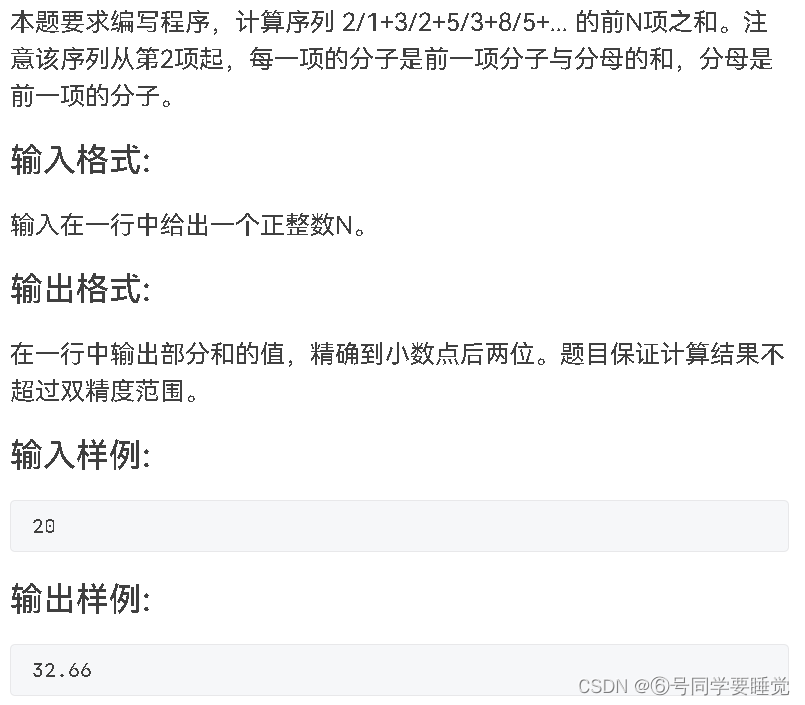
#include<bits/stdc++.h>
using namespace std;
#define INF 0x3f3f3f3f
typedef long long LL;
const int N = 40;
int main(){
int n;
cin >> n;
double sum = 0;
double z = 2.0, m = 1.0;
for(int i = 1; i <= n; i ++ ){
sum += z / m;
double t = z;
z += m;
m = t;
}
printf("%.2lf", sum);
return 0;
}
7-58 求e的近似值 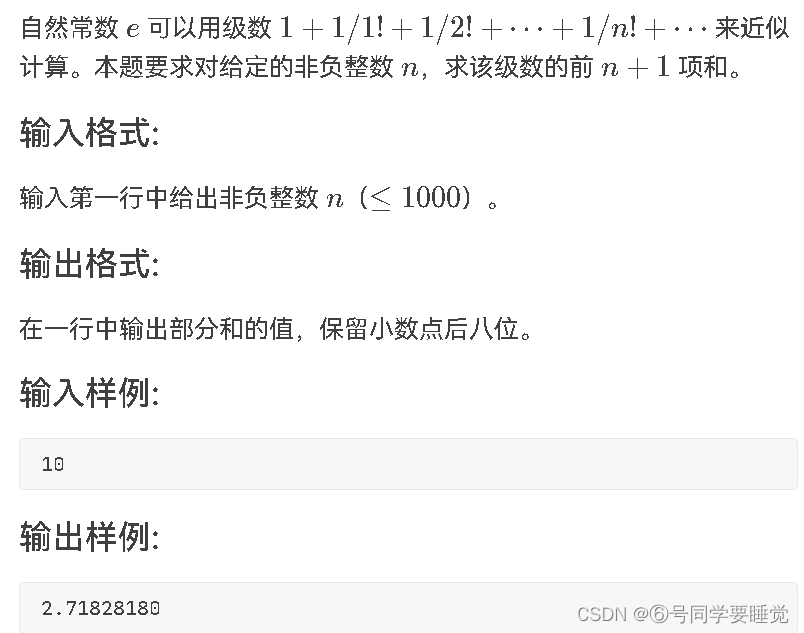
自定的fun函数int型过不了,要double
#include<bits/stdc++.h>
using namespace std;
#define INF 0x3f3f3f3f
typedef long long LL;
const int N = 40;
double fun(int n){
double ans = 1;
for(int i = 1; i <= n; i ++ ){
ans *= i;
}
return ans;
}
int main(){
int n;
cin >> n;
double sum = 1;
for(int i = 1; i <= n; i ++ ){
sum += 1.0 / fun(i);
}
printf("%.8lf", sum);
return 0;
}
7-61 求幂级数展开的部分和 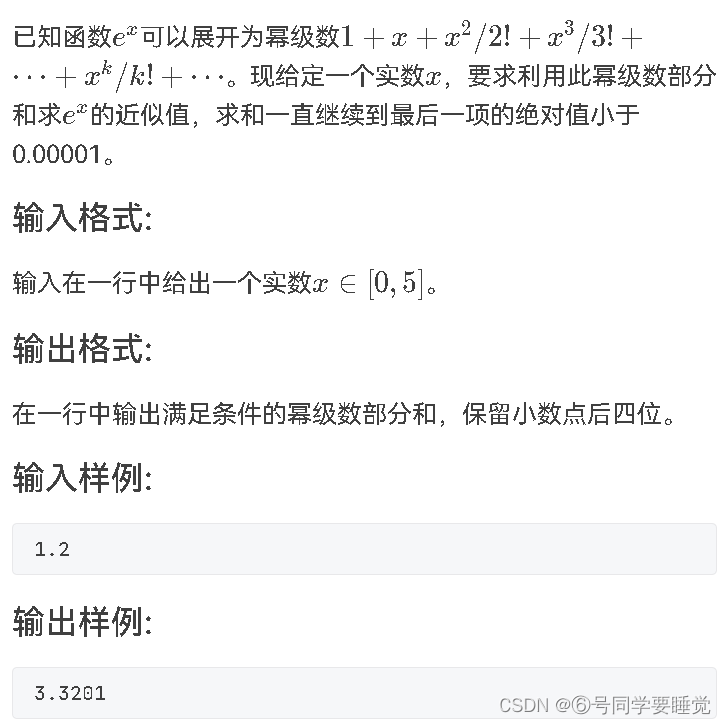
a啊啊啊啊啊·,还是没做出来,超时了一个点QAQ
#include<bits/stdc++.h>
using namespace std;
#define INF 0x3f3f3f3f
typedef long long LL;
//const int N = 40;
int main(){
double x;
cin >> x;
double sum = 1.0, ans = 1.0;
for(int i = 1;; i ++ ){
ans = (ans * x) / i;
sum += ans;
if(ans < 1e-5) break;
}
printf("%.4lf", sum);
return 0;
}
7-71 交换最小值和最大值
交换位置的时候amax要在amin前面,不然 最小值出现最后 的测试点不能过
分析一下,如果最小值在最后,最大值在最前面的情况下:
eg:8 2 5 4 1
ac的代码来分析,先找到的是和amax相等的a[0], 交换后就变成了 1 2 5 4 8
继续循环,然后找到了和amin相等的a[0], 交换后变成了 1 2 5 4 8
拿 最小值出现最后 的测试点不能过的代码来说:
eg:8 2 5 4 1
先找到的是和amax相等的a[0], 交换后变成了1 2 5 4 8
但是这时候只能继续循环开始 i = 1 了,可是此时最小值在 i = 0 , 所以无法交换最小值到最后的位置
//最小值出现在最后的测试点过不了
for(int i = 0; i < n; i ++ ){
if(amin == a[i]){
a[i] = a[0] ^ a[i];
a[0] = a[0] ^ a[i];
a[i] = a[0] ^ a[i];
}
if(amax == a[i]){
a[n - 1] = a[n - 1] ^ a[i];
a[i] = a[n - 1] ^ a[i];
a[n - 1] = a[n - 1] ^ a[i];
}
}
AC代码
#include<bits/stdc++.h>
using namespace std;
#define INF 0x3f3f3f3f
typedef long long LL;
const int N = 10;
int a[N];
int main(){
int n;
cin >> n;
for(int i = 0; i < n; i ++ ) cin >> a[i];
int amin = a[0], amax = a[0];
for(int i = 1; i < n; i ++ ){
amin = min(amin, a[i]);
amax = max(amax, a[i]);
}
for(int i = 0; i < n; i ++ ){
if(amax == a[i]){
int t = a[n - 1];
a[n - 1] = amax;
a[i] = t;
}
if(amin == a[i]){
int t = a[0];
a[0] = amin;
a[i] = t;
}
}
for(int i = 0; i < n; i ++ ) cout << a[i] << " ";
return 0;
}
7-75 找出不是两个数组共有的元素
#include<bits/stdc++.h>
using namespace std;
#define INF 0x3f3f3f3f
typedef long long LL;
const int N = 25;
int a[N], b[N], c[N];
int main(){
int n, m, i, j;
int k = 0;
cin >> n;
for(i = 0; i < n; i ++ ) cin >> a[i];
cin >> m;
for(j = 0; j < m; j ++ ) cin >> b[j];
for(i = 0; i < n; i ++ ){
for(j = 0; j < m; j ++ ){
if(a[i] == b[j]) break;
}
if(j == m) c[k ++ ] = a[i];
}
for(i = 0; i < m; i ++ ){
for(j = 0; j < n; j ++ ){
if(a[j] == b[i]) break;
}
if(j == n) c[k ++ ] = b[i];
}
cout << c[0];
for(i = 1; i < k; i ++ ){
for(j = 0; j < i; j ++ ){
if(c[i] == c[j]) break;
}
if(j == i) cout << " " << c[i];
}
return 0;
}
7-85 螺旋方阵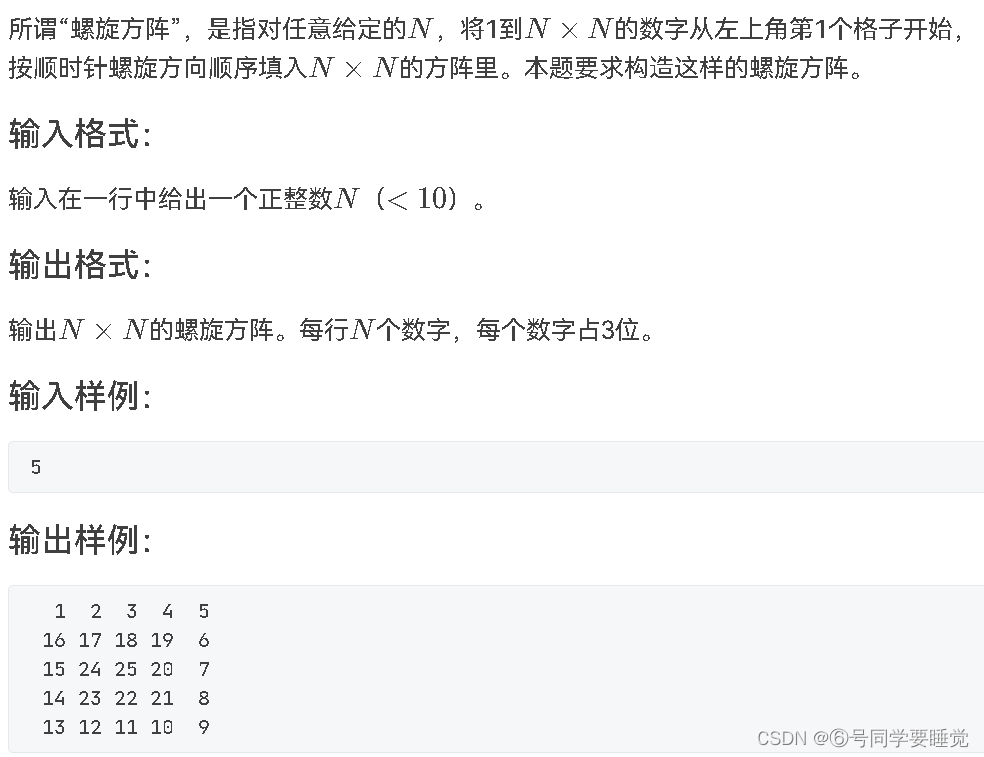
#include<bits/stdc++.h>
using namespace std;
#define INF 0x3f3f3f3f
typedef long long LL;
const int N = 15;
int a[N][N];
int main(){
int n;
cin >> n;
int row = 0, col = n - 1, num = 1;
while(row <= col){
for(int i = row; i <= col; i ++ ){
a[row][i] = num ++ ;
}
for(int i = row + 1; i <= col; i ++ ){
a[i][col] = num ++ ;
}
for(int i = col - 1; i >= row; i -- ){
a[col][i] = num ++ ;
}
for(int i = col - 1; i >= row + 1; i -- ){
a[i][row] = num ++ ;
}
row ++ , col -- ;
}
for(int i = 0; i < n; i ++ ){
for(int j = 0; j < n; j ++ ){
printf("%3d", a[i][j]);
}
puts("");
}
return 0;
}
7-88 统计字符出现次数
#include<bits/stdc++.h>
using namespace std;
#define INF 0x3f3f3f3f
typedef long long LL;
const int N = 350;
int a[N];
int main(){
char c;
c = getchar();
while(c != '\n'){
a[c] ++ ;
c = getchar();
}
c = getchar();
cout << a[c];
return 0;
}
7-92 字符串转换成十进制整数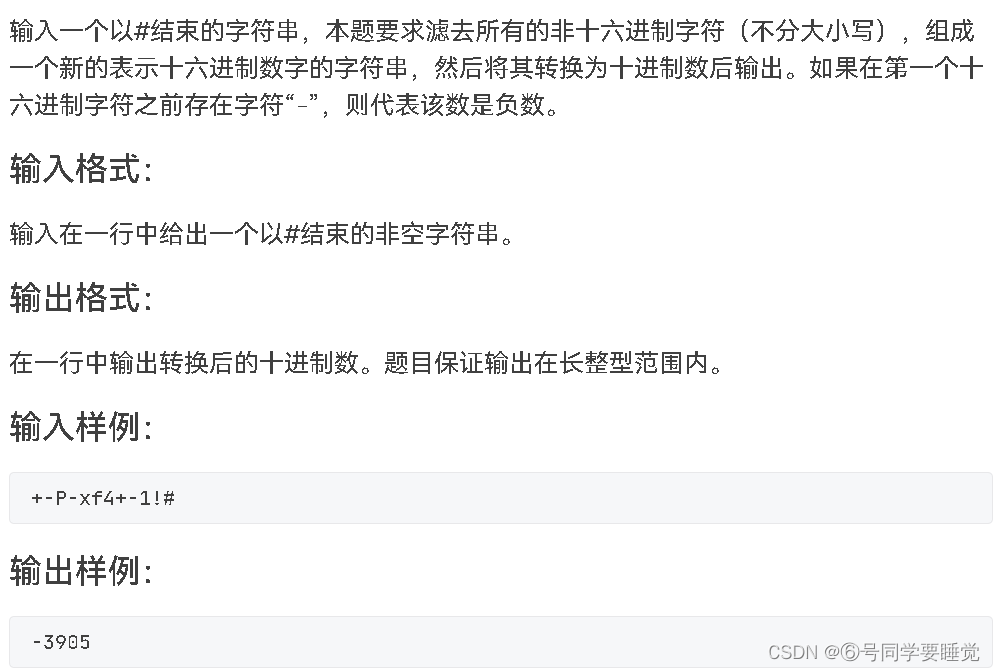
感觉就是有没有做过和看看ASCII的问题
#include<bits/stdc++.h>
using namespace std;
#define INF 0x3f3f3f3f
typedef long long LL;
const int N = 1e5 + 10;
LL fun(char *str){
LL x = 0;
for(int i = 0; i < strlen(str); i ++ ){
if(str[i] >= '0' && str[i] <= '9')
x = x * 16 + str[i] - '0';
else if(str[i] >= 'A' && str[i] <= 'F')
x = x * 16 + str[i] - 'A' + 10;
}
return x;
}
int main(){
char s[N], str[N];;
cin >> s;
int flag = 0, mul = 0, cnt = 0;
for(int i = 0; i < strlen(s); i ++ ){
s[i] = toupper(s[i]);
if(flag == 0){
if(s[i] == '-'){
flag = 1;
mul = 1;
}
else if((s[i] >= '0' && s[i] <= '9') || (s[i] >= 'A' && s[i] <= 'F')){
flag = 1;
str[cnt ++ ] = s[i];
}
}
else{
if((s[i] >= '0' && s[i] <= '9') || (s[i] >= 'A' && s[i] <= 'F')){
str[cnt ++] = s[i];
}
}
}
LL ans = fun(str);
if(mul == 1) ans *= -1;
cout << ans << endl;
return 0;
}
7-103 查找书籍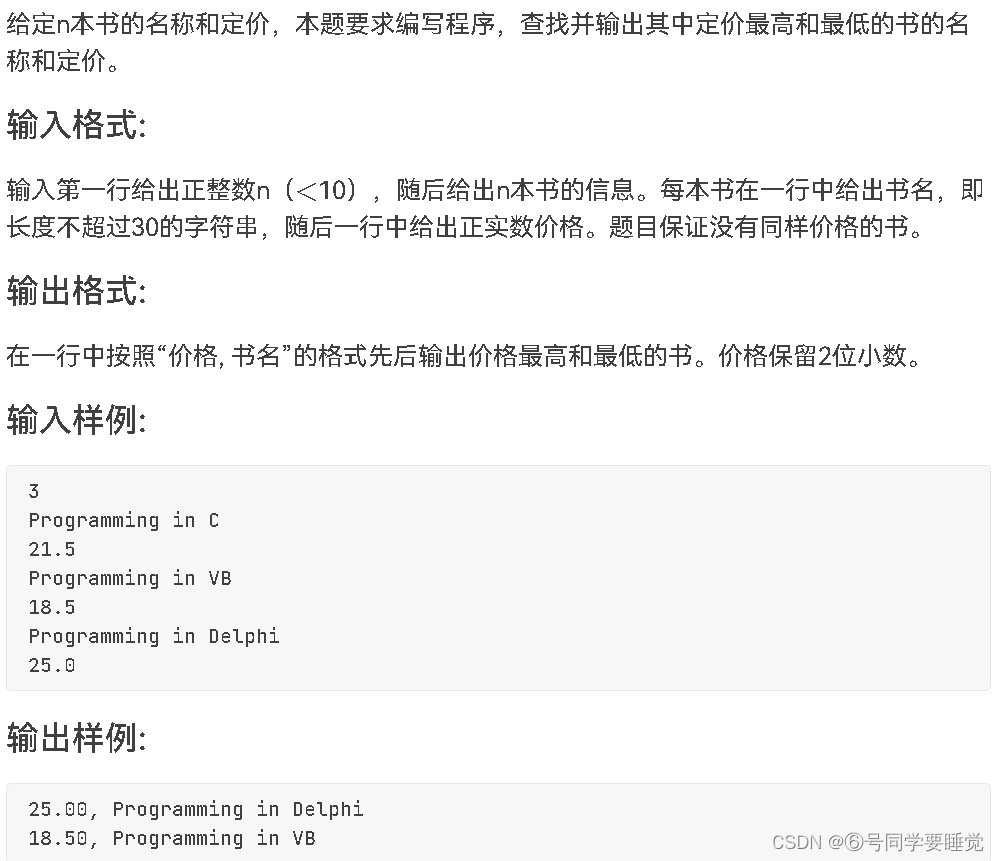
一开始忘记map还有自动根据key值排序的功能了
#include<bits/stdc++.h>
#define INF 0x3f3f3f3f
#define x first
#define y second
using namespace std;
typedef long long LL;
const int N = 1e5 + 10;
map<double, string> mp;
int main(){
int n;
cin >> n;
getchar();
while(n -- ){
string name;
double price;
getline(cin, name);
cin >> price;
getchar();
mp[price] = name;
}
cout << fixed << setprecision(2) << (--mp.end())->x << ", " << (--mp.end())->y << endl;
cout << fixed << setprecision(2) << mp.begin()->x << ", " << mp.begin()->y << endl;
return 0;
}
7-105 平面向量加法 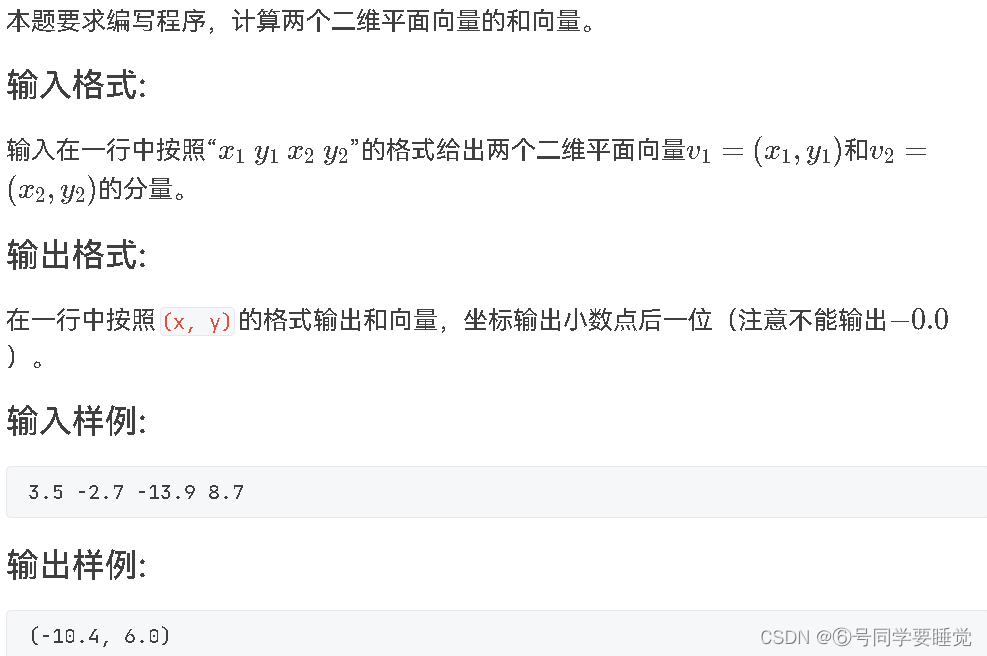
一开始nt了一下,进位考虑应该考虑保留最后一位的后一位
#include<bits/stdc++.h>
using namespace std;
#define INF 0x3f3f3f3f
#define x first
#define y second
typedef long long LL;
const int N = 1e5 + 10;
int main(){
double x1, x2, y1, y2;
cin >> x1 >> y1 >> x2 >> y2;
double x = x1 + x2;
double y = y1 + y2;
if(fabs(x) < 0.05) x = 0.0;
if(fabs(y) < 0.05) y = 0.0;
printf("(%.1lf, %.1lf)", x, y);
return 0;
}
7-108 英文单词排序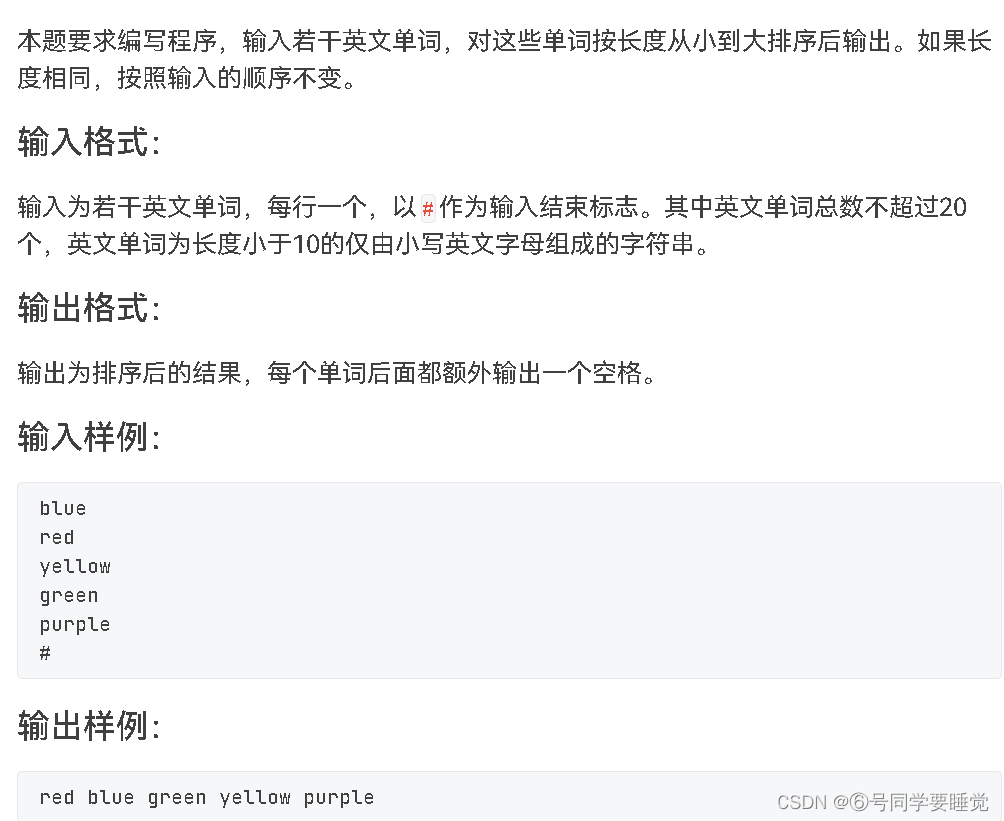
哥们觉得vector pair auto 用法需要记一下
#include<bits/stdc++.h>
using namespace std;
#define INF 0x3f3f3f3f
#define x first
#define y second
typedef long long LL;
const int N = 1e5 + 10;
typedef pair<int, int> pr;
vector<pr> v;
int cnt;
string t[N];
int main(){
string s;
while(cin >> s && s != "#"){
v.push_back({s.size(), cnt});
t[cnt ++ ] = s;
}
sort(v.begin(), v.end());
for(auto i : v){
cout << t[i.y] << " ";
}
return 0;
}
7-109 藏头诗
这道题上次做还是int行,改成UTF-8把我干蒙了,触及到只是盲区了
#include<bits/stdc++.h>
using namespace std;
#define INF 0x3f3f3f3f
#define x first
#define y second
typedef long long LL;
const int N = 1e5 + 10;
int main(){
string s;
for(int i = 0; i < 4; i ++ ){
getline(cin, s);
cout << s[0] << s[1] << s[2];
}
return 0;
}
7-110 自动售货机 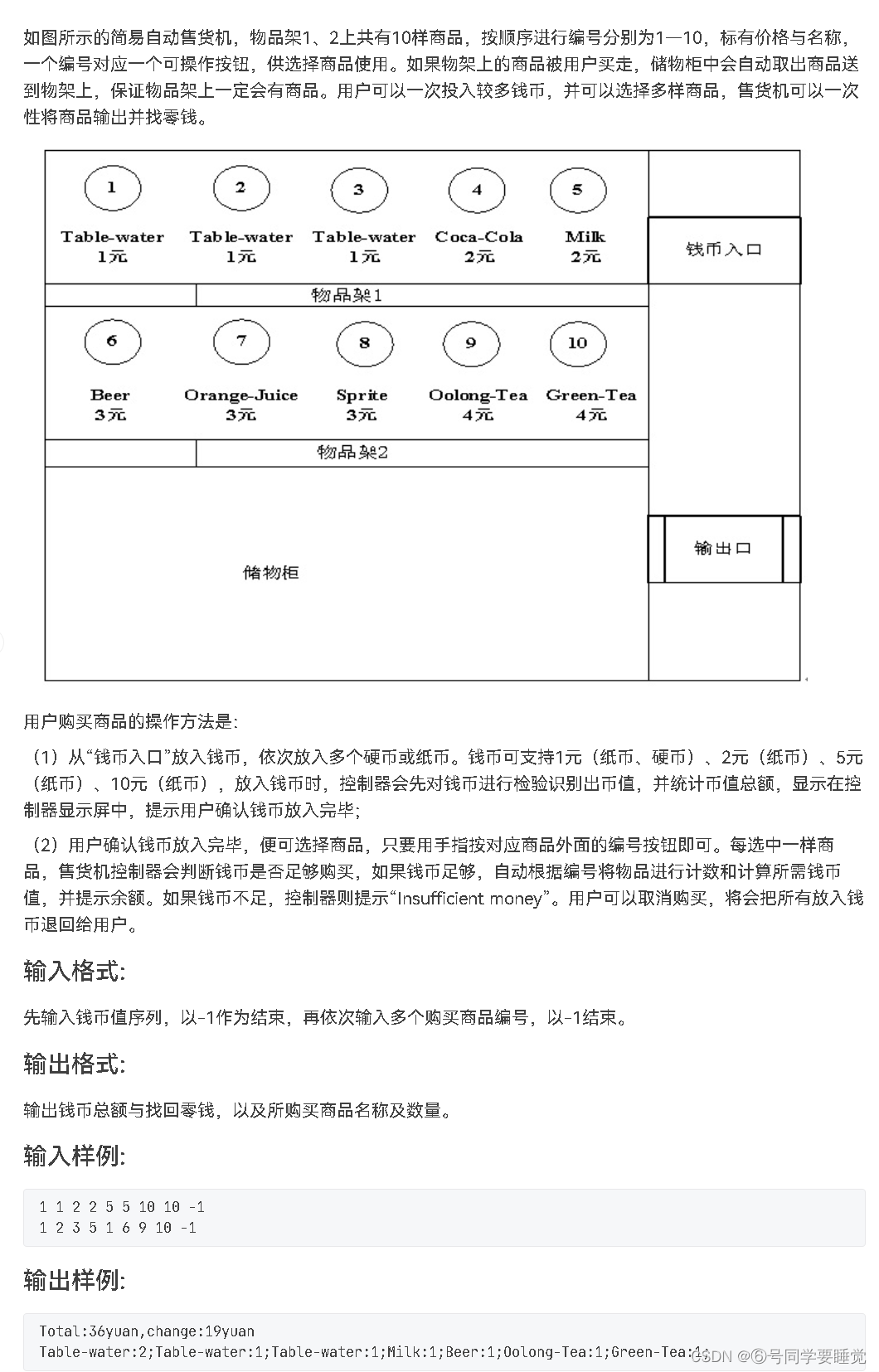
一开始没注意,被以前的思维带偏了,我为什么要想他一样的物品剩多少匹配多少的问题,NND
#include<bits/stdc++.h>
using namespace std;
#define INF 0x3f3f3f3f
#define x first
#define y second
typedef long long LL;
const int N = 20;
int a[N];
int sum, total;
int main(){
int n;
while(cin >> n, n != -1){
total += n;
}
while(cin >> n, n != -1){
a[n] ++ ; //编号为n的总数量
if(n > 0 && n < 4) sum ++ ;
else if(n > 3 && n < 6) sum += 2;
else if(n > 5 && n < 9) sum += 3;
else sum += 4;
}
if(total < sum) cout << "Insufficient money" << endl;
else{
printf("Total:%dyuan,change:%dyuan\n", total, total - sum);
for(int i = 0; i < 11; i ++ ){
if(a[i]){
switch(i){
case 1:printf("Table-water:%d;", a[i]);break;
case 2:printf("Table-water:%d;", a[i]);break;
case 3:printf("Table-water:%d;", a[i]);break;
case 4:printf("Coca-Cola:%d;", a[i]);break;
case 5:printf("Milk:%d;", a[i]);break;
case 6:printf("Beer:%d;", a[i]);break;
case 7:printf("Orange-Juice:%d;", a[i]);break;
case 8:printf("Sprite:%d;", a[i]);break;
case 9:printf("Oolong-Tea:%d;", a[i]);break;
case 10:printf("Green-Tea:%d;", a[i]);break;
}
}
}
}
return 0;
}
7-111 停车场管理 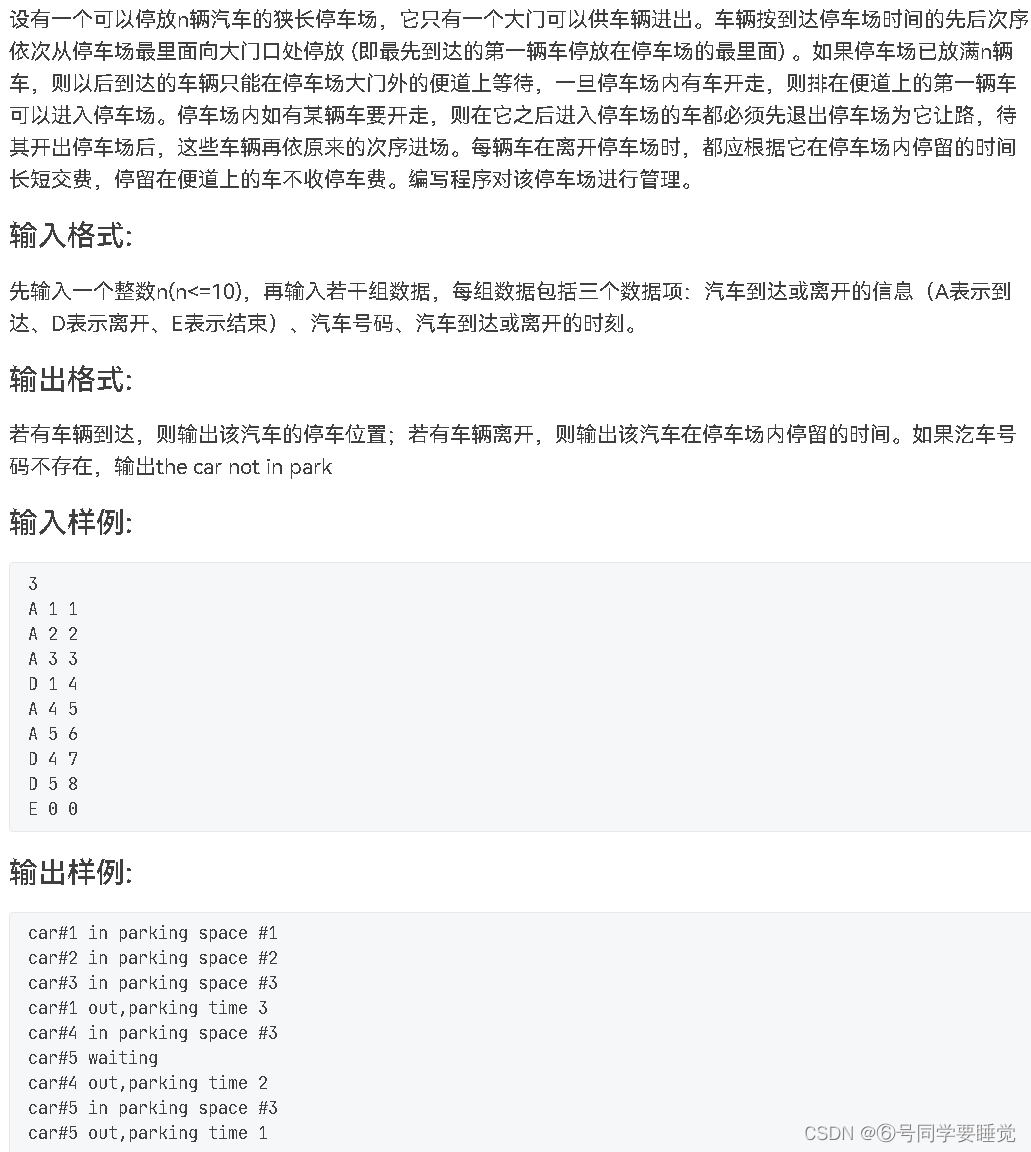
怪,这题真的怪,代码好像可以卡bug
#include<bits/stdc++.h>
using namespace std;
#define INF 0x3f3f3f3f
#define x first
#define y second
typedef long long LL;
const int N = 11;
int place[N], tim[N], wait[N];
int waiti = -1;
int main(){
int n;
cin >> n;
while(1){
char x;
int carnum, cartime;
cin >> x >> carnum >> cartime;
if(x == 'E') break;
else if(x == 'A'){
int flag = 0;
for(int i = 1; i <= n; i ++ ){
if(place[i] == 0){
flag = i;
break;
}
}
if(flag){
place[flag] = carnum;
cout << "car#" << carnum << " in parking space #" << flag << endl;
}
else{
wait[++ waiti] = carnum;
cout << "car#" << carnum << " waiting" << endl;
}
}
else if(x == 'D'){
int flag = 0;
for(int i = 0; i <= n; i ++ ){
if(place[i] == carnum){
flag = i;
break;
}
}
if(!flag) cout << "the car not in park" << endl;
else{
cout << "car#" << carnum << " out,parking time " << tim[flag] << endl;
place[flag] = 0;
tim[flag] = 0;
int t = 0;
for(int i = flag; i <= n; i ++ ){
place[i] = place[i + 1];
tim[i] = tim[i + 1];
if(i == n){
place[i] = 0;
tim[i] = 0;
}
t = i;
}
if(waiti != -1){
place[t] = wait[0];
for(int i = 0; i < waiti; i ++ ){
wait[i] = wait[i + 1];
}
waiti -- ;
cout << "car#" << place[t] << " in parking space #" << t << endl;
}
}
}
for(int i = 1; i <= n; i ++ ){
if(place[i]) tim[i] ++ ;
}
}
return 0;
}
7-112 值班安排 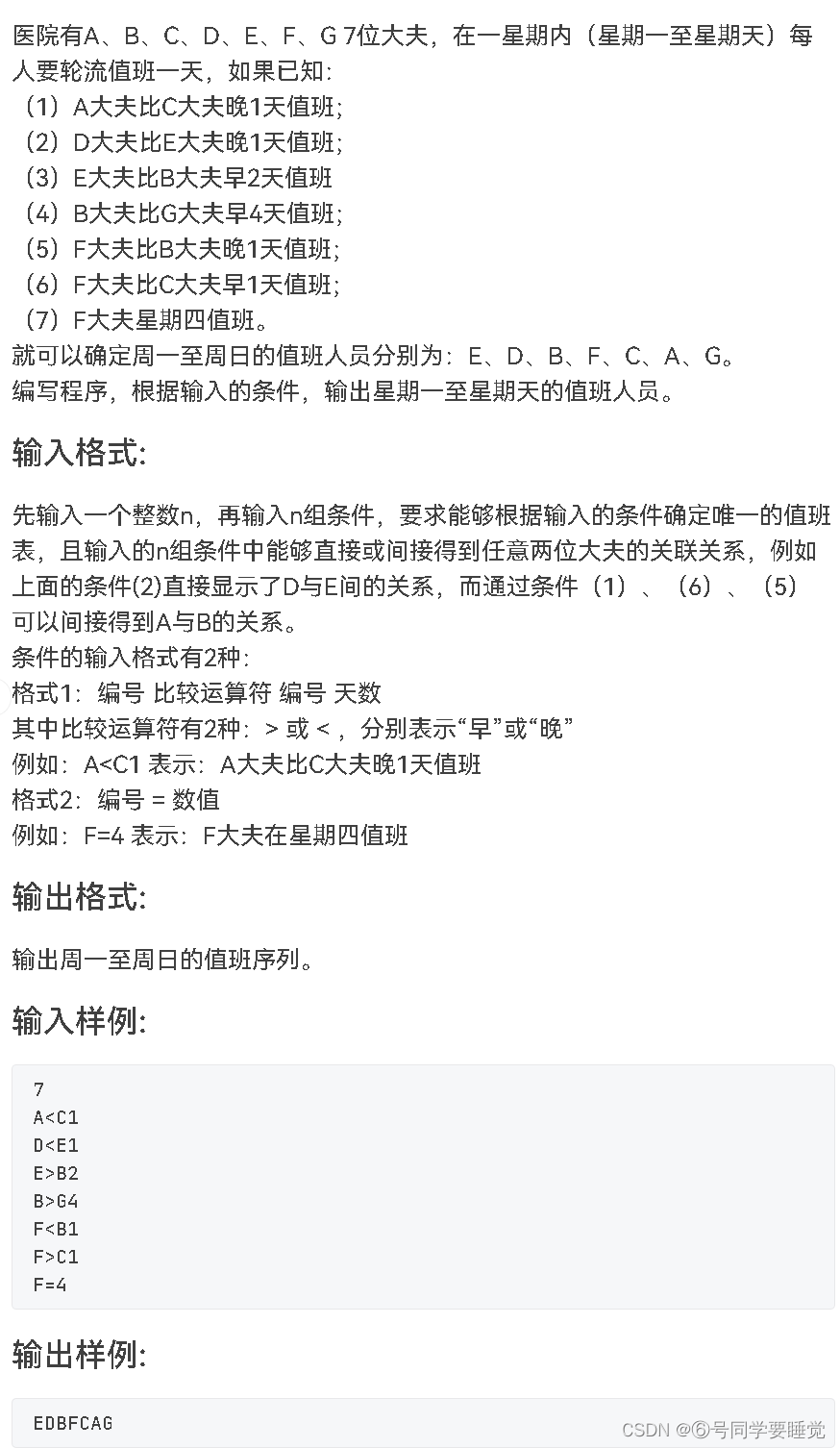
#include<bits/stdc++.h>
using namespace std;
#define INF 0x3f3f3f3f
#define x first
#define y second
typedef long long LL;
const int N = 20;
struct st{
int obj1;
int obj2;
int num;
int ret;
}st[N];
int judge(int *d, int n){
int ret = 1;
for(int i = 0; i < n; i ++ ){
if(st[i].ret){
if(d[st[i].obj1] != st[i].num - 1){
ret = 0;
break;
}
}
else if(d[st[i].obj1] + st[i].num != d[st[i].obj2]){
ret = 0;
break;
}
}
return ret;
}
int main(){
int n;
cin >> n;
getchar();
string s;
for(int i = 0; i < n; i ++ ){
getline(cin, s);
st[i].obj1 = s[0] - 'A';
if(s[1] == '='){
st[i].ret = 1;
st[i].num = s[2] - '0';
}
else{
st[i].ret = 0;
st[i].obj2 = s[2] - 'A';
st[i].num = s[3] - '0';
if(s[1] == '<') st[i].num *= -1;
}
}
int day[7];
int a, b, c, d, e, f, g;
for(a = 0; a < 7; a ++ ){
for(b = 0; b < 7; b ++ ){
for(c = 0; c < 7; c ++ ){
for(d = 0; d < 7; d ++ ){
for(e = 0; e < 7; e ++ ){
for(f = 0; f < 7; f ++ ){
for(g = 0; g < 7; g ++ ){
day[0] = a;
day[1] = b;
day[2] = c;
day[3] = d;
day[4] = e;
day[5] = f;
day[6] = g;
if(judge(day, n)) goto E;
}
}
}
}
}
}
}
E:
if(judge(day, n)){
char Day[7];
for(int i = 0; i < 7; i ++ ){
Day[day[i]] = i + 'A';
}
Day[7] = '\0';
puts(Day);
}
return 0;
}
7-113 完美的代价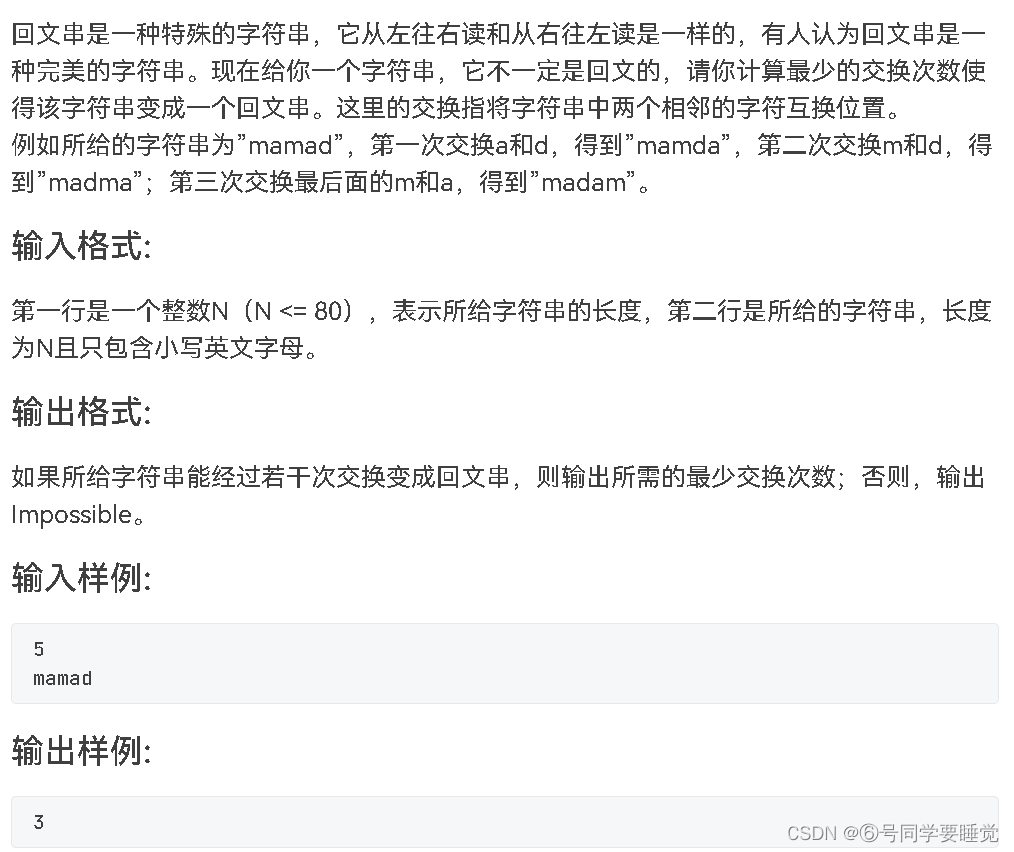
Impossible有两个条件:
1.当n为奇数,如果有两个及以上不能配对的,说明不符合回文串条件
2.当n为偶数,如果相同数字出现奇数次
#include<bits/stdc++.h>
using namespace std;
#define INF 0x3f3f3f3f
#define x first
#define y second
typedef long long LL;
const int N = 11;
int main(){
int n;
string s;
cin >> n >> s;
int ed = n - 1;
int step = 0;
bool flag = false;
for(int i = 0; i < ed; i ++ ){
for(int j = ed; j >= i; j -- ){
if(i == j){
if(n % 2 == 0 || flag == true){
cout << "Impossible" << endl;
return 0;
}
flag = true;
step += n / 2 - i;//当前位置到中间位置需要的步数
}
else if(s[i] == s[j]){
for(int k = j; k < ed; k ++ ){
swap(s[k], s[k + 1]);
step ++ ;
}
ed -- ;
break;//重点
}
}
}
cout << step << endl;
return 0;
}
L2-1 盲盒包装流水线 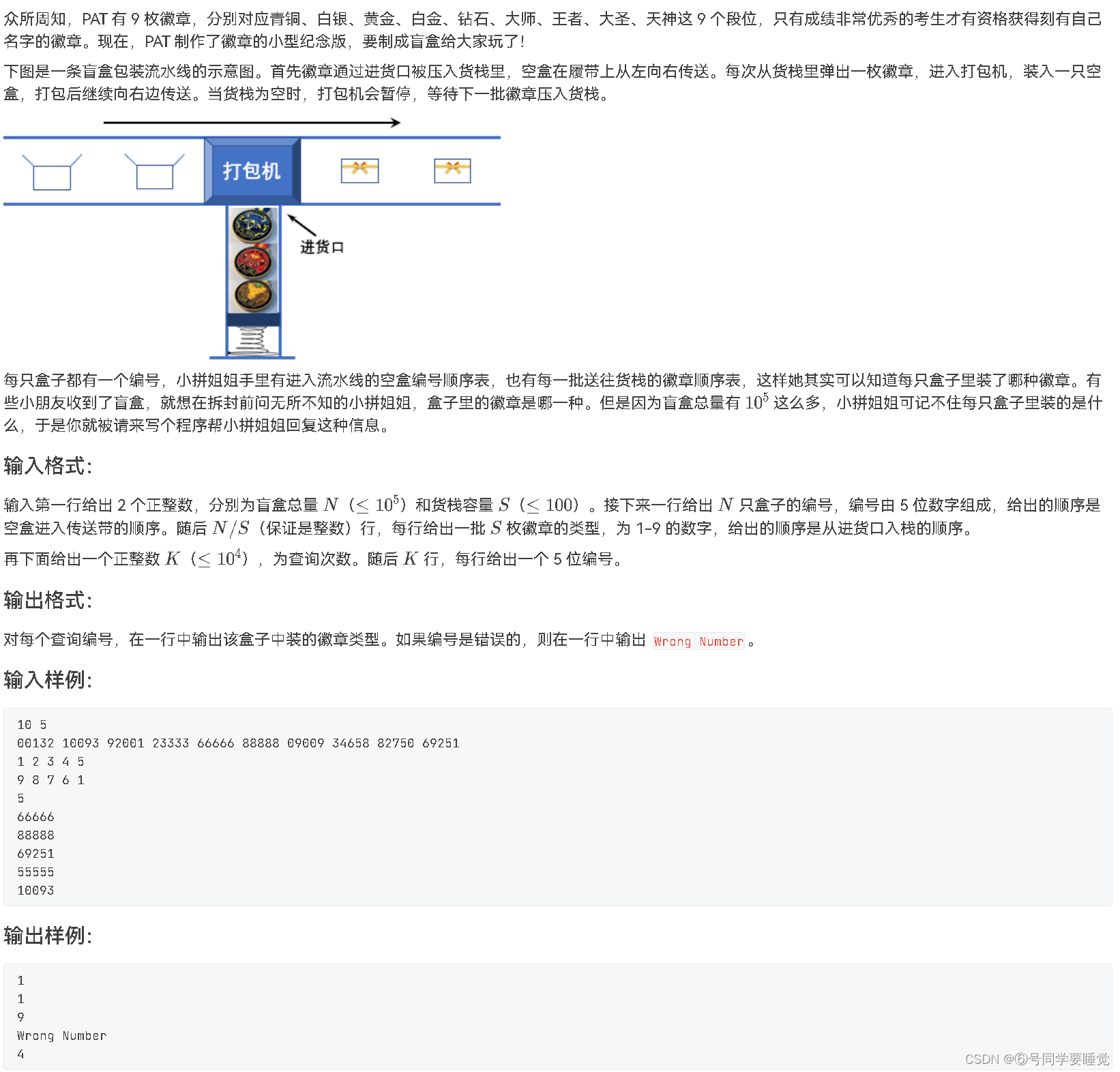
一开始只有22分,卡在了开的不够大,紫砂 ,现在st开的数据我不知道具体怎么算的 QAQ,感觉·就看样例的话就是N=可能的最大数据 / S本题样例5 就不会溢出了(
#include<bits/stdc++.h>
using namespace std;
#define INF 0x3f3f3f3f
#define x first
#define y second
typedef long long LL;
const int N = 1e5 + 10;
stack<int> st[50010];
queue<int> q;
int a[N];
int main(){
int n, s, k;
cin >> n >> s;
for(int i = 1; i <= n; i ++ ){
int x;
cin >> x;
q.push(x);
}
int col = n / s;
for(int i = 0; i < col; i ++ ){
for(int j = 0; j < s; j ++ ){
int x;
cin >> x;
st[i].push(x);
}
for(int j = 0; j < s; j ++ ){
int x = q.front();
q.pop();
int y = st[i].top();
st[i].pop();
a[x] = y;
}
}
cin >> k;
while(k -- ){
int x;
cin >> x;
if(a[x] == 0) cout << "Wrong Number" << endl;
else cout << a[x] << endl;
}
return 0;
}
L2-2 点赞狂魔 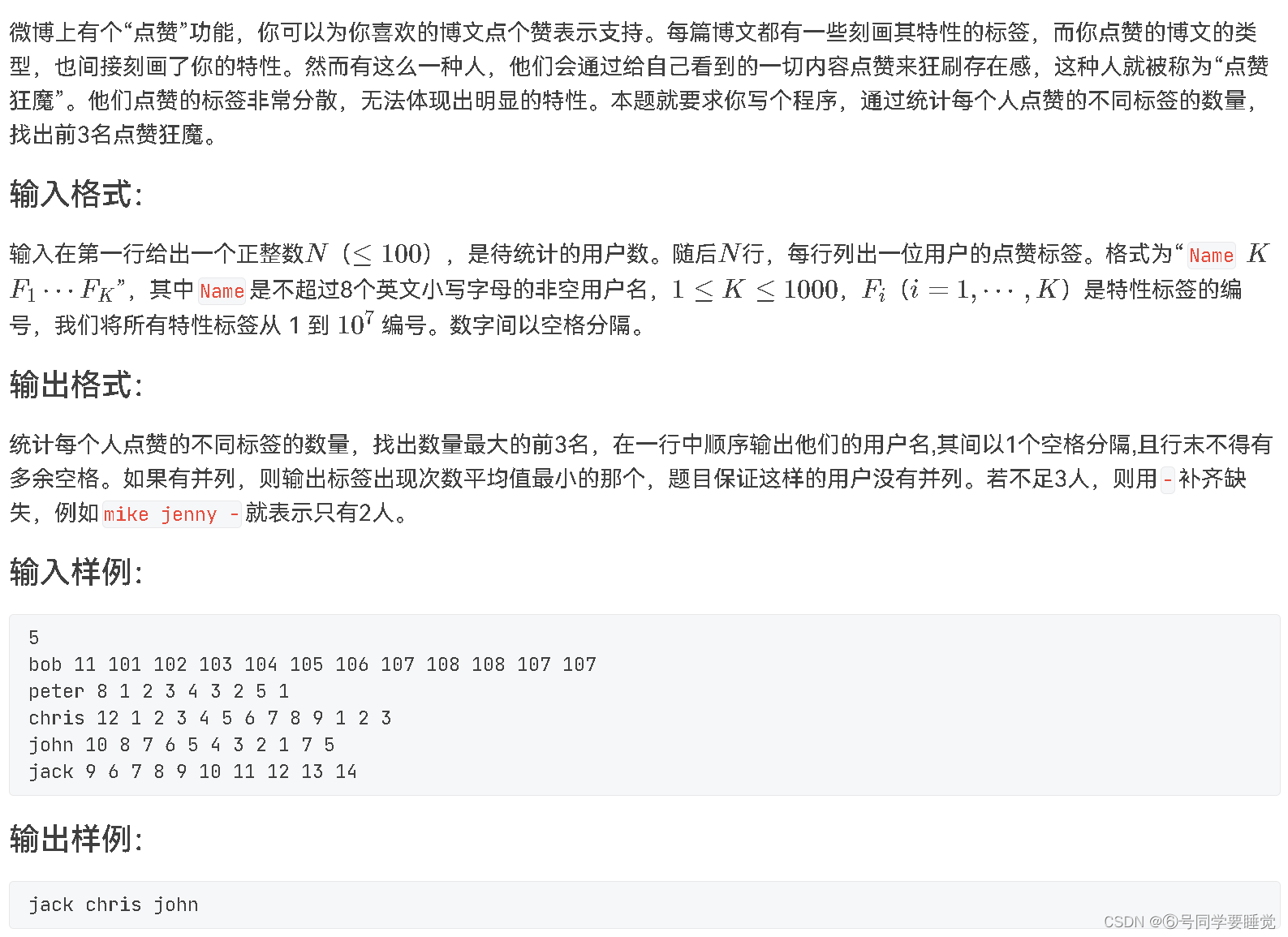
#include<bits/stdc++.h>
using namespace std;
#define INF 0x3f3f3f3f
#define x first
#define y second
typedef long long LL;
const int N = 110;
struct person{
string name;
set<LL> tag;
double ave;
}p[N];
bool cmp(person x, person y){
if(x.tag.size() == y.tag.size()) return x.ave < y.ave;
return x.tag.size() > y.tag.size();
}
int main(){
int n;
cin >> n;
for(int i = 0; i < n; i ++ ){
string s;
cin >> s;
p[i].name = s;
int k;
cin >> k;
for(int j = 0; j < k; j ++ ){//woc用while循环过不了
LL x;
cin >> x;
p[i].tag.insert(x);
}
p[i].ave = 1.0 * k / p[i].tag.size();
}
sort(p, p + n, cmp);
if(n == 0) cout << "- - -" << endl;
else if(n == 1) cout << p[0].name << " - -" << endl;
else if(n == 2) cout << p[0].name << " " << p[1].name << " -" << endl;
else cout << p[0].name << " " << p[1].name << " " << p[2].name << endl;
return 0;
}
L2-3 浪漫侧影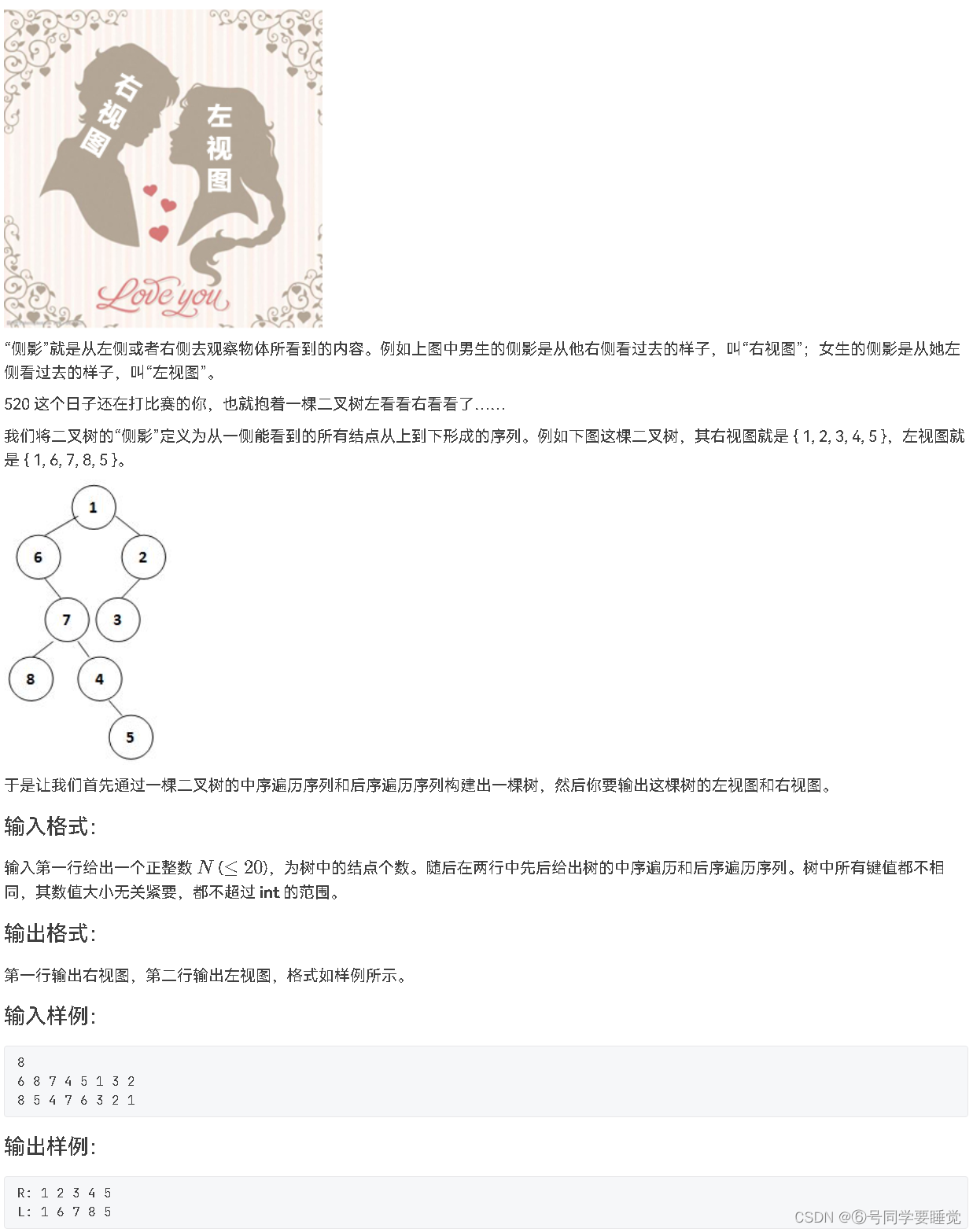
#include<bits/stdc++.h>
using namespace std;
#define INF 0x3f3f3f3f
#define x first
#define y second
typedef long long LL;
const int N = 1e5 + 10;
int midt[25], postt[25], tree[N], level;
void createTree(int *postt, int *midt, int n, int idx){
if(!n) return ;
int k = 0;
while(postt[n - 1] != midt[k]) k ++ ;
tree[idx] = midt[k];
level = max(level, idx);
createTree(postt, midt, k, idx * 2);
createTree(postt + k, midt + k + 1, n - k - 1, idx * 2 + 1);
}
int main(){
int n;
cin >> n;
for(int i = 0; i < n; i ++ ) cin >> midt[i];
for(int i = 0; i < n; i ++ ) cin >> postt[i];
createTree(postt, midt, n, 1);
for(int i = 1; i <= 22; i ++ ){
if(level < pow(2, i)){
level = i;
break;
}
}
cout << "R: ";
int cnt = 0, idx = 1;
while(cnt < level){
if(tree[idx]) cout << tree[idx];
if(tree[idx * 2 + 1]) idx = idx * 2 + 1;
else if(tree[idx * 2]) idx *= 2;
else{
idx *= 2;
while(!tree[idx]) idx -- ;
}
cnt ++ ;
if(cnt != level) cout << ' ';
else cout << endl;
}
cout << "L: ";
cnt = 0, idx = 1;
while(cnt < level){
if(tree[idx]) cout << tree[idx];
if(tree[idx * 2]) idx *= 2;
else if(tree[idx * 2 + 1]) idx = idx * 2 + 1;
else{
idx *= 2;
while(!tree[idx]) idx ++ ;
}
cnt ++ ;
if(cnt != level) cout << ' ';
else cout << endl;
}
return 0;
}