云函数
可以看做java或者php,作为后端服务
cloudfunctions/myCloud/index.js
exports.main = async (event, context) => {
const { name, age } = event
return `我是${name},今年${age}`
};
pages/index/index.vue
//callFunction方法 在前端和云端都可以调用另一个云函数
uniCloud.callFunction({
name: "myCloud",
data:{
name:"小白",
age:22
}
}).then(res => {
console.log(res)
})
浏览器
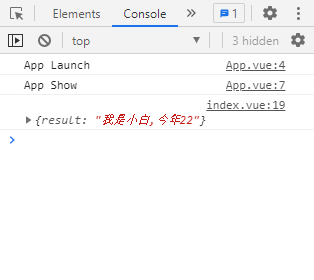
云函数通过传统方式操作数据库
查-cloudfunctions/cloudDome/index.js
const db = uniCloud.database();
exports.main = async (event, context) => {
// 获取 `users` 集合的引用
const collection = await db.collection('users').get()
return collection
};
查-pages/index/index.vue
<template>
<view class="content" v-for="item in user" :key="item._id">
{{item.name}} -- {{item.gender}}
</view>
</template>
<script>
import { reactive, toRefs } from "vue"
export default {
setup() {
const data = reactive({
user:{}
})
uniCloud.callFunction({
name: "cloudDome",
data:{}
}).then(res => {
data.user = res.result.data
})
return {
...toRefs(data)
}
}
}
</script>
查-浏览器
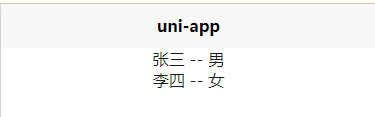
doc,指定 id 的记录的引用
const db = uniCloud.database();
exports.main = async (event, context) => {
const collection = await db.collection('users').doc("640aa8330c801c2ac9373731").get()
return collection
};
limit
const db = uniCloud.database();
exports.main = async (event, context) => {
const collection = await db.collection('users').limit(3).get()
return collection
};
skip,与limit实现翻页效果,skip指跳过的数据
const db = uniCloud.database();
exports.main = async (event, context) => {
const collection = await db.collection('users').limit(3).skip(3).get()
return collection
};
orderBy
const db = uniCloud.database();
exports.main = async (event, context) => {
const collection = await db.collection('users').orderBy("_id","desc").get()
return collection
};
field,指定返回字段
const db = uniCloud.database();
exports.main = async (event, context) => {
const collection = await db.collection('users').field({ 'name': true,'_id':false }).get()
return collection
};
where与查询筛选指令 Query Command
const db = uniCloud.database();
const dbCmd = db.command
exports.main = async (event, context) => {
const collection = await db.collection('users').where({
//age:dbCmd.gt(20).and(dbCmd.lt(48))
age:dbCmd.and(dbCmd.gt(8),dbCmd.lt(48))
//age:dbCmd.or(dbCmd.and(dbCmd.gt(8),dbCmd.lt(48)),dbCmd.eq(50))
}).get()
return collection
};
正则表达式查询
const db = uniCloud.database();
const dbCmd = db.command
exports.main = async (event, context) => {
const { keyWord } = event
const collection = await db.collection('users').where({
name: new RegExp(keyWord,'ig') //i表示忽略大小写 g表示全局搜索
}).get()
return collection
};
增-cloudfunctions/cloudDome/index.js
const db = uniCloud.database();
exports.main = async (event, context) => {
const obj = {
"name": "小红",
"gender": "未知",
"tel": 1333333333,
"mail": "564654654@qq.com"
}
const collection = await db.collection('users').add(obj)
return collection
};
增-pages/index/index.vue
uniCloud.callFunction({
name: "cloudDome",
data:{}
}).then(res => {
console.log(res)
})
增-数据库

改-cloudfunctions/cloudDome/index.js
const db = uniCloud.database()
exports.main = async (event, context) => {
const { keyWord } = event
const collection = await db.collection('users').doc("640aa8330c801c2ac9373731").update({
name:'张三被改名了',
age:0
})
return {
message:'修改成功',
collection
}
};
改-数据库

批量改-cloudfunctions/cloudDome/index.js
const db = uniCloud.database()
const dbCmd = db.command
exports.main = async (event, context) => {
const { keyWord } = event
const collection = await db.collection('users').where({
age:dbCmd.lt(20)
}).update({
text:'小于二十岁的小年轻'
})
return {
message:'修改成功',
collection
}
};
批量改-数据库
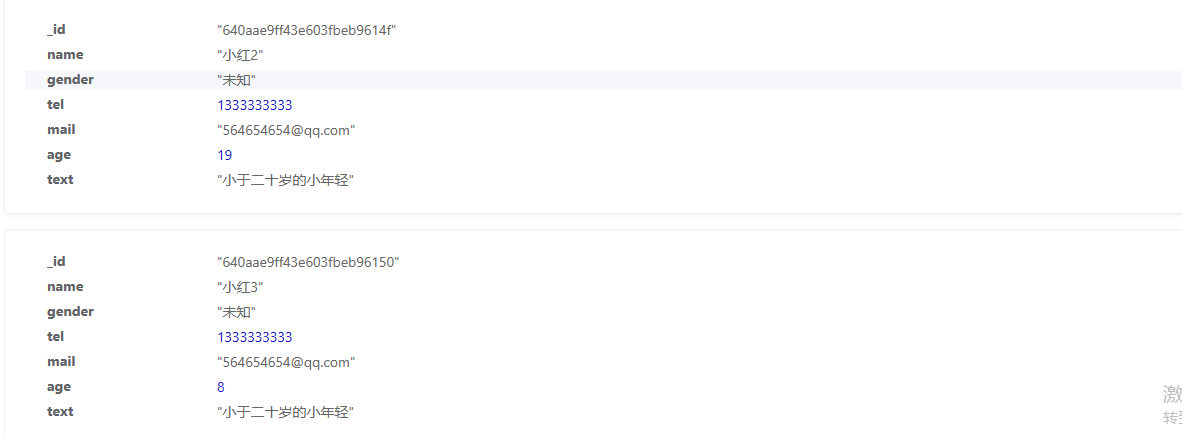
push,向数组尾部追加元素
const db = uniCloud.database()
const dbCmd = db.command
exports.main = async (event, context) => {
const { keyWord } = event
const collection = await db.collection('users').doc("640aa867f43e603fbeb7771b").update({
like:dbCmd.push(["烟花"])
})
return {
message:'修改成功',
collection
}
};
//中间添加
const collection = await db.collection('users').doc("640aa867f43e603fbeb7771b").update({
like: dbCmd.push({
each: ["大","白"],
position: 1
})
})
inc,多用户的点赞
const collection = await db.collection('users').doc("640aa867f43e603fbeb7771b").update({
love:dbCmd.inc(1)
})
删-cloudfunctions/cloudDome/index.js
const db = uniCloud.database()
exports.main = async (event, context) => {
const collection = await db.collection('users').doc("640aae9ff43e603fbeb96150").remove()
return {
message: '修改成功',
collection
}
};
批量删-cloudfunctions/cloudDome/index.js
const db = uniCloud.database()
const dbCmd = db.command
exports.main = async (event, context) => {
const collection = await db.collection('users').where({
name:/小/ig
}).remove()
return {
message: '修改成功',
collection
}
};