一、需求分析
完成一个简单的用户信息管理系统,超级管理员可以登录本系统,查询用户信息、实现用户的管理功能。
1.1功能:
主要操作和管理的对象:用户。
用户分为两类:超级管理员/普通用户。
登录功能(只针对超管有效,普通用户不能登录)
列表查询分页列表功能
添加用户(普通用户/超级管理员)
修改用户
删除用户(单条删除和多条删除)
1.2使用的技术
该系统主要选择了Java的SpringBoot框架技术进行开发,采用了分层的架构,每个层次在保持独立的同时具有一定松散性,这样可以实现系统开发结构的清晰性,从而提高了系统的开发效率和性能。集成Mybatis,后台数据库使用MySQL对数据进行存储,以及Spring MVC,还添加了拦截器/统一异常处理/统一数据返回。
二、项目准备
2.1创建数据库
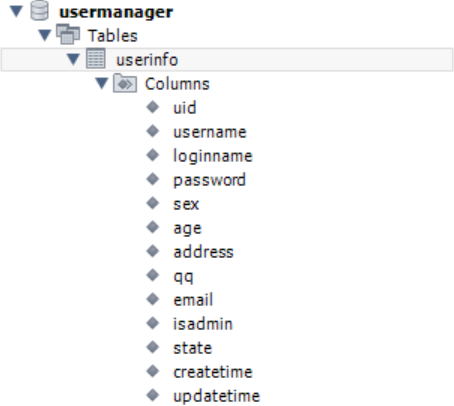
drop database if exists usermanager;
create database usermanager character set 'utf8mb4';
create table userinfo(
uid int primary key auto_increment,
username varchar(250) not null,
loginname varchar(250) not null,
password varchar(65) not null,
sex varchar(2) default '男',
age int default 0,
address varchar(250) ,
qq varchar(250),
email varchar(250),
isadmin bit default 0,
state int default 1,
createtime datetime defalt now(),
updatetime datetime default noe()
)default charset = 'utf8mb4';
2.2搭建SMM项目
配置连接数据库字符串和mybatis xml保存路径
# 开发环境配置文件
# 配置数据库的连接字符串
spring:
datasource:
url: jdbc:mysql://127.0.0.1/usermanager?characterEncoding=utf8
username: root
password: 200177
driver-class-name: com.mysql.cj.jdbc.Driver
# 设置 Mybatis 的 xml 保存路径
mybatis:
mapper-locations: classpath:mapper/**Mapper.xml
configuration: # 配置打印 MyBatis 执行的 SQL
log-impl: org.apache.ibatis.logging.stdout.StdOutImpl
2.3添加公共模块
(1)创建实体类
/**
* 用户实体类
*/
@Data
public class UserInfo {
private int uid;
private String username;
private String loginname;
private String password;
private String sex;
private int age;
private String address;
private String qq;
private String email;
private boolean isadmin;
private int state;
private String createtime;
private String updatetime;
}
(2)将工具类hutool添加项目中
<dependency>
<groupId>cn.hutool</groupId>
<artifactId>hutool-all</artifactId>
<version>5.8.12</version>
</dependency>
(3)添加统一异常的处理器/添加用户登录拦截器/添加统一返回格式
/**
* 统一异常拦截处理类
*/
@RestControllerAdvice
public class MyExceptionAdvice {
@ExceptionHandler(Exception.class)
public Object exceptionAdvice(Exception e) {
HashMap<String, Object> result = new HashMap<>();
result.put("state", -1);
result.put("msg", "程序出现异常:" + e.getMessage());
result.put("data", "");
return result;
}
}
添加用户登录拦截器需要先创建自定义拦截器,然后将自定义拦截器加入到系统配置中,并且设置拦截规则,使用户必须先进入登录界面,不能先进入更新等界面。
/**
* 全局变量
*/
public class ConstVariable {
// session 中的用户 id
public static final String USER_SESSION_KEY = "userinfo_session_key";
}
/**
* 自定义用户拦截器
*/
@Component
public class LoginIntercept implements HandlerInterceptor {
/**
* true 表示用户已经登录,会继续访问目标方法
* false 表示未登录,跳转到登录页面
*/
public boolean preHandle(HttpServletRequest request,
HttpServletResponse response, Object handler) throws Exception {
HttpSession session = request.getSession(false);
if (session != null && session.getAttribute(ConstVariable.USER_SESSION_KEY) != null) {
// 表示已经登录
return true;
}
// 执行到此行表示未登录,跳转到登录页面
response.sendRedirect("/login.html");
return false;
}
}
/**
* 系统配置文件类
*/
@Configuration
public class AppConfig implements WebMvcConfigurer {
@Autowired
private LoginIntercept loginIntercept;
@Override
public void addInterceptors(InterceptorRegistry registry) {
registry.addInterceptor(loginIntercept).
addPathPatterns("/**").
excludePathPatterns("/login").
excludePathPatterns("/css/**").
excludePathPatterns("/fonts/**").
excludePathPatterns("/images/**").
excludePathPatterns("/js/**").
excludePathPatterns("/**/login.html");
}
}
/**
* 返回统一的数据格式
*/
@ControllerAdvice
public class MyResponseAdvice implements ResponseBodyAdvice {
@Override
public boolean supports(MethodParameter returnType, Class converterType) {
return true;
}
@Override
public Object beforeBodyWrite(Object body, MethodParameter returnType, MediaType selectedContentType, Class selectedConverterType, ServerHttpRequest request, ServerHttpResponse response) {
HashMap<String, Object> result = new HashMap<>();
result.put("state", 1);
result.put("msg", "");
result.put("data", body);
return result;
}
}
2.4创建密码工具
添加加密工具的目的主要是为了防止外部人员获取内部信息比如所有用户的密码。
在添加和更新时都要使用加密,在超级管理员登陆的时候需要使用解密才能登陆上。
2.4.1加密
public static String encrypt(String password) {
// 随机盐值
String salt = IdUtil.simpleUUID();
// 密码(md5(随机盐值+密码))
String finalPassword = SecureUtil.md5(salt + password);
return salt + "$" + finalPassword;
}
2.4.2解密
public static boolean decrypt(String password, String securePassword) {
boolean result = false;
if (StringUtils.hasLength(password) && StringUtils.hasLength(securePassword)) {
if (securePassword.length() == 65 && securePassword.contains("$")) {
String[] securePasswordArr = securePassword.split("\\$");
// 盐值
String slat = securePasswordArr[0];
String finalPassword = securePasswordArr[1];
// 使用同样的加密算法和随机盐值生成最终加密的密码
password = SecureUtil.md5(slat + password);
if (finalPassword.equals(password)) {
result = true;
}
}
}
return result;
}
三、功能实现
3.1登录功能
3.1.1实现思路
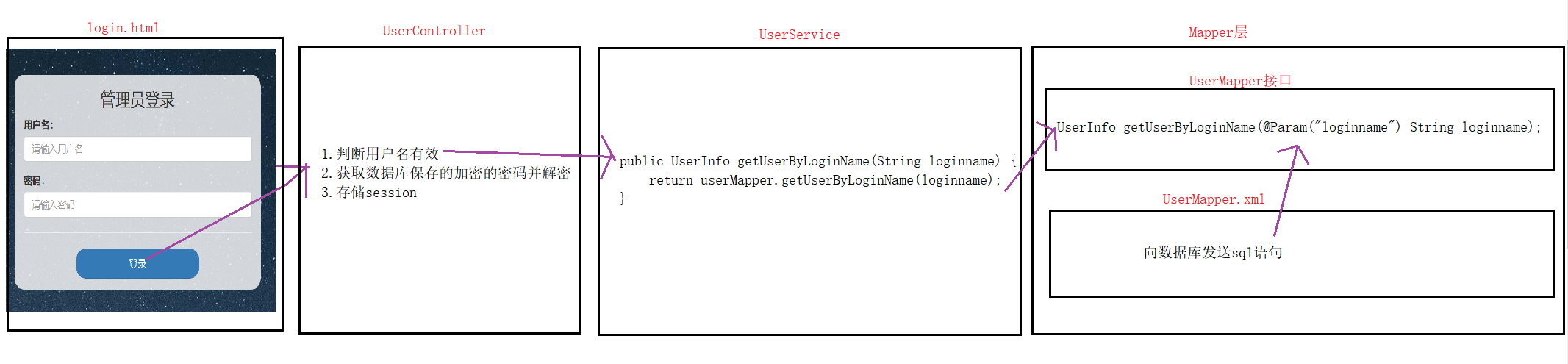
3.1.2成果图
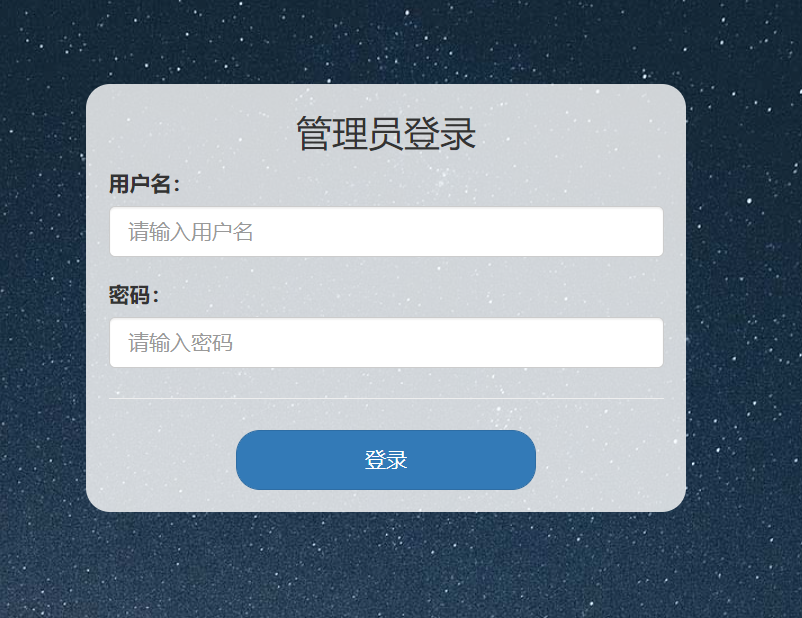
3.2列表功能
3.2.1实现思路
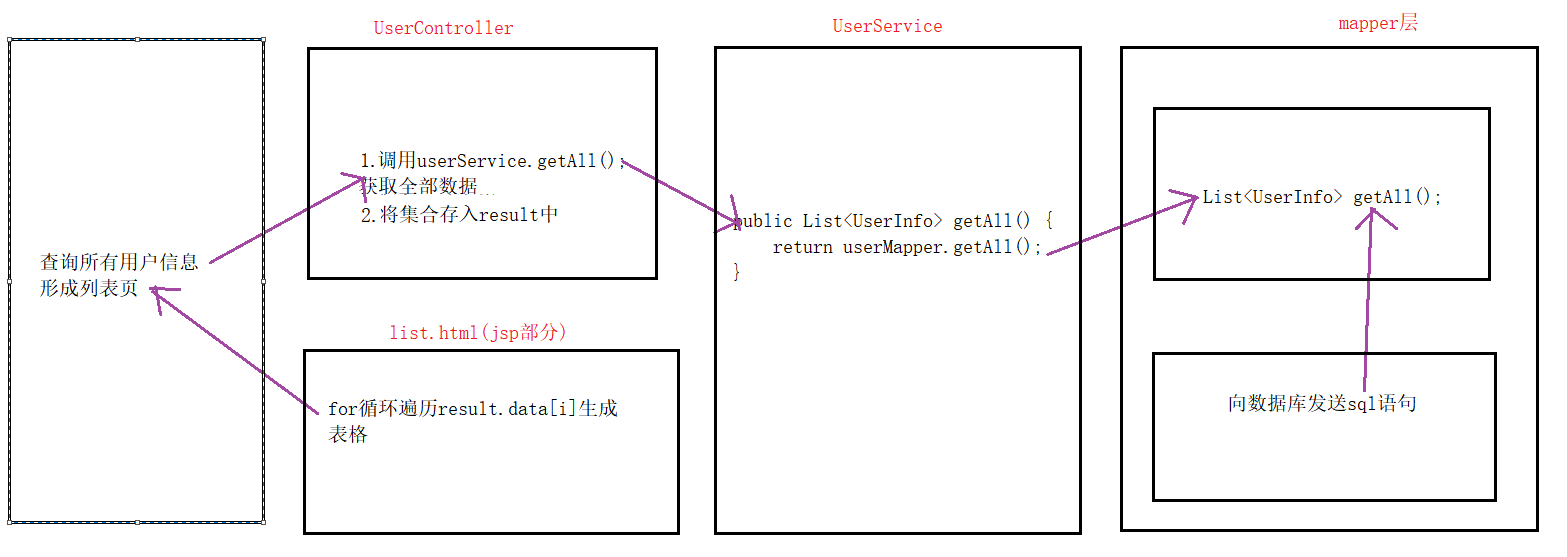
3.2.2成果图
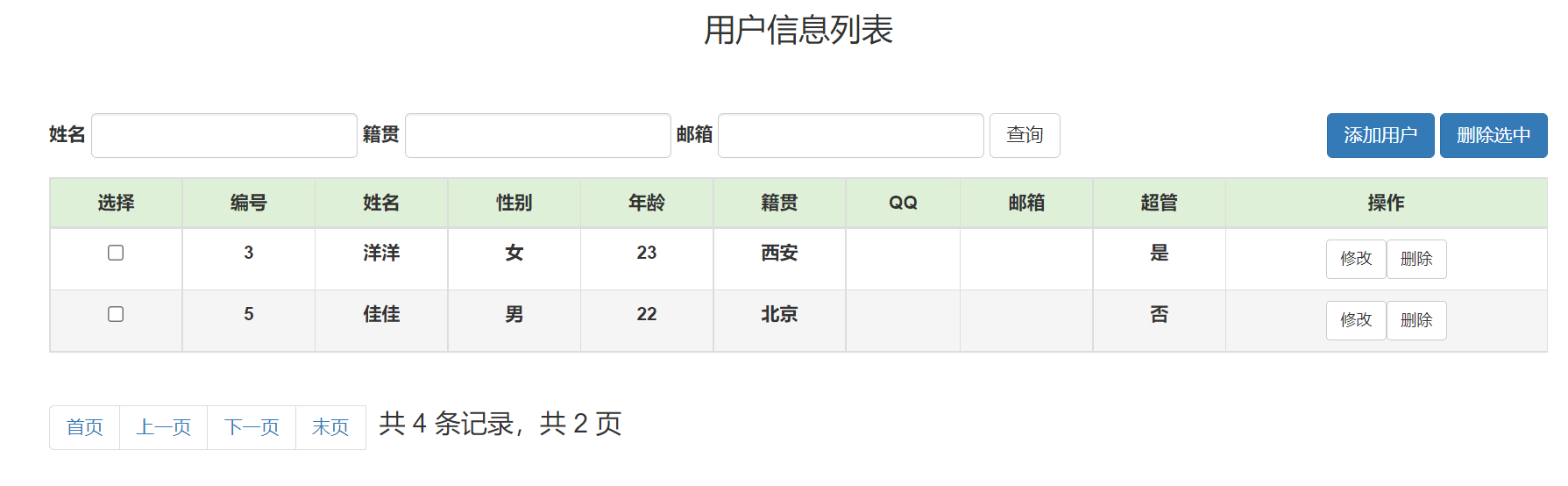
3.3添加功能
3.3.1实现思路
在添加界面中,需要注意有两个名字,一个登录名,一个姓名,登录名是不可以重复的。
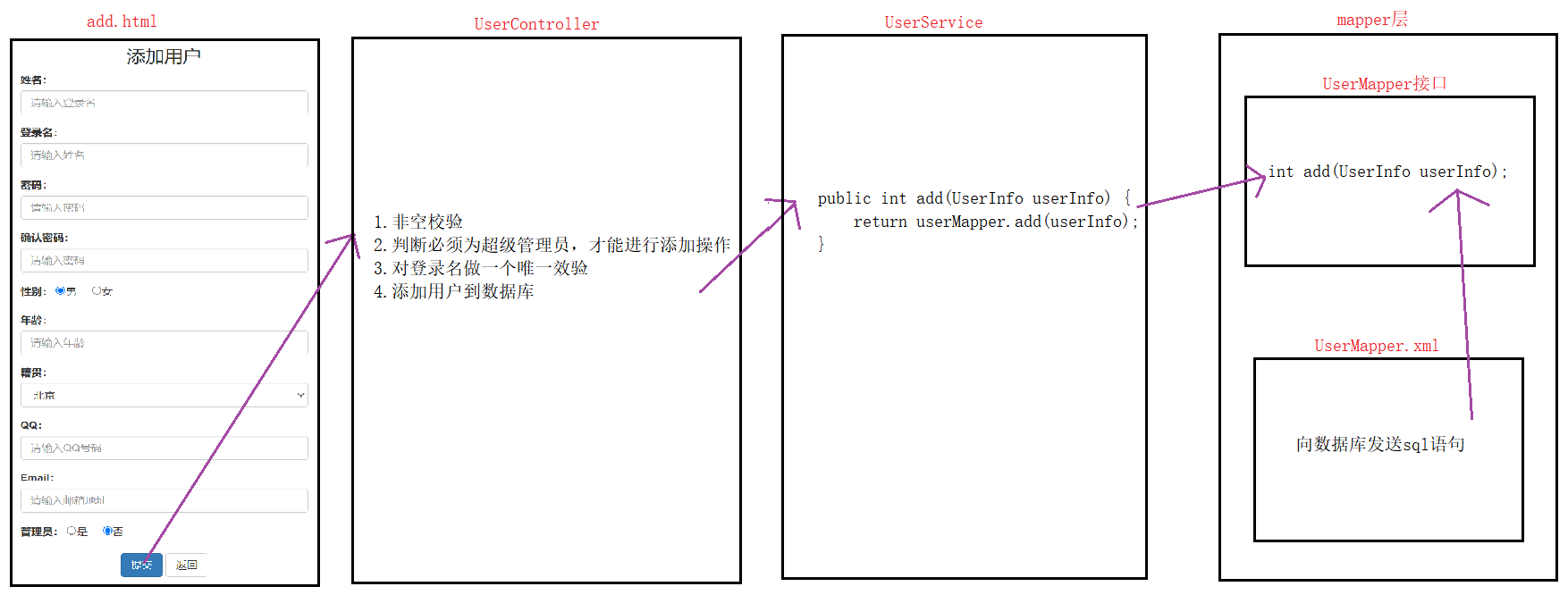
3.3.2成果图
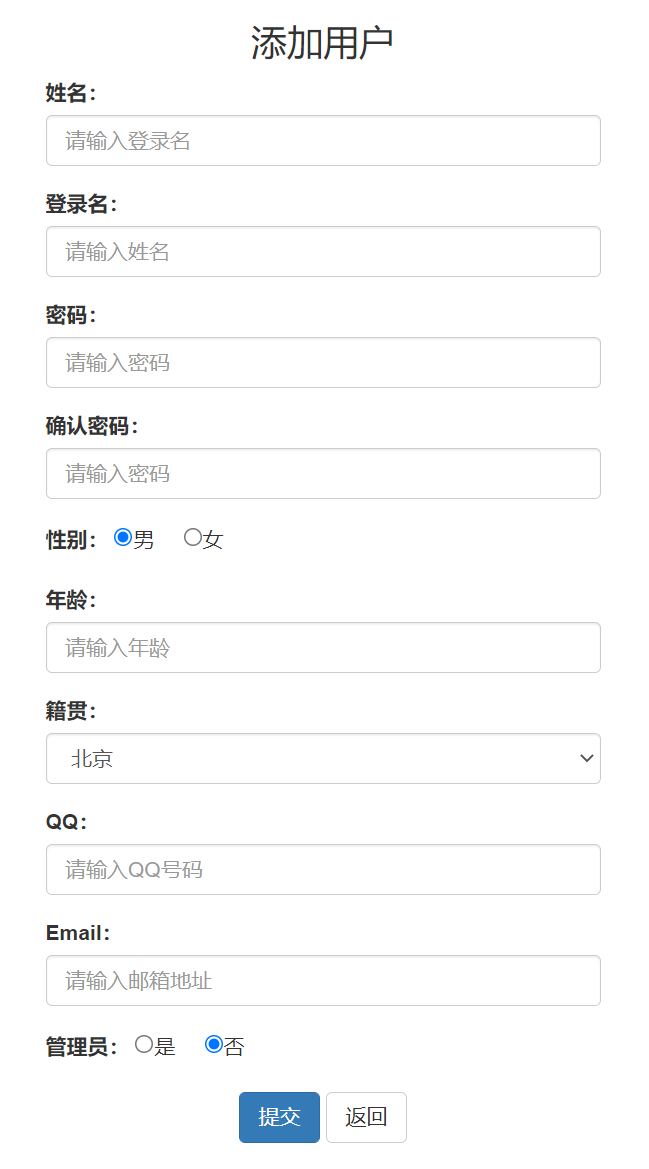
3.4删除功能
3.4.1实现思路
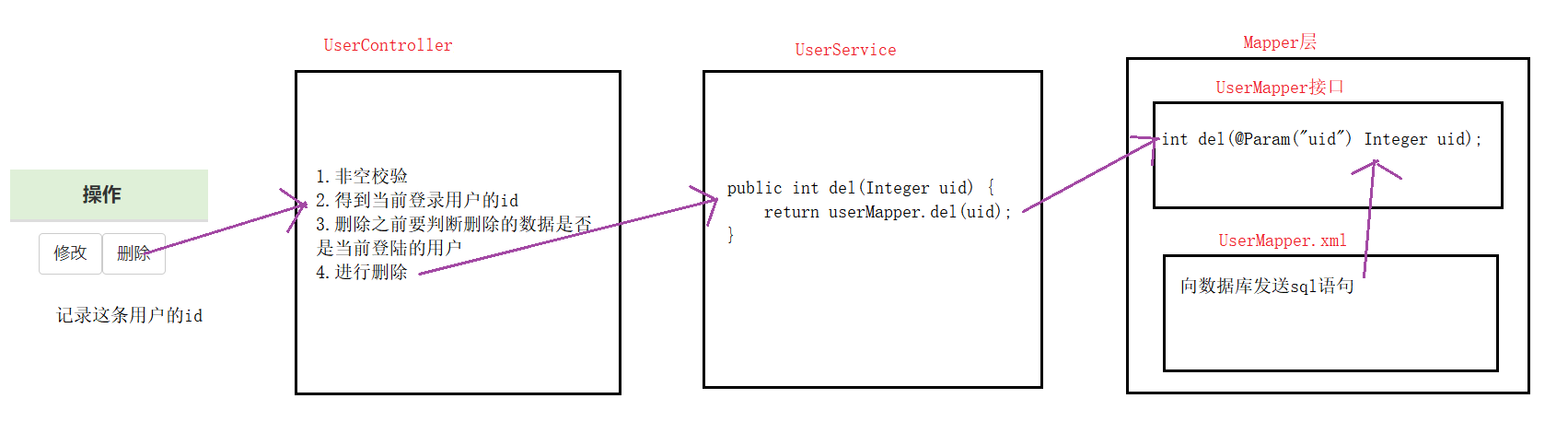
3.4.2成果图
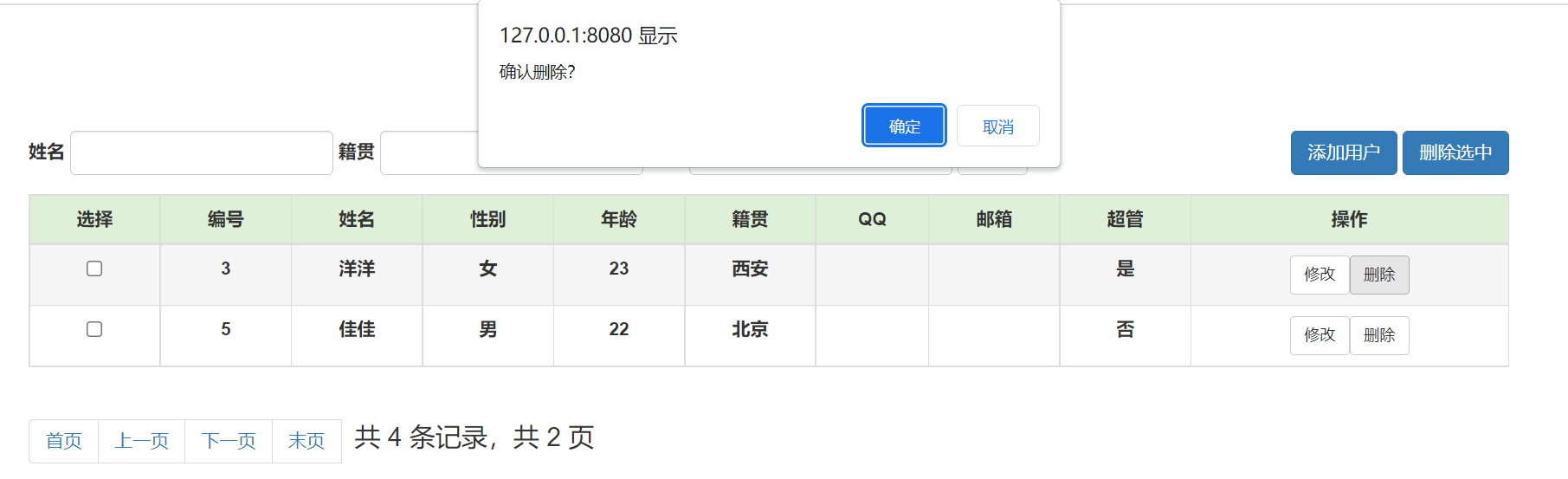
3.5修改功能
3.5.1实现思路
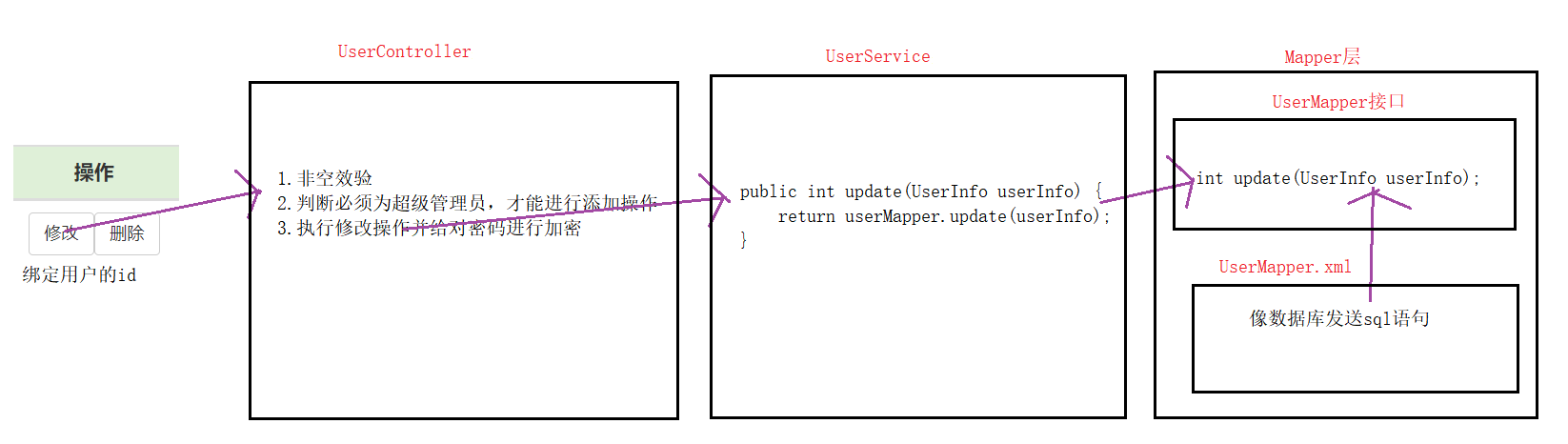
3.5.2成果图
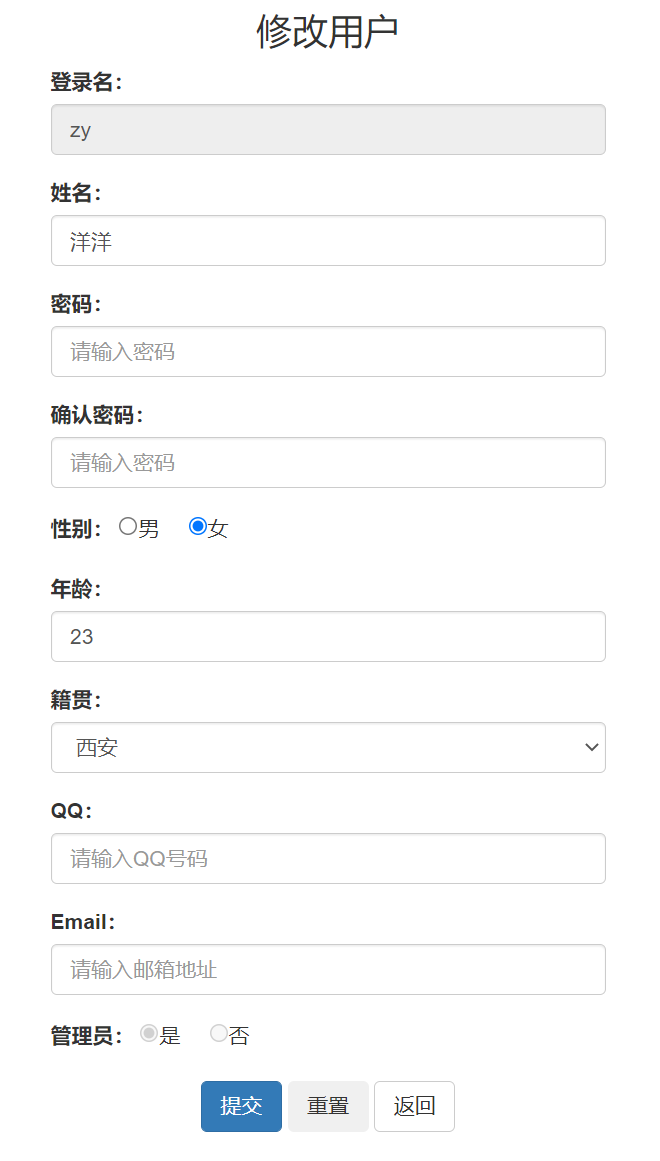
3.6多选删除功能
3.6.1实现思路
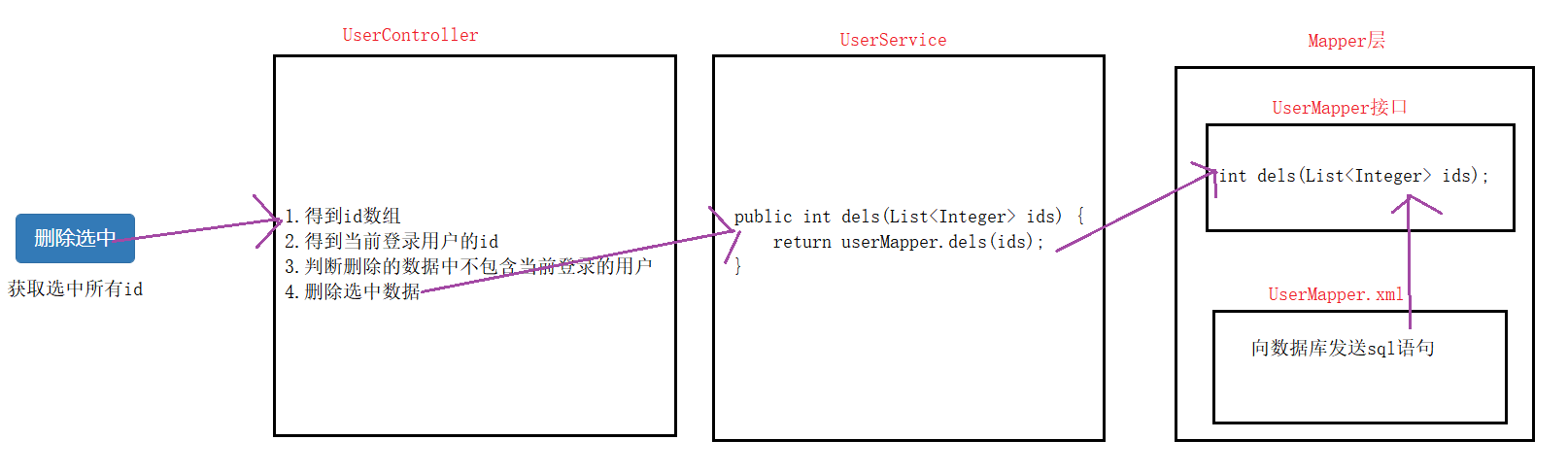
3.6.2成果图
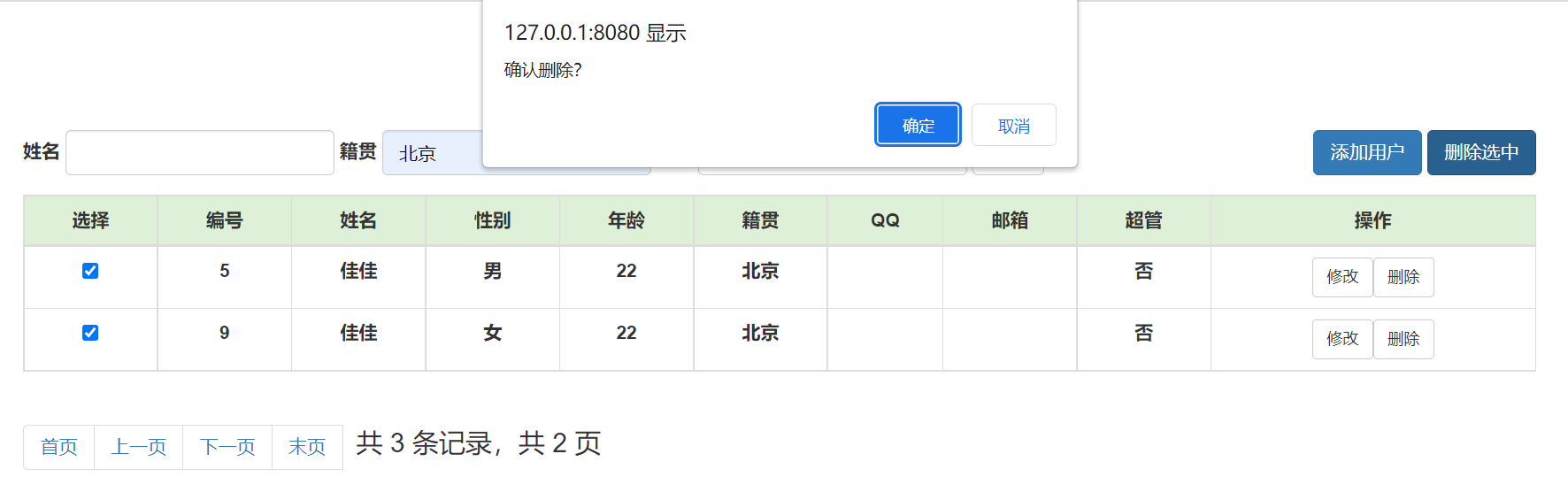
3.7分页功能
3.7.1实现思路
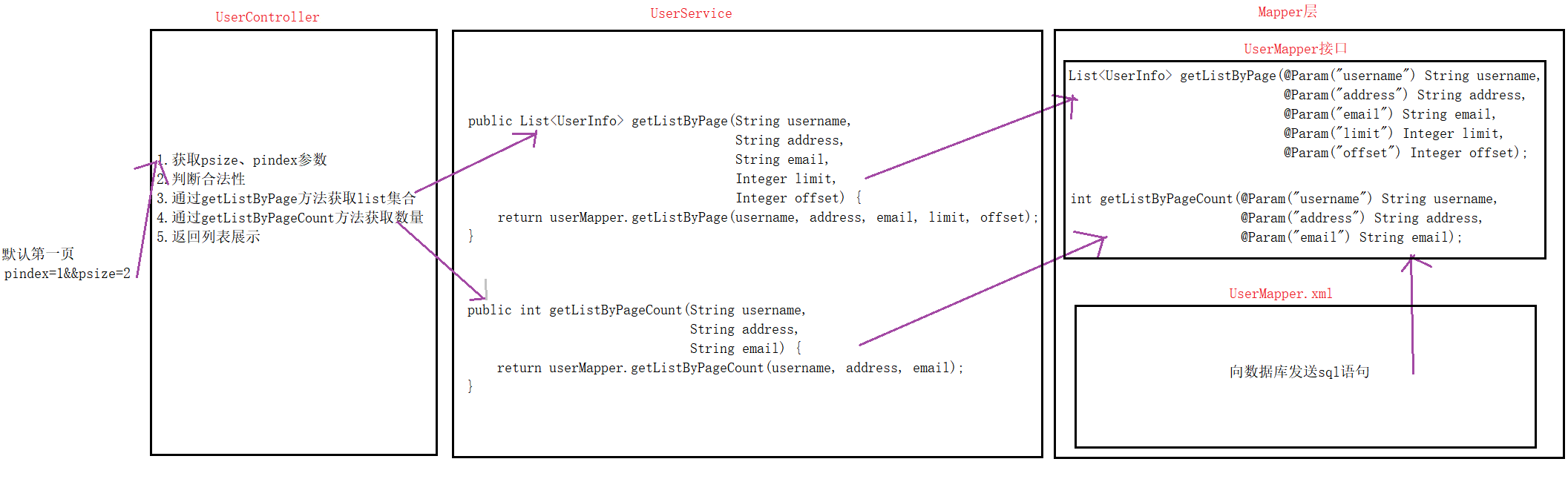
3.7.2成果图
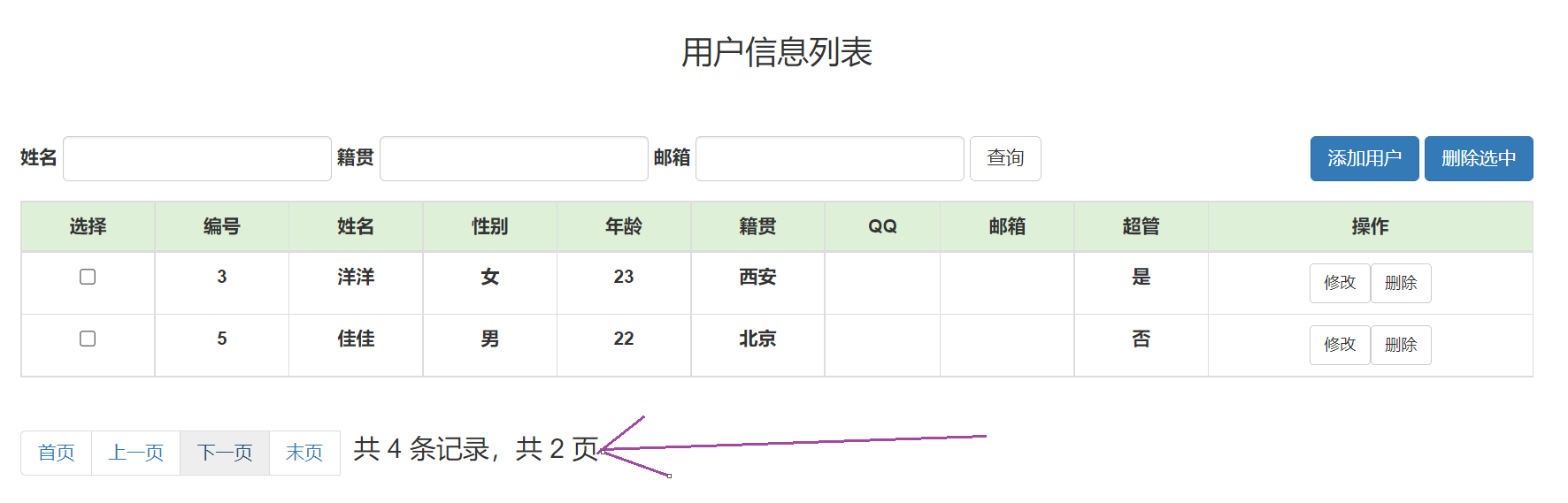
3.8条件查询功能
3.8.1实现思路
在jsp中利用jQuery获取姓名、地址、邮件的值
然后getList()进行查询。
3.8.2成果图
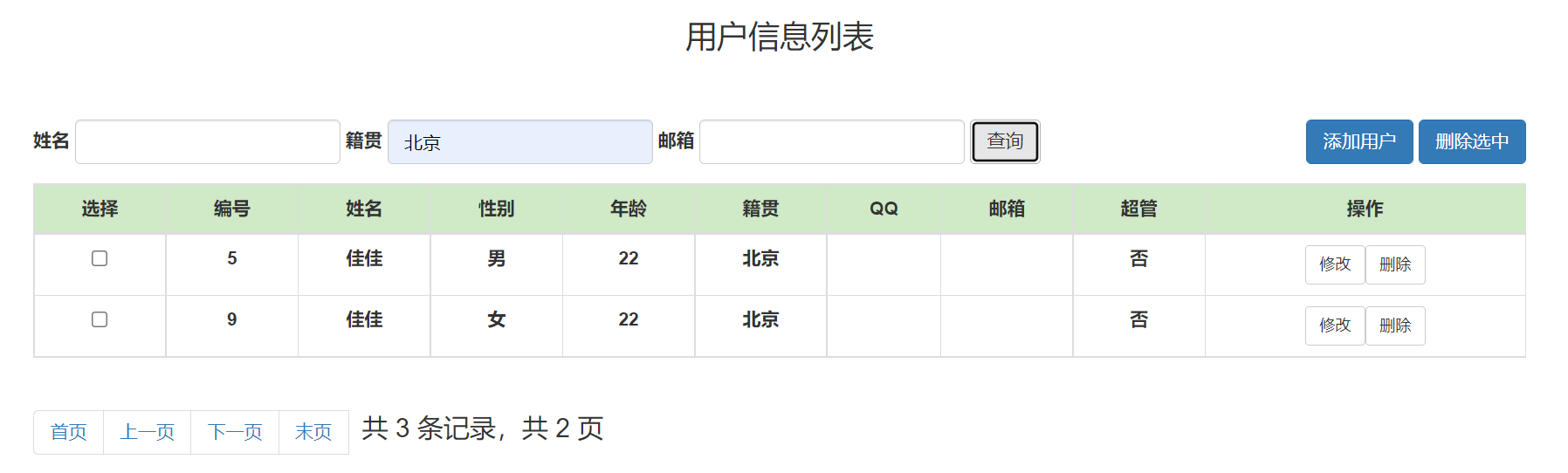