bool与引用类型
bool类型
介绍与使用
bool(布尔类型)
大小:1个字节
返回值有两个:1(true),0(false)
#include<iostream>
using namespace std;
int main()
{
bool a = false;
bool b = true;
cout << "a = " << a << endl;
cout << "b = " << b << endl;
system("pause");
return 0;
}
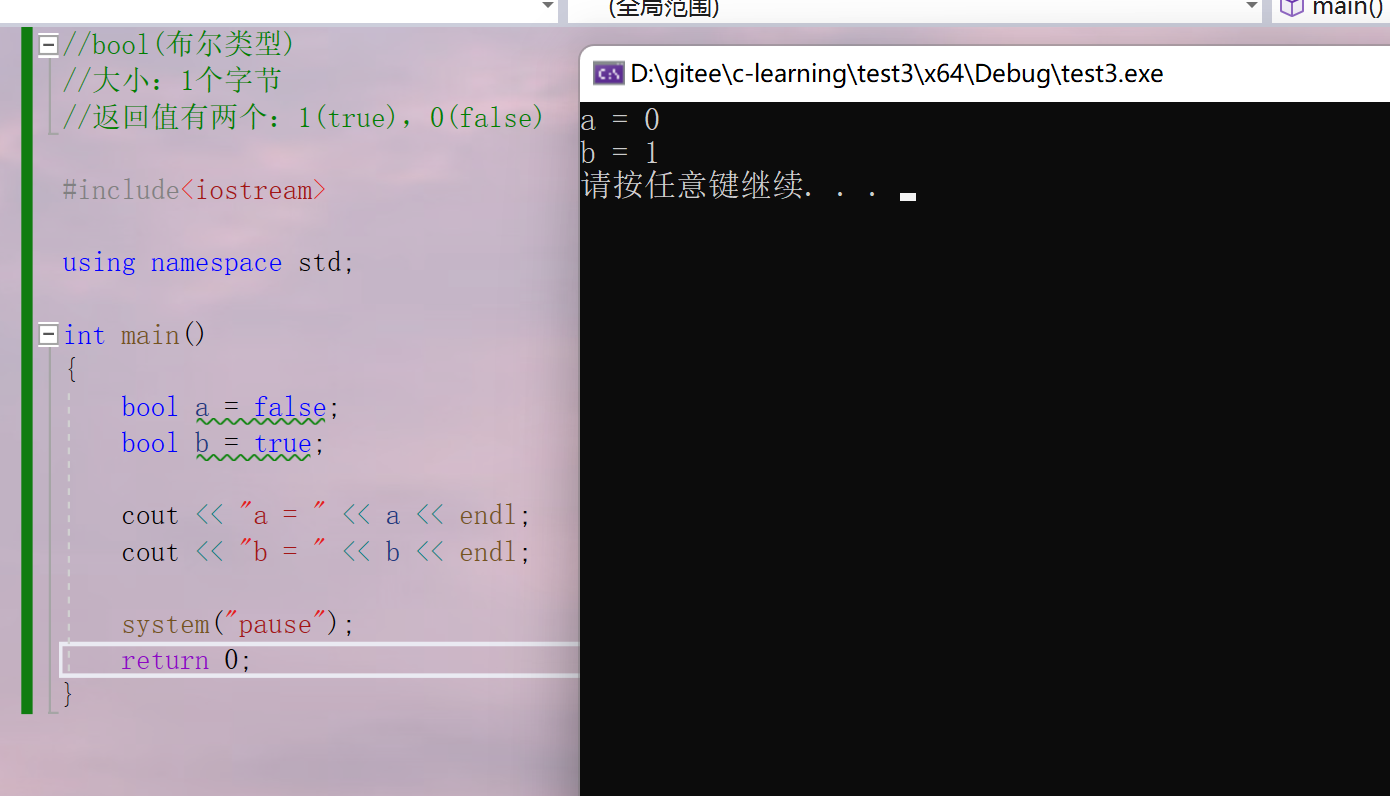
bool类型的用途
1.作某功能的开关
2.做标记 -->查找类的操作
3.做函数返回值
引用类型
可以当做一个起别名的用法
#include<iostream>
using namespace std;
int main()
{
int a = 1;
int& b = a; //起别名,a 的别名为 b,
//就跟一个人的小名一样,a 的小名是b, a就是b
system("pause");
return 0;
}
左值引用
当作函数参数,直接修改实参,防止拷贝木的产生
#include<iostream>
using namespace std;
void swap(int& a, int& b) //当作函数参数,直接修改实参
{
int c = a;
a = b;
b = c;
}
int main()
{
int a = 10;
int b = 11;
swap(a, b);
cout << "a = " << a << endl << "b = " << b << endl;
system("pause");
return 0;
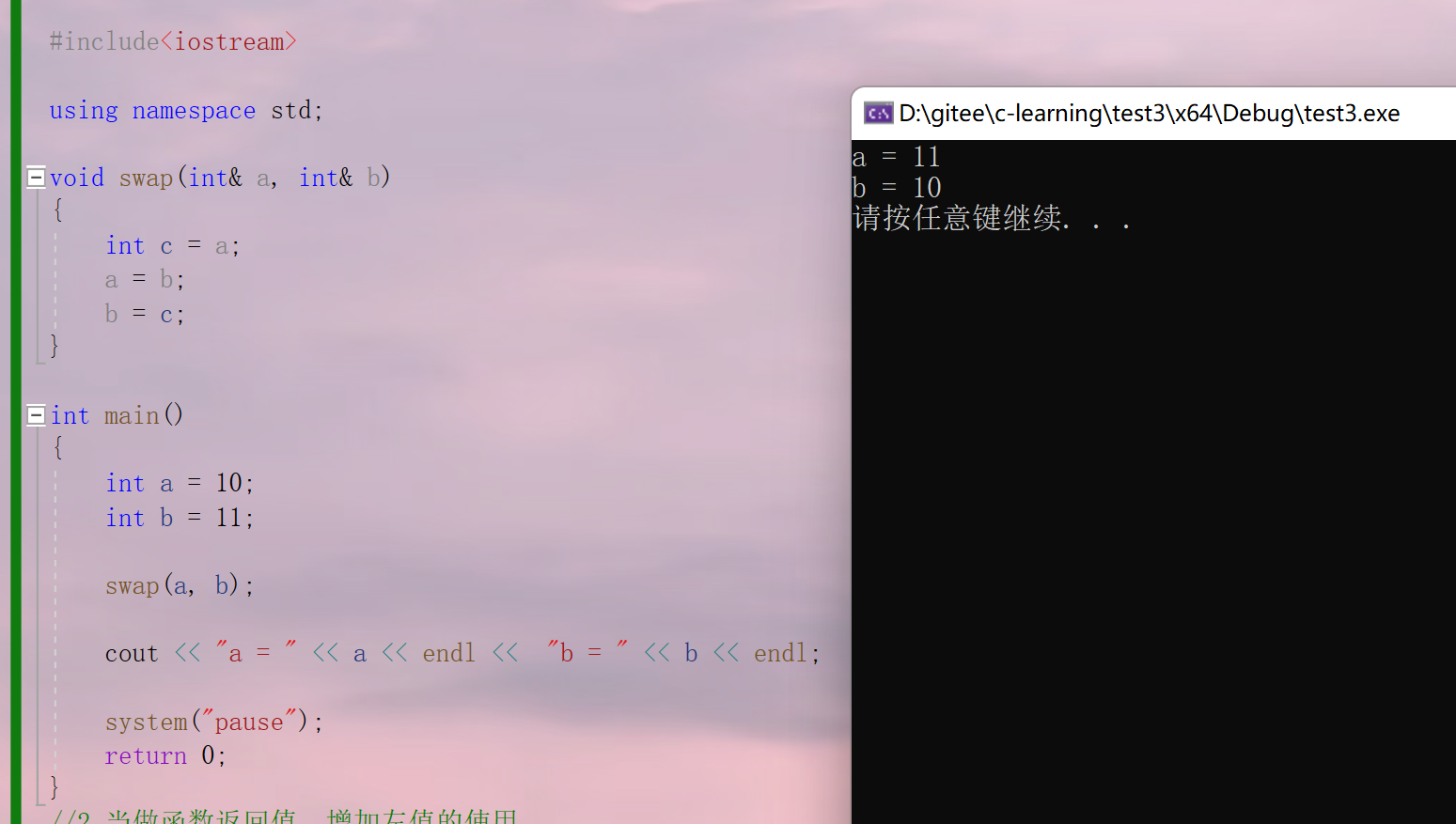
2.当做函数返回值,增加左值的使用
#include<iostream>
using namespace std;
int a = 1;
int b = 1;
int& mm()
{
return a;
}
int MM()
{
return b;
}
int main()
{
mm() = 2; //正确通过左值引用,可以进行修改
MM() = 4; //错误,不能对左值进行修改
system("pause");
return 0;
}
右值引用
给右边的取别名
给常量起别名
1.用const 给常量起别名
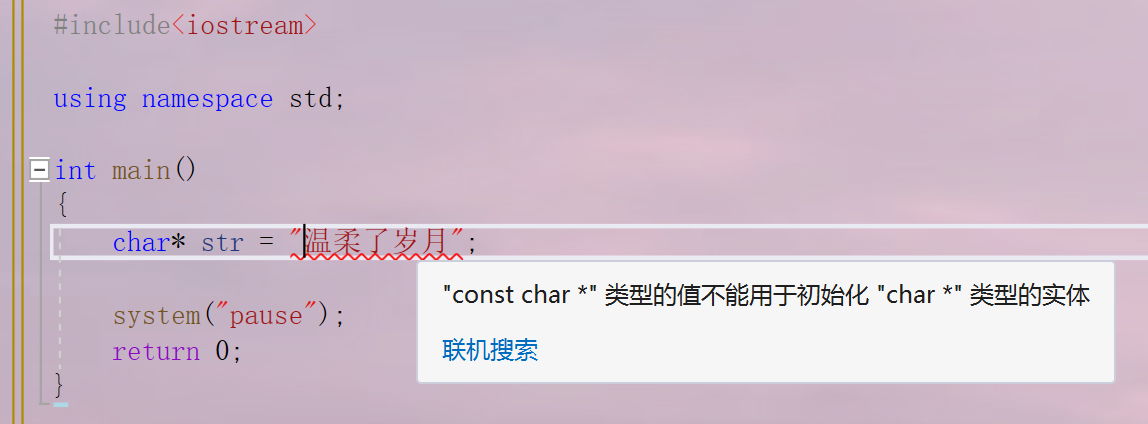
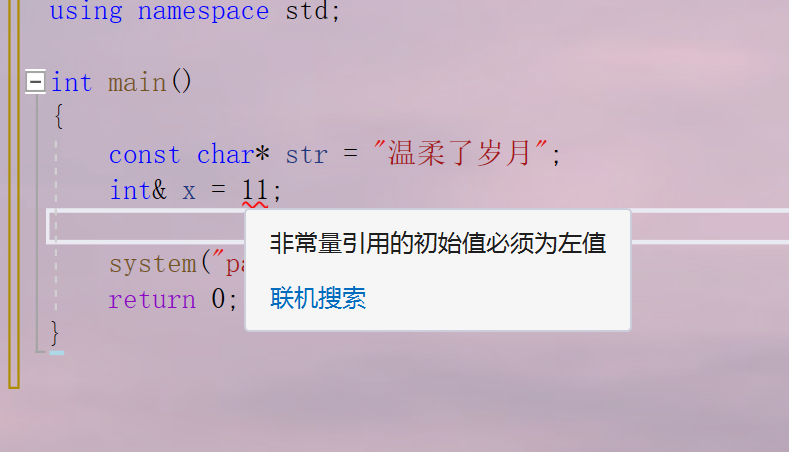
#include<iostream>
using namespace std;
int main()
{
const char* str = "温柔了岁月";
const int& x = 11;
system("pause");
return 0;
}
但用const有一个局限性,那就是不能进行修改
2.所以一般采用第二种方法,右值引用来解决(&&)
#include<iostream>
using namespace std;
int main()
{
const char* str = "温柔了岁月";
const int& x = 11;
int&& a = 11; //右值引用
a = 22; //修改值
system("pause");
return 0;
}
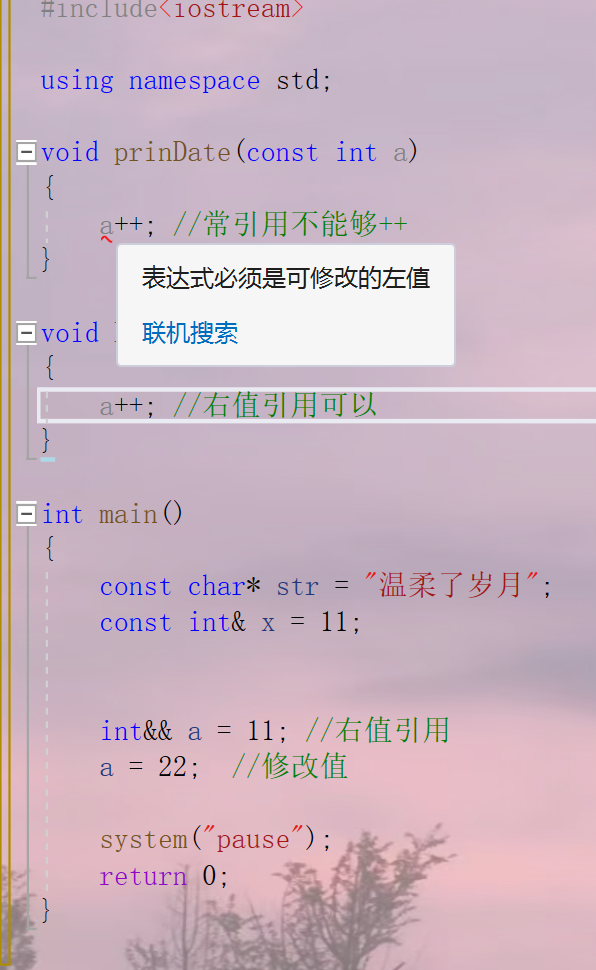
move()函数的应用
这里需要注意的是右值引用只能传入左值,不然就会出现如图所示的报错
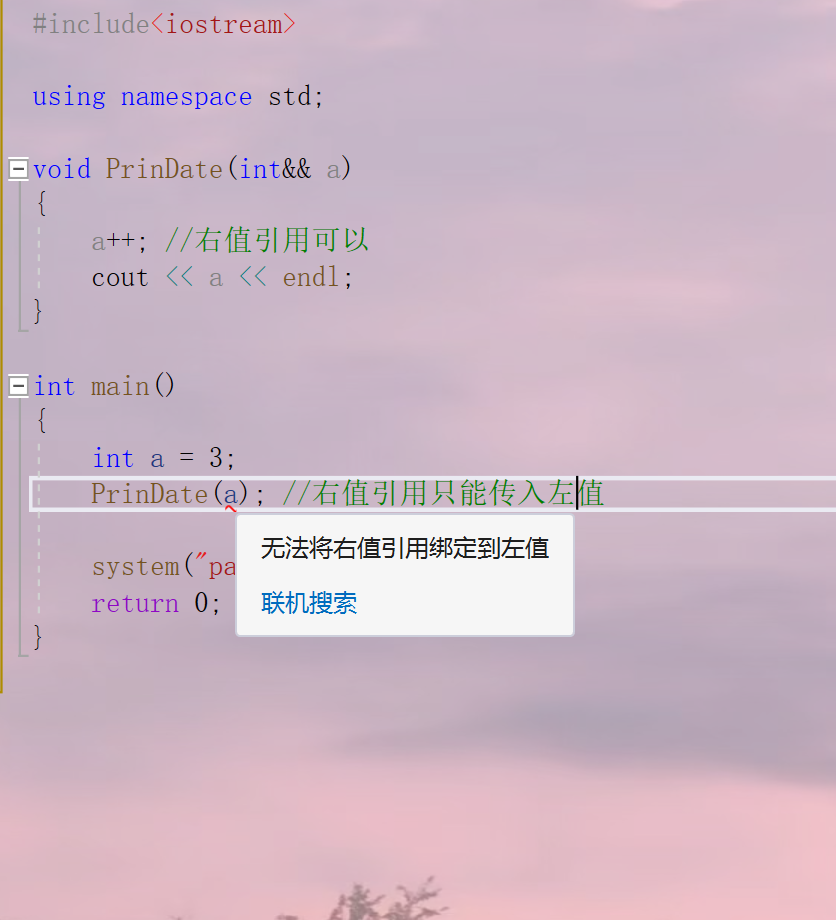
这里就可以通过move()函数,将右值转化为左值
#include<iostream>
using namespace std;
void PrinDate(int&& a)
{
a++; //右值引用可以
cout << a << endl;
}
int main()
{
int a = 3;
PrinDate(move(a)); //右值引用只能传入左值,通过move()函数,将右值转化为左值
system("pause");
return 0;
}
#include<iostream>
using namespace std;
int main()
{
int date = 100;
int&& b = move(date);
system("pause");
return 0;
}
引用小结
引用其实就是起别名的用法,
左值引用,对左边的起别名,传入右值
右值引用,对右边的起别名,传入左值