阿华代码,不是逆风,就是我疯
你们的点赞收藏是我前进最大的动力!!
希望本文内容能够帮助到你!!
目录
一:什么是Spring Web MVC
1:Servlet
2:总结
二:MVC
1:定义
2:解释
3:Spring MVC和MVC的关系
三:Spring MVC
1:SpringBoot 和 SpringMVC之间的关系
四:实践
1:建立连接
2:@RequestMapping注解介绍
(1)既可以修饰类也可以修饰方法
(2)既支持get也支持post请求
3:RequestController注解
4:传递参数
(1)参数使用包装类型
(2)传参顺序不影响结果
5:传递对象
6:Requestparam
(1)后端参数映射
(2)更改为非必要传参
7:传递数组
8:传递集合
9:传递JSON数据
(1)传递失败
(2)RequestBody
10:JSON字符串和Java对象的转换
(1)第三方工具
(2)Person类
(3)ObjectMapper类
一:什么是Spring Web MVC
1:Servlet
2:总结
二:MVC
1:定义
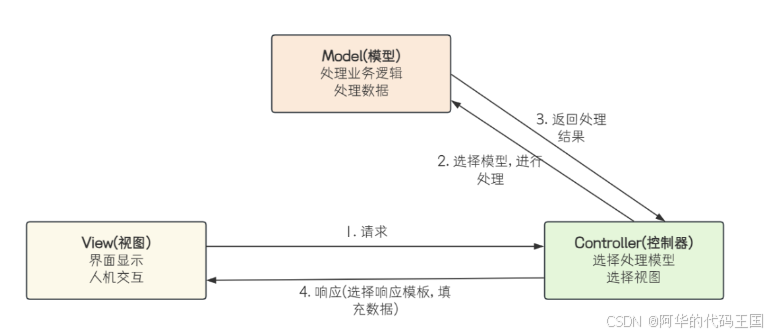
2:解释
3:Spring MVC和MVC的关系
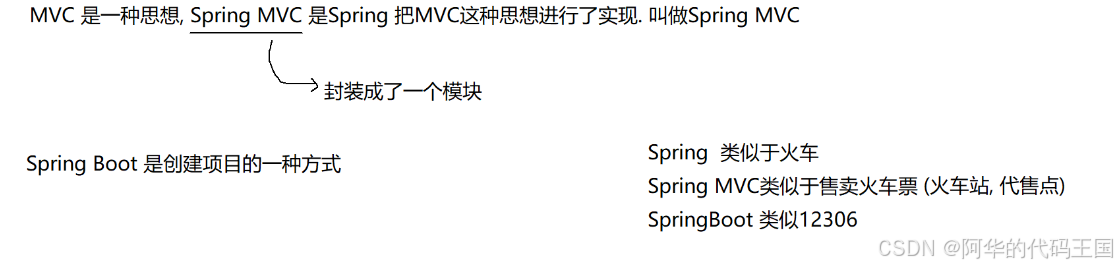
三:Spring MVC
1:SpringBoot 和 SpringMVC之间的关系
四:实践
1:建立连接
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
@RestController
public class UserController {
// 路由器规则注册
@RequestMapping("/sayHi")
public String sayHi(){
return "hello,Spring MVC";
}
}
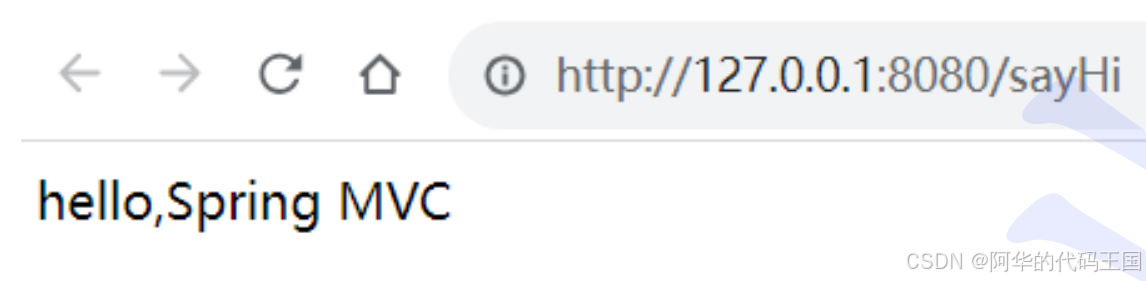
2:@RequestMapping注解介绍
(1)既可以修饰类也可以修饰方法
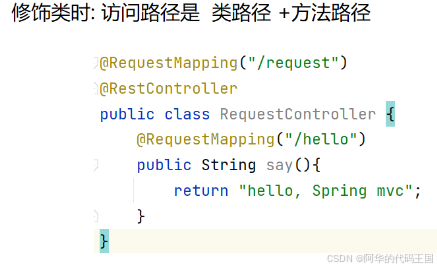
(2)既支持get也支持post请求

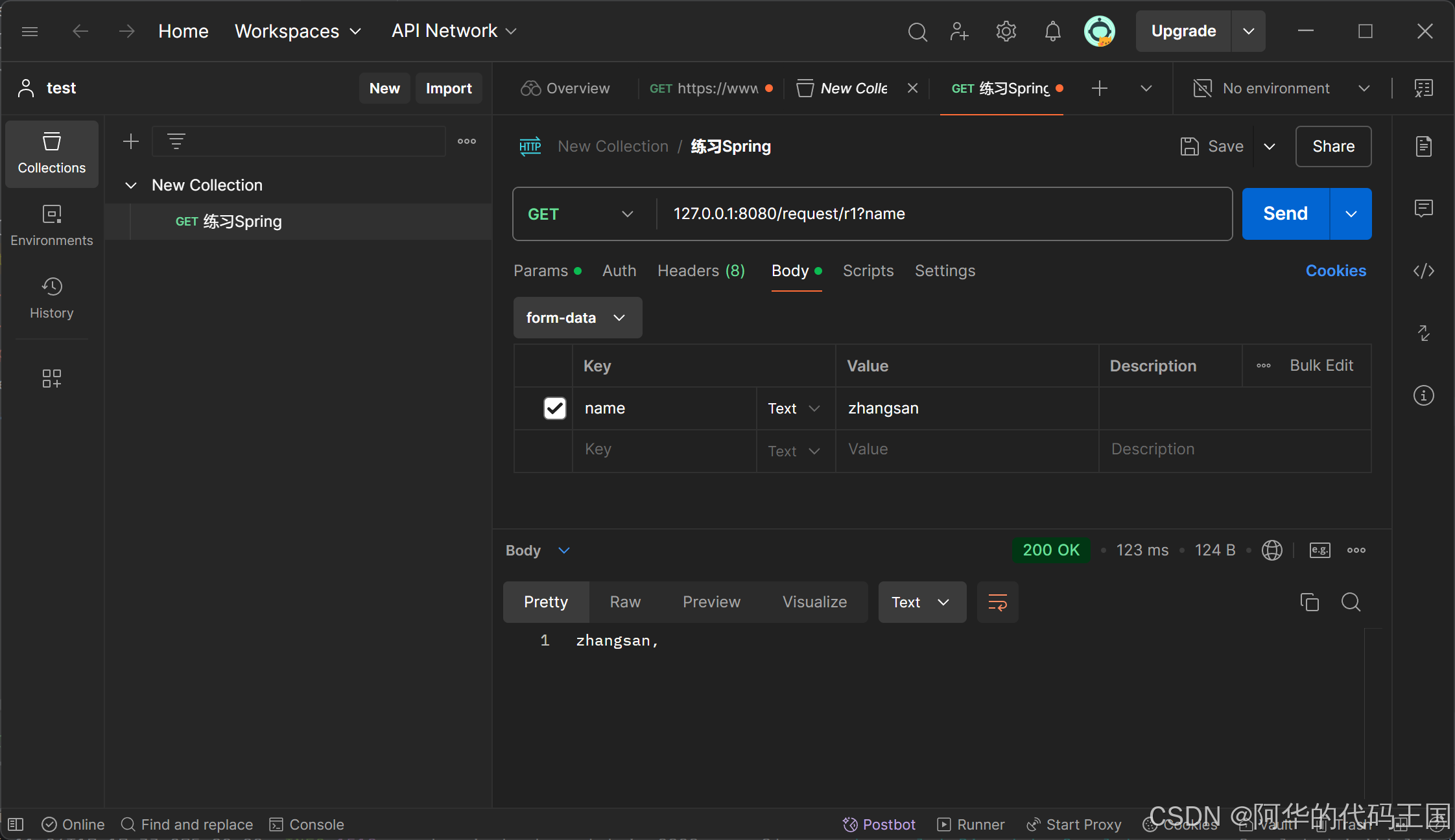
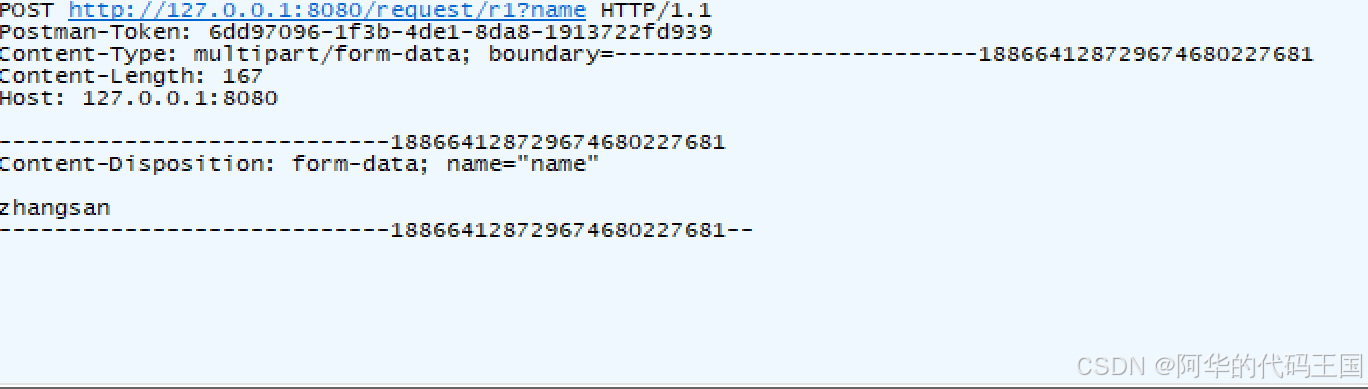
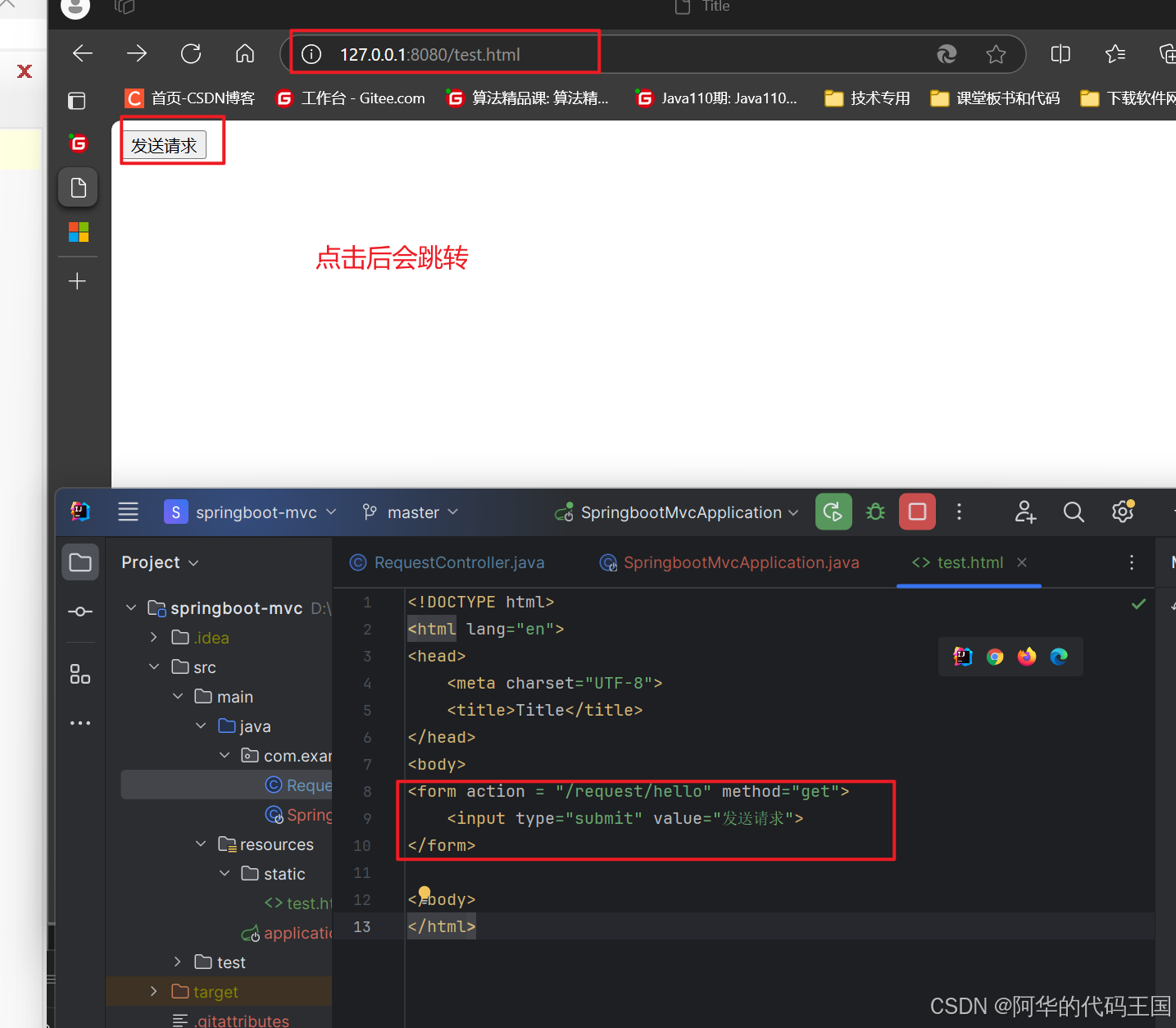
@RequestMapping("/request")
@RestController
public class RequestController {
@RequestMapping("/hello")
public String say(){
return "hello byte";
}
@RequestMapping("/r0")
public String say1(){
return "hello byte";
}
@RequestMapping("/r1")
public String r1(String name){
return name;
}
@RequestMapping("/r2")
public int r2(int age){
return age;
}
@RequestMapping("/r3")
public String r3(Integer age){
return "接收到的参数为:"+age;
}
}
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<form action = "/request/hello" method="get">
<input type="submit" value="发送请求">
</form>
</body>
</html>
3:RequestController注解
⼀个项⽬中, 会有很多类, 每个类可能有很多的⽅法
4:传递参数
(1)参数使用包装类型
(2)传参顺序不影响结果
5:传递对象
package com.example.springbootmvc;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
/**
* Created with IntelliJ IDEA.
* Description:
* User: Hua YY
* Date: 2024-11-04
* Time: 21:25
*/
@RequestMapping("/request")
@RestController
public class RequestController {
@RequestMapping("/hello")
public String say(){
return "hello byte";
}
@RequestMapping("/r0")
public String say1(){
return "hello byte";
}
@RequestMapping("/r1")
public String r1(String name){
return name;
}
@RequestMapping("/r2")
public int r2(int age){
return age;
}
@RequestMapping("/r3")
public String r3(Integer age){
return "接收到的参数为:"+age;
}
@RequestMapping("/m1")
public Object method1(Person p){
return p.toString();
}
}
@RequestMapping("/m1")
public Object method1(Person p){
return p.toString();
}
可以看到, 后端程序正确拿到了Person对象⾥各个属性的值
Spring 会根据参数名称⾃动绑定到对象的各个属性上, 如果某个属性未传递, 则赋值为null(基本类型则赋值为默认初识值, ⽐如int类型的属性, 会被赋值为0)
6:Requestparam
(1)后端参数映射
@RequestMapping("r4")
public Object r4(@RequestParam("time") String createtime){
return "接受到参数createtime:" + createtime;
}
(2)更改为非必要传参
源码
7:传递数组
@RequestMapping("/r5")
public String r5(String[] arrayParam){
return Arrays.toString(arrayParam);
}
8:传递集合
@RequestMapping("/r6")
public String r6(@RequestParam List<String> listParam){
return "size:"+listParam.size()+" "+"listParam:"+listParam;
}
9:传递JSON数据
JSON的语法:1. 数据在 键值对 (Key/Value) 中2. 数据由逗号 , 分隔3. 对象⽤ {} 表⽰4. 数组⽤ [] 表⽰5. 值可以为对象, 也可以为数组, 数组中可以包含多个对象
(1)传递失败
@RequestMapping("/r7")
public Object r7(Person p){
return p.toString();
}
可以看到我们用postman发送json请求,服务器并没有收到我们想要的值为什么呢?
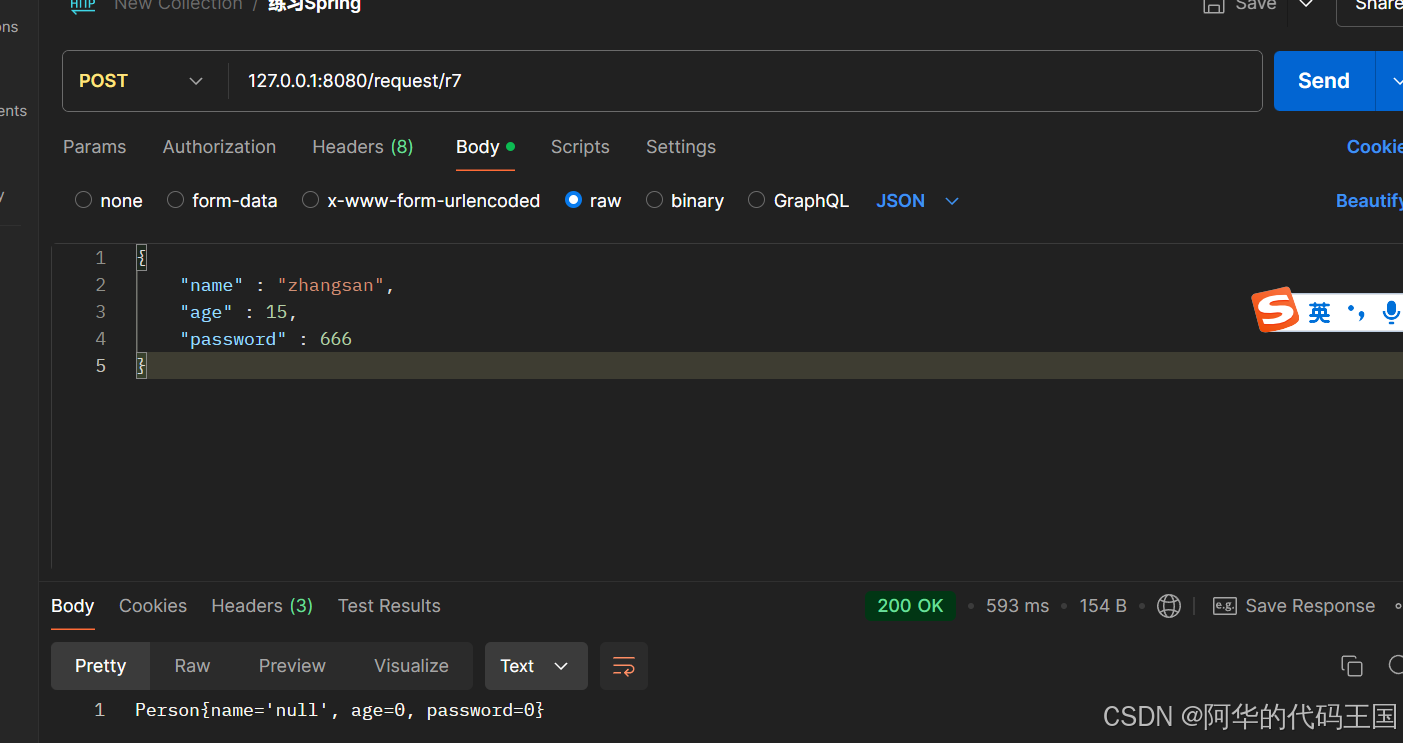
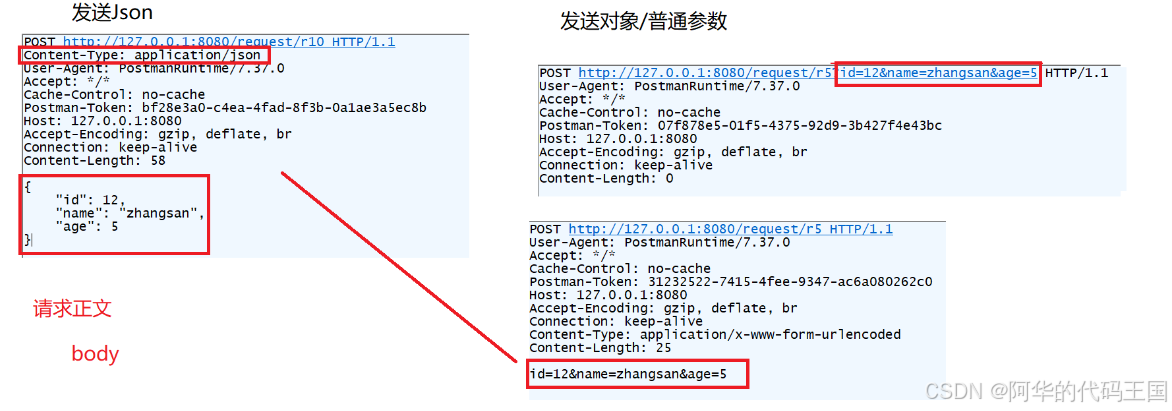
(2)RequestBody
加上RequesstBody注解后,才能读取HTTP中body中的json数据
@RequestMapping("/r8")
public Object r8(@RequestBody Person p){
return p.toString();
}
10:JSON字符串和Java对象的转换
(1)第三方工具
(2)Person类
在json字符串转换为Java对象的时候,要先进行类加载,我们尽量把无参的构造方法也写入类中,避免后续,传参时,找不到对应的构造方法
package com.example.springbootmvc;
import com.fasterxml.jackson.databind.ObjectMapper;
/**
* Created with IntelliJ IDEA.
* Description:
* User: Hua YY
* Date: 2024-11-06
* Time: 17:59
*/
public class Person {
private String name;
private int age;
private int password;
public Person(){
}
public Person(String name, int age, int password) {
this.name = name;
this.age = age;
this.password = password;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
public int getPassword() {
return password;
}
public void setName(String name) {
this.name = name;
}
public void setAge(int age) {
this.age = age;
}
public void setPassword(int password) {
this.password = password;
}
@Override
public String toString() {
return "Person{" +
"name='" + name + '\'' +
", age=" + age +
", password=" + password +
'}';
}
}
(3)ObjectMapper类
.readValue 字符串转对象
.writeValueAsString() 对象转字符串
package com.example.springbootmvc;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import org.springframework.validation.ObjectError;
/**
* Created with IntelliJ IDEA.
* Description:
* User: Hua YY
* Date: 2024-11-07
* Time: 12:23
*/
public class JSONTest {
public static void main(String[] args) throws JsonProcessingException {
//调用ObjectMapper中的两个方法才能实现Json字符串和java对象的转换
ObjectMapper objectMapper = new ObjectMapper();
//格式化JSON字符串
String jsonStr = "{\"name\":\"zhangsan\",\"age\":15,\"password\":666}";
//JSON字符串转化为java对象,先加载Person类在,解析字符串
Person p = objectMapper.readValue(jsonStr,Person.class);
System.out.println(p.toString());
//java对象转化为Json字符串
String s = objectMapper.writeValueAsString(p);
System.out.println(s);
}
}