//@author:shark_ddd
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
//使用函数来减少长度
namespace List_T
{
class Student
{
public string Name { get; set; }
public int Age { get; set; }
}
internal class Program
{
static void PrintStudents(List<Student> students)
{
foreach (Student student in students)
{
Console.Write("Name:" + student.Name);
Console.WriteLine(", Age:" + student.Age);
}
}
static void PrintNumbers(List<int> numbers)
{
foreach (int number in numbers)
{
Console.Write(" " + number);
}
Console.WriteLine();
}
static void PrintStrings(List<string> strings)
{
foreach (string str in strings)
{
Console.Write(" " + str);
}
Console.WriteLine();
}
static void Main(string[] args)
{
List<Student> studentList = new List<Student>
{
new Student { Name = "Alice", Age = 20 },
new Student { Name = "Ben", Age = 18 },
new Student { Name = "Clark", Age = 18 }
};
Console.WriteLine("Students:");
PrintStudents(studentList);
Console.WriteLine("=================================================================================");
// 创建数字列表
List<int> numberList = new List<int> { 1, 2, 3, 4, 5 };
// 在数字列表末尾加入 6
numberList.Add(6);
// 在数字列表末尾加入 7、8、9
numberList.AddRange(new List<int> { 7, 8, 9 });
Console.WriteLine("now_List:");
PrintNumbers(numberList);
// 获取数字列表中的所有偶数
List<int> evenNumbers = numberList.FindAll(n => n % 2 == 0);
Console.WriteLine("获取的偶数有:");
PrintNumbers(evenNumbers);
// 删除数字列表中的 9
numberList.Remove(9);
Console.WriteLine("now_List:");
PrintNumbers(numberList);
// 删除数字列表中索引为 0 的元素
numberList.RemoveAt(0);
Console.WriteLine("now_List:");
PrintNumbers(numberList);
// 打印第一个
Console.WriteLine("First_number:" + numberList.First());
Console.WriteLine("=================================================================================");
// 水果列表
List<string> fruitList = new List<string> { "apple", "banana", "cherry" };
// 在索引为 1 的位置插入 orange
fruitList.Insert(1, "orange");
Console.WriteLine("now_Fruit:");
PrintStrings(fruitList);
// 复制水果列表从索引 0 开始的两个元素到 myFruit 数组中,并从数组的索引 0 开始存入
string[] myFruit = new string[2];
fruitList.CopyTo(0, myFruit, 0, 2);
Console.WriteLine("myFruit:");
Console.WriteLine(string.Join(" ", myFruit));
// 复制水果列表从索引 1 开始的三个元素到 subList 中
List<string> subList = fruitList.GetRange(1, 3);
Console.WriteLine("subList:");
PrintStrings(subList);
// 翻转水果列表元素排序顺序
fruitList.Reverse();
Console.WriteLine("now_Fruit:");
PrintStrings(fruitList);
// 对水果列表元素进行排序
fruitList.Sort();
Console.WriteLine("now_Fruit:");
PrintStrings(fruitList);
// 清空水果列表
fruitList.Clear();
Console.WriteLine("now_Fruit:");
PrintStrings(fruitList);
Console.ReadLine();
}
}
}
//转换成字符串string.Join
//using System;
//using System.Collections.Generic;
//using System.Linq;
//using System.Text;
//using System.Threading.Tasks;
//namespace List_T
//{
// class Student
// {
// public string Name { get; set; }
// public int Age { get; set; }
// }
// internal class Program
// {
// static void Main(string[] args)
// {
// List<Student> studentList = new List<Student>
// {
// new Student { Name = "Alice", Age = 20 },
// new Student { Name = "Ben", Age = 18 },
// new Student { Name = "Clark", Age = 18 }
// };
// Console.WriteLine("Students: " + string.Join(", ", studentList.Select(s => $"Name:{s.Name}, Age:{s.Age}")));
// Console.WriteLine("=================================================================================");
// // 创建数字列表
// List<int> numberList = new List<int> { 1, 2, 3, 4, 5 };
// // 在数字列表末尾加入 6
// numberList.Add(6);
// // 在数字列表末尾加入 7、8、9
// numberList.AddRange(new List<int> { 7, 8, 9 });
// Console.WriteLine("now_List: " + string.Join(" ", numberList));
// // 获取数字列表中的所有偶数
// List<int> evenNumbers = numberList.FindAll(n => n % 2 == 0);
// Console.WriteLine("获取的偶数有: " + string.Join(" ", evenNumbers));
// // 删除数字列表中的 9
// numberList.Remove(9);
// Console.WriteLine("now_List: " + string.Join(" ", numberList));
// // 删除数字列表中索引为 0 的元素
// numberList.RemoveAt(0);
// Console.WriteLine("now_List: " + string.Join(" ", numberList));
// // 打印第一个
// Console.WriteLine("First_number:" + numberList.First());
// Console.WriteLine("=================================================================================");
// // 水果列表
// List<string> fruitList = new List<string> { "apple", "banana", "cherry" };
// // 在索引为 1 的位置插入 orange
// fruitList.Insert(1, "orange");
// Console.WriteLine("now_Fruit: " + string.Join(" ", fruitList));
// // 复制水果列表从索引 0 开始的两个元素到 myFruit 数组中,并从数组的索引 0 开始存入
// string[] myFruit = new string[2];
// fruitList.CopyTo(0, myFruit, 0, 2);
// Console.WriteLine("myFruit: " + string.Join(" ", myFruit));
// // 复制水果列表从索引 1 开始的三个元素到 subList 中
// List<string> subList = fruitList.GetRange(1, 3);
// Console.WriteLine("subList: " + string.Join(" ", subList));
// // 翻转水果列表元素排序顺序
// fruitList.Reverse();
// Console.WriteLine("now_Fruit: " + string.Join(" ", fruitList));
// // 对水果列表元素进行排序
// fruitList.Sort();
// Console.WriteLine("now_Fruit: " + string.Join(" ", fruitList));
// // 清空水果列表
// fruitList.Clear();
// Console.WriteLine("now_Fruit: " + string.Join(" ", fruitList));
// Console.ReadLine();
// }
// }
//}
/*冗长的foreach
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace List_T
{
class Student
{
public string Name { get; set; }
public int Age { get; set; }
}
internal class Program
{
static void Main(string[] args)
{
List<Student> studentList = new List<Student>
{
new Student { Name = "Alice", Age = 20 },
new Student { Name = "Ben", Age = 18 },
new Student { Name = "Clark", Age = 18 }
};
foreach (Student student in studentList)
{
Console.Write("Name:" + student.Name);
Console.WriteLine(", Age:" + student.Age);
}
Console.WriteLine("=================================================================================");
// 创建数字列表
List<int> numberList = new List<int> { 1, 2, 3, 4, 5 };
// 在数字列表末尾加入 6
numberList.Add(6);
// 在数字列表末尾加入 7、8、9
numberList.AddRange(new List<int> { 7, 8, 9 });
Console.Write("now_List:");
foreach (int number in numberList)
{
Console.Write(" " + number);
}
Console.WriteLine();
// 获取数字列表中的所有偶数
List<int> evenNumbers = numberList.FindAll(n => n % 2 == 0);
Console.Write("获取的偶数有:");
foreach (int number in evenNumbers)
{
Console.Write(" " + number);
}
Console.WriteLine();
// 删除数字列表中的 9
numberList.Remove(9);
Console.Write("now_List:");
foreach (int number in numberList)
{
Console.Write(" " + number);
}
Console.WriteLine();
// 删除数字列表中索引为 0 的元素
numberList.RemoveAt(0);
Console.Write("now_List:");
foreach (int number in numberList)
{
Console.Write(" " + number);
}
Console.WriteLine();
//打印第一个
Console.WriteLine("First_number:" + numberList.First());
Console.WriteLine("=================================================================================");
// 水果列表
List<string> fruitList = new List<string> { "apple", "banana", "cherry" };
// 在索引为 1 的位置插入 orange
fruitList.Insert(1, "orange");
Console.Write("now_Fruit:");
foreach (string number in fruitList)
{
Console.Write(" " + number);
}
Console.WriteLine();
// 复制水果列表从索引 0 开始的两个元素到 myFruit 数组中,并从数组的索引 0 开始存入
string[] myFruit = new string[2];
fruitList.CopyTo(0, myFruit, 0, 2);
Console.Write("myFruit:");
foreach (string number in myFruit)
{
Console.Write(" " + number);
}
Console.WriteLine();
// 复制水果列表从索引 1 开始的三个元素到 subList 中
List<string> subList = fruitList.GetRange(1, 3);
Console.Write("subList:");
foreach (string number in subList)
{
Console.Write(" " + number);
}
Console.WriteLine();
// 翻转水果列表元素排序顺序
fruitList.Reverse();
Console.Write("now_Fruit:");
foreach (string number in fruitList)
{
Console.Write(" " + number);
}
Console.WriteLine();
// 对水果列表元素进行排序
fruitList.Sort();
Console.Write("now_Fruit:");
foreach (string number in fruitList)
{
Console.Write(" " + number);
}
Console.WriteLine();
// 清空水果列表
fruitList.Clear();
Console.Write("now_Fruit:");
foreach (string number in fruitList)
{
Console.Write(" " + number);
}
Console.WriteLine();
Console.ReadLine();
}
}
}*/
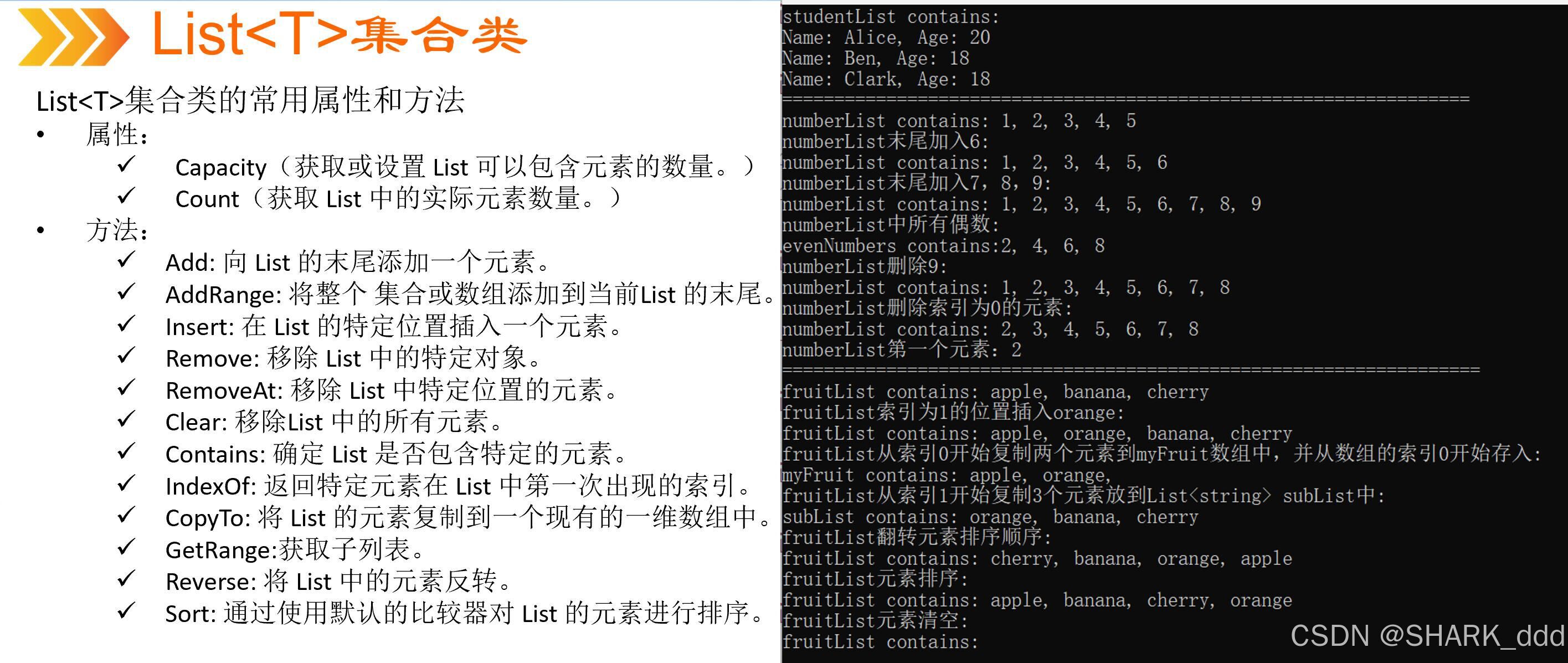