文章目录
- 获取分类关联的品牌
- CategoryBrandController.java
- CategoryBrandServiceImpl.java
- BrandVo.java
- 获取分类下的所有分组&关联属性
- AttrGroupController.java
- AttrGroupServiceImpl.java
- 保存七张表
- sql
- tb_spu_info.sql
- tb_spu_info_desc.sql
- tb_spu_images.sql
- tb_product_attr_value.sql
- tb_sku_info.sql
- tb_sku_images.sql
- tb_sku_sale_attr_value.sql
- vo
- Attr.java
- BaseAttrs.java
- Images.java
- Skus.java
- SpuSaveVo.java
- controller
- SpuInfoController.java
- impl
- SpuInfoServiceImpl.java
- SpuInfoDescServiceImpl.java
- SpuImagesServiceImpl.java
- ProductAttrValueServiceImpl.java
- SkuInfoServiceImpl.java
https://www.bejson.com/json2javapojo/new/#google_vignette
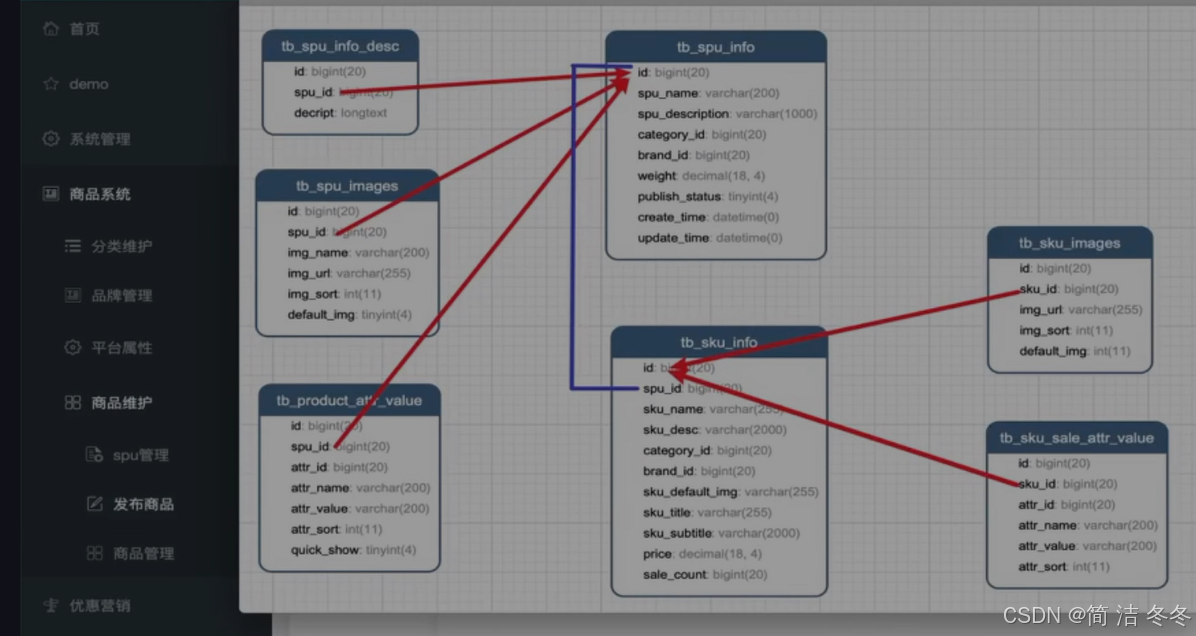
获取分类关联的品牌
CategoryBrandController.java
/**
* 获取分类关联的品牌
* @return
*/
@GetMapping("/brands/list")
public R relationBrandList(@RequestParam("categoryId") Integer categoryId){
List<BrandEntity> brandEntities = categoryBrandService.getBrandByCategoryId(categoryId);
List<BrandVo> collect = brandEntities.stream().map(brandEntity -> {
BrandVo brandVo = new BrandVo();
brandVo.setBrandId(brandEntity.getId());
brandVo.setBrandName(brandEntity.getName());
return brandVo;
}).collect(Collectors.toList());
return R.ok().put("data", collect);
}
CategoryBrandServiceImpl.java
/**
* 获取分类关联的品牌
* @param categoryId
* @return
*/
@Override
public List<BrandEntity> getBrandByCategoryId(Integer categoryId) {
List<CategoryBrandEntity> categoryBrandEntities = categoryBrandDao.selectList(
new QueryWrapper<CategoryBrandEntity>().eq("category_id", categoryId)
);
List<BrandEntity> collect = categoryBrandEntities.stream().map(item->{
Integer brandId = item.getBrandId();
BrandEntity brandEntity = brandService.getById(brandId);
return brandEntity;
}).collect(Collectors.toList());
return collect;
}
BrandVo.java
package com.xd.cubemall.product.vo;
import lombok.Data;
@Data
public class BrandVo {
/**
* 品牌ID
*/
private Integer brandId;
/**
* 品牌名称
*/
private String brandName;
}
获取分类下的所有分组&关联属性
AttrGroupController.java
/**
* 获取分类下所有分组&关联属性
* @return
*/
@GetMapping("/{categoryId}/withattr")
public R getAttrGroupWithAttrs(@PathVariable("categoryId") Long categoryId){
//1.查询当前分类下的所有属性分组
//2.查询当前属性分组下所有的属性
List<AttrGroupWithAttrsVo> vos = attrGroupService.getAttrGroupWithAttrsByCategoryId(categoryId);
return R.ok().put("data", vos);
}
AttrGroupServiceImpl.java
/**
* 获取分类下的所有分组&关联属性
* @param categoryId
* @return
*/
@Override
public List<AttrGroupWithAttrsVo> getAttrGroupWithAttrsByCategoryId(Long categoryId) {
//1.查询该分类下所有的分组信息
List<AttrGroupEntity> attrGroupEntities = this.list(new QueryWrapper<AttrGroupEntity>().eq("category_id",categoryId));
//2.查询出所有属性
List<AttrGroupWithAttrsVo> collect = attrGroupEntities.stream().map(attrGroupEntity -> {
AttrGroupWithAttrsVo attrsVo = new AttrGroupWithAttrsVo();
//属性对拷
BeanUtils.copyProperties(attrGroupEntity, attrsVo);
//设置关联的属性
List<AttrEntity> relationAttr = attrService.getRelationAttr(attrsVo.getId());
attrsVo.setAttrs(relationAttr);
return attrsVo;
}).collect(Collectors.toList());
return collect;
}
保存七张表
sql
tb_spu_info.sql
SET NAMES utf8mb4;
SET FOREIGN_KEY_CHECKS = 0;
-- ----------------------------
-- Table structure for tb_spu_info
-- ----------------------------
DROP TABLE IF EXISTS `tb_spu_info`;
CREATE TABLE `tb_spu_info` (
`id` bigint(0) NOT NULL AUTO_INCREMENT COMMENT '自增ID',
`spu_name` varchar(200) CHARACTER SET utf8mb4 COLLATE utf8mb4_bin NULL DEFAULT NULL COMMENT 'spu名称',
`spu_description` varchar(1000) CHARACTER SET utf8mb4 COLLATE utf8mb4_bin NULL DEFAULT NULL COMMENT 'spu描述',
`category_id` bigint(0) NULL DEFAULT NULL COMMENT '分类ID',
`brand_id` bigint(0) NULL DEFAULT NULL COMMENT '品牌ID',
`weight` decimal(18, 4) NULL DEFAULT NULL COMMENT '权重',
`publish_status` tinyint(0) NULL DEFAULT NULL COMMENT '发布状态',
`create_time` datetime(0) NULL DEFAULT NULL,
`update_time` datetime(0) NULL DEFAULT NULL,
PRIMARY KEY (`id`) USING BTREE
) ENGINE = InnoDB AUTO_INCREMENT = 7 CHARACTER SET = utf8mb4 COLLATE = utf8mb4_bin COMMENT = 'spu信息' ROW_FORMAT = Dynamic;
SET FOREIGN_KEY_CHECKS = 1;
tb_spu_info_desc.sql
SET NAMES utf8mb4;
SET FOREIGN_KEY_CHECKS = 0;
-- ----------------------------
-- Table structure for tb_spu_info_desc
-- ----------------------------
DROP TABLE IF EXISTS `tb_spu_info_desc`;
CREATE TABLE `tb_spu_info_desc` (
`id` bigint(0) NOT NULL AUTO_INCREMENT COMMENT '自增ID',
`spu_id` bigint(0) NOT NULL COMMENT 'spu_id',
`decript` longtext CHARACTER SET utf8mb4 COLLATE utf8mb4_bin NULL COMMENT '描述',
PRIMARY KEY (`id`) USING BTREE
) ENGINE = InnoDB AUTO_INCREMENT = 6 CHARACTER SET = utf8mb4 COLLATE = utf8mb4_bin COMMENT = 'spu描述' ROW_FORMAT = Dynamic;
SET FOREIGN_KEY_CHECKS = 1;
tb_spu_images.sql
SET NAMES utf8mb4;
SET FOREIGN_KEY_CHECKS = 0;
-- ----------------------------
-- Table structure for tb_spu_images
-- ----------------------------
DROP TABLE IF EXISTS `tb_spu_images`;
CREATE TABLE `tb_spu_images` (
`id` bigint(0) NOT NULL AUTO_INCREMENT COMMENT 'id',
`spu_id` bigint(0) NULL DEFAULT NULL COMMENT 'spu_id',
`img_name` varchar(200) CHARACTER SET utf8mb4 COLLATE utf8mb4_bin NULL DEFAULT NULL COMMENT '图片名称',
`img_url` varchar(255) CHARACTER SET utf8mb4 COLLATE utf8mb4_bin NULL DEFAULT NULL COMMENT '图片url',
`img_sort` int(0) NULL DEFAULT NULL COMMENT '图片顺序',
`default_img` tinyint(0) NULL DEFAULT NULL COMMENT '默认图片',
PRIMARY KEY (`id`) USING BTREE
) ENGINE = InnoDB AUTO_INCREMENT = 7 CHARACTER SET = utf8mb4 COLLATE = utf8mb4_bin COMMENT = 'spu图片' ROW_FORMAT = Dynamic;
SET FOREIGN_KEY_CHECKS = 1;
tb_product_attr_value.sql
SET NAMES utf8mb4;
SET FOREIGN_KEY_CHECKS = 0;
-- ----------------------------
-- Table structure for tb_product_attr_value
-- ----------------------------
DROP TABLE IF EXISTS `tb_product_attr_value`;
CREATE TABLE `tb_product_attr_value` (
`id` bigint(0) NOT NULL AUTO_INCREMENT COMMENT 'id',
`spu_id` bigint(0) NULL DEFAULT NULL COMMENT 'spuid',
`attr_id` bigint(0) NULL DEFAULT NULL COMMENT '属性id',
`attr_name` varchar(200) CHARACTER SET utf8mb4 COLLATE utf8mb4_bin NULL DEFAULT NULL COMMENT '属性名称',
`attr_value` varchar(200) CHARACTER SET utf8mb4 COLLATE utf8mb4_bin NULL DEFAULT NULL COMMENT '属性值',
`attr_sort` int(0) NULL DEFAULT NULL COMMENT '属性排序',
`quick_show` tinyint(0) NULL DEFAULT NULL COMMENT '显示',
PRIMARY KEY (`id`) USING BTREE
) ENGINE = InnoDB AUTO_INCREMENT = 20 CHARACTER SET = utf8mb4 COLLATE = utf8mb4_bin COMMENT = 'spu' ROW_FORMAT = Dynamic;
SET FOREIGN_KEY_CHECKS = 1;
tb_sku_info.sql
SET NAMES utf8mb4;
SET FOREIGN_KEY_CHECKS = 0;
-- ----------------------------
-- Table structure for tb_sku_info
-- ----------------------------
DROP TABLE IF EXISTS `tb_sku_info`;
CREATE TABLE `tb_sku_info` (
`id` bigint(0) NOT NULL AUTO_INCREMENT COMMENT '自增ID',
`spu_id` bigint(0) NULL DEFAULT NULL COMMENT 'spuId',
`sku_name` varchar(255) CHARACTER SET utf8mb4 COLLATE utf8mb4_bin NULL DEFAULT NULL COMMENT 'sku名称',
`sku_desc` varchar(2000) CHARACTER SET utf8mb4 COLLATE utf8mb4_bin NULL DEFAULT NULL COMMENT 'sku描述',
`category_id` bigint(0) NULL DEFAULT NULL COMMENT '分类id',
`brand_id` bigint(0) NULL DEFAULT NULL COMMENT '品牌id',
`sku_default_img` varchar(255) CHARACTER SET utf8mb4 COLLATE utf8mb4_bin NULL DEFAULT NULL COMMENT '默认sku图片',
`sku_title` varchar(255) CHARACTER SET utf8mb4 COLLATE utf8mb4_bin NULL DEFAULT NULL COMMENT 'sku名称',
`sku_subtitle` varchar(2000) CHARACTER SET utf8mb4 COLLATE utf8mb4_bin NULL DEFAULT NULL COMMENT 'sku子名称',
`price` decimal(18, 4) NULL DEFAULT NULL COMMENT '价格',
`sale_count` bigint(0) NULL DEFAULT NULL COMMENT '销售数量',
PRIMARY KEY (`id`) USING BTREE
) ENGINE = InnoDB AUTO_INCREMENT = 18 CHARACTER SET = utf8mb4 COLLATE = utf8mb4_bin COMMENT = 'sku??Ϣ' ROW_FORMAT = Dynamic;
SET FOREIGN_KEY_CHECKS = 1;
tb_sku_images.sql
SET NAMES utf8mb4;
SET FOREIGN_KEY_CHECKS = 0;
-- ----------------------------
-- Table structure for tb_sku_images
-- ----------------------------
DROP TABLE IF EXISTS `tb_sku_images`;
CREATE TABLE `tb_sku_images` (
`id` bigint(0) NOT NULL AUTO_INCREMENT COMMENT 'id',
`sku_id` bigint(0) NULL DEFAULT NULL COMMENT 'sku_id',
`img_url` varchar(255) CHARACTER SET utf8mb4 COLLATE utf8mb4_bin NULL DEFAULT NULL COMMENT '图片地址',
`img_sort` int(0) NULL DEFAULT NULL COMMENT '图片排序',
`default_img` int(0) NULL DEFAULT NULL COMMENT '默认图片',
PRIMARY KEY (`id`) USING BTREE
) ENGINE = InnoDB AUTO_INCREMENT = 15 CHARACTER SET = utf8mb4 COLLATE = utf8mb4_bin COMMENT = 'sku图片表' ROW_FORMAT = Dynamic;
SET FOREIGN_KEY_CHECKS = 1;
tb_sku_sale_attr_value.sql
SET NAMES utf8mb4;
SET FOREIGN_KEY_CHECKS = 0;
-- ----------------------------
-- Table structure for tb_sku_sale_attr_value
-- ----------------------------
DROP TABLE IF EXISTS `tb_sku_sale_attr_value`;
CREATE TABLE `tb_sku_sale_attr_value` (
`id` bigint(0) NOT NULL AUTO_INCREMENT COMMENT 'id',
`sku_id` bigint(0) NULL DEFAULT NULL COMMENT 'sku_id',
`attr_id` bigint(0) NULL DEFAULT NULL COMMENT 'attr_id',
`attr_name` varchar(200) CHARACTER SET utf8mb4 COLLATE utf8mb4_bin NULL DEFAULT NULL COMMENT '属性名称',
`attr_value` varchar(200) CHARACTER SET utf8mb4 COLLATE utf8mb4_bin NULL DEFAULT NULL COMMENT '属性值',
`attr_sort` int(0) NULL DEFAULT NULL COMMENT '属性排序',
PRIMARY KEY (`id`) USING BTREE
) ENGINE = InnoDB AUTO_INCREMENT = 36 CHARACTER SET = utf8mb4 COLLATE = utf8mb4_bin COMMENT = 'sku销售属性值' ROW_FORMAT = Dynamic;
SET FOREIGN_KEY_CHECKS = 1;
vo
Attr.java
/**
* Copyright 2021 json.cn
*/
package com.xd.cubemall.product.vo;
import lombok.Data;
@Data
public class Attr {
private int attrId;
private String attrName;
private String attrValue;
}
BaseAttrs.java
/**
* Copyright 2021 json.cn
*/
package com.xd.cubemall.product.vo;
import lombok.Data;
@Data
public class BaseAttrs {
private int attrId;
private String attrValues;
private int showDesc;
}
Images.java
/**
* Copyright 2021 json.cn
*/
package com.xd.cubemall.product.vo;
import lombok.Data;
@Data
public class Images {
private String imgUrl;
private int defaultImg;
}
Skus.java
/**
* Copyright 2021 json.cn
*/
package com.xd.cubemall.product.vo;
import lombok.Data;
import java.math.BigDecimal;
import java.util.List;
@Data
public class Skus {
private List<Attr> attr;
private String skuName;
private BigDecimal price;
private String skuTitle;
private String skuSubtitle;
private List<Images> images;
private List<String> descar;
private int fullCount;
private int countStatus;
private BigDecimal fullPrice;
private int priceStatus;
}
SpuSaveVo.java
/**
* Copyright 2021 json.cn
*/
package com.xd.cubemall.product.vo;
import lombok.Data;
import java.math.BigDecimal;
import java.util.Date;
import java.util.List;
@Data
public class SpuSaveVo {
private String spuName;
private String spuDescription;
private Long categoryId;
private Long brandId;
private BigDecimal weight;
private int publishStatus;
private String decript;
private List<String> images;
private List<BaseAttrs> baseAttrs;
private List<Skus> skus;
}
SELECT * FROM tb_spu_info;
SELECT * FROM tb_spu_info_desc;
SELECT * FROM tb_spu_images;
SELECT * FROM tb_product_attr_value;
SELECT * FROM tb_sku_info;
SELECT * FROM tb_sku_images;
SELECT * FROM tb_sku_sale_attr_value;
controller
SpuInfoController.java
/**
* 保存
*/
@RequestMapping("/save")
//@RequiresPermissions("product:spuinfo:save")
public R save(@RequestBody SpuSaveVo spuSaveVo){
spuInfoService.saveSpuInfo(spuSaveVo);
return R.ok();
}
impl
SpuInfoServiceImpl.java
import com.xd.cubemall.common.utils.PageUtils;
import com.xd.cubemall.common.utils.Query;
import com.xd.cubemall.product.entity.*;
import com.xd.cubemall.product.service.*;
import com.xd.cubemall.product.vo.*;
import org.apache.commons.lang3.StringUtils;
import org.springframework.beans.BeanUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.Date;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import com.baomidou.mybatisplus.core.metadata.IPage;
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl;
import com.xd.cubemall.product.dao.SpuInfoDao;
import org.springframework.transaction.annotation.Transactional;
import org.springframework.web.bind.MethodArgumentNotValidException;
@Service("spuInfoService")
public class SpuInfoServiceImpl extends ServiceImpl<SpuInfoDao, SpuInfoEntity> implements SpuInfoService {
@Autowired
private SpuInfoDescService spuInfoDescService;
@Autowired
private SpuImagesService spuImagesService;
@Autowired
private AttrService attrService;
@Autowired
private ProductAttrValueService productAttrValueService;
@Autowired
private SkuInfoService skuInfoService;
@Autowired
private SkuImagesService skuImagesService;
@Autowired
private SkuSaleAttrValueService skuSaleAttrValueService;
/**
* 对7张数据库表进行保存操作
* @param spuSaveVo
*/
@Transactional(rollbackFor = MethodArgumentNotValidException.class)//不设置,在springboot低版本不会进行事务的回滚
@Override
public void saveSpuInfo(SpuSaveVo spuSaveVo) {
//1.保存spu基本信息: tb_spu_info
SpuInfoEntity spuInfoEntity = new SpuInfoEntity();
BeanUtils.copyProperties(spuSaveVo, spuInfoEntity);
spuInfoEntity.setCreateTime(new Date());
spuInfoEntity.setUpdateTime(new Date());
this.saveBaseSpuInfo(spuInfoEntity);
//2.保存spu的描述信息: tb_spu_info_desc
SpuInfoDescEntity spuInfoDescEntity = new SpuInfoDescEntity();
spuInfoDescEntity.setSpuId(spuInfoEntity.getId());
spuInfoDescEntity.setDecript(spuSaveVo.getDecript());
spuInfoDescService.saveSpuInfoDesc(spuInfoDescEntity);
//3.保存spu图片集: tb_spu_images
List<String> images = spuSaveVo.getImages();
spuImagesService.saveImage(spuInfoEntity.getId(), images);
//4.保存spu的规格参数: tb_product_attr_value
List<BaseAttrs> baseAttrs = spuSaveVo.getBaseAttrs();
List<ProductAttrValueEntity> collect = baseAttrs.stream().map(attr->{
ProductAttrValueEntity productAttrValueEntity = new ProductAttrValueEntity();
productAttrValueEntity.setSpuId(spuInfoEntity.getId());
productAttrValueEntity.setAttrId((long) attr.getAttrId());
AttrEntity attrEntity = attrService.getById(attr.getAttrId());
productAttrValueEntity.setAttrName(attrEntity.getName());
productAttrValueEntity.setAttrValue(attr.getAttrValues());
productAttrValueEntity.setQuickShow(attr.getShowDesc());
return productAttrValueEntity;
}).collect(Collectors.toList());
productAttrValueService.saveProductAttr(collect);
//5.保存当前spu对应的所有的sku信息
List<Skus> skus = spuSaveVo.getSkus();
if (skus != null && skus.size()>0) {
skus.forEach(item->{
//获取默认图片
String defaultImg = "";
for (Images image : item.getImages()) {
if (image.getDefaultImg() == 1){
defaultImg = image.getImgUrl();
}
}
//5.1 保存sku的基本信息:tb_sku_info
SkuInfoEntity skuInfoEntity = new SkuInfoEntity();
BeanUtils.copyProperties(item, skuInfoEntity);
skuInfoEntity.setBrandId(spuInfoEntity.getBrandId());
skuInfoEntity.setCategoryId(spuInfoEntity.getCategoryId());
skuInfoEntity.setSaleCount(0L);
skuInfoEntity.setSpuId(spuInfoEntity.getId());
skuInfoEntity.setSkuDefaultImg(defaultImg);
skuInfoService.saveSkuInfo(skuInfoEntity);
//5.2 保存sku的图片信息:tb_sku_images
Long skuId = skuInfoEntity.getId();
List<SkuImagesEntity> imagesEntities = item.getImages().stream().map(img->{
SkuImagesEntity skuImagesEntity = new SkuImagesEntity();
skuImagesEntity.setSkuId(skuId);
skuImagesEntity.setImgUrl(img.getImgUrl());
skuImagesEntity.setDefaultImg(img.getDefaultImg());
return skuImagesEntity;
}).filter(entity->{
return StringUtils.isEmpty(entity.getImgUrl());
}).collect(Collectors.toList());
skuImagesService.saveBatch(imagesEntities);
//5.3 保存sku的销售属性: tb_sku_sale_attr_value
List<Attr> attrs = item.getAttr();
if (attrs != null && attrs.size() > 0) {
List<SkuSaleAttrValueEntity> skuSaleAttrValueEntities = attrs.stream().map(attr -> {
SkuSaleAttrValueEntity skuSaleAttrValueEntity = new SkuSaleAttrValueEntity();
BeanUtils.copyProperties(attr, skuSaleAttrValueEntity);
skuSaleAttrValueEntity.setSkuId(skuId);
return skuSaleAttrValueEntity;
}).collect(Collectors.toList());
skuSaleAttrValueService.saveBatch(skuSaleAttrValueEntities);
}
});
}
}
/**
* 1.保存spu基本信息: tb_spu_info
* @param spuInfoEntity
*/
public void saveBaseSpuInfo(SpuInfoEntity spuInfoEntity) {
this.baseMapper.insert(spuInfoEntity);
}
}
SpuInfoDescServiceImpl.java
import com.xd.cubemall.common.utils.PageUtils;
import com.xd.cubemall.common.utils.Query;
import org.springframework.stereotype.Service;
import java.util.Map;
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import com.baomidou.mybatisplus.core.metadata.IPage;
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl;
import com.xd.cubemall.product.dao.SpuInfoDescDao;
import com.xd.cubemall.product.entity.SpuInfoDescEntity;
import com.xd.cubemall.product.service.SpuInfoDescService;
@Service("spuInfoDescService")
public class SpuInfoDescServiceImpl extends ServiceImpl<SpuInfoDescDao, SpuInfoDescEntity> implements SpuInfoDescService {
/**
* 2.保存spu的描述信息: tb_spu_info_desc
* @param spuInfoDescEntity
*/
@Override
public void saveSpuInfoDesc(SpuInfoDescEntity spuInfoDescEntity) {
this.baseMapper.insert(spuInfoDescEntity);
}
}
SpuImagesServiceImpl.java
import com.xd.cubemall.common.utils.PageUtils;
import com.xd.cubemall.common.utils.Query;
import org.springframework.stereotype.Service;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import com.baomidou.mybatisplus.core.metadata.IPage;
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl;
import com.xd.cubemall.product.dao.SpuImagesDao;
import com.xd.cubemall.product.entity.SpuImagesEntity;
import com.xd.cubemall.product.service.SpuImagesService;
@Service("spuImagesService")
public class SpuImagesServiceImpl extends ServiceImpl<SpuImagesDao, SpuImagesEntity> implements SpuImagesService {
/**
* 3.保存spu图片集: tb_spu_images
* @param id
* @param images
*/
@Override
public void saveImage(Long id, List<String> images) {
if (images == null || images.size()<=0) {
return;
}
List<SpuImagesEntity> collect = images.stream().map(img->{
SpuImagesEntity spuImagesEntity = new SpuImagesEntity();
spuImagesEntity.setSpuId(id);
spuImagesEntity.setImgUrl(img);
return spuImagesEntity;
}).collect(Collectors.toList());
this.saveBatch(collect);
}
}
ProductAttrValueServiceImpl.java
import com.xd.cubemall.common.utils.PageUtils;
import com.xd.cubemall.common.utils.Query;
import org.springframework.stereotype.Service;
import java.util.List;
import java.util.Map;
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import com.baomidou.mybatisplus.core.metadata.IPage;
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl;
import com.xd.cubemall.product.dao.ProductAttrValueDao;
import com.xd.cubemall.product.entity.ProductAttrValueEntity;
import com.xd.cubemall.product.service.ProductAttrValueService;
@Service("productAttrValueService")
public class ProductAttrValueServiceImpl extends ServiceImpl<ProductAttrValueDao, ProductAttrValueEntity> implements ProductAttrValueService {
/**
* 4.保存spu的规格参数: tb_product_attr_value
* @param collect
*/
@Override
public void saveProductAttr(List<ProductAttrValueEntity> collect) {
this.saveBatch(collect);
}
}
SkuInfoServiceImpl.java
import com.xd.cubemall.common.utils.PageUtils;
import com.xd.cubemall.common.utils.Query;
import com.xd.cubemall.product.entity.SpuInfoEntity;
import com.xd.cubemall.product.vo.SpuSaveVo;
import org.springframework.beans.BeanUtils;
import org.springframework.stereotype.Service;
import java.util.Date;
import java.util.Map;
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import com.baomidou.mybatisplus.core.metadata.IPage;
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl;
import com.xd.cubemall.product.dao.SkuInfoDao;
import com.xd.cubemall.product.entity.SkuInfoEntity;
import com.xd.cubemall.product.service.SkuInfoService;
@Service("skuInfoService")
public class SkuInfoServiceImpl extends ServiceImpl<SkuInfoDao, SkuInfoEntity> implements SkuInfoService {
/**
* 5.1 保存sku的基本信息:tb_sku_info
* @param skuInfoEntity
*/
@Override
public void saveSkuInfo(SkuInfoEntity skuInfoEntity) {
this.baseMapper.insert(skuInfoEntity);
}
}