1- 思路
自定义Node结点 + 哈希表实现
- ① 自定义 Node 结点:
- 自定义 Node 结点中有 value 和 cnt 字段,其中 value 为具体的数字,cnt 为具体的值
- 实现 ① getCnt 、② addCnt、**③ getVal **方法
- ② 哈希表实现
- 使用HashMap,其中 key 存储对应的数组值,value 存储为 Node,Node的value为值,cnt 为次数
- 如果首次遇见则加入到 ArrayList中,借助 ArrayList 利用 Lambda 表达式实现快速的排序,收集结果
2- 实现
⭐347. 前 K 个高频元素——题解思路
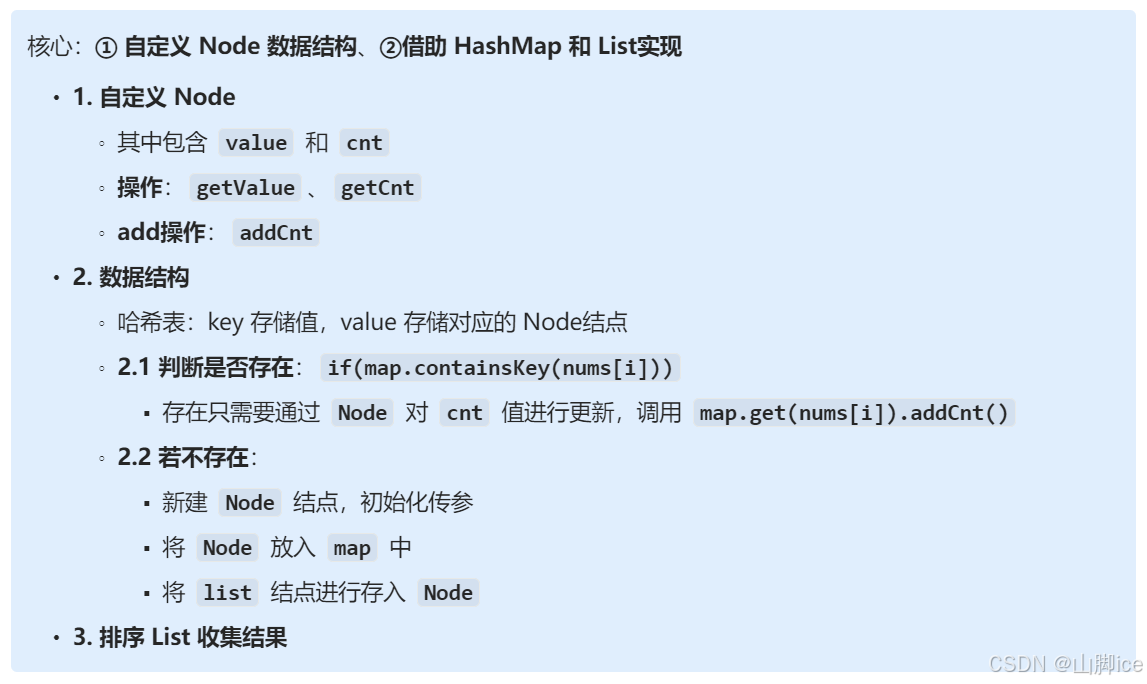
class Node{
int value;
int cnt;
public Node(int v,int c){
value = v;
cnt = c;
}
public int getValue(){
return value;
}
public int getCnt(){
return cnt;
}
public void addCnt(){
cnt++;
}
}
class Solution {
public int[] topKFrequent(int[] nums, int k) {
HashMap<Integer,Node> map = new HashMap<>();
ArrayList<Node> list = new ArrayList<>();
for(int i = 0 ; i < nums.length ; i++){
if(map.containsKey(nums[i])){
map.get(nums[i]).addCnt();
}else{
Node newNode = new Node(nums[i],1);
map.put(nums[i],newNode);
list.add(newNode);
}
}
Collections.sort(list,(o1,o2) -> o2.getCnt() - o1.getCnt());
int[] res = new int[k];
for(int i = 0 ; i < k ; i++){
res[i] = list.get(i).getValue();
}
return res;
}
}
3- ACM实现
package Daily_LC.Month9_Week1.Day147;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.Scanner;
public class getK {
static class Node{
int val;
int cnt;
public Node(int v,int c){
val = v;
cnt = c;
}
public int getVal(){
return val;
}
public int getCnt(){
return cnt;
}
public void addCnt(){
cnt++;
}
}
public static int[] getBeforeK(int[] nums ,int k){
HashMap<Integer,Node> map = new HashMap<>();
ArrayList<Node> list = new ArrayList<>();
for(int i = 0 ; i < nums.length;i++){
if(map.containsKey(nums[i])){
map.get(nums[i]).addCnt();
}else{
Node newNode = new Node(nums[i],1);
map.put(nums[i],newNode);
list.add(newNode);
}
}
Collections.sort(list,(o1,o2) -> o2.getCnt() - o1.getCnt());
int[] res = new int[k];
for(int i = 0 ; i < k;i++){
res[i] = list.get(i).getVal();
}
return res;
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
String input = sc.nextLine();
String[] parts = input.split(" ");
int[] nums = new int[parts.length];
for(int i = 0 ; i < nums.length ; i++){
nums[i] = Integer.parseInt(parts[i]);
}
System.out.println("输入K");
int k = sc.nextInt();
}
}