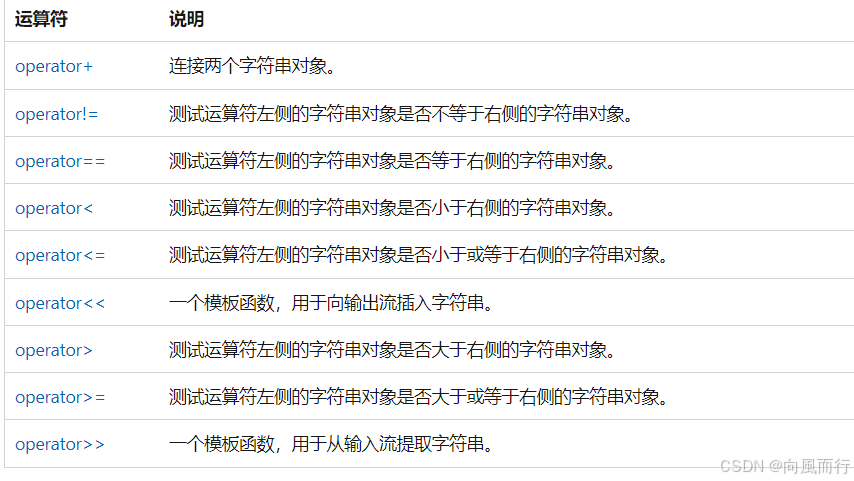
#include<iostream>
#include<cstring> //可以使用string类
#include<string> //#include <string.h>
using namespace std;
class Mystring
{
public:
Mystring():str(nullptr), len(0){
}
Mystring(const char *const str1)
{
if (str1)
{
len = strlen(str1);
str = new char[len + 1];
strcpy(str, str1);
}
else
{
str = nullptr;
len = 0;
}
}
~Mystring()
{
delete[] str;
}
// 拷贝构造函数
Mystring(const Mystring &other)
{
len =other.len;
str = new char[len+1];
strcpy(str, other.str);
}
//拷贝赋值函数
Mystring& operator=(const Mystring& other)
{
if(&other != this)
{
if(str != nullptr)
{
delete []str;
str = nullptr;
}
len = other.len;
str = new char[len+1];
strcpy(str, other.str);
}
return *this;
}
//data()
char* data()
{
return str;
}
//size()
int size()
{
return len;
}
//empty()
bool empty()
{
return 0==len;
}
//at()
char& at(int index)
{
if(index<0 || index>=len)
{
cout << index <<"位置越界" << endl;
return str[0];
}
return str[index]; //*(str+index)
}
//拼接
Mystring operator+(const Mystring& R) const
{
Mystring s;
s.len = R.len+len;
s.str=new char[s.len+1];
strcpy(s.str,str);
strcat(s.str,R.str);
return s;
}
//两侧字符串不相等
bool operator!=(const Mystring& R) const
{
return strcmp(str,R.str)!=0;
}
//两侧字符串相等
bool operator==(const Mystring& R) const
{
return strcmp(str,R.str)==0;
}
//一侧字符串小于另一侧
bool operator<(const Mystring& R) const
{
return strcmp(str,R.str)<0;
}
//一侧字符串小于等于另一侧
bool operator<=(const Mystring& R) const
{
return strcmp(str,R.str)<=0;
}
//一侧字符串大于另一侧
bool operator>(const Mystring& R) const
{
return strcmp(str,R.str)>0;
}
//一侧字符串大于等于另一侧
bool operator>=(const Mystring& R) const
{
return strcmp(str,R.str)>=0;
}
friend istream& operator>>(istream& L,Mystring& R);
friend ostream& operator<<(ostream& L,const Mystring& R);
private:
int len;
char *str;
};
//重载<<
ostream& operator<<(ostream& L,const Mystring& R)
{
L<<R.str;
return L;
}
//重载>>
istream& operator>>(istream& L,Mystring& R)
{
char buf[100];
L>>buf;
R=Mystring(buf);
return L;
}
int main(int argc,const char* argv[])
{
Mystring str("ni hao");
//字符串拼接
Mystring str1;
str1=str+" wen jie";
cout<<"str1="<<str1<<endl;
//两侧字符串不相等
Mystring str2("keli");
bool res0 = str2 != str;
cout<<"res0="<<res0<<endl;
//两侧字符串相等
Mystring str3("ycd");
bool res1 = str3 == "ycd";
cout<<"res1="<<res1<<endl;
//一侧字符串小于另一侧
Mystring str4("ycdab");
bool res2 = str4 < "ycdab";
cout<<"res2="<<res2<<endl;
//一侧字符串小于等于另一侧
Mystring str5("ycdab");
bool res3 = str5 <= "ycdab";
cout<<"res3="<<res3<<endl;
//一侧字符串大于另一侧
Mystring str6("ycdabe");
bool res4 = str6 > "ycdab";
cout<<"res4="<<res4<<endl;
//一侧字符串大于等于另一侧
Mystring str7("ycdab");
bool res5 = str7 >= "ycdab";
cout<<"res5="<<res5<<endl;
Mystring str8;
cout<<"请输入一个字符串"<<endl;
cin>>str8;
cout<<"str8="<<str8<<endl;
return 0;
}