还没写完
#include <iostream>
#include <cstring>
using namespace std;
class myString
{
private:
char *str; //字符串
int size; //实际字符长度
int len; //字符串容量
public:
myString():size(10) //无参构造函数
{
len = size + 1;
str = new char[size];
}
myString(const char*s):size(strlen(s)) //有参构造函数
{
//cout<<size<<endl;
len = size + 1;
str = new char[size+1];
strcpy(str,s);
}
~myString() //析构函数
{
delete []str;
}
myString &operator=(const myString &R); //=重载
char &at(const int &index); //at
char &operator[](const int &index); //下标重载
char *data(); //data
char *c_str(); //c_str
bool empty(); //判空
int sszie(); //字符实际长度
int capcity(); //字符串容量
void clear(); //清空
void push_back(const char &s); //尾插1个字符
void pop_back(); //尾删1个字符
void expand(); //二倍扩容
myString &operator+=(const myString &R); //+=重载
myString &operator+(const myString &R);
myString &operator+(const char &R);
void show(); //展示
};
//=
myString & myString:: operator=(const myString &R)
{
/*delete [] str;
size = R.size;
len = R.len;
char *newstr = new char[len];
strcpy(newstr,R.str);
str = newstr;*/
size = R.size;
len = R.len;
char *newstr = new char[len];
strcpy(newstr,R.str);
delete [] str;
str = newstr;
return *this;
}
//at
char &myString :: at(const int &index)
{
if(index > size || index < 0) //判断是否下标越界
{
cout<<"下标越界"<<endl;
exit(0);
}
return str[index];
}
//[]
char &myString::operator[](const int &index)
{
return str[index];
}
//data
char *myString ::data()
{
return &str[0];
}
//c_str
char *myString :: c_str()
{
return str;
}
//empty
bool myString::empty()
{
return size==0;
}
//ssize
int myString::sszie()
{
return size;
}
//capcity
int myString::capcity()
{
return len;
}
//clear
void myString::clear()
{
// delete [] str;
// str = new char[size+1];
memset(str,0,size+1);
}
//show
void myString ::show()
{
// cout<<size<<endl;
// cout<<strlen(str)<<endl;
cout<<str<<endl;
}
//尾插
void myString::push_back(const char &s)
{
if((size + 1) >= len) //判断是否需要二倍扩容
{
expand();
}
str[size] = s;
size++;
str[size] = '\0';
}
//尾删一个字符
void myString::pop_back()
{
str[size-1] = 0;
size--;
}
//+=
myString & myString::operator+=(const myString &R)
{
while(size + int(strlen(R.str)) >= len)
{
expand();
}
strcat(this->str,R.str);
return *this;
}
//二倍扩容
void myString::expand()
{
len = len * 2 - 1;
char*newstr = new char[len];
memset(newstr,0,len);
strcpy(newstr,str);
delete []str;
str = newstr;
}
//+
myString &myString::operator+(const myString &R) //两个字符串相加
{
while((size + R.size) >= len)
{
expand();
}
strcat(str,R.str);
size = size + R.size;
return *this;
}
myString &myString::operator+(const char &R) //字符串和单个字符相加
{
if(size + 1 >= len)
{
expand();
}
push_back(R);
return *this;
}
int main()
{
myString s1("nihao");
myString s2("hello world");
s1 = s2;
s1.show();
cout<<s1.at(1)<<endl;
s1[0] = 'H';
s1.show();
s1.push_back('!');
s1.show();
s1.pop_back();
s1.show();
myString s3;
s3 = s1 + s2 + s2;
s3.show();
cout<<"**************"<<endl;
s3 = s3 + '?' + '!';
s3.show();
s1.clear();
s1.show();
s1.at(-1);
return 0;
}
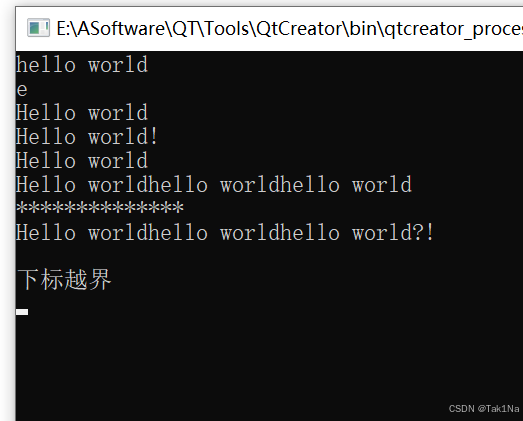