public async Task AddAsync<T>(T entity) where T : class
{
await _context.Set<T>().AddAsync(entity);
await _context.SaveChangesAsync();
}
//增加实体
public 表面方法是公开的,所有其他类都可以调用
async 表方法内可能包含异步操作,允许方法在内部使用"await"
await 在异步操作中使用,会暂停当前方法的执行(阻塞当前线程),直到方法执行完成后,才会继续执行下面的代码,暂停期间,控制权会返回给调用方(如UI线程)
Task 当一个 方法的返回类型是Task时,表面这个方法是异步的,但它不返回任何值(即它是'void'的异步版本,同理int的异步版本为Task<int>)。通过Task,调用者可以选择是否等待这个方法完成
AddAsync<T>(T entity) T是泛型类型的参数,它使得这个方法可以处理任意类型的实体对象。T由调用者传入的entity类型所决定。如果不使用泛型,只处理某一实体类型如User,也可以写成AddUserAsync(User entity)
where T : class 约束条件,限制了T必须是一个类
定义数据库
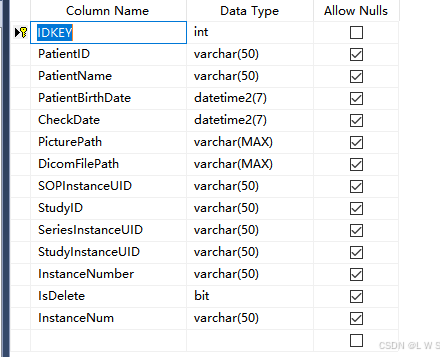
数据库名称:T_dicomPatientMsg
注意5大约束条件:
1.主键约束:primary key IDKEY设置为主键,主键设置自增长
2.唯一性约束:unique
3.默认约束:default 所有值都要设置默认值,除了主键
4.检查约束:check
5.外键约束:foreign key
定义实体
public class DicomPatientMsg
{
[Key]
public int IDKEY { get; set; } //设为主键,注意实体名称需要与数据库实体名称一致
public string PatientID { get; set; }
public string PatientName { get; set; }
public DateTime PatientBirthDate { get; set; }
public DateTime CheckDate { get; set; }
public string PicturePath { get; set; }
public string DicomFilePath { get; set; }
public string SOPInstanceUID { get; set; }
public string StudyID { get; set; }
public string StudyInstanceUID { get; set; }
public string SeriesInstanceUID { get; set; }
public string InstanceNum { get; set; }
public bool IsDelete { get; set; }
}
SQL帮助类
单例模式实现
public class SqlHelper
{
private static readonly Lazy<SqlHelper> instance = new Lazy<SqlHelper>(()=>new SqlHelper());
private readonly AppDbContext context;
// 私有构造函数,防止外部实例化
private SqlHelper()
{
var optionsBuilder = new DbContextOptionsBuilder<AppDbContext>();
var connectionString = "Server=.\\SQLEXPRESS;Database=Colposcope;User Id=sa;Password=123;TrustServerCertificate=True;";
optionsBuilder.UseSqlServer(connectionString);
context = new AppDbContext(optionsBuilder.Options);
}
//静态属性,返回单例
public static SqlHelper Instance => instance.Value;
// 增加实体
public async Task AddAsync<T>(T entity) where T : class
{
await context.Set<T>().AddAsync(entity);
await context.SaveChangesAsync();
}
// 获取所有实体
public async Task<List<T>> GetAllAsync<T>() where T : class
{
return await context.Set<T>().ToListAsync();
}
// 根据ID获取实体
public async Task<T> GetByIdAsync<T>(int id) where T : class
{
return await context.Set<T>().FindAsync(id);
}
// 更新实体
public async Task UpdateAsync<T>(T entity) where T : class
{
context.Set<T>().Update(entity);
await context.SaveChangesAsync();
}
// 删除实体
public async Task DeleteAsync<T>(T entity, bool isDelete = false) where T : class
{
if (isDelete)
{
context.Set<T>().Remove(entity);
}
else
{
var property = entity.GetType().GetProperty("IsDeleted");
if (property != null && property.PropertyType == typeof(bool))
{
property.SetValue(entity, true);
context.Set<T>().Update(entity);
}
else
{
throw new InvalidOperationException("Error");
}
}
await context.SaveChangesAsync();
}
}
使用数据库
private readonly static SqlHelper sqlHelper = SqlHelper.Instance;
DicomPatientMsg msg = new DicomPatientMsg()
{
DicomFilePath = patientMsg.DicomFilePath,
PatientID = patientMsg.PatientID,
PatientName = patientMsg.PatientName,
CheckDate = checkDate,
PicturePath = patientMsg.PicturePath,
SOPInstanceUID = dataset.GetString(DicomTag.SOPInstanceUID),
StudyID = dataset.GetString(DicomTag.StudyID),
StudyInstanceUID = dataset.GetString(DicomTag.StudyInstanceUID),
SeriesInstanceUID = dataset.GetString(DicomTag.SeriesInstanceUID),
InstanceNum = dataset.GetString(DicomTag.InstanceNumber),
};
//存储到sql
await sqlHelper.AddAsync(msg);