海康相机触发模式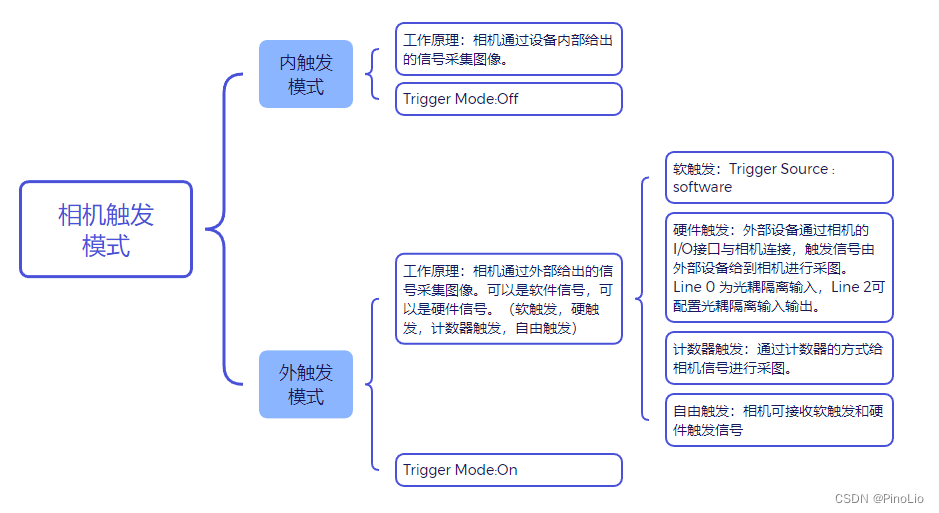
软件设置:先设置触发模式,在设置触发源。
目的:模拟编码器发送信号触发相机采集,通过一个矩形波信号触发采集图像。
计数器触发
说明书MSV客户端步骤:
实验过程
1、编码器周长300mm,转一圈产生300个脉冲信号,编码器每产生一个脉冲信号时,代表皮带向前移动300mm/300=1mm.
2、皮带速度为1500mm/s,相机横向视野为750mm,因此相机没秒会拍摄1500/750=2张图像。
3、没经过500ms或者750/1mm=750个脉冲,拍一张图像。
4、程序上,收到一个编码器的脉冲信号,变量自增1,当变量的值可以被750整除时,进行采集。
实际客户端步骤
接线
绿色接地线,黄色接+,灰色接电源地,橙色接电源正极。
程序代码
三部分代码:
//回调函数
void __stdcall ImageCallBackEx(unsigned char * pData, MV_FRAME_OUT_INFO_EX* pFrameInfo, void* pUser)
{
MVCamera* camera;
camera = (MVCamera*)pUser;
// 图像转换信息
MV_CC_PIXEL_CONVERT_PARAM stConvertParam = {0};
stConvertParam.nWidth = pFrameInfo->nWidth; // image width
stConvertParam.nHeight = pFrameInfo->nHeight; // image height
stConvertParam.pSrcData = pData; // input data buffer
stConvertParam.nSrcDataLen = pFrameInfo->nFrameLen; // input data size
stConvertParam.enSrcPixelType = pFrameInfo->enPixelType; // input pixel format
stConvertParam.enDstPixelType = PixelType_Gvsp_BGR8_Packed; // output pixel format
stConvertParam.pDstBuffer = (unsigned char*)malloc(pFrameInfo->nWidth * pFrameInfo->nHeight * 3); // output data buffer
stConvertParam.nDstBufferSize = pFrameInfo->nWidth * pFrameInfo->nHeight * 3; // output buffer size
MV_CC_ConvertPixelType(camera->hDevHandle, &stConvertParam);
int nRet = camera->convertPix(stConvertParam);
if (MV_OK != nRet)
{
qDebug() << "图像格式转化错误";
}
camera->nowImage = cv::Mat(cv::Size(4096, 3000), CV_8UC3, stConvertParam.pDstBuffer).clone();//修改1624*1240
camera->isHaveImage = true;
// std::cout << "call back" << std::endl;
}
//设置相机配置
int MVCamera::encoderCountGrbbingSetting()
{
if (!isConn)
{
qDebug() << "相机未连接";
return -1;
}
if (isStreaming)
{
qDebug() << "相机正在取流,无法设置参数,请先停止取流";
return -1;
}
//设置触发源为开启状态
int nRet = MV_CC_SetEnumValueByString(hDevHandle, "TriggerMode", "On");
if (MV_OK != nRet)
{
qDebug() << "设置触发模式失败";
return -1;
}
//设置触发源类型
nRet = MV_CC_SetEnumValueByString(hDevHandle, "TriggerSource", "Counter0");
if (MV_OK != nRet)
{
qDebug() << "设置触发源类型失败";
return -1;
}
//设置计数器控制中的计数器选择
nRet = MV_CC_SetEnumValueByString(hDevHandle, "CounterSelector", "Counter0");
if (MV_OK != nRet)
{
qDebug() << "设置计数器选择类型失败";
return -1;
}
//设置计数器控制中的计数器事件源
nRet = MV_CC_SetEnumValueByString(hDevHandle, "CounterEventSource", "Line0");
if (MV_OK != nRet)
{
qDebug() << "设置计数器事件源选择类型失败";
return -1;
}
nRet = MV_CC_SetEnumValueByString(hDevHandle, "CounterResetSource", "Off");
if (MV_OK != nRet)
{
qDebug() << "设置计数器复位原类型失败";
return -1;
}
nRet = MV_CC_SetIntValue(hDevHandle,"CounterValue",500);
if (MV_OK != nRet)
{
qDebug() << "设置计数器复位原类型失败";
return -1;
}
// 设置回调函数
nRet = MV_CC_RegisterImageCallBackEx(hDevHandle, ImageCallBackEx, this);
if (MV_OK != nRet)
{
qDebug() << "设置回调函数失败";
return -1;
}
unsigned int nImageNodeNum = 2;
// 设置缓存图像个数
nRet = MV_CC_SetImageNodeNum(hDevHandle, nImageNodeNum);
if (MV_OK != nRet)
{
qDebug() << "设置相机缓存成功";
return -1;
}
nRet = MV_CC_SetGrabStrategy(hDevHandle, MV_GrabStrategy_LatestImagesOnly);//仅从输出缓存列表中获取最新的一帧图像,同时清空输出缓存列表
if (MV_OK != nRet)
{
qDebug() << "设置相机取图策略成功";
}
qDebug() << "主动取流设置成功";
return 0;
}
//打开相机
int MVCamera::startStreaming()
{
if (!isConn)
{
qDebug() << "相机未连接";
return -1;
}
int nRet = nRet = MV_CC_StartGrabbing(hDevHandle);
if (MV_OK != nRet) //判断是否开始采集图像
{
qDebug() << "相机开始采集图像失败";
return -1;
}
qDebug() << "相机开始拉流";
isStreaming = true;
return 0;
}
导入文件到相机以及导出相机文件
#include <stdio.h>
#include <Windows.h>
#include <process.h>
#include <conio.h>
#include "MvCameraControl.h"
unsigned int g_nMode = 0;
int g_nRet = MV_OK;
// Wait for key press
void WaitForKeyPress(void)
{
while(!_kbhit())
{
Sleep(10);
}
_getch();
}
bool PrintDeviceInfo(MV_CC_DEVICE_INFO* pstMVDevInfo)
{
if (NULL == pstMVDevInfo)
{
printf("The Pointer of pstMVDevInfo is NULL!\n");
return false;
}
if (pstMVDevInfo->nTLayerType == MV_GIGE_DEVICE)
{
int nIp1 = ((pstMVDevInfo->SpecialInfo.stGigEInfo.nCurrentIp & 0xff000000) >> 24);
int nIp2 = ((pstMVDevInfo->SpecialInfo.stGigEInfo.nCurrentIp & 0x00ff0000) >> 16);
int nIp3 = ((pstMVDevInfo->SpecialInfo.stGigEInfo.nCurrentIp & 0x0000ff00) >> 8);
int nIp4 = (pstMVDevInfo->SpecialInfo.stGigEInfo.nCurrentIp & 0x000000ff);
// print current ip and user defined name
printf("CurrentIp: %d.%d.%d.%d\n" , nIp1, nIp2, nIp3, nIp4);
printf("UserDefinedName: %s\n\n" , pstMVDevInfo->SpecialInfo.stGigEInfo.chUserDefinedName);
}
else if (pstMVDevInfo->nTLayerType == MV_USB_DEVICE)
{
printf("UserDefinedName: %s\n", pstMVDevInfo->SpecialInfo.stUsb3VInfo.chUserDefinedName);
printf("Serial Number: %s\n", pstMVDevInfo->SpecialInfo.stUsb3VInfo.chSerialNumber);
printf("Device Number: %d\n\n", pstMVDevInfo->SpecialInfo.stUsb3VInfo.nDeviceNumber);
}
else
{
printf("Not support.\n");
}
return true;
}
static unsigned int __stdcall ProgressThread(void* pUser)
{
int nRet = MV_OK;
MV_CC_FILE_ACCESS_PROGRESS stFileAccessProgress = {0};
while(1)
{
// Get progress of file access
nRet = MV_CC_GetFileAccessProgress(pUser, &stFileAccessProgress);
printf("State = 0x%x,Completed = %I64d,Total = %I64d\r\n",nRet,stFileAccessProgress.nCompleted,stFileAccessProgress.nTotal);
if (nRet != MV_OK || (stFileAccessProgress.nCompleted != 0 && stFileAccessProgress.nCompleted == stFileAccessProgress.nTotal))
{
break;
}
Sleep(50);
}
return 0;
}
static unsigned int __stdcall FileAccessThread(void* pUser)
{
MV_CC_FILE_ACCESS stFileAccess = {0};
stFileAccess.pUserFileName = "UserSet1.bin";
stFileAccess.pDevFileName = "UserSet1";
if (1 == g_nMode)
{
// Read mode
g_nRet = MV_CC_FileAccessRead(pUser, &stFileAccess);
if (MV_OK != g_nRet)
{
printf("File Access Read fail! nRet [0x%x]\n", g_nRet);
}
}
else if (2 == g_nMode)
{
// Write mode
g_nRet = MV_CC_FileAccessWrite(pUser, &stFileAccess);
if (MV_OK != g_nRet)
{
printf("File Access Write fail! nRet [0x%x]\n", g_nRet);
}
}
return 0;
}
int main()
{
int nRet = MV_OK;
void* handle = NULL;
do
{
// Enum device
MV_CC_DEVICE_INFO_LIST stDeviceList;
memset(&stDeviceList, 0, sizeof(MV_CC_DEVICE_INFO_LIST));
nRet = MV_CC_EnumDevices(MV_GIGE_DEVICE | MV_USB_DEVICE, &stDeviceList);
if (MV_OK != nRet)
{
printf("Enum Devices fail! nRet [0x%x]\n", nRet);
break;
}
if (stDeviceList.nDeviceNum > 0)
{
for (unsigned int i = 0; i < stDeviceList.nDeviceNum; i++)
{
printf("[device %d]:\n", i);
MV_CC_DEVICE_INFO* pDeviceInfo = stDeviceList.pDeviceInfo[i];
if (NULL == pDeviceInfo)
{
break;
}
PrintDeviceInfo(pDeviceInfo);
}
}
else
{
printf("Find No Devices!\n");
break;
}
printf("Please Input camera index:");
unsigned int nIndex = 0;
scanf_s("%d", &nIndex);
if (nIndex >= stDeviceList.nDeviceNum)
{
printf("Input error!\n");
break;
}
// Select device and create handle
nRet = MV_CC_CreateHandle(&handle, stDeviceList.pDeviceInfo[nIndex]);
if (MV_OK != nRet)
{
printf("Create Handle fail! nRet [0x%x]\n", nRet);
break;
}
// Open device
nRet = MV_CC_OpenDevice(handle);
if (MV_OK != nRet)
{
printf("Open Device fail! nRet [0x%x]\n", nRet);
break;
}
// Read mode
g_nMode = 1;
printf("Read to file.\n");
unsigned int nThreadID = 0;
void* hReadHandle = (void*) _beginthreadex( NULL , 0 , FileAccessThread , handle, 0 , &nThreadID );
if (NULL == hReadHandle)
{
break;
}
Sleep(5);
nThreadID = 0;
void* hReadProgressHandle = (void*) _beginthreadex( NULL , 0 , ProgressThread , handle, 0 , &nThreadID );
if (NULL == hReadProgressHandle)
{
break;
}
WaitForMultipleObjects(1, &hReadHandle, TRUE, INFINITE);
WaitForMultipleObjects(1, &hReadProgressHandle, TRUE, INFINITE);
if (MV_OK == g_nRet)
{
printf("File Access Read Success!\n");
}
printf("\n");
// Write mode
g_nMode = 2;
printf("Write from file.\n");
nThreadID = 0;
void* hWriteHandle = (void*) _beginthreadex( NULL , 0 , FileAccessThread , handle, 0 , &nThreadID );
if (NULL == hWriteHandle)
{
break;
}
Sleep(5);
nThreadID = 0;
void* hWriteProgressHandle = (void*) _beginthreadex( NULL , 0 , ProgressThread , handle, 0 , &nThreadID );
if (NULL == hWriteProgressHandle)
{
break;
}
WaitForMultipleObjects(1, &hWriteHandle, TRUE, INFINITE);
WaitForMultipleObjects(1, &hWriteProgressHandle, TRUE, INFINITE);
if (MV_OK == g_nRet)
{
printf("File Access Write Success!\n");
}
// Close device
nRet = MV_CC_CloseDevice(handle);
if (MV_OK != nRet)
{
printf("ClosDevice fail! nRet [0x%x]\n", nRet);
break;
}
// Destroy handle
nRet = MV_CC_DestroyHandle(handle);
if (MV_OK != nRet)
{
printf("Destroy Handle fail! nRet [0x%x]\n", nRet);
break;
}
} while (0);
if (nRet != MV_OK)
{
if (handle != NULL)
{
MV_CC_DestroyHandle(handle);
handle = NULL;
}
}
printf("Press a key to exit.\n");
WaitForKeyPress();
return 0;
}