表格是在前端页面中是经常被用到的,尤其是管理系统,几乎每个页面都会存在表格,所以掌握表格组件是非常有必要的。element-plus提供el-table,el-table-column来渲染表格,
1. el-table
组件主要属性
属性名 | 作用 | 值类型 | 枚举值 | 默认值 |
---|
data | 表格数据 | 对象数组 | | [] |
row-key | 关键属性,用于加速渲染的,类似v-for的key属性 | function 或string | | |
border | 是否有边框 | boolean | | false |
stripe | 是否有斑马线 | boolean | | false |
highlight-current-row | 高亮选择的行 | boolean | | false |
tree-props | 子属性以属性结构映射 | object | | { hasChildren: ‘hasChildren’, children: ‘children’ } |
lazy | 懒加载子元素 | boolean | | false |
load | 展开子元素时加载函数,可远程加载子元素,返回promise | function | | |
组件主要事件
事件名名 | 作用 | |
---|
select | 选择行 | 对象数组 |
sort-change | 排序 | function 或string |
current-change | 当前选择的行 | boolean |
stripe | 是否有斑马线 | boolean |
highlight-current-row | 高亮选择的行 | boolean |
page-sizes | 每页条目选择列表 | 数字数组 |
layout | 组件布局 ,上下翻页,跳页等布局设置 ,多项逗号分割 | 字符串 |
1. el-table-column (表格列组件)
组件主要属性
属性名 | 作用 | 值类型 | 枚举值 | 默认值 |
---|
label | 表格列的标题 | string | | |
prop | 属性名于el-table的data元素的属性名对应 | string | | |
type | 类型 | boolean | selection,index,expand | false |
width | 宽度,单位px | number | | |
fixed | 是否固定列 | string | left,right | false |
sortable | 高亮选择的行 | boolean | | false |
sort-method | 指定数据按照哪个属性进行排序,仅当sortable设置为true的时候有效。 应该如同 Array.sort 那样返回一个 Number | function | | |
sort-by | 指定数据按照哪个属性进行排序,仅当 sortable 设置为 true 且没有设置 sort-method 的时候有效。 如果 sort-by 为数组,则先按照第 1 个属性排序,如果第 1 个相等,再按照第 2 个排序,以此类推 | Function,string,string[] | | false |
sort-orders | 数据在排序时所使用排序策略的轮转顺序,仅当 sortable 为 true 时有效。 需传入一个数组,随着用户点击表头,该列依次按照数组中元素的顺序进行排序 | string[] | | |
组件插槽
插槽名 | 作用 | 作用域属性 |
---|
default | 自定义列内容 | row,column,$index |
header | 自定义表头 | column,$indexcustom才有效 |
3.用法例子
<script setup lang="ts">
import { aG } from 'vitest/dist/reporters-LqC_WI4d.js';
import { onMounted, reactive, ref, watch } from 'vue'
const pageSizes = ref([10, 20, 50, 100]);
const currentPageSize = ref(10)
const currentPage = ref(1)
class Student {
id: number
name: string
age: number
address: string
children: Student[]
hasChildren: boolean
constructor(id: number, name: string, age: number, address: string) {
this.id = id
this.name = name
this.age = age
this.address = address
this.children = []
this.hasChildren = false
}
}
watch(currentPage, (newPage, oldPage) => {
console.log(newPage, oldPage)
})
const tableData = ref([
new Student(1, "zhangsanxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx", 30, "beijing"),
new Student(2, "lisi1", 30, "shanghai"),
new Student(3, "lisi2", 30, "shanghai"),
new Student(4, "lisi3", 30, "shanghai"),
new Student(5, "lisi4", 30, "shanghai"),
new Student(6, "lisi5", 30, "shanghai"),
new Student(7, "lisi6", 30, "shanghai"),
new Student(8, "lisi7", 30, "shanghai"),
new Student(9, "lisi8", 30, "shanghai"),
{
id: 10, name: "lisi8", age: 30, address: "shanghai",
hasChildren: true,
children: [
{
id: 91, name: "wangwu1", age: 30, address: "shenzhen"
},
{
id: 92, name: "wangwu12", age: 30, address: "shenzhen"
}
]
},
])
const load = (
row: Student,
treeNode: unknown,
resolve: (date: Student[]) => void
) => {
setTimeout(() => {
resolve([
{
id: 91, name: "wangwu1", age: 30, address: "shenzhen", hasChildren: false, children: []
},
{
id: 92, name: "wangwu12", age: 30, address: "shenzhen", hasChildren: false, children: []
},
])
}, 1000)
}
const currentRow = ref()
const rowChange = (s: Student) => {
console.log(s)
}
const sortChange = (cloumn: any, prop: string, order: string) => {
console.log(cloumn, prop, order)
}
const selectChange=(selectionsS:Student[],row:Student)=>{
console.log(selectionsS,row)
}
const toDo=(scope:any)=>{
console.log(scope.id)
}
</script>
<template>
<div>
<el-container>
<el-header></el-header>
<el-main>
<el-table :data="tableData" lazy :load="load" row-key="id" @select="selectChange"
:tree-props="{ hasChildren: 'hasChildren', children: 'children' }" border stripe height="250" style="width:100%"
highlight-current-row @sort-change="sortChange" @current-change="rowChange">
<el-table-column label="id" sortable fixed prop="id" width="100"></el-table-column>
<el-table-column label="name" sortable="custom" prop="name" width="100" show-overflow-tooltip>
</el-table-column>
<el-table-column label="age" prop="age">
</el-table-column>
<el-table-column label="address" prop="address">
</el-table-column>
<el-table-column fixed="right" label="Operations" min-width="120">
<template #default="scope">
<el-button
link
type="primary"
size="small"
@click.prevent="toDo(scope.row)"
>
Remove
</el-button>
</template>
</el-table-column>
</el-table>
</el-main>
<el-footer></el-footer>
</el-container>
<el-container>
<el-header></el-header>
<el-main>
<el-table :data="tableData" border stripe height="250" style="width:100%" highlight-current-row @select="selectChange"
@sort-change="sortChange" @current-change="rowChange">
<el-table-column type="selection" width="100"></el-table-column>
<el-table-column label="id" sortable fixed prop="id" width="100"></el-table-column>
<el-table-column label="name" sortable="custom" prop="name" width="100" show-overflow-tooltip>
</el-table-column>
<el-table-column label="age" prop="age">
</el-table-column>
<el-table-column label="address" prop="address">
</el-table-column>
<el-table-column fixed="right" label="Operations" min-width="120">
<template #default="scope">
<el-button type="primary" @click.prevent="toDo(scope.row)">修改</el-button>
</template>
</el-table-column>
</el-table>
</el-main>
<el-footer></el-footer>
</el-container>
<el-pagination :page-sizes="pageSizes" v-model:page-size="currentPageSize" v-model:current-page="currentPage"
layout="sizes,prev,next,jumper,pager,>" :total="200"></el-pagination>
</div>
</template>
<style scoped></style>
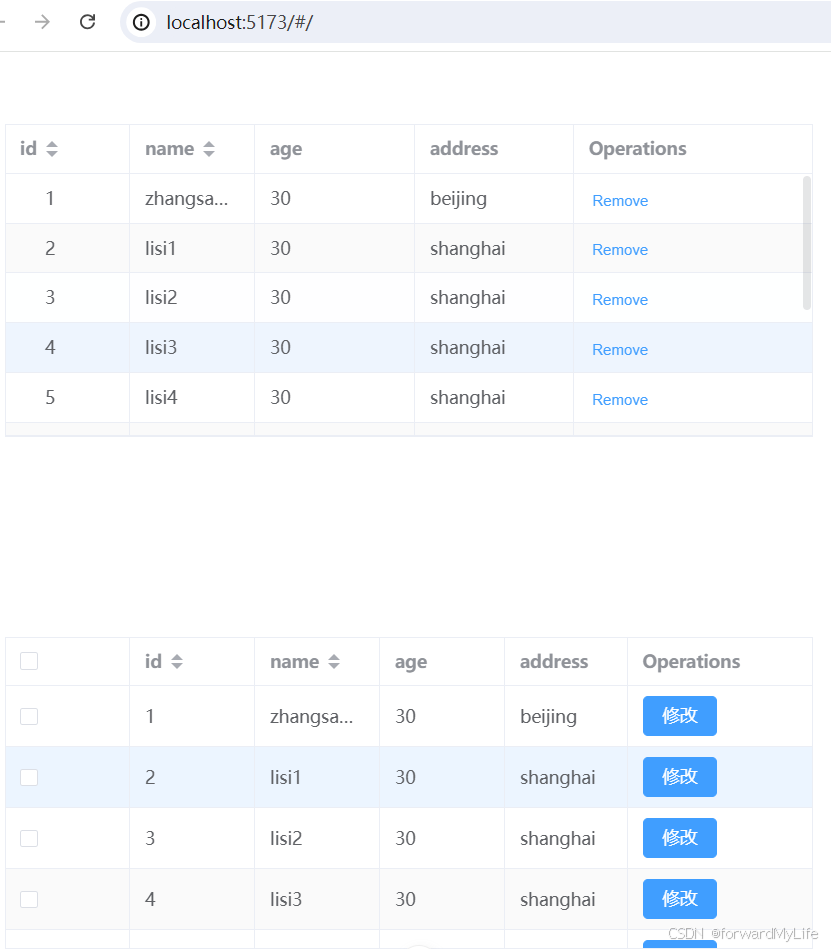
https://element-plus.org/zh-CN/component/table.html#%E8%A1%A8%E6%A0%BC%E5%B8%83%E5%B1%80