tcpSer.c
#include <myhead.h>
#define SER_IP "192.168.119.143" // 设置IP地址
#define SER_PORT 6666 // 设置端口号
int main(int argc, const char *argv[])
{
// 1.创建socket
int serfd = socket(AF_INET, SOCK_STREAM, 0);
// 参数1表示ipv4
// 参数2表示tcp通信
// 参数3表示通讯协议
if (serfd == -1)
{
perror("socket error");
return -1;
}
// 将端口号快速重用
int reuse = 1;
if (setsockopt(serfd, SOL_SOCKET, SO_REUSEADDR, &reuse, sizeof(reuse)) == -1)
{
perror("setsockopt error");
return -1;
}
// 2.绑定信息
struct sockaddr_in sin;
sin.sin_family = AF_INET; // 设置通讯域
sin.sin_port = htons(SER_PORT); // 设置端口号
sin.sin_addr.s_addr = inet_addr(SER_IP); // 设置ip地址
if (bind(serfd, (struct sockaddr *)&sin, sizeof(sin)) == -1)
{
perror("bind error");
return -1;
}
printf("bind success\n");
// 3.设置为监听
if (listen(serfd, 128))
{
perror("listen error");
return -1;
}
printf("listen success\n");
// 4.连接
struct sockaddr_in cin;
socklen_t socklen = sizeof(cin);
int newfd = accept(serfd, (struct sockaddr *)&cin, &socklen);
if (newfd == -1)
{
perror("accept error");
return -1;
}
printf("[%s:%d]accept success\n", inet_ntoa(cin.sin_addr), ntohs(cin.sin_port));
// 5.接收数据
char buf[128] = "";
while (1)
{
// 数据初始化
bzero(buf, sizeof(buf));
int res = recv(newfd, buf, sizeof(buf), 0);
if (res == -1)
{
printf("读取失败\n");
return -1;
}
else if (res == 0)
{
printf("客户端以下线\n");
return -1;
}
if (strcmp(buf, "quit") == 0)
{
printf("服务端退出\n");
break;
}
printf("[%s:%d]:%s\n", inet_ntoa(cin.sin_addr), ntohs(cin.sin_port), buf);
bzero(buf, sizeof(buf));
strcat(buf, "*-*");
if (send(newfd, buf, sizeof(buf), 0) == -1)
{
perror("send error");
return -1;
} // 回复消息
printf("回复成功\n");
}
// 6.关闭
close(newfd);
return 0;
}
tcpCli.c
#include <myhead.h>
#define SER_IP "192.168.119.143"
#define SER_PORT 6666
#define CLI_IP "192.168.119.143"
#define CLI_PORT 8888
int main(int argc, const char *argv[])
{
// 1.创建
int clifd = socket(AF_INET, SOCK_STREAM, 0);
if (clifd == -1)
{
perror("socket error");
return -1;
}
// 2.绑定
struct sockaddr_in cin;
cin.sin_family = AF_INET;
cin.sin_port = htons(CLI_PORT);
cin.sin_addr.s_addr = inet_addr(CLI_IP);
if (bind(clifd, (struct sockaddr *)&cin, sizeof(cin)) == -1)
{
perror("bind error");
return -1;
}
printf("bind success\n");
// 3.连接
struct sockaddr_in sin;
sin.sin_family = AF_INET;
sin.sin_port = htons(SER_PORT);
sin.sin_addr.s_addr = inet_addr(SER_IP);
if (connect(clifd, (struct sockaddr *)&sin, sizeof(sin)) == -1)
{
perror("connect error");
return -1;
}
printf("connect success\n");
// 4.数据收发
char buf[128] = "";
while (1)
{
bzero(buf, sizeof(buf));
printf("请输入>>>");
fgets(buf, sizeof(buf), stdin);
buf[strlen(buf) - 1] = 0;
if (send(clifd, buf, sizeof(buf), 0) == -1)
{
perror("send error");
return -1;
}
if (strcmp(buf, "quit") == 0)
{
printf("客户端退出\n");
break;
}
printf("发送成功\n");
bzero(buf, sizeof(buf));
recv(clifd, buf, sizeof(buf), 0);
printf("收到服务器的消息:%s\n", buf);
}
// 5.关闭
close(clifd);
return 0;
}
效果展示
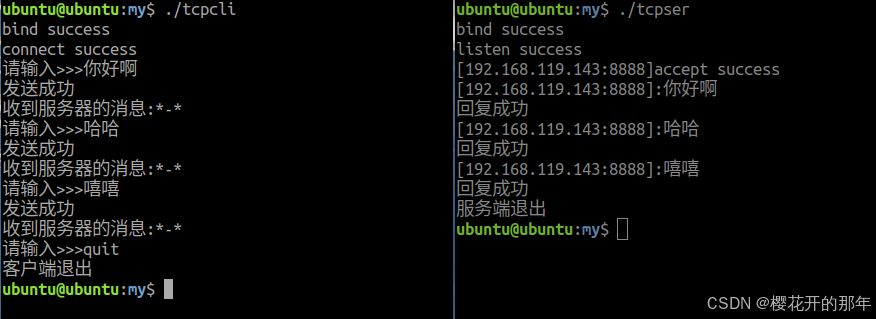