1,获取网络的通断。
//方法1:无效果,并不能反映当前网络通断
bool available=System.Windows.Forms.SystemInformation.Network
//方法2:通过VB获取网络状态,可反映当前网络通断
Microsoft.VisualBasic.Devices.Network network = new Microsoft.VisualBasic.Devices.Network();
bool available=network.IsAvailable;
//方法3,可反映当前网络通断
bool available=System.Net.NetworkInformation.NetworkInterface.GetIsNetworkAvailable()
2,自定义获取Host IP ,Mac,局域网内活动IP与Mac的类。
/// <summary>
/// 本机网络连接类
/// </summary>
class NetworkInfo
{
/// <summary>
/// 是否有网络连接
/// </summary>
public bool Available
{
get
{
return NetworkInterface.GetIsNetworkAvailable();
}
}
/// <summary>
/// 获取主机上在用的IP信息集合
/// </summary>
/// <returns></returns>
public NetworkAddress[] GetHostNetworkAddress()
{
List<NetworkAddress> list = new List<NetworkAddress>();
foreach (var item in NetworkInterface.GetAllNetworkInterfaces())
{
if (item.OperationalStatus == OperationalStatus.Up)
{
if (item.NetworkInterfaceType == NetworkInterfaceType.Ethernet || item.NetworkInterfaceType == NetworkInterfaceType.Wireless80211)
{
NetworkAddress info = new NetworkAddress();
info.Description = item.Description;
foreach (var t in item.GetIPProperties().UnicastAddresses)
{
if (t.Address.AddressFamily == AddressFamily.InterNetwork)
{
info.IPv4Address = t.Address.ToString();
}
if (t.Address.AddressFamily == AddressFamily.InterNetworkV6)
{
info.IPv6Address = t.Address.ToString();
}
}
string physical = item.GetPhysicalAddress().ToString();
if (physical != null && physical.Length > 0)
{
List<string> tempList = new List<string>();
for (int i = 0; i < physical.Length; i += 2)
{
tempList.Add(physical.Substring(i, 2));
}
physical = string.Join("-", tempList);
}
info.PhysicalAddress = physical;
list.Add(info);
}
}
}
return list.ToArray();
}
/// <summary>
/// 获取局域网上所有活动的IP与Mac(耗时长)
/// </summary>
/// <param name="ipv4">ipv4格式的IP 地址</param>
/// <returns></returns>
public async Task<NetworkAddress[]> ScanLocalAreaNetworkAsync(string ipv4)
{
//格式验证
if (!System.Text.RegularExpressions.Regex.IsMatch(ipv4, @"\d{1,3}\.\d{1,3}\.\d{1,3}\.\d{1,3}"))
{
throw new ArgumentException("格式错误,不是有效的IP地址", nameof(ipv4));
}
string networkNumber = ipv4.Remove(ipv4.LastIndexOf('.'));
await Task.Run(() =>
{
// for / L % i IN(1, 1, 254) DO ping - w 2 - n 1 192.168.0.% i
var result = RunDOS($"for /L %i IN (1,1,254) DO ping -w 1 -n 1 {networkNumber}.%i");
});
//获取局域网中活动的IP命令:arp -a
string msg = RunDOS("arp -a");
// string msg = RunDOS("ipconfig/all");
var arr = System.Text.RegularExpressions.Regex.Matches(msg, @"(\d{1,3}\.\d{1,3}\.\d{1,3}\.\d{1,3})\s+(\w[0-9a-z\-]+)\s");
List<NetworkAddress> list = new List<NetworkAddress>();
foreach (System.Text.RegularExpressions.Match item in arr)
{
if (item.Groups.Count == 3)
{
NetworkAddress netip = new NetworkAddress
{
IPv4Address = item.Groups[1].Value,
PhysicalAddress = item.Groups[2].Value
};
list.Add(netip);
}
}
return list.ToArray();
}
/// <summary>
/// 通过Ping获取活动的Local Area Network IPv4地址
/// </summary>
/// <param name="ipv4"></param>
/// <returns></returns>
public NetworkAddress[] ScanLocalAreaNetwork(string ipv4)
{
//格式验证
if (!System.Text.RegularExpressions.Regex.IsMatch(ipv4, @"\d{1,3}\.\d{1,3}\.\d{1,3}\.\d{1,3}"))
{
throw new ArgumentException("格式错误,不是有效的IP地址", nameof(ipv4));
}
string networkNumber = ipv4.Remove(ipv4.LastIndexOf('.'));
List<NetworkAddress> list = new List<NetworkAddress>();
Ping ping = new Ping();
for (int i = 1; i < 255; i++)
{
string targetIp = $"{networkNumber}.{i}";
PingReply reply = ping.Send(targetIp, 1000);
if (reply.Status == IPStatus.Success)
{
NetworkAddress net = new NetworkAddress
{
IPv4Address = reply.Address.ToString(),
PhysicalAddress = GetMacAddress(reply.Address.ToString())
};
list.Add(net);
}
}
return list.ToArray();
}
/// <summary>
/// 获取公网IP
/// </summary>
/// <returns></returns>
public string GetPublicNetworkIp()
{
if (!Available)
{
return "网络断开,无可用网络!";
}
try
{
WebClient client = new WebClient();
string querySite = "https://qifu-api.baidubce.com/ip/local/geo/v1/district";
client.Encoding = Encoding.UTF8;
string result = client.DownloadString(querySite);
dynamic json = Newtonsoft.Json.JsonConvert.DeserializeObject(result);
string msg = $"{json.data.owner} {json.data.prov} {json.data.city} {json.ip}";
return msg;
}
catch (Exception ex)
{
return ex.Message;
}
}
/// <summary>
/// 运行Dos指令
/// </summary>
/// <param name="cmdStr">DOS命令</param>
/// <returns></returns>
private string RunDOS(string cmdStr)
{
ProcessStartInfo startInfo = new ProcessStartInfo();
startInfo.FileName = "cmd.exe";
startInfo.UseShellExecute = false;
startInfo.CreateNoWindow = true;
startInfo.RedirectStandardInput = true;
startInfo.RedirectStandardOutput = true;
startInfo.RedirectStandardError = true;
Process p = Process.Start(startInfo);
//注意这里需要使用writeLine,因为这个带有回车符代表执行
p.StandardInput.WriteLine(cmdStr);
p.StandardInput.Close();
string result = p.StandardOutput.ReadToEnd();
p.WaitForExit();
p.Close();
return result;
}
public static string GetMacAddress(string RemoteIP)
{
StringBuilder macAddress = new StringBuilder();
try
{
Int32 remote = inet_addr(RemoteIP);
Int64 macInfo = new Int64();
Int32 length = 6;
SendARP(remote, 0, ref macInfo, ref length);
string temp = Convert.ToString(macInfo, 16).PadLeft(12, '0').ToUpper();
int x = 12;
for (int i = 0; i < 6; i++)
{
if (i == 5)
{
macAddress.Append(temp.Substring(x - 2, 2));
}
else
{
macAddress.Append(temp.Substring(x - 2, 2) + "-");
}
x -= 2;
}
return macAddress.ToString();
}
catch
{
return macAddress.ToString();
}
}
[DllImport("Iphlpapi.dll")]
static extern int SendARP(Int32 DestIP, Int32 SrcIP, ref Int64 MacAddr, ref Int32 PhyAddrLen);
[DllImport("Ws2_32.dll")]
static extern Int32 inet_addr(string ipaddr);
///<summary>
/// SendArp获取MAC地址
///</summary>
///<param name="RemoteIP">目标机器的IP地址如(192.168.1.1)</param>
///<returns>目标机器的mac 地址</returns>
}
class NetworkAddress
{
/// <summary>
/// IPv4地址
/// </summary>
public string IPv4Address { get; set; }
/// <summary>
/// IPv6地址
/// </summary>
public string IPv6Address { get; set; }
/// <summary>
/// 物理地址
/// </summary>
public string PhysicalAddress { get; set; }
public string Description { get; set; }
}
3,效果:
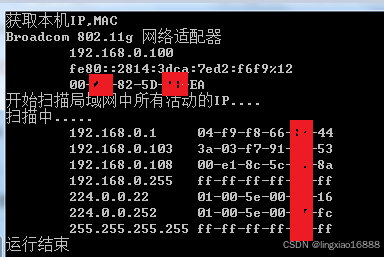