使用两个线程完成两个文件的拷贝,分支线程1拷贝前一半,分支线程2拷贝后一半,主线程回收两个分支线程的资源
#include <myhead.h>
struct Buf
{
const char *file1;
const char *file2;
int start;
int size;
};
int get_len(const char *arr,const char *brr)
{
int fd1 = -1,fd2 = -1;
if( (fd1=open(arr,O_RDONLY))==-1 )
{
perror("open error\n");
return -1;
}
if( (fd2=open(brr,O_WRONLY|O_CREAT|O_TRUNC,0644))==-1 )
{
perror("open error\n");
return -1;
}
int len = lseek(fd1,0,SEEK_END);
close(fd1);
close(fd2);
return len;
}
void copy_file(const char *arr,const char *brr,int start,int size)
{
int fd1 = -1,fd2 = -1;
if( (fd1=open(arr,O_RDONLY))==-1 )
{
perror("open error\n");
return ;
}
if( (fd2=open(brr,O_WRONLY))==-1 )
{
perror("open error\n");
return ;
}
lseek(fd1,start,SEEK_SET);
lseek(fd2,start,SEEK_SET);
char buf[128]="";
int num = 0;
while(1)
{
int res = read(fd1,buf,sizeof(buf));
num+=res;
if(res==0 || num>size)
{
write(fd2,buf,res-(num-size));
break;
}
write(fd2,buf,res);
}
close(fd1);
close(fd2);
}
void *task1(void*arg)
{
copy_file(((struct Buf *)arg)->file1,((struct Buf *)arg)->file2,((struct Buf *)arg)->start,((struct Buf *)arg)->size);
}
void *task2(void*arg)
{
copy_file(((struct Buf *)arg)->file1,((struct Buf *)arg)->file2,((struct Buf *)arg)->start,((struct Buf *)arg)->size);
}
int main(int argc,const char *argv[])
{
if(argc!=3)
{
perror("error\n");
return -1;
}
int len = get_len(argv[1],argv[2]);
struct Buf buf1 = {argv[1],argv[2],0,len/2};
struct Buf buf2 = {argv[1],argv[2],len/2,len-len/2};
pthread_t tid1 = -1,tid2 = -1;
if( pthread_create(&tid1,NULL,task1,&buf1)!=0 )
{
printf("pthread_create1 error\n");
return -1;
}
if( pthread_create(&tid2,NULL,task2,&buf2)!=0 )
{
printf("pthread_create2 error\n");
}
pthread_join(tid1,NULL);
pthread_join(tid2,NULL);
return 0;
}
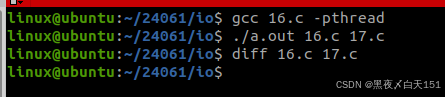
思维导图
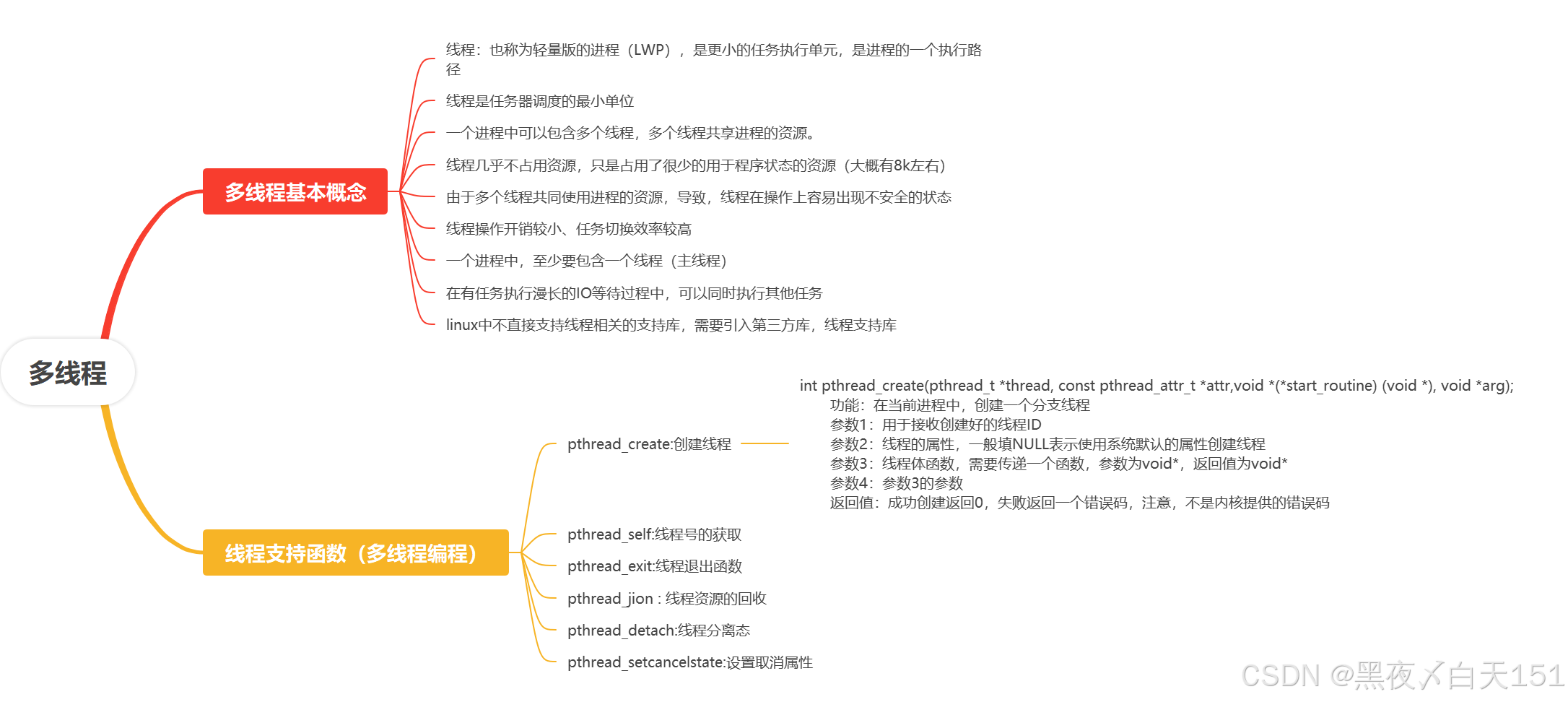