对象拷贝:
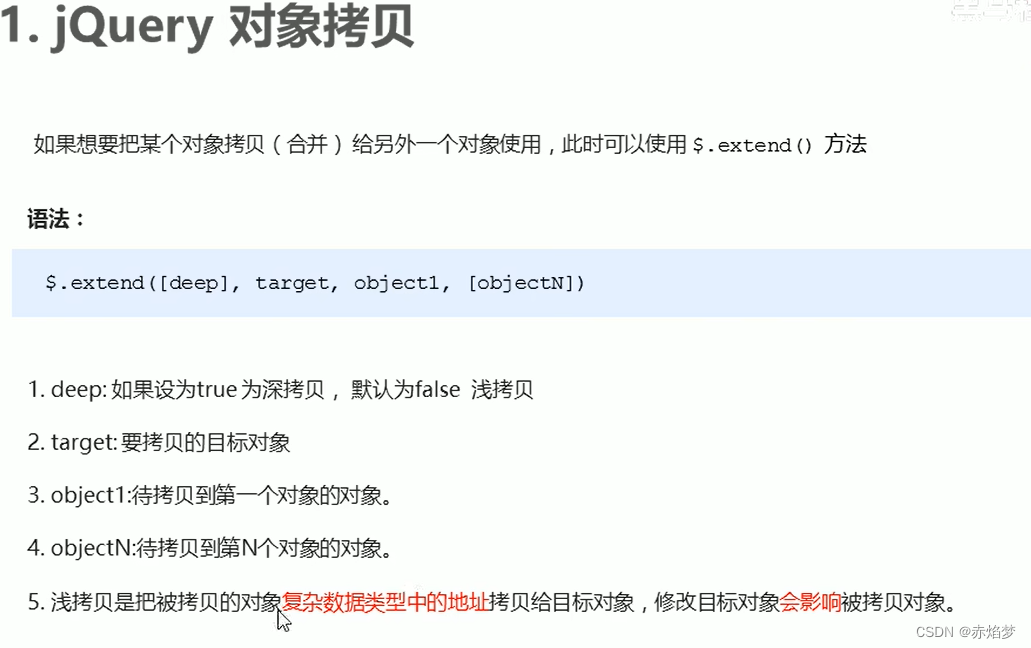
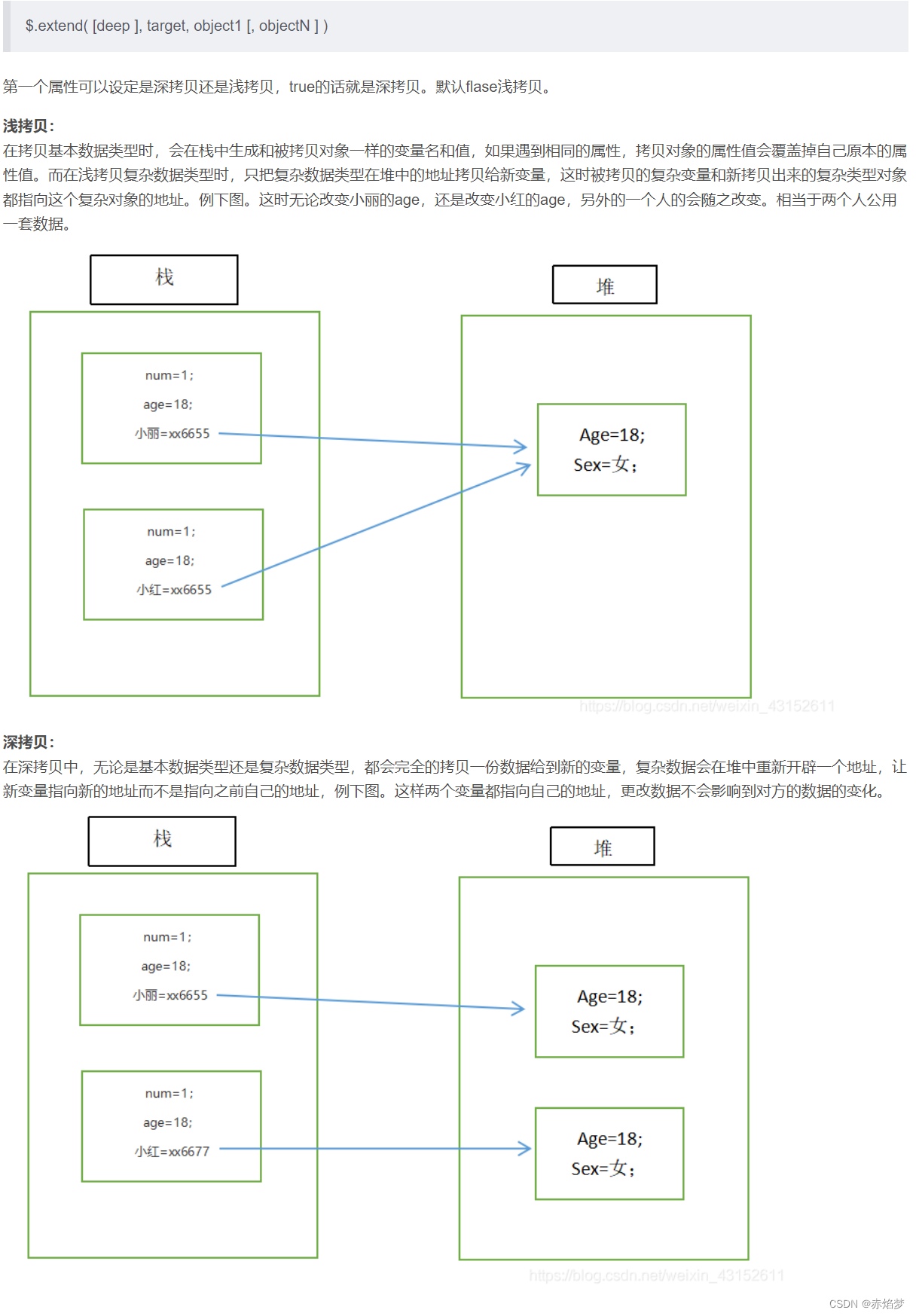
<script src="jQuery.min.js"></script>
<script>
$(function(){
// var targetObj={};
// var obj={
// id:0,
// name:"xinyi",
// location:"henan"
// };
// //覆盖以前的相同key值对应的数据
// $.extend(targetObj,obj);
// console.log(targetObj);
var targetObj={};
var obj={
id:0,
name:"xinyi",
location:"henan",
msg:{
age:18,
}
};
//覆盖以前的相同key值对应的数据
$.extend(targetObj,obj);
console.log(targetObj);
})
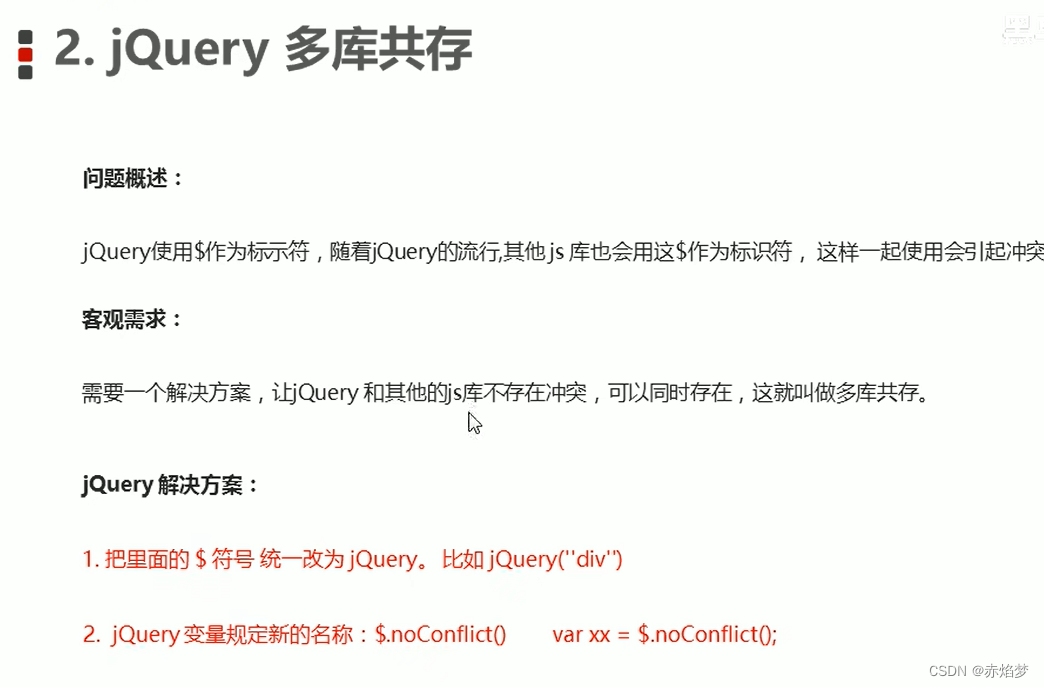
绑定多个事件:
<style>
div{
margin:200px auto;
width: 200px;
height: 200px;
background-color: pink;
}
.current{
background-color: purple;
}
</style>
<body>
<div></div>
</body>
<script src="jQuery.min.js"></script>
<script>
$("div").on("mouseenter mouseleave",function(){
$("div").toggleClass("current")
})
</script>
事件委托和动态绑定以及解除绑定
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<style>
ul li {
list-style: none;
cursor: pointer;
}
</style>
<script src="jQuery.min.js"></script>
<body>
<div></div>
<ul>
<li>1</li>
<li>2</li>
<li>3</li>
<li>4</li>
<li>5</li>
</ul>
<ol></ol>
<p>世界我来了</p>
</body>
<script>
$("ul").on("click", "li", function () {
alert("11");
});
$("ol").on("click", "li", function () {
alert("你好");
});
var li = $("<li>后来</li>");
$("ol").append(li);
$("ol").off("click", "li");
$("p").one("click", function () {
alert("22");
});
</script>
</html>
微博发布案例:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<style>
body{
display: flex;
justify-content: center;
align-items:center ;
}
.box{
margin-top: 200px;
border:1px solid black;
width: 800px;
height: 400px;
}
textarea{
margin-top:50px;
width: 600px;
height: 200px;
}
span {
font-weight: 700;
font-size: 20px;
font-family: Arial, Helvetica, sans-serif;
}
button{
font-weight: 700;
font-size: 20px;
font-family: Arial, Helvetica, sans-serif;
}
li{
font-size: 20px;
list-style: none;
}
a{
font-size: 20px;
text-decoration: none;
color: black;
}
a:hover{
color:red;
}
</style>
<body>
<div class="box" id="weibo">
<span>微博</span>
<textarea name="" id="" cols="30" rows="10" class="txt"></textarea>
<button class="btn">发布</button>
<ul></ul>
</div>
</body>
<script src="jQuery.min.js"></script>
<script>
$(".btn").on("click",function() {
var li=$("<li></lil>");
li.html($(".txt").val()+"<a href='javascript:;'>删除</a>");
$("ul").prepend(li);
li.slideDown();
$(".txt").val("");
})
$("ul ").on("click", "a",function() {
$(this).parent().slideUp(function() {
$(this).remove();
})
})
</script>
</html>