信息验证遇到的问题Message Authentication
In the context of communications across a network, the following attacks can be identified.
泄密Disclosure
流量分析Traffic analysis
伪装Masquerade
Content modification
Sequence modification
Time modification
Source repudiation
Destination repudiation
Any message authentication or digital signature mechanism has two levels of functionality.
At the lower level, there must be some sort of function that produces an authenticator: a value to be used to authenticate a message.
This lower-level function is then used as a primitive in a higher-level authentication protocol that enables a receiver to verify the authenticity of a message.
Message Authentication Code (MAC)
An alternative authentication technique involves the use of a secret key to generate a small fixed-size block of data that is appended to the message.
This technique assumes that two communicating parties, say A and B, share a common secret key K. When A has a message to send to B, it calculates the MAC as a function of the message and the key:
MAC based on Hash functions: HMAC
Digital Signatures
The most important development from the work on public-key cryptography is the digital signature.
The digital signature provides a set of security capabilities that would be difficult to implement in any other way.
The message is authenticated both in terms of source and in terms of data integrity.
Digital Signature Algorithm (DSA)
S-DES
class SDES:
def __init__(self):
self.P8_table = [6, 3, 7, 4, 8, 5, 10, 9]
self.P10_table = [3, 5, 2, 7, 4, 10, 1, 9, 8, 6]
def P8(self, key):
k = key
return [k[i - 1] for i in self.P8_table]
def P10(self, key):
k = key
return [k[i - 1] for i in self.P10_table]
def Shift(self, value):
return value[1:5] + value[0:1] + value[6:10] + value[5:6]
def generate_subkeys(self, key):
key = self.P10(key)
left = self.Shift(key[:5])
right = self.Shift(key[5:])
subkey1 = self.P8(left + right)
left = self.Shift(left)
right = self.Shift(right)
left = self.Shift(left)
right = self.Shift(right)
subkey2 = self.P8(left + right)
return subkey1, subkey2
def IP(self, data):
return [data[i - 1] for i in [2, 6, 3, 1, 4, 8, 5, 7]]
def IP_inv(self, data):
return [data[i - 1] for i in [4, 1, 3, 5, 7, 2, 8, 6]]
def F(self, data, subkey):
right = data
right_expanded = [right[i - 1] for i in [4, 1, 2, 3, 2, 3, 4, 1]]
xor_result = [subkey[i] ^ int(right_expanded[i]) for i in range(8)]
sbox_result = self.sbox(xor_result)
return sbox_result
def F_K(self, data, subkey):
left = data[:4]
right = data[4:]
right_F = self.F(right, subkey)
xor_result = [int(left[i]) ^ int(right_F[i]) for i in range(4)]
return xor_result+right
def SW(self, data):
return data[4:]+data[0:4]
def sbox(self, data):
sbox1 = [
[1, 0, 3, 2],
[3, 2, 1, 0],
[0, 2, 1, 3],
[3, 1, 3, 2]
]
sbox2 = [
[0, 1, 2, 3],
[2, 0, 1, 3],
[3, 0, 1, 0],
[2, 1, 0, 3]
]
row = int(''.join([str(data[0]), str(data[3])]), 2)
col = int(''.join([str(data[1]), str(data[2])]), 2)
return [int(x) for x in bin(sbox1[row][col])[2:].zfill(2) + bin(sbox2[row][col])[2:].zfill(2)]
def encrypt(self, plaintext, key):
key1, key2 = self.generate_subkeys(key)
plaintext = self.IP(plaintext)
plaintext = self.F_K(plaintext, key1)
plaintext = plaintext[4:] + plaintext[:4]
plaintext = self.F_K(plaintext, key2)
return self.IP_inv(plaintext)
def decrypt(self, ciphertext, key):
key1, key2 = self.generate_subkeys(key)
ciphertext = self.IP(ciphertext)
ciphertext = self.F_K(ciphertext, key2)
ciphertext = ciphertext[4:] + ciphertext[:4]
ciphertext = self.F_K(ciphertext, key1)
return self.IP_inv(ciphertext)
def ascii_to_binary_8(plaintext):
binary_list = []
for char in plaintext:
ascii_value = ord(char)
# Convert ASCII to binary, removing '0b' prefix
binary_string = bin(ascii_value)[2:]
binary_string = '0' * (8 - len(binary_string)) + \
binary_string # Ensure 10-bit length
binary_list.append(binary_string)
return binary_list
def binary_to_ascii(binary_list):
plaintext = ""
for binary_string in binary_list:
binary = ''.join(str(bit) for bit in binary_string)
# 将二进制字符串转换为整数
ascii_value = int(binary, 2)
# 将整数转换为字符,并添加到明文字符串中
plaintext += chr(ascii_value)
return plaintext
# 示例
plaintext = ('Network security encompasses all the steps taken to protect the integrity of a computer network and the '
'data within it. Network security is important because it keeps sensitive data safe from cyber attacks '
'and ensures the network is usable and trustworthy. Successful network security strategies employ '
'multiple security solutions to protect users and organizations from malware and cyber attacks, '
'like distributed denial of service')
key = '1100011110'
key = [int(i) for i in key]
s = SDES()
plaintext_binary = ascii_to_binary_8(plaintext)
print("明文:", plaintext_binary)
ciphertext = []
for binary_str in plaintext_binary:
encrypted_text = s.encrypt(binary_str, key)
ciphertext.append(encrypted_text)
print("密文:", ciphertext)
decrypted_text = []
for encrypted_text in ciphertext:
decrypted_text.append(s.decrypt(encrypted_text, key))
print("解密后的明文(ASCII):", decrypted_text)
decrypted_text = binary_to_ascii(decrypted_text)
print("解密后的明文:", decrypted_text)
S-AES
公钥密码学Public-Key Cryptography
公钥密码学Public-Key Cryptography-CSDN博客
对称密钥分配Symmetric Key Distribution
Symmetric Encryption
For two parties A and B, key distribution can be achieved in a number of ways, as follows:
- A can select a key and physically deliver it to B.
- If A and B have previously and recently used a key, one party can transmit the new key to the other, encrypted using the old key.
- A third party can select the key and physically deliver it to A and B.
- If A and B each has an encrypted connection to a third party C, C can deliver a key on the encrypted links to A and B.
KTC(A key translation center)
Transfer symmetric keys for future communication between two entities, at least one of whom has the ability to generate or acquire symmetric keys by themselves. Entity A generates or acquires a symmetric key to be used as a session key for communication with B. A encrypts the key using the master key it shares with the KTC and sends the encrypted key to the KTC. The KTC decrypts the session key, re-encrypts the session key in the master key it shares with B, and either sends that re-encrypted session key to A (Figure a) for A to forward to B or sends it directly to B (Figure b).
KDC(A key distribution center)
Generate and distribute session keys. Entity A sends a request to the KDC for a symmetric key to be used as a session key for communication with B. KDC generates a symmetric session key, and then encrypts the session key with the master key it shares with A and sends it to A. The KDC also encrypts the session key with the master key is shares with B and sends it to B (Figure c). Alternatively, KDC sends both encrypted key values to A, and A forwards the session key encrypted with the master key shared by the KDC and B to B (Figure d).
KDC vs KTC
kdc的密钥是第三方分发,ktc是用户自己产生密钥
非对称加密Asymmetric Encryption
If A wishes to communicate with B, the following procedure is employed:
- A generates a public/private key pair {PUa, PRa} and transmits a message to B consisting of PUa and an identifier of A, IDA.
- B generates a secret key, Ks, and transmits it to A, which is encrypted with A’s public key.
- A computes D(PRa, E(PUa, Ks)) to recover the secret key. Because only A can decrypt the message, only A and B will know the identity of Ks.
- A discards PUa and PRa and B discards PUa.
Secret Key Distribution with Confidentiality and Authentication
challenge and response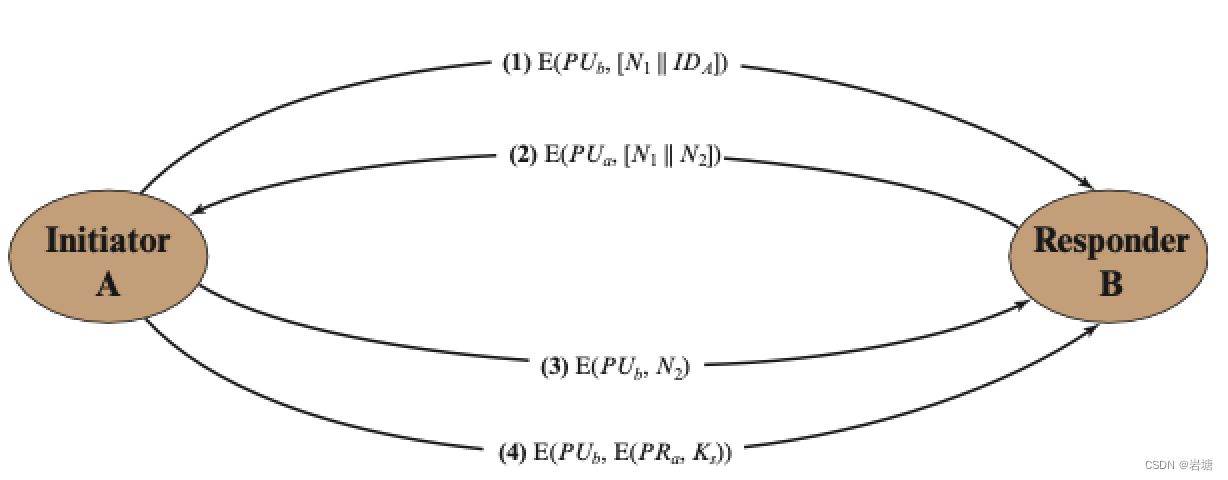
Distribution of Public Keys
Several techniques have been proposed for the distribution of public keys Public announcement Publicly available directory Public-key authority Public-key certificates
Public Announcement
The point of public-key encryption is that the public key is public. Thus, if there is some broadly accepted public-key algorithm, such as RSA, any participant can send his or her public key to any other participant or broadcast the key to the community. Anyone can forge such a public announcement.
Publicly Available Directory
A greater degree of security can be achieved by maintaining a publicly available dynamic directory of public keys. Maintenance and distribution of the public directory would have to be the responsibility of some trusted entity or organization.
- The authority maintains a directory with a {name, public key} entry for each participant.
- Each participant registers a public key with the directory authority. Registration would have to be in person or by some form of secure authenticated communication.
- A participant may replace the existing key with a new one at any time, either because of the desire to replace a public key that has already been used for a large amount of data, or because the corresponding private key has been compromised in some way.
- Participants could also access the directory electronically. For this purpose, secure, authenticated communication from the authority to the participant is mandatory.
Public-Key Authority
Stronger security for public-key distribution can be achieved by providing tighter control over the distribution of public keys from the directory.
Assume that a central authority maintains a dynamic directory of public keys of all participants.
Each participant reliably knows a public key for the authority, with only the authority knowing the corresponding private key.
Step 6, Step 7: Challenge and Response
- A total of seven messages are required.
- However, the initial five messages need to be used only infrequently because both A and B can save the other’s public key for future use A technique known as caching
- Periodically, a user should request fresh copies of the public keys to ensure currency.
- The public-key authority could be somewhat of a bottleneck in the system.
公钥证书Public-Key Certificates
Certificates can be used by participants to exchange keys without contacting a public-key authority.
A certificate consists of a public key, an identifier of the key owner, and the whole block signed by a trusted third party.
The third party is a certificate authority, such as a government agency or a financial institution, that is trusted by the user community.
Requirements
- Any participant can read a certificate to determine the name and public key of the certificate’s owner.
- Any participant can verify that the certificate originated from the certificate authority and is not counterfeit.
- Only the certificate authority can create and update certificates.
- 任何参与者都可以验证证书的时间有效性。 Any participant can verify the time validity of the certificate.
X.509 Certificates
一个证书的标准
X.509 has become universally accepted for formatting public-key certificates. X.509 certificates are used in most network security applications, including IP security, transport layer (TLS), and S/MIME. X.509 was initially issued in 1988. The standard was subsequently revised in 1993 to address some of the security. The standard is currently at edition eight, issued in 2016.
User Certificates generated by a CA have the following characteristics: Any user with access to the public key of the CA can verify the user public key that was certified. No party other than the CA can modify the certificate without this being detected. Because certificates are unforgeable, they can be placed in a directory without the need for the directory to make special efforts to protect them. A user can transmit his certificate directly to other users.
With many users, it may be more practical for there to be a number of CAs, each of which securely provides its public key to some fraction of the users. Now suppose that A has obtained a certificate from certification authority X1 and B has obtained a certificate from CA X2. If A does not securely know the public key of X2, then B’s certificate, issued by X2, is useless to A. A can read B’s certificate, but A cannot verify the signature.
If the two CAs have securely exchanged their own public keys, the following procedure will enable A to obtain B’s public key.
Step 1: A obtains from the directory the certificate of X2 signed by X1. Because A securely knows X1’s public key, A can obtain X2’s public key from its certificate and verify it by means of X1’s signature on the certificate.
Step 2: A then goes back to the directory and obtains the certificate of B signed by X2. Because A now has a trusted copy of X2’s public key, A can verify the signature and securely obtain B’s public key.