1、在index.html添加超链接,添加新库存add.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<link rel="stylesheet" href="style/index.css">
<script src="script/axios.min.js"></script>
<script src="script/index.js"></script>
<script src="script/common.js"></script>
</head>
<body>
<div id="div0">
<div id="div_title">
<p>欢迎使用水果库存系统</p>
<div style="float: right;border: 0px solid red;margin-right:8%;margin-bottom: 4px">
<a href="add.html" style="text-decoration: none">添加新库存</a>
</div>
</div>
<div id="div_fruit_table">
<table id="fruit_tbl">
<tr>
<th class="w25">名称</th>
<th class="w25">单价</th>
<th class="w25">库存</th>
<th>操作</th>
</tr>
<!--
<tr>
<td><a href='edit.html?fid=1'>苹果</a></td>
<td>5</td>
<td>100</td>
<td><img class="delImg" src="imgs/del.png" onclick='delFruit(1)'/></td>
</tr>
-->
</table>
</div>
</div>
</body>
</html>
2、编写add.html添加点击事件负责提交onclick
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<link rel="stylesheet" href="style/index.css">
<script src="script/axios.min.js"></script>
<script src="script/add.js"></script>
<script src="script/common.js"></script>
</head>
<body>
<div id="div0">
<div id="div_title">
<p>添加水果库存信息</p>
</div>
<div id="div_fruit_table">
<table id="fruit_tbl">
<tr>
<th class="w25">名称:</th>
<td><input type="text" id="fname" onkeyup="checkFname(this.value)"/><span id="fnameSpan"> </span></td>
</tr>
<tr>
<th class="w25">单价:</th>
<td><input type="text" name="price" id="price"/> </td>
</tr>
<tr>
<th class="w25">库存:</th>
<td><input type="text" name="fcount" id="fcount"/> </td>
</tr>
<tr>
<th class="w25">备注:</th>
<td><input type="text" name="remark" id="remark"/> </td>
</tr>
<tr>
<th colspan="2">
<input type="button" value="添加" onclick="add()"/>
<input type="button" value="取消"/>
</th>
</tr>
</table>
</div>
</div>
</body>
</html>
3、编写add.js
3.1、common.js
function $(key){
if(key){
if(key.startsWith("#")){
key = key.substring(1)
return document.getElementById(key)
}else{
let nodeList = document.getElementsByName(key)
return Array.from(nodeList)
}
}
}
function add(){
let fname = $("#fname").value
let price = $("#price").value
let fcount = $("#fcount").value
let remark = $("#remark").value
//let fruit = {"fname":fname,"price":price,"fcount":fcount,"remark":remark}
let fruit = {}
fruit.fname=fname
fruit.price = price
fruit.fcount=fcount
fruit.remark=remark
axios({
method:'post',
url:'add',
data:fruit
}).then(response=>{
if(response.data.flag){
window.location.href='index.html'
}
})
}
/*
function checkFname(fname){
axios({
method:'get',
url:"getFname",
params:{
fname:fname
}
}).then(response=>{
let fnameSpan= $("#fnameSpan");
if(response.data.flag){
fnameSpan.innerText = '名称可添加'
fnameSpan.style.color='green'
}else{
fnameSpan.innerText = '名称已存在'
fnameSpan.style.color='red'
}
})
}*/
4、编写Controller层AddServlet
package com.csdn.fruit.servlet;
import com.csdn.fruit.dao.FruitDao;
import com.csdn.fruit.dao.impl.FruitDaoImpl;
import com.csdn.fruit.dto.Result;
import com.csdn.fruit.pojo.Fruit;
import com.csdn.fruit.util.RequestUtil;
import com.csdn.fruit.util.ResponseUtil;
import jakarta.servlet.ServletException;
import jakarta.servlet.annotation.WebServlet;
import jakarta.servlet.http.HttpServlet;
import jakarta.servlet.http.HttpServletRequest;
import jakarta.servlet.http.HttpServletResponse;
import java.io.IOException;
@WebServlet("/add")
public class AddServlet extends HttpServlet {
private FruitDao fruitDao = new FruitDaoImpl();
@Override
protected void service(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
Fruit fruit = (Fruit) RequestUtil.readObject(req, Fruit.class);
fruitDao.addFruit(fruit);
ResponseUtil.print(resp, Result.OK());
}
}
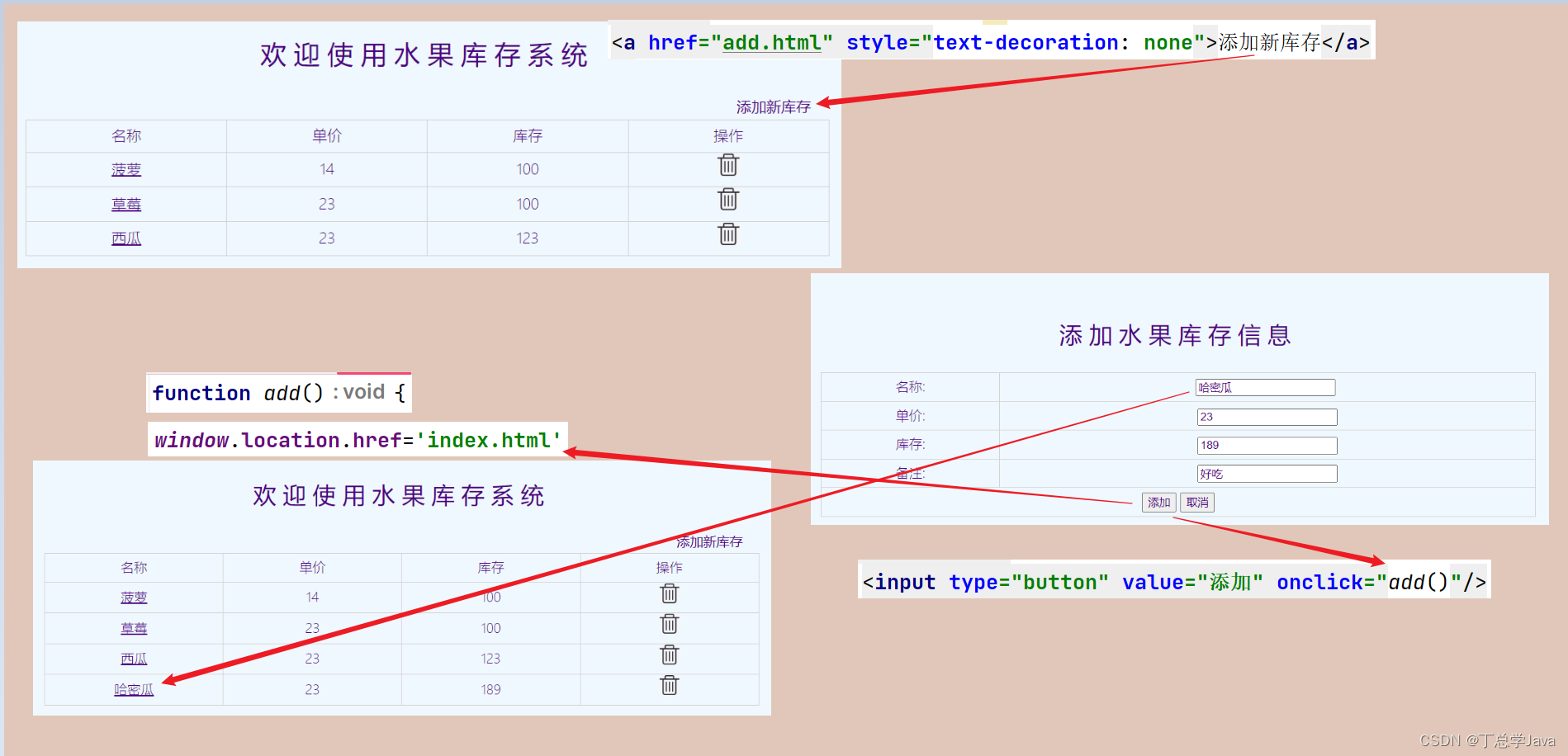