本次操作是将达梦数据库的数据插入到MySQL数据库中。
准备工作
- 创建一个 Spring Boot 项目
- 添加依赖
- 配置数据源属性
POM文件
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.32</version>
</dependency>
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>dynamic-datasource-spring-boot-starter</artifactId>
<version>3.5.2</version>
</dependency>
<!-- 添加dm8 jdbc jar 包依赖-->
<dependency>
<groupId>com.dm</groupId>
<artifactId>DmJdbcDriver</artifactId>
<version>1.8.0</version>
</dependency>
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.5.3.2</version>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
YML文件配置多数据源
spring:
datasource:
dynamic:
druid:
initial-size: 5
min-idle: 5
maxActive: 20
# 配置获取连接等待超时的时间
maxWait: 60000
# 配置间隔多久才进行一次检测,检测需要关闭的空闲连接,单位是毫秒
timeBetweenEvictionRunsMillis: 6000
# 配置一个连接在池中最小生存的时间,单位是毫秒
minEvictableIdleTimeMillis: 60000
# 配置一个连接在池中最大生存的时间,单位是毫秒
maxEvictableIdleTimeMillis: 900000
validationQuery: SELECT 1 FROM DUAL
testWhileIdle: true
testOnBorrow: false
testOnReturn: false
# 打开PSCache,并且指定每个连接上PSCache的大小
poolPreparedStatements: true
maxPoolPreparedStatementPerConnectionSize: 20
primary: dm #设置默认的数据源或者数据源组,默认值即为dm
strict: false #严格匹配数据源,默认false. true未匹配到指定数据源时抛异常,false使用默认数据源
datasource:
dm:
driver-class-name: dm.jdbc.driver.DmDriver
url: jdbc:dm://localhost:5236
username: xxxx
password: xxxxxxxxx
mysql:
driver-class-name: com.mysql.cj.jdbc.Driver # 3.2.0开始支持SPI可省略此配置
url: jdbc:mysql://localhost:3306/lps?serverTimezone=GMT%2B8&useUnicode=true&characterEncoding=utf-8&useSSL=false&allowMultiQueries=true&allowPublicKeyRetrieval=true
username: xxxxx
password: xxxxxxxxxxx
实体类
@Data
public class CfdData implements Serializable {
/**
*
*/
@TableField(value = "TIME")
private LocalDateTime time;
/**
*
*/
@TableField(value = "VAL")
private String val;
@TableField(exist = false)
private static final long serialVersionUID = 1L;
}
DAO层
@Mapper
public interface CfdDataMapper extends BaseMapper<CfdData> {
void insertForMysql(CfdData cfdData);
Long selectCountFromMysql();
List<CfdData> selectFromDm();
}
XML映射层(达梦的SQL语句需要带上模式名称,而MySQL不需要)
<insert id="insertForMysql">
INSERT INTO CFD_DATA (TIME, VAL)
VALUES (#{time}, #{val});
</insert>
<select id="selectFromDm" resultType="com.lp.domain.CfdData">
SELECT * FROM LPS.CFD_DATA
</select>
<select id="selectCountFromMysql" resultType="java.lang.Long">
SELECT COUNT(1) FROM CFD_DATA
</select>
Service接口层
/**
* @author 阿水
* @ClassName CfdDataMySql
* @description: TODO
* @date 2023年10月21日
* @version: 1.0
*/
public interface CfdDataMySqlService extends IService<CfdData> {
Long insertForMysql(List<CfdData> cfdData);
Long selectCountFromMysql();
}
/**
* @author 19449
* @description 针对表【CFD_DATA】的数据库操作Service
* @createDate 2023-10-21 23:32:05
*/
public interface CfdDataService extends IService<CfdData> {
List<CfdData> selectFromDm();
}
Service实现类(使用ds注解来选择数据源)
@Service
@DS("mysql")
public class CfdDataMySqlServiceImpl extends ServiceImpl<CfdDataMapper, CfdData>
implements CfdDataMySqlService {
@Resource
private SqlSessionFactory sqlSessionFactory;
@Resource
private CfdDataMapper cfdDataMapper;
@Override
public Long insertForMysql(List<CfdData> cfdData) {
SqlSession session = sqlSessionFactory.openSession(ExecutorType.BATCH);
try{
CfdDataMapper mapper = session.getMapper(CfdDataMapper.class);
cfdData.forEach(item->{
mapper.insertForMysql(item);
});
session.commit();
}
finally {
session.close();
}
return (long) cfdData.size();
}
@Override
public Long selectCountFromMysql() {
return cfdDataMapper.selectCountFromMysql();
}
}
/**
* @author 19449
* @description 针对表【CFD_DATA】的数据库操作Service实现
* @createDate 2023-10-21 23:32:05
*/
@Service
@DS("dm")
public class CfdDataServiceImpl extends ServiceImpl<CfdDataMapper, CfdData>
implements CfdDataService {
@Autowired
private CfdDataMapper cfdDataMapper;
@Override
public List<CfdData> selectFromDm() {
return cfdDataMapper.selectFromDm();
}
}
测试代码
@SpringBootTest
class JdbcTemplateDemo1ApplicationTests {
//达梦数据库
@Resource
CfdDataService dmService;
//mysql数据库
@Resource
CfdDataMySqlService mySqlService;
@Test
void contextLoads() {
List<CfdData> cfdData = dmService.selectFromDm();
for (CfdData cfdDatum : cfdData) {
System.out.println(cfdDatum);
}
System.out.println("本次DM数据数据条数为"+cfdData.size()+"条");
Long countFromMysql = mySqlService.selectCountFromMysql();
System.out.println("mysql当前条数为"+countFromMysql+"条");
System.out.println("开始插入");
mySqlService.insertForMysql(cfdData);
System.out.println("插入完成");
Long countAfterInsert = mySqlService.selectCountFromMysql();
System.out.println("mysql插入完成后当前条数为"+countAfterInsert+"条");
Long count = mySqlService.getBaseMapper().selectCount(null);
/**
* 这个走的是默认
* primary: dm #设置默认的数据源或者数据源组,默认值即为dm
*/
System.out.println("mybatisplus查询出来的count为"+count+"条");
}
}
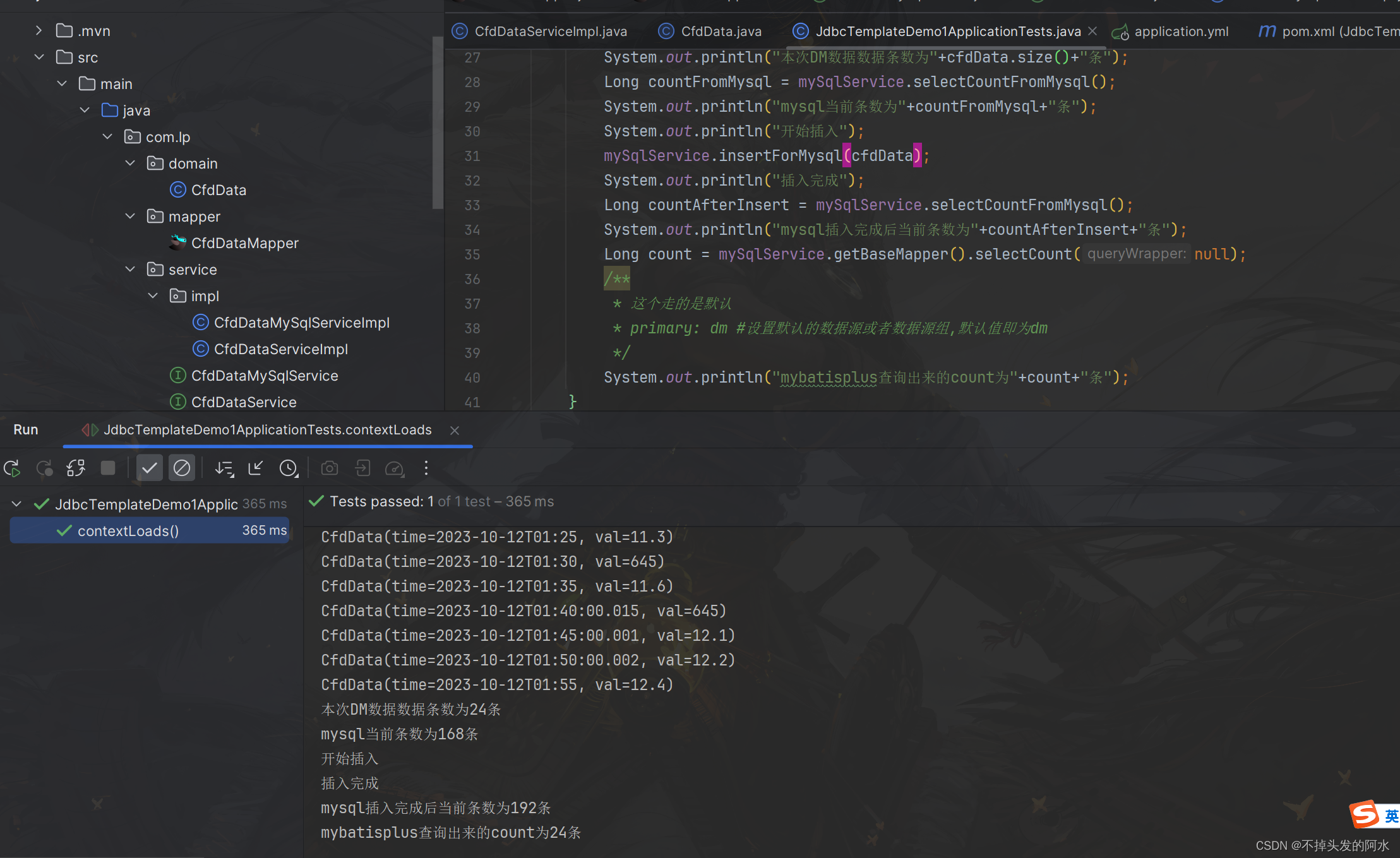
整体结构如下
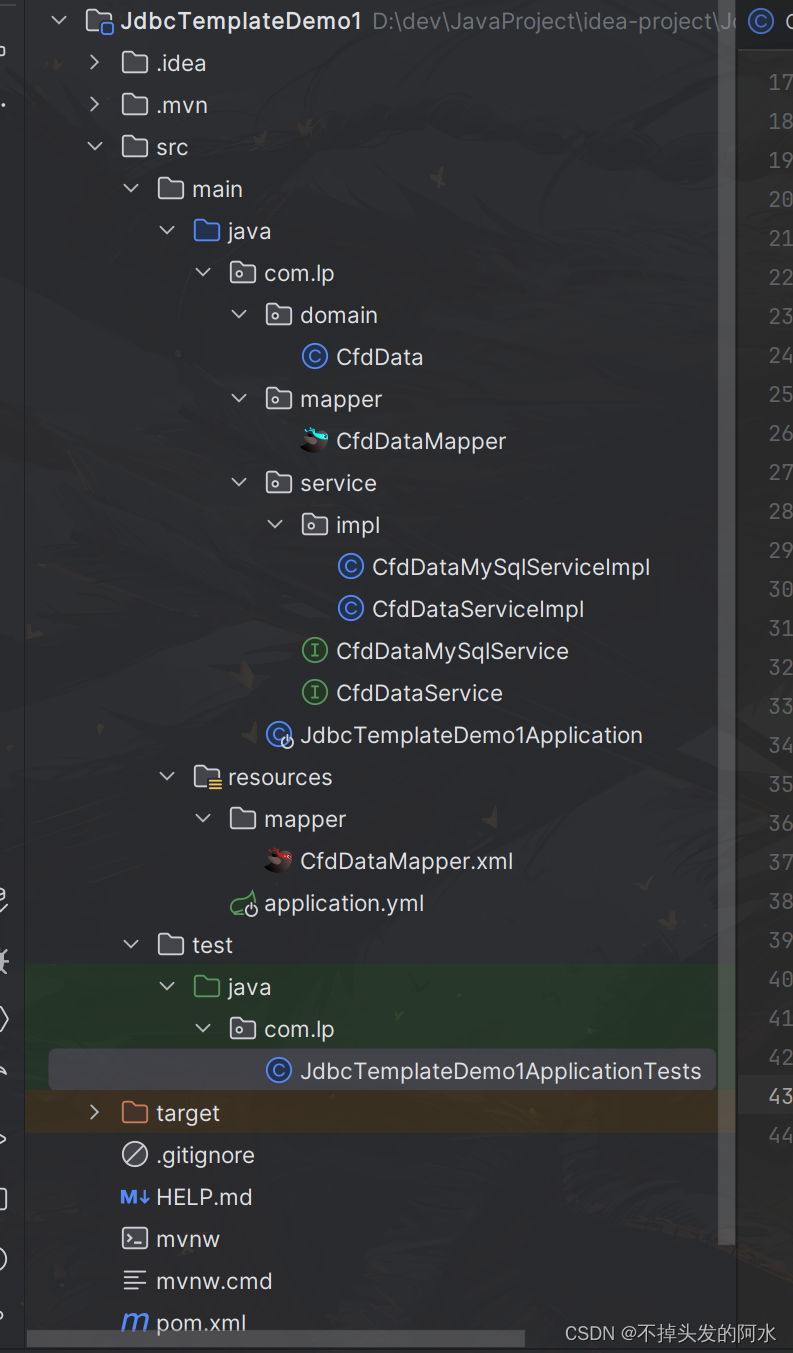
结论以及注意事项:不指定的话会走默认数据源
注解 | 结果 |
---|
没有@DS | 默认数据源 |
@DS("dsName") | dsName可以为组名也可以为具体某个库的名称 |