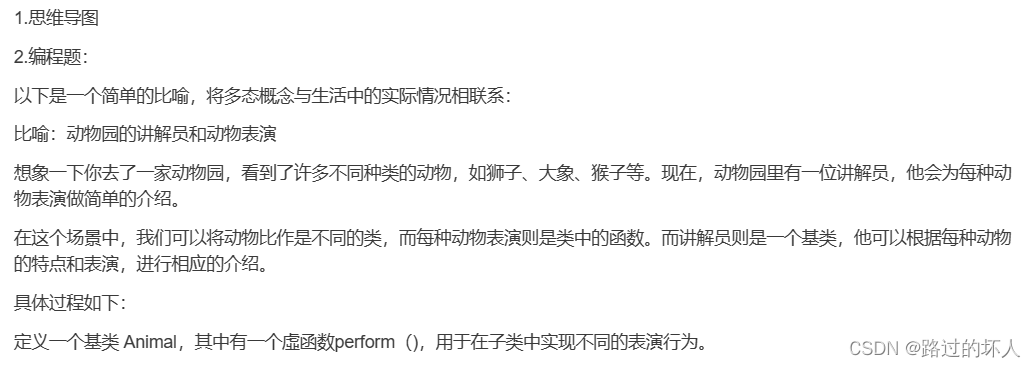
#include <iostream>
using namespace std;
//定义动物类
class Animal
{
private:
string name;
public:
Animal(){}
Animal(string name):name(name){}
~Animal(){}
//定义虚函数
virtual void perform()=0;
//表演的节目
void show()
{
cout << "Please enjoy the special performance of " << name << endl;
}
};
//管理员
class Guide:public Animal
{
public:
Guide(){}
Guide(string name):Animal(name){}
};
//表演
class Perform:public Guide
{
public:
Perform(){}
Perform(string name):Guide(name){}
//重写虚函数
void perform()
{
Animal::show();
}
};
int main()
{
Perform a("elephants");
Perform b("lions");
Perform c("tigers");
Perform d("crocodiles");
Perform e("hippos");
Perform f("camels");
Perform g("peacocks");
Guide *p;
p=&a;
p->perform();
p=&b;
p->perform();
p=&c;
p->perform();
p=&d;
p->perform();
p=&e;
p->perform();
p=&f;
p->perform();
p=&g;
p->perform();
return 0;
}
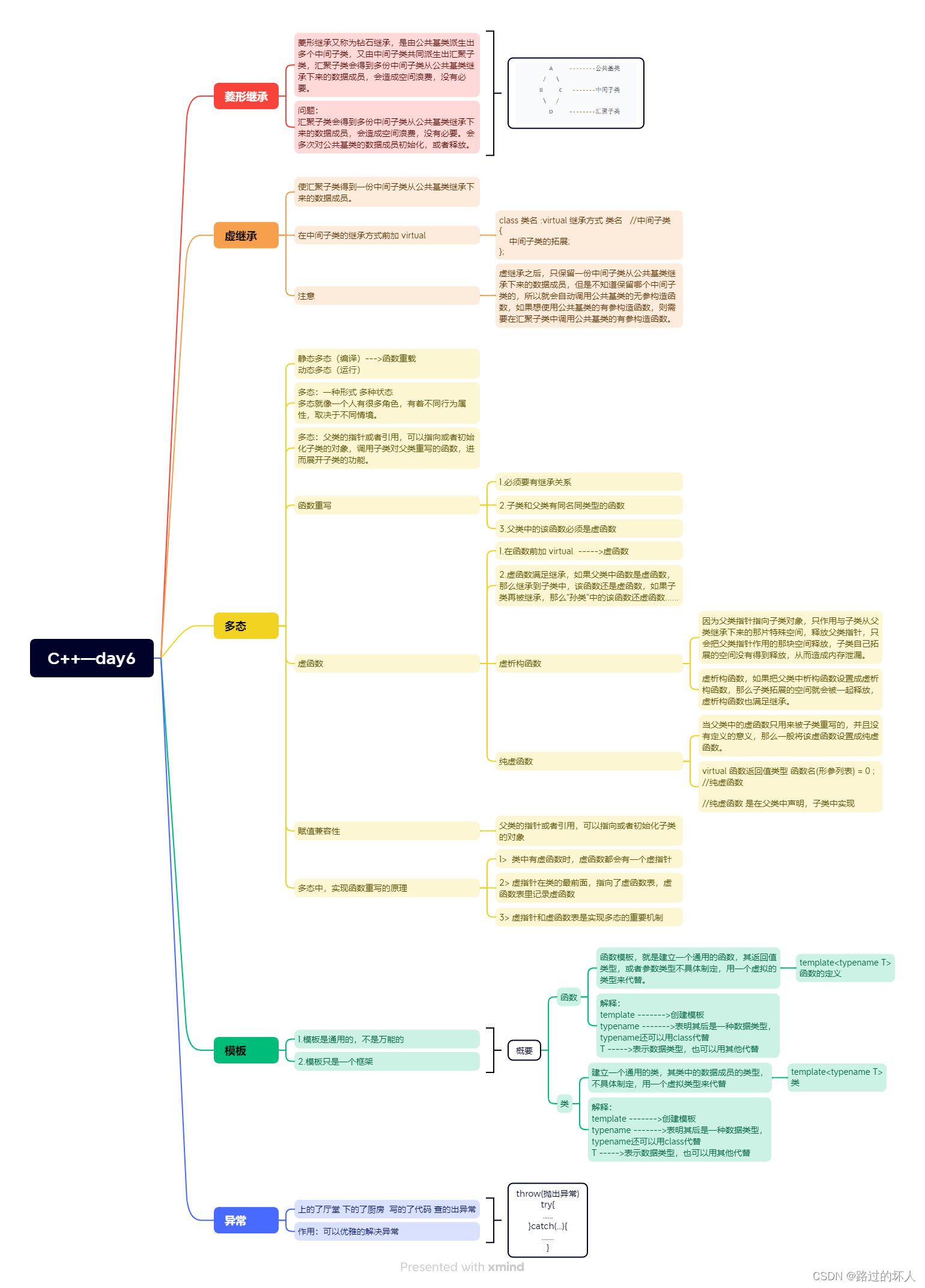