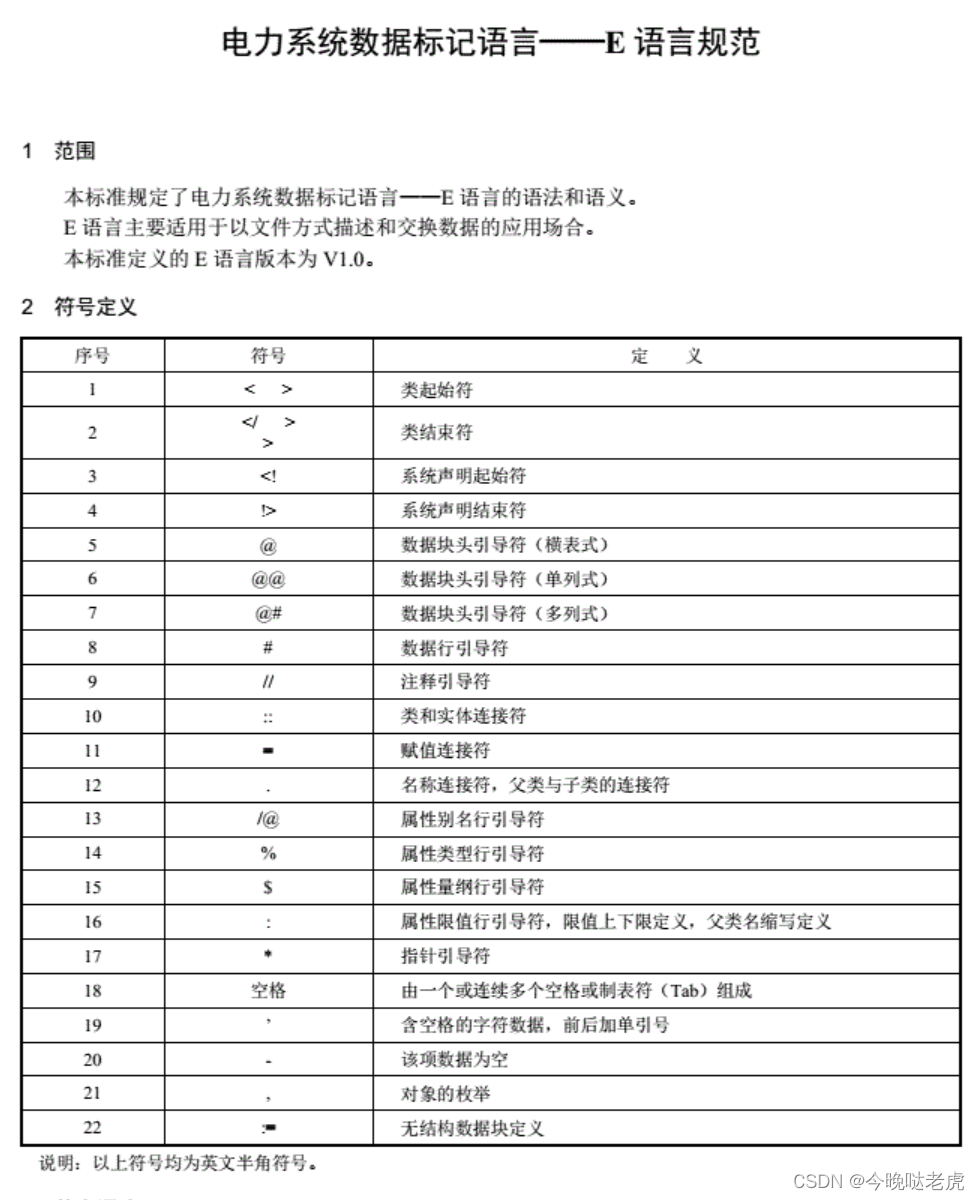
import lombok.extern.slf4j.Slf4j;
import java.io.*;
import java.nio.charset.StandardCharsets;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
/**
* @Description E文件工具类
*/
@Slf4j
public class EFileUtils {
/**
* 读字符串
* @param text 内容
* @param node 节点
* @return
*/
public static List<List<String>> readText(String text, String node) {
try {
int startIndex = 0;
int thisIndex = 0;
int endIndex = 0;
boolean flag = false;
text = text.substring(text.indexOf("<" + node), text.indexOf("</" + node) + node.length() + 2);
String[] lines = text.split("\\r?\\n");
List<List<String>> listDatas = new ArrayList<List<String>>();
for (int i = 0; i < lines.length; i++) {
if (flag) break;
thisIndex++;
if (lines[i].startsWith("<" + node)) {
startIndex = thisIndex;
} else if (lines[i].startsWith("</" + node)) {
endIndex = thisIndex;
flag = true;
} else if (startIndex != 0) {
String[] split = lines[i].split("\\s+");
List<String> lineDatas = new ArrayList<String>(Arrays.asList(split));
lineDatas.remove(0);//
listDatas.add(lineDatas);
}
}
log.info(node + "节点标签在第" + startIndex + "-" + endIndex);
return listDatas;
} catch (Exception e) {
log.error(e.getMessage());
}
return null;
}
/**
* 读文件
* path 读取的文件路径
* node E文件里的节点名称
*/
public static List<List<String>> readEFile(String path, String node) {
try {
int startIndex = 0;
int thisIndex = 0;
int endIndex = 0;
boolean flag = false;
//文件内容的字符集 UTF8
BufferedReader reader = new BufferedReader(new InputStreamReader(new FileInputStream(path), StandardCharsets.UTF_8));
String line = reader.readLine();
List<List<String>> listDatas = new ArrayList<List<String>>();
while ((line = reader.readLine()) != null && !flag) {
thisIndex++;
if (line.startsWith("<" + node)) {
startIndex = thisIndex;
} else if (line.startsWith("</" + node)) {
endIndex = thisIndex;
flag = true;
} else if (startIndex != 0) {
String[] split = line.split("\\s+");
List<String> lineDatas = new ArrayList<String>(Arrays.asList(split));
lineDatas.remove(0);//
listDatas.add(lineDatas);
}
}
log.info(node + "节点标签在第" + startIndex + "-" + endIndex);
reader.close();
return listDatas;
} catch (IOException e) {
log.error(e.getMessage());
}
return null;
}
/**
* 写文件
*
* @param txtInfo 内容
* @param filePath 文件路径
* @throws IOException
*/
public static void writeTxt(String txtInfo, String filePath) throws IOException {
File file = new File(filePath);
if (!file.exists()) {
file.getParentFile().mkdirs();
}
file.createNewFile();
// write 解决中文乱码问题
// FileWriter fw = new FileWriter(file, true);
// 写入文件的字符集 GBK 看需求而设定
OutputStreamWriter fw = new OutputStreamWriter(new FileOutputStream(file), StandardCharsets.UTF_8);
BufferedWriter bw = new BufferedWriter(fw);
bw.write(txtInfo);
bw.flush();
bw.close();
fw.close();
}
}