在Qt中,QGraphicsEffect有四个子类,分别为QGraphicsBlurEffect, QGraphicsColorizeEffect, QGraphicsDropShadowEffect, and QGraphicsOpacityEffect,用于实现模糊、着色、阴影、透明度功能
下面就是对这四种功能以及效果做测试
1.QGraphicsBlurEffect,将图片或窗口模糊化,呈现失焦效果
代码如下
QFrame* pTestFrame = new QFrame(this);
pTestFrame->setFixedSize(QSize(200, 200));
pTestFrame->move(100, 110);
QGraphicsBlurEffect* blurEffect = new QGraphicsBlurEffect;
blurEffect->setBlurHints(QGraphicsBlurEffect::QualityHint);
blurEffect->setBlurRadius(2);
pTestFrame->setAutoFillBackground(true);
QPalette palette;
palette.setBrush(QPalette::Background, QBrush(QColor(255,255,255, 40)));
pTestFrame->setPalette(palette);
QGraphicsEffect* oldEffect = pTestFrame->graphicsEffect();
pTestFrame->setGraphicsEffect(blurEffect);
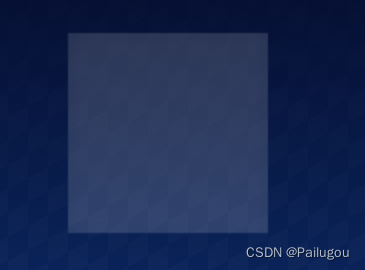
针对于setBlurHints函数主要用于模糊性能参数的设置
QGraphicsBlurEffect::PerformanceHint
QGraphicsBlurEffect::QualityHint
QGraphicsBlurEffect::AnimationHint
2.QGraphicsColorizeEffect,图片或窗口、控件着色
代码如下
QFrame* pTestFrame = new QFrame(this);
pTestFrame->setFixedSize(QSize(200, 200));
pTestFrame->move(100, 110);
pTestFrame->setAutoFillBackground(true);
auto blur = new QGraphicsColorizeEffect(this);
blur->setStrength(0.5);
blur->setColor(Qt::green);
QPalette palette;
palette.setBrush(QPalette::Background, QBrush(QColor(255, 255, 255, 40)));
pTestFrame->setPalette(palette);
auto oldEffect = pTestFrame->graphicsEffect();
pTestFrame ->setGraphicsEffect(blur);
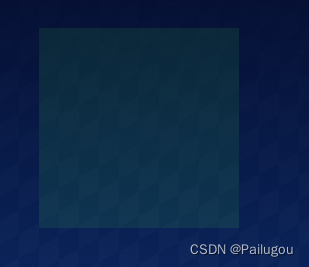
QGraphicsDropShadowEffect,图片或窗口、控件设置阴影
代码如下
QFrame* pTestFrame = new QFrame(this);
pTestFrame->setFixedSize(QSize(200, 200));
pTestFrame->move(100, 110);
pTestFrame->setAutoFillBackground(true);
QGraphicsDropShadowEffect* shadoweffect = new QGraphicsDropShadowEffect(this);
shadoweffect->setOffset(5,5);
shadoweffect->setColor(Qt::green);
shadoweffect->setBlurRadius(10);
pTestFrame->setGraphicsEffect(shadoweffect);
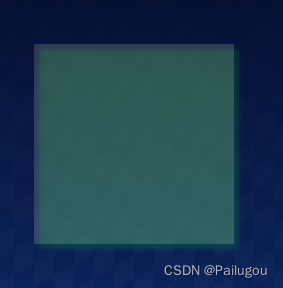
4.QGraphicsOpacityEffect,图片或窗口、控件设置透明度
代码如下
QFrame* pTestFrame = new QFrame(this);
pTestFrame->setFixedSize(QSize(200, 200));
pTestFrame->move(100, 110);
pTestFrame->setAutoFillBackground(true);
auto rect = pTestFrame ->rect();
QLinearGradient alphaGradient(rect.topLeft(), rect.bottomLeft());
alphaGradient.setColorAt(0.0, Qt::transparent);
alphaGradient.setColorAt(0.5, Qt::black);
alphaGradient.setColorAt(1.0, Qt::transparent);
auto blur = new QGraphicsOpacityEffect(this);
blur->setOpacity(0.5);
blur->setOpacityMask(alphaGradient);
QPalette palette;
palette.setBrush(QPalette::Background, QBrush(QColor(255, 255, 255, 40)));
pTestFrame->setPalette(palette);
pTestFrame ->setGraphicsEffect(blur);
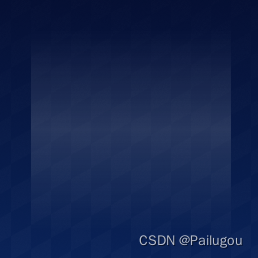