统一接口返回
前后端分离项目,通常后端会返回给前端统一的数据格式,一般包括code,msg,data信息。
创建返回统一实体类
package com.example.exceptionspring.domain;
import lombok.Data;
@Data
public class Result {
private Integer code;
private String msg;
public Object data;
public Result(Integer code, String msg) {
this.code = code;
this.msg = msg;
}
public Result(ErrorEnum error) {
this.code=error.getCode();
this.msg=error.getMsg();
}
public Result() {
}
}
创建枚举类,定义code和msg信息
package com.example.exceptionspring.domain;
public enum ErrorEnum {
SUCCESS(200,"操作成功"),
NOT_AUTH(401,"没有权限"),
NOT_FOUND(404,"找不到资源"),
ERROR(500,"系统错误");
private Integer code;
public String msg;
ErrorEnum(Integer code,String msg) {
this.code=code;
this.msg=msg;
}
public Integer getCode() {
return code;
}
public void setCode(Integer code) {
this.code = code;
}
public String getMsg() {
return msg;
}
public void setMsg(String msg) {
this.msg = msg;
}
}
返回测试
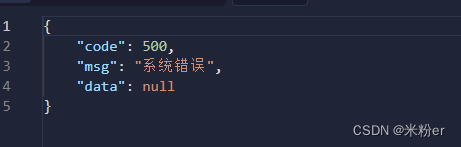
全局异常处理
Exception异常在开发中难免会碰到,使用之前的处理方法try{}catch{}
会造成大量的代码重复,每个接口都需要try{}catch{}
,代码会变得臃肿,SpringBoot为解决这一问题,推出了全局异常处理,通过注解@RestControllerAdvice
和 @ExceptionHandler
很好的进行控制。
本篇文章通过在Controller层和Service层演示异常的处理方式。
Controller层演示模拟java经典异常int i=i/0;
不使用数据库模拟异常
- 1.新建一个SpringBoot项目,创建项目的目录结构
- 2.新建全局异常处理类
GlobalException.java
package com.example.exceptionspring.exception;
import com.example.exceptionspring.domain.ErrorEnum;
import com.example.exceptionspring.domain.Result;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.RestControllerAdvice;
@RestControllerAdvice
public class GlobalException {
@ExceptionHandler(value = Exception.class)
public Result handleException(Exception e){
return new Result(ErrorEnum.ERROR);
}
}
@RestControllerAdvice注解是@ResponseBody+@ControllerAdvice注解,如果在该类使用注解,则方法上不需要添加@ResponseBody ,如果在类上使用@ControllerAdvice注解,则需在方法上添加@ResponseBody注解。
@ExceptionHandler value属性值为具体的异常类型,Exception.class为最顶级异常,所有的异常都继承Exception.class。
- 3.编写测试接口模拟异常
package com.example.exceptionspring.controller;
import com.example.exceptionspring.domain.Result;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class StudentController {
@GetMapping("/getException")
public Result getException(){
int i=1/0;
return new Result();
}
}
Service层演示通过MySQL数据库模拟异常
-
- 配置数据库连接参数
spring:
datasource:
driver-class-name: com.mysql.jdbc.Driver
url: jdbc:mysql://localhost:3306/test
username: root
password: 123456
- 2.编写方法和sql
@GetMapping("/getException1")
public Result getException1(String username){
studentService.getByName(username);
return new Result(ErrorEnum.SUCCESS);
}
@Override
public Map<String, Object> getByName(String username) {
Map<String, Object> user = dao.getByName(username);
if(user==null){
throw new ServiceException(ErrorEnum.ERROR.getCode(),"用户不存在");
}
return user;
}
@Mapper
public interface StudentDao {
@Select("select * from user where username=#{username}")
Map<String,Object> getByName(String username);
}
- 3.创建业务异常类,并注册到全局异常处理方法中
package com.example.exceptionspring.exception;
import lombok.Data;
@Data
public class ServiceException extends RuntimeException{
private Integer code;
private String msg;
public ServiceException(Integer code, String msg) {
this.code = code;
this.msg = msg;
}
}
GlobalException类中新建handleServiceException方法
@ExceptionHandler(value = ServiceException.class)
public Result handleServiceException(ServiceException e){
return new Result(ErrorEnum.ERROR.getCode(),e.getMsg());
}
- 4.测试