1、加号运算符重载
#include <iostream>
using namespace std;
#include <string>
//加号运算符重载
class Person {
public:
//1、成员函数重载+号
//Person operator+(Person& p) {
// Person temp;
// temp.m_A = this->m_A + p.m_A;
// temp.m_B = this->m_B + p.m_B;
// return temp;
//}
int m_A;
int m_B;
};
//2、全局函数重载+号
Person operator+(Person &p1,Person &p2) {
Person temp;
temp.m_A= p1.m_A + p2.m_A;
temp.m_B = p1.m_B + p2.m_B;
return temp;
}
//函数重载的版本
Person operator+(Person& p1, int num) {
Person temp;
temp.m_A = p1.m_A + num;
temp.m_B = p1.m_B + num;
return temp;
}
void test01() {
Person p1;
p1.m_A = 10;
p1.m_B = 10;
Person p2;
p2.m_A = 10;
p2.m_B = 10;
//成员函数重载本质调用
//Person p3 = p1.operator+(p2);
//全局函数重载本质调用
//Person p3 = operator+(p1, p2);
//运算符重载 也可以发生函数重载
Person p4 = p1 + 100;// Person + int
cout << "p4.m_A = " << p4.m_A << endl;
cout << "p4.m_B = " << p4.m_B << endl;
Person p3 = p1 + p2;
cout << "p3.m_A = " << p3.m_A << endl;
cout << "p3.m_B = " << p3.m_B << endl;
}
int main() {
test01();
system("pause");
return 0;
}
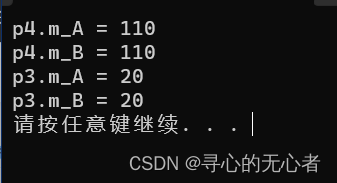
2、左移运算符重载
#include <iostream>
using namespace std;
#include <string>
//左移运算符
class Person {
friend ostream& operator<<(ostream& cout, Person& p);
//public:
public:
Person(int a, int b) {
m_A = a;
m_B = b;
}
private:
//利用成员函数重载 左移运算符 p,operator<<(cout) 简化版本p<<cout
//不会利用成员函数重载<<运算符 因为无法实现 cout在左侧
/*void operator<<(cout) {
}*/
int m_A;
int m_B;
};
//只能利用全局函数重载左移运算符
ostream& operator<<(ostream& cout, Person& p) {//本质:operator<<(cout,p) 简化cout<<p
cout << "m_A = " << p.m_A << " m_B = " << p.m_B ;
return cout;
}
void test01() {
/*Person p;
p.m_A = 10;
p.m_B = 10;*/
Person p(10,10);
cout << p << endl;;
}
int main() {
test01();
system("pause");
return 0;
}
3、递增运算符重载
#include <iostream>
using namespace std;
#include <string>
//递增运算符重载
//自定义整型
class MyInteger {
friend ostream& operator<<(ostream& cout, MyInteger myint);
public:
MyInteger() {
m_Num = 0;
}
//重载前置++运算符 返回引用为了一直对一个数据进行递增操作
MyInteger& operator++() {
//先进行++运算 在将自身做返回
m_Num++;
return *this;
}
//重载后置++运算符
//void operator++(int) int代表占位参数,可以用于区分前置和后置
MyInteger operator++(int) {
//先 记录当前结果
MyInteger temp = *this;
//后递增
m_Num++;
//最后将记录结果做返回
return temp;
}
private:
int m_Num;
};
//重载<<运算符
ostream& operator<<(ostream& cout, MyInteger myint) {
cout << myint.m_Num;
return cout;
}
void test01() {
MyInteger myint;
//cout << ++myint << endl;
cout << ++(++myint) << endl;
cout << myint << endl;
}
void test02() {
MyInteger myint;
cout << myint++ << endl;
cout << myint << endl;
}
int main() {
//test01();
test02();
system("pause");
return 0;
}
4、赋值运算符重载
#include <iostream>
using namespace std;
#include <string>
//赋值运算符重载
class Person {
public:
Person(int age) {
m_Age=new int(age);
}
~Person() {
if (m_Age != NULL) {
delete m_Age;
m_Age = NULL;
}
}
//重载 赋值运算符
Person& operator=(Person& p) {
//编译器提供浅拷贝
//m_Age = p.m_Age;
//应该先判断是否有属性在堆区,如果有先释放干净,融合再深拷贝
if (m_Age != NULL) {
delete m_Age;
m_Age = NULL;
}
//深拷贝
m_Age = new int(*p.m_Age);
return *this;
}
int* m_Age;
};
void test01() {
Person p1(18);
Person p2(20);
Person p3(30);
p3 = p2 = p1;//赋值操作
cout << "p1的年龄为:" << *p1.m_Age << endl;
cout << "p2的年龄为:" << *p2.m_Age << endl;
cout << "p3的年龄为:" << *p3.m_Age << endl;
}
int main() {
test01();
system("pause");
return 0;
}
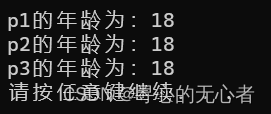
5、关系运算符重载
#include <iostream>
using namespace std;
#include <string>
//重载关系运算符
class Person {
public:
Person(string name, int age) {
m_Name = name;
m_Age = age;
}
//重载关系运算符==
bool operator== (Person& p) {
if (this->m_Name == p.m_Name && this->m_Age == p.m_Age) {
return true;
}
return false;
}
//重载关系运算符!=
bool operator!= (Person& p) {
if (this->m_Name == p.m_Name && this->m_Age == p.m_Age) {
return false;
}
return true;
}
string m_Name;
int m_Age;
};
void test01() {
Person p1("Tom", 18);
Person p2("Tom", 18);
if (p1 == p2) {
cout << "p1和p2是相等的" << endl;
}
else {
cout << "p1和p2是不相等的" << endl;
}
if (p1 != p2) {
cout << "p1和p2是不相等的" << endl;
}
else {
cout << "p1和p2是相等的" << endl;
}
}
int main() {
test01();
system("pause");
return 0;
}
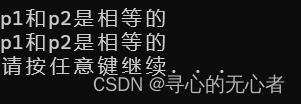
6、函数调用运算符重载
#include <iostream>
using namespace std;
#include <string>
//函数调用运算符重载
//打印输出类
class MyPrint {
public:
//重载函数调用运算符
void operator()(string test){
cout << test << endl;
}
};
void test01() {
MyPrint myPrint;
myPrint("hello world");//由于使用起来非常类似于函数调用 因此称为仿函数
}
//仿函数非常灵活,没有固定的写法
//加法类
class MyAdd {
public:
int operator()(int num1, int num2) {
return num1 + num2;
}
};
void test02() {
MyAdd myadd;
int ret=myadd(100, 100);
cout << "ret = " << ret << endl;
//匿名函数对象
cout << MyAdd()(100, 100) << endl;
}
int main() {
//test01();
test02();
system("pause");
return 0;
}
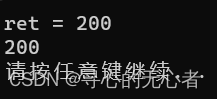