一、正则表达式
- 边界符
^
:表示以后面字符开头
$
:表示以前方字符结尾 - 量词
*
:前面的字符至少出现0次
+
:前面的字符至少出现1次
?
:前面的字符出现0/1次
{n}
:重复n次
{n,}
:至少重复n次
{n,m}
:重复n~m次 - 字符类
/^[a-z]$/
[^a-z]匹配除a-z的字符
- 预定义类
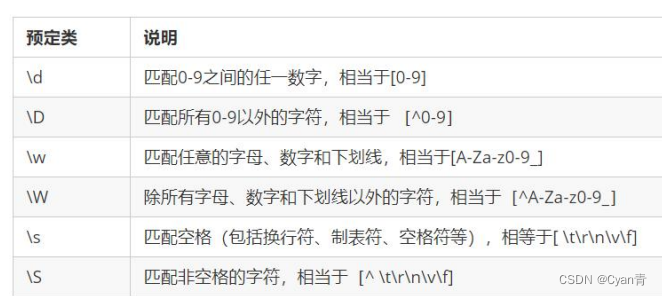
- 修饰符
i:忽略大小写
g:全部匹配
/^abc/i.test('Abc')
/^abc/g.test('abc123abc')
str = 'abc123Abc'
str.replace(/abc/ig,'哈哈')
二、正则的使用
const regex = /^abc$/
regex.test('abc')
regex.exex('abcdefgabc')
登录案例
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<link rel="stylesheet" type="text/css" href="login.css">
</head>
<body>
<div class="box">
<div class="nav">
<a href="javascript:;" class="active">账户登录</a>
|
<a href="javascript:;">二维码登录</a>
</div>
<form class="user-login">
<p>手机验证码登录</p>
<div class="input">
<span>🐷</span>
<input type="text" placeholder="请输入用户名称">
</div>
<div class="input">
<span>🔒</span>
<input type="password" placeholder="请输入密码">
</div>
<div class="agree">
<input type="checkbox">
我已同意《<a href="#">服务条款</a>》和《<a href="#">服务条款</a>》
</div>
<button class="login">
登录
</button>
<div class="footer">
<a href="#">忘记密码?</a>
<a href="#">免费注册</a>
</div>
</form>
<div class="code-login">
<img src="./JS基础/WebAPI/images/desktop_2.jpg" alt="">
<p>请使用手机扫描二维码登录</p>
</div>
</div>
</body>
<script>
const form = document.querySelector('form')
const usrL = document.querySelector('.nav a:nth-child(1)')
const code = document.querySelector('.nav a:nth-child(2)')
const nav = document.querySelector('.nav')
const usrlog = document.querySelector('.user-login')
const codelog = document.querySelector('.code-login')
form.addEventListener('submit', function (e) {
const usr = document.querySelectorAll('.input input')
const agree = document.querySelector('.agree input:nth-child(1)')
const regex = /^\w+$/
if (!(regex.test(usr[0].value) && usr[1].value !== '' )){
console.log(usr[0].value);
e.preventDefault()
return alert('请完善账号密码!')
}
if(!agree.checked){
e.preventDefault()
return alert('请勾选同意')
}
alert('登录成功!')
form.reset()
})
nav.addEventListener('click',function(e){
if(e.target === usrL){
document.querySelector('.active').classList.remove('active')
usrL.classList.add('active')
codelog.style.display = 'none'
usrlog.style.display = 'block'
}
if(e.target === code){
document.querySelector('.active').classList.remove('active')
code.classList.add('active')
codelog.style.display = 'block'
usrlog.style.display = 'none'
}
})
</script>
</html>
* {
margin: 0 auto;
padding: 0 auto;
}
body {
background-color: #ddd;
}
.box {
width: 300px;
height: 320px;
padding: 20px 35px;
background-color: #fff;
text-align: center;
margin-top: 100px;
color: #CFCDCD;
}
a {
text-decoration: none;
}
.nav {
width: 100%;
height: 50px;
color: black;
position: relative;
}
.nav a {
padding-bottom: 5px;
color: black;
font-size: 20px;
}
.nav a:nth-child(1) {
position: absolute;
left: 0px;
}
.nav a:nth-child(2) {
position: absolute;
right: 0px;
}
.user-login {
width: 100%;
}
.user-login p {
text-align: right;
color: #5fa094;
cursor: pointer;
}
.code-login {
display: none;
}
.login {
width: 100%;
height: 50px;
background-color: #43b99a;
color: white;
line-height: 50px;
border: #fff;
cursor: pointer;
}
.input {
border: 1px solid #17bb9b;
width: 100%;
height: 36px;
margin-top: 17px;
display: flex;
background: #CFCDCD;
}
.input input {
flex: 1;
padding-left: 15px;
background: #fff;
outline: none;
border: none;
}
.input span {
width: 34px;
height: 34px;
text-align: center;
line-height: 34px;
font-size: 20px;
}
.agree {
width: 100%;
text-align: left;
margin: 20px 0;
}
.agree a {
color: #56606b;
}
.footer {
margin-top: 10px;
position: relative;
}
.footer a:nth-child(1){
position: absolute;
left: 0;
color: black;
}
.footer a:nth-child(2){
position: absolute;
right: 0;
color: black;
}
.active {
border-bottom: 2px solid #17bb9b;
}
.code-login {
width: 300px;
height: 200px;
}
.code-login img {
width: 100%;
height: 100%;
margin-bottom: 20px;
}