目录
1、定时器
2、计时器
2.1 QTime 时间转换成字符串函数
3、QT 获取日期,时间,星期
4、综合应用
定时器是用来处理周期性事件的一种对象,类似于硬件定时器。例如设置一个定时器的定时周期为 1000 毫 秒,那么每 1000 毫秒就会发射定时器的 timeout() 信号,在信号关联的槽函数里就可以做相应的处理。
1、定时器
使用 QTimer 定时器类。使用过程:
1
、 首先创建一个定时器类的对象 QTimer *timer = new QTimer(this);
2
、
timer
超时后会发出
timeout()
信号,所以在创建好定时器对象后给其建立信号与槽 connect(timer, SIGNAL(timeout()), this, SLOT(onTimeout()));
3
、 在需要开启定时器的地方调用 void QTimer::start ( int msec ); 这个 start
函数参数也是毫秒级别; timer->start(msec );
4
、 在自己的超时槽函数里面做超时处理。
例如:
#ifndef _MYTIMER_H
#define _MYTIMER_H
#include <QObject>
class QTimer;
class MyTimer : public QObject
{
Q_OBJECT
public:
MyTimer(QObject* parent = NULL);
~MyTimer();
public slots:
void handleTimeout(); //超时处理函数
private:
QTimer *m_pTimer;
};
#endif //_MYTIMER_H
#include "widget.h"
#include<QDebug>
#include <QTimer>
#define TIMER_TIMEOUT (5*1000)
MyTimer::MyTimer(QObject *parent)
:QObject(parent)
{
m_pTimer = new QTimer(this);
connect(m_pTimer, SIGNAL(timeout()), this, SLOT(doTimeout()));
m_pTimer->start(TIMER_TIMEOUT);
}
MyTimer::~MyTimer()
{
}
void MyTimer::doTimeout()
{
qDebug()<<"Enter timeout processing function\n";
if(m_pTimer->isActive()){
m_pTimer->stop();
}
}
2、计时器
当
Qt
需要使用计时时
,
就可以使用
Qt
的计时类
Qtime
。
1. QTime
的计时方式主要是通过时分秒计时
2. QTime
可以转换为
HH:MM:SS
这种的数据格式
3. QTime
不会自动计时
,
需要自己手动加时间
,
时间可以是
1
秒
,
也可以是自定义的时长
4. QTime
增加时间使用的函数
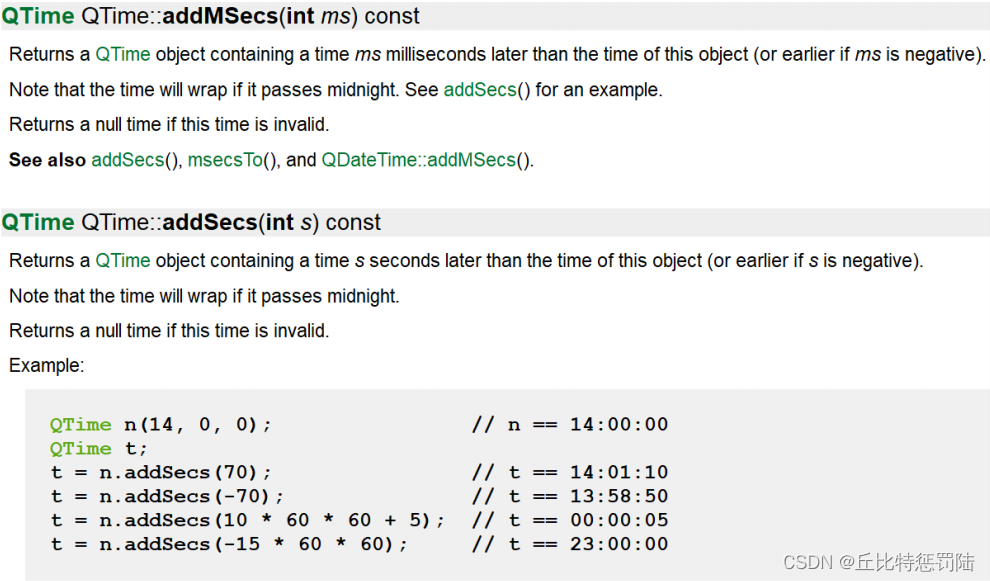
2.1 QTime 时间转换成字符串函数
例如:
Widget::Widget(QWidget *parent) :
QWidget(parent),
ui(new Ui::Widget)
{
ui->setupUi(this);
QTime time = QTime::currentTime();//获取当前事件
QString strTime = time.toString("hh:mm:ss.zzz");//转换成 QString
time = QTime::fromString("02:23:45.789","hh:mm:ss.zzz");//QString 转 QTime
strTime = time.toString("hh:mm:ss.zzz");
QTime time1(7,30,5 ,100);//传递时分秒毫秒构造函数
strTime = time1.toString("hh:mm:ss.zzz");
int hour = time1.hour();//获取小时,7
qDebug()<<hour;
int minute=time1.minute();//获取分钟,30
qDebug()<<minute;
int second = time1.second();//获取秒数,5
qDebug()<<second;
int mssecond = time1.msec();//获取毫秒,100
qDebug()<<mssecond;
QTime t1 = time1.addMSecs(100);//增加 100ms
qDebug()<<t1.msec();
QTime t2 = time1.addSecs(80);//增加 80s
qDebug()<<t2.second();
if(time < time1)//比较两个 QTime,时间晚的大
qDebug()<<"time<time1";
QTime t3;
t3.start();//开始计时
Sleep(1000);
int elspsed = t3.elapsed();//计算多少 ms 过去了
t3.restart();//重新计时
int milsecond = QTime::currentTime().msecsSinceStartOfDay();//返回从零点开
始共计多少 ms
qDebug()<<milsecond;
QTime t4(22,25,10);
QTime t5(22,24,0);
qDebug()<<t4.secsTo(t5);//两者相差了多少秒,t4 到 t5 需要多少秒,如果 t5<t4,返
回负值
qDebug()<<strTime;
}
3、QT 获取日期,时间,星期
QT
类库
QT
提供
QDate
、
QTime
、
QDateTime
三个类对日期,时间等进行操作。
功能接口
QDateTime::currentDateTime()
→
获取当前日期时间,返回一个
QDateTime
对象
toString(“yyyy.MM.dd hh:mm:ss.zzz ddd”)
→
将
QDateTime
对象的日期时间,转换为
date time
标准格式。
Widget::Widget(QWidget *parent) :
QWidget(parent),
ui(new Ui::Widget)
{
ui->setupUi(this);
QDateTime current_date_time = QDateTime::currentDateTime();
qDebug()<<current_date_time;
QString current_week = current_date_time.toString("ddd");
qDebug() << "week: "<<current_week; //获取星期,“周一”,“周二”,“周三”等
等。
QString current_date = current_date_time.toString("yyyy.MM.dd hh:mm:ss.zzz
ddd"); //格式化为 QString
qDebug()<<current_date;
QTime current_time = QTime::currentTime(); //获取当前时间
qDebug()<<current_time;
int minute = current_time.minute(); //获取分钟数据,int 类型
qDebug()<<"minute : "<< minute;
int hour = current_time.hour(); //获取小时数据,int 类型
qDebug()<<"hour: "<<hour;
}
4、综合应用
Qt
中的定时器类是
QTimer
。
QTimer
不是一个可见的界面组件,在
UI
设计器的组件面板里找不到它。图 1 中的实例程序实现了一个计时器的功能,就是计算定时器开始到停止持续的时间长度,计时器是
QTime
类。
QTimer
主要的属性是
interval
,是定时中断的周期,单位毫秒。
QTimer
主要的信号是
timeout()
,在定时中断时发射此信号,要想在定时中断里做出响应,这就需要编写 timeout()
信号的槽函数。下面是窗口类中增加的 定义。
class Dialog : public QDialog
{
private:
QTimer *fTimer; //定时器
QTime fTimeCounter;//计时器
private slots:
void on_timer_timeout () ; //定时溢出处理槽函数
};
这里定义了一个定时器
fTimer
,—个计时器
fTimeCounter
。还定义了一个槽函数
on_timer_ timeout()
,作为 定时器的 timeout()
信号的响应槽函数。 需要在窗口类的构造函数里创建定时器,并进行信号与槽的关联。
Dialog::Dialog(QWidget *parent) : QDialog(parent), ui(new Ui::Dialog)
{
ui->setupUi(this);
fTimer=new QTimer(this);
fTimer->stop();
fTimer->setInterval (1000) ;//设置定时周期,单位:毫秒
connect(fTimer,SIGNAL(timeout()),this,SLOT(on_timer_timeout()));
}
槽函数
on_timer_timeout()
的实现代码如下:
void Dialog::on_timer_timeout()
{
//定时器中断响
QTime curTime=QTime::currentTime(); //获取当前时间
ui->LCDHour->display (curTime.hour()); //显示小时
ui->LCDMin->display (curTime.minute()); //显示分钟
ui->LCDSec->display(curTime.second()); //显示秒
int va=ui->progressBar->value();
va++;
if (va>100)
va=0;
ui->progressBar->setValue(va);
}
这段代码首先用
QTime
类的静态函数
Qtime::currentTime()
获取当前时间,然后用
QTime
的成员函数 hour()、
minute()
、
second()
分别获取小时、分钟、秒,并在几个
LCDNumber
组件上显不。循环更新
progressBar的值,是为了让界面有变化,表示定时器在运行。
设置定时器的周期,只需调用
Qtimer::setlnterval()
函数即可。
QTimer::start()
函数用于启动定时器,界面上的“开始”按钮代码如下:
void Dialog::on_btnStart_clicked()
{
fTimer->start () ;//定时器开始工作
fTimeCounter.start () ; //计时器开始工作
ui->btnStart->setEnabled(false);
ui->btnStop->setEnabled(true);
ui->btnSetIntv->setEnabled(false);
}
计时器
fTimeCounter
执行
start()
是将当前时间作为计时器的时间。
QTimer::Stop()
函数停止定时器,界面上的“停止”按钮可实现这一功能,其代码如下:
void Dialog::on_btnStop_clicked()
{
fTimer->stop () ; //定时器停止
int tmMsec=fTimeCounter.elapsed() ;//毫秒数
int ms=tmMsec%1000;
int sec=tmMsec/1000;
QString str=QString::asprintf ("流逝时间:%d 秒,%d 毫秒", sec, ms);
ui->LabElapsTime->setText(str);
ui->btnStart->setEnabled(true);
ui->btnStop->setEnabled(false);
ui->btnSetIntv->setEnabled(true);
}
例如: