【多线程的应用】顺序打印
- 题目
- 注意点:
- 1. 每个线程循环10次,利用锁的wait 和 计数器count
- 调节线程的执行顺序
- 2. count++后 lock.notifyAll 唤醒所有线程
- 3. Thread.currentThread().getName()
- 4. 锁中的逻辑是:进入锁中后,如果while不
- 满足 那就wait,count++所有wait唤醒,再判断count
- 当然每次一定是 解锁一个wait 其余在while继续wait
- 2. 多线程的顺序打印
- 同时启动的话,那么每个线程中 join一下优先打印的
题目
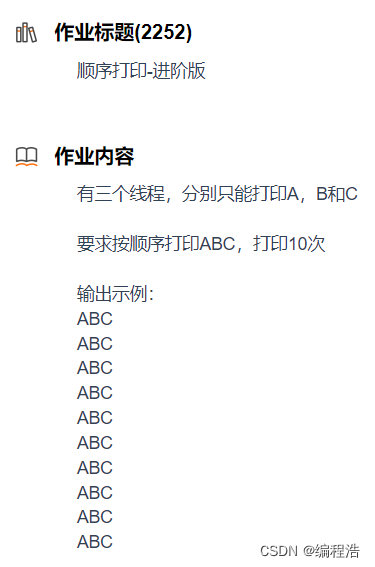
class Thread_2252 {
private static volatile int COUNTER = 0;
private static Object lock = new Object();
public static void main(String[] args) {
Thread t1 = new Thread(() -> {
for (int i = 0; i < 10; i++) {
synchronized (lock) {
while (COUNTER % 3 != 0) {
try {
lock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
System.out.print(Thread.currentThread().getName());
COUNTER++;
lock.notifyAll();
}
}
}
, "A");
Thread t2 = new Thread(() -> {
for (int i = 0; i < 10; i++) {
synchronized (lock) {
while (COUNTER % 3 != 1) {
try {
lock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
System.out.print(Thread.currentThread().getName());
COUNTER++;
lock.notifyAll();
}
}
}, "B");
Thread t3 = new Thread(() -> {
for (int i = 0; i < 10; i++) {
synchronized (lock) {
while (COUNTER % 3 != 2) {
try {
lock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
System.out.println(Thread.currentThread().getName());
COUNTER++;
lock.notifyAll();
}
}
}, "C");
t1.start();
t2.start();
t3.start();
}
}
注意点:
1. 每个线程循环10次,利用锁的wait 和 计数器count
调节线程的执行顺序
2. count++后 lock.notifyAll 唤醒所有线程
3. Thread.currentThread().getName()
4. 锁中的逻辑是:进入锁中后,如果while不
满足 那就wait,count++所有wait唤醒,再判断count
当然每次一定是 解锁一个wait 其余在while继续wait
2. 多线程的顺序打印
同时启动的话,那么每个线程中 join一下优先打印的
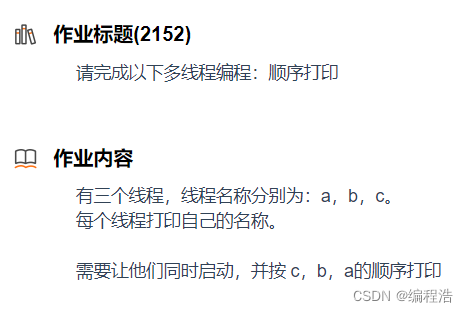
public class Thread_2152 {
public static void main(String[] args) throws InterruptedException {
Thread tc = new Thread(() -> {
System.out.print(Thread.currentThread().getName() + " ");
}, "c");
Thread tb = new Thread(() -> {
try {
tc.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.print(Thread.currentThread().getName() + " ");
}, "b");
Thread ta = new Thread(() -> {
try {
tb.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.print(Thread.currentThread().getName() + " ");
}, "a");
ta.start();
tb.start();
tc.start();
}
}