1、Vue概述
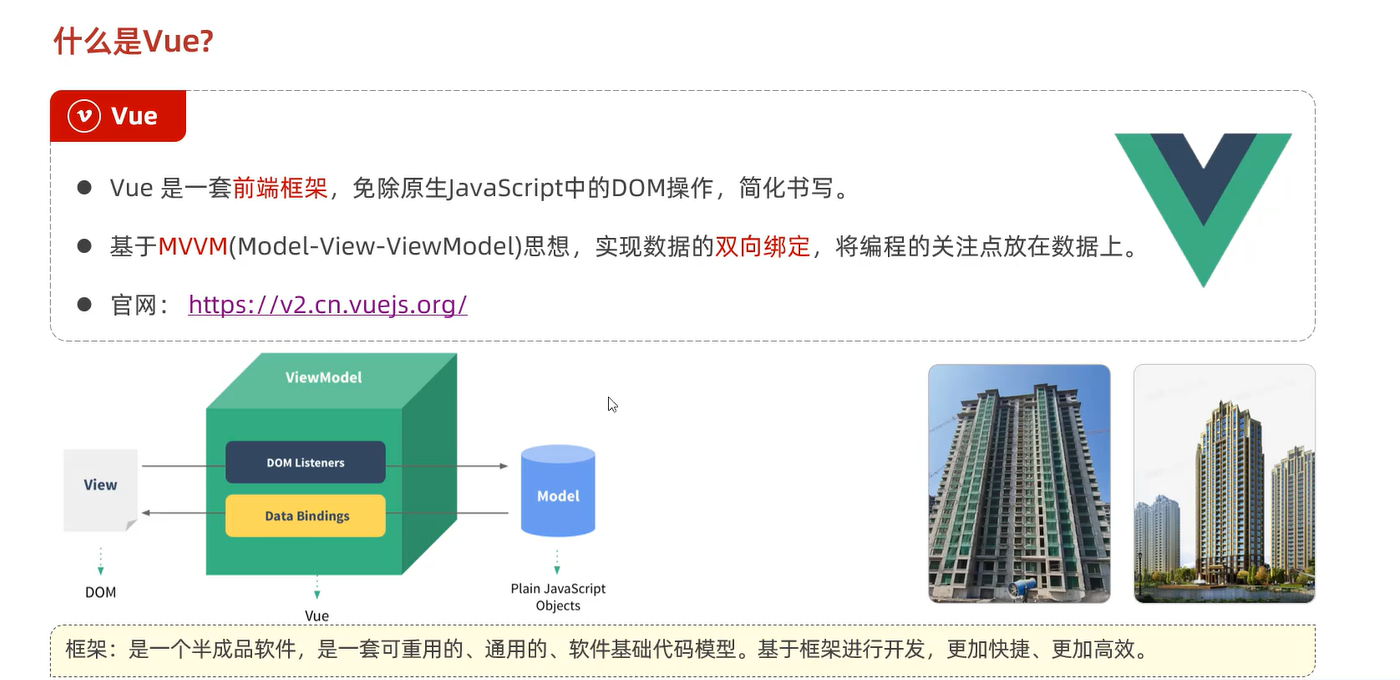
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Vue-快速入门</title>
<!-- 1、引入vue.js -->
<script src="./js/vue.js"></script>
</head>
<body>
<!-- 3、定义视图:负责展示 -->
<div id="app">
<input type="text" v-model="message"><!-- v-model:绑定一个数据模型。v-model叫指令! -->
{{message}}<!-- 直接在页面展示message数据模型,{{message}}:插值表达式 -->
</div>
</body>
<script>
// 2、定义vue对象
new Vue({
el: "#app",/* el代表Vue所接管的数据区域 */
data:{/* data是定义数据模型 */
message:"Hello Vue"
}
})
</script>
</html>
输入框和{{message}}使用的都是同一个数据模型,由于vue的双向绑定,所以输入框的视图发生变化,就会影响数据模型的变化,数据模型的变化会影响视图数据的变化。所以输入框输入什么,后面也跟着显示什么数据。
2、Vue-常用指令
1、v-bind和v-model
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="js/vue.js"></script>
</head>
<body>
<div id="app">
<a v-bind:href="url">链接1</a>
<!-- v-bind:href可以简写为:href -->
<a :href="url">链接2</a>
<input type="text" v-model="url">
</div>
</body>
<script>
new Vue({
el: "#app",
data:{
url:"https://www.baidu.com"
}
})
</script>
</html>
v-model:表单元素有input、textarea、select
2、v-on
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="js/vue.js"></script>
</head>
<body>
<div id="app">
<button v-on:click="handle()">按钮1</button>
<button @click="handle()">按钮2</button>
</div>
</body>
<script>
new Vue({
//el、data、methods都是对象的属性,对象用{}
el: "#app",//vue接管区域
data:{
},
methods:{
handle:function(){
alert("你点了我一下....");
}
}
})
</script>
</html>
3、v-if&v-show&v-for
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="js/vue.js"></script>
<title>v-if&v-show&v-for</title>
</head>
<body>
<div id="app">
<input type="text" v-model="age">
年龄:{{age}},经判定为:
<span v-if="age <= 35">年轻人</span>
<span v-else-if="age > 35 && age < 60">中年人</span>
<span v-else>老年人</span>
<br/>
<input type="text" v-model="age">
年龄:{{age}},经判定为:
<span v-show="age <= 35">年轻人</span>
<span v-show="age > 35 && age < 60">中年人</span>
<span v-show="age >= 60">老年人</span>
</div>
</body>
<script>
new Vue({
el : "#app",
data:{
age:20
},
methods:{
}
})
</script>
</html>
可以看到,用v-show时,三个span标签都会被渲染,但是另外两个的display为none。
v-for
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="js/vue.js"></script>
</head>
<body>
<div id="app">
<div v-for="(addr,index) in addrs">
<table border="1">
<tr>
<td>{{index+1}}位</td>
<td>{{addr}}</td>
</tr>
</table>
</div>
</div>
</body>
<script>
new Vue({
el : "#app",
data:{
addrs:['北京','上海','山东','济南']
},
methods:{
}
})
</script>
</html>
4、v-指令-案例
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="js/vue.js"></script>
</head>
<body>
<div id="app">
<!-- border="1" cellspacing="0" width="60%" -->
<table border="1" cellspacing="0" width="60%">
<tr>
<th>编号</th>
<th>姓名</th>
<th>年龄</th>
<th>性别</th>
<th>成绩</th>
<th>等级</th>
</tr>
<tr v-for="(userifm,index) in user">
<td>{{index+1}}</td>
<td>{{userifm.name}}</td>
<td>{{userifm.age}}</td>
<td v-if="userifm.gender==1">男</td>
<td v-if="userifm.gender==2">女</td>
<td>{{userifm.score}}</td>
<td v-if="userifm.score >=85 ">优秀</td>
<td v-else-if="userifm.score >=60 ">及格</td>
<td v-else style="color: red;">不及格</td>
</tr>
</table>
</div>
</body>
<script>
new Vue({
el: "#app",
data:{
user:[{
name:"Tom",
age:20,
gender:1,
score:78
},{
name:"Rose",
age:18,
gender:2,
score:86
},{
name:"Jerry",
age:26,
gender:1,
score:90
},{
name:"Jim",
age:22,
gender:1,
score:59
}]
},
methods:{
}
})
</script>
</html>
5、vue- 生命周期
mounted方法主要就是用来发送请求到服务端来获取或者加载数据。