文章目录
- 1.common-mybatis-plus-starter
- 1.目录
- 2.PageInfo.java
- 3.PageResult.java
- 4.SunPageHelper.java
1.common-mybatis-plus-starter
1.目录
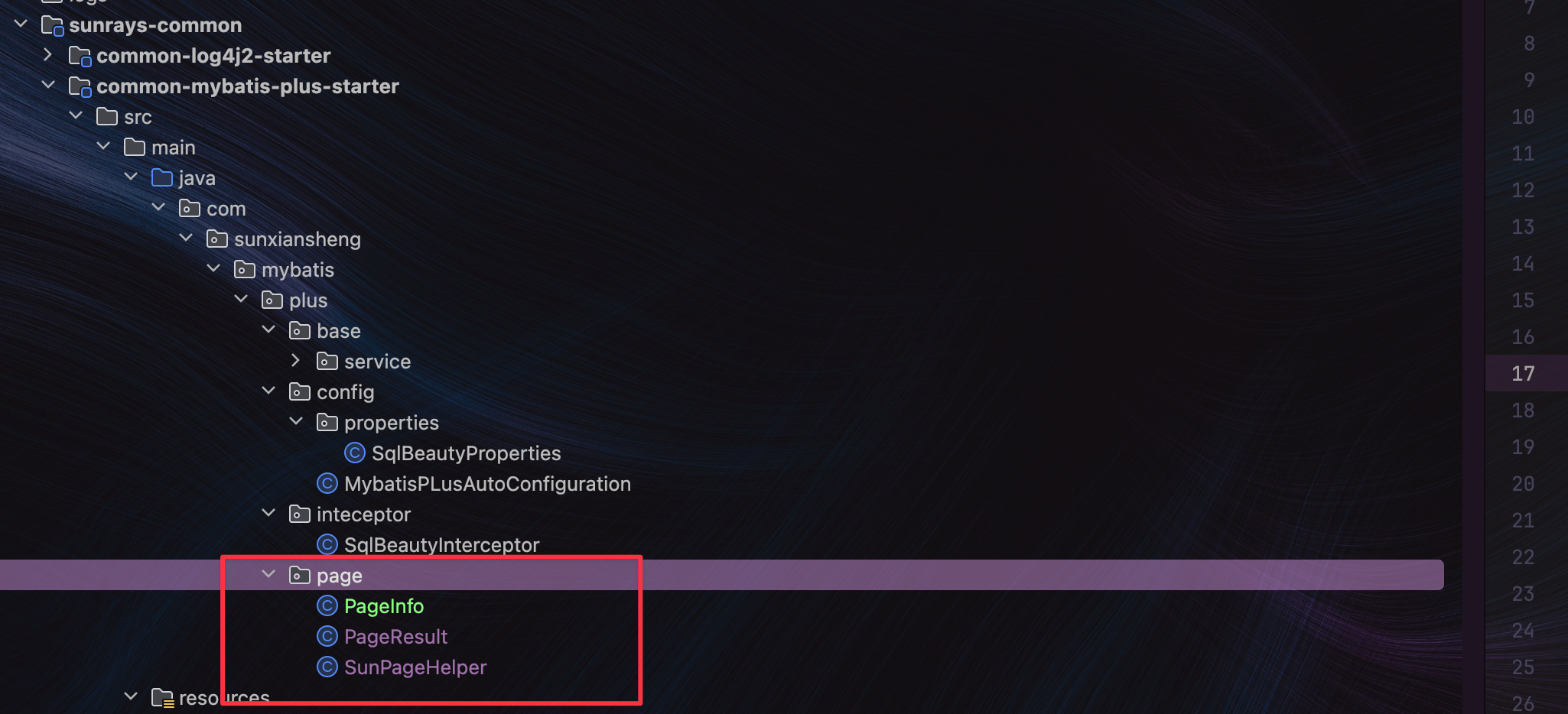
2.PageInfo.java
package com.sunxiansheng.mybatis.plus.page;
import lombok.EqualsAndHashCode;
import lombok.ToString;
import java.io.Serializable;
@EqualsAndHashCode
@ToString
public class PageInfo implements Serializable {
private static final long serialVersionUID = 1L;
private Long pageNo = 1L;
private Long pageSize = 20L;
public Long getPageNo() {
return (pageNo == null || pageNo < 1) ? 1 : pageNo;
}
public Long getPageSize() {
return (pageSize == null || pageSize < 1) ? 20 : pageSize;
}
public PageInfo setPageNo(Long pageNo) {
this.pageNo = pageNo;
return this;
}
public PageInfo setPageSize(Long pageSize) {
this.pageSize = pageSize;
return this;
}
}
3.PageResult.java
package com.sunxiansheng.mybatis.plus.page;
import lombok.*;
import java.io.Serializable;
import java.util.List;
import static java.util.Objects.requireNonNull;
@EqualsAndHashCode
@ToString
@NoArgsConstructor
@Getter
public class PageResult<T> implements Serializable {
private static final long serialVersionUID = 1L;
private Long pageNo;
private Long pageSize;
private Long total;
private Long totalPages;
private List<T> result;
private Long start;
private Long end;
private boolean hasNextPage;
private boolean hasPreviousPage;
private PageResult(Builder<T> builder) {
this.pageNo = builder.pageNo;
this.pageSize = builder.pageSize;
this.total = builder.total;
this.result = builder.result;
calculateTotalPages();
calculateStartAndEnd();
this.hasPreviousPage = this.pageNo > 1;
this.hasNextPage = this.pageNo < this.totalPages;
}
public static class Builder<T> {
private Long pageNo;
private Long pageSize;
private Long total;
private List<T> result;
public Builder<T> pageNo(Long pageNo) {
this.pageNo = requireNonNull(pageNo, "Page number cannot be null");
return this;
}
public Builder<T> pageSize(Long pageSize) {
this.pageSize = requireNonNull(pageSize, "Page size cannot be null");
return this;
}
public Builder<T> total(Long total) {
this.total = requireNonNull(total, "Total count cannot be null");
return this;
}
public Builder<T> result(List<T> result) {
this.result = requireNonNull(result, "Result list cannot be null");
return this;
}
public PageResult<T> build() {
if (pageNo < 1) {
throw new IllegalArgumentException("Page number must be greater than zero.");
}
if (pageSize < 1) {
throw new IllegalArgumentException("Page size must be greater than zero.");
}
return new PageResult<>(this);
}
}
private void calculateTotalPages() {
if (this.pageSize > 0) {
this.totalPages = (this.total / this.pageSize) + (this.total % this.pageSize == 0 ? 0 : 1);
} else {
this.totalPages = 0L;
}
}
private void calculateStartAndEnd() {
if (this.pageSize > 0) {
this.start = (this.pageNo - 1) * this.pageSize + 1;
this.end = Math.min(this.pageNo * this.pageSize, this.total);
} else {
this.start = 1L;
this.end = this.total;
}
}
}
4.SunPageHelper.java
package com.sunxiansheng.mybatis.plus.page;
import java.util.Collections;
import java.util.List;
import java.util.function.BiFunction;
import java.util.function.Supplier;
public class SunPageHelper {
public static <T> PageResult<T> paginate(Long pageNo, Long pageSize,
Supplier<Long> totalSupplier,
BiFunction<Long, Long, List<T>> recordsSupplier) {
Long total;
try {
total = totalSupplier.get();
} catch (Exception e) {
throw new RuntimeException("Failed to get total count", e);
}
if (total == 0) {
return new PageResult.Builder<T>()
.pageNo(pageNo)
.pageSize(pageSize)
.total(total)
.result(Collections.emptyList())
.build();
}
Long offset = calculateOffset(pageNo, pageSize);
List<T> records;
try {
records = recordsSupplier.apply(offset, pageSize);
} catch (Exception e) {
throw new RuntimeException("Failed to get records", e);
}
return new PageResult.Builder<T>()
.pageNo(pageNo)
.pageSize(pageSize)
.total(total)
.result(records)
.build();
}
public static Long calculateOffset(Long pageNo, Long pageSize) {
return (pageNo - 1) * pageSize;
}
}