一.实现效果展示
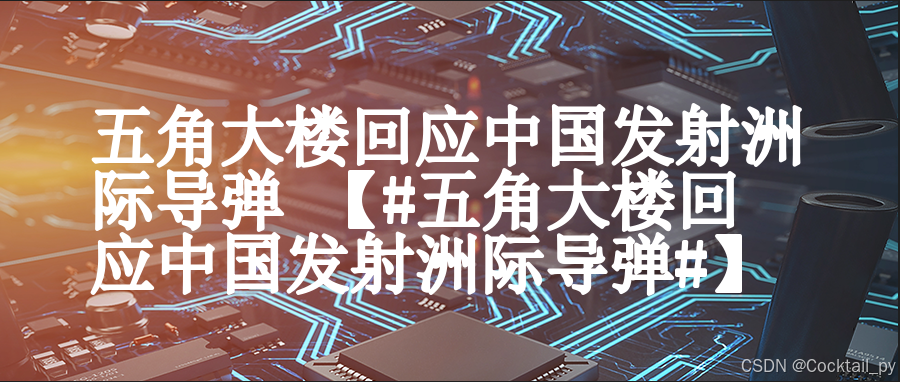
二.代码
from PIL import Image, ImageFont, ImageDraw
def split_string(s, num_parts):
length = len(s)
chunk_size = length // num_parts
remainder = length % num_parts
parts = ['' for _ in range(num_parts)]
start = 0
for i in range(num_parts):
end = start + chunk_size + (1 if i < remainder else 0)
parts[i] = s[start:end]
start = end
return parts
def image_add_text(background_image_path,title_text,border_width=30):
"""
图片加文字 文字自动居中对齐
白色、加粗、字号65px、上下左右居中
:param background_image_path 背景图
:param title_text 需要加的文字
:border_width 设置距离单边距离
"""
image = Image.open(background_image_path)
font = ImageFont.truetype('simsun.ttc', 65)
draw = ImageDraw.Draw(image)
cnt = 1
img_width = image.width
flg =False
while True:
all_tx_list = split_string(title_text,cnt)
for inx,txt in enumerate(all_tx_list):
text_width, text_height = draw.textsize(txt, font=font)
if text_width < (img_width -border_width*2) and (inx+1 == len(all_tx_list)):
flg=True
break
if flg==True:
break
cnt +=1
txt_new = "\n".join(all_tx_list).strip()
text_width, text_height = draw.textsize(txt_new, font=font)
x = (image.width - text_width) // 2
y = (image.height - text_height) // 2
text_color = (255, 255, 255)
draw.text((x, y), txt_new, font=font, fill=text_color, stroke_width=2, stroke_fill=None)
image.save('image_with_title.png')
if __name__ == '__main__':
title_text = '五角大楼回应中国发射洲际导弹 【#五角大楼回应中国发射洲际导弹#】'
background_image_path = '900x380背景.png'
image_add_text(background_image_path,title_text,border_width=20)