1. 常用方法
1.1 字符串构造
1. 使用常量串构造String s1 = "hello" ;2. 直接 newString 对象String s2 = new String ( "hello" );3. 使用字符数组进行构造char [] array = { 'h' , 'e' , 'l' , 'l' , 'o' };String s3 = new String ( array );
代码示例如下:
public class test1 {
public static void main(String[] args) {
// 使用常量串构造
String s1 = "hello bit";
System.out.println(s1);
// 直接newString对象
String s2 = new String("hello bit");
System.out.println(s1);
// 使用字符数组进行构造
char[] array = {'h','e','l','l','o','b','i','t'};
String s3 = new String(array);
System.out.println(s1);
}
}
运行结果如下:
注意: 1. String是引用类型,内部并不存储字符串本身,在String类的实现源码中,String类实例变量如下:
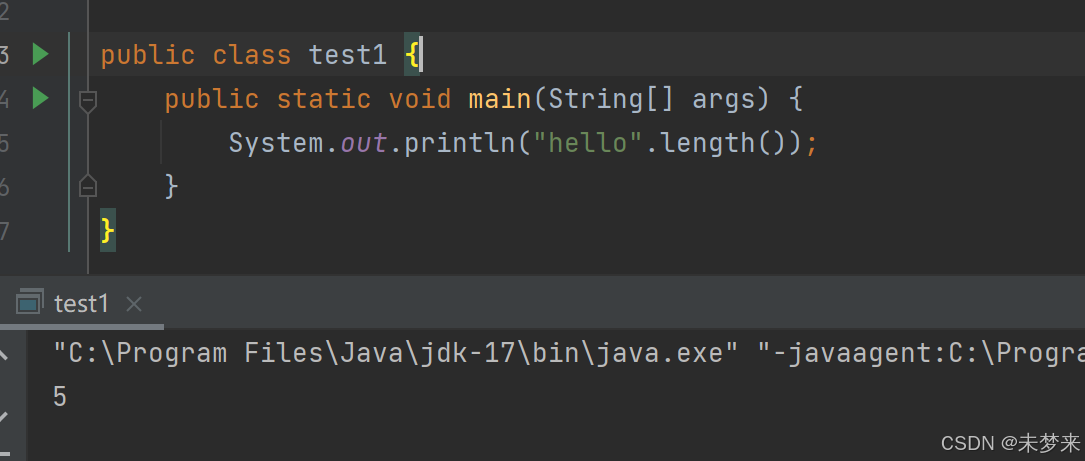
1.2 String 对象的比较
字符串的比较是常见操作之一,比如:字符串排序。Java中总共提供了4中方式:
1.2.1 == 比较是否引用同一个对象
public class test1 {
public static void main(String[] args) {
int a = 10;
int b = 20;
int c = 10;
// 对于基本类型变量,==比较两个变量中存储的值是否相同
System.out.println(a == b); // false
System.out.println(a == c); // true
// 对于引用类型变量,==比较两个引用变量引用的是否为同一个对象
String s1 = new String("hello");
String s2 = new String("hello");
String s3 = new String("world");
String s4 = s1;
System.out.println(s1 == s2); // false
System.out.println(s2 == s3); // false
System.out.println(s1 == s4); // true
}
}
运行结果如下:
注意:对于内置类型,== 比较的是变量中的值;对于引用类型 == 比较的是引用中的地址。
1.2.2 boolean equals(Object anObject) 方法:按照字典序比较
public class test1 {
public static void main(String[] args) {
String s1 = new String("hello");
String s2 = new String("hello");
String s3 = new String("Hello");
// s1、s2、s3引用的是三个不同对象,因此==比较结果全部为false
System.out.println(s1 == s2); // false
System.out.println(s1 == s3); // false
// equals比较:String对象中的逐个字符
// 虽然s1与s2引用的不是同一个对象,但是两个对象中放置的内容相同,因此输出true
// s1与s3引用的不是同一个对象,而且两个对象中内容也不同,因此输出false
System.out.println(s1.equals(s2)); // true
System.out.println(s1.equals(s3)); // false
}
}
运行结果如下:
注意:equals 的具体比较规则如下:
1.2.3 int compareTo(String s) 方法: 按照字典序进行比较
public class test1 {
public static void main(String[] args) {
String s1 = new String("abc");
String s2 = new String("ac");
String s3 = new String("abc");
String s4 = new String("abcdef");
System.out.println(s1.compareTo(s2)); // 不同输出字符差值-1
System.out.println(s1.compareTo(s3)); // 相同输出 0
System.out.println(s1.compareTo(s4)); // 前k个字符完全相同,输出长度差值 -3
}
}
运行结果如下:
1.2.4. int compareToIgnoreCase(String str) 方法:与compareTo方式相同,但是忽略大小写比较
代码示例:
public class test1 {
public static void main(String[] args) {
String s1 = new String("abc");
String s2 = new String("ac");
String s3 = new String("ABc");
String s4 = new String("abcdef");
System.out.println(s1.compareToIgnoreCase(s2)); // 不同输出字符差值-1
System.out.println(s1.compareToIgnoreCase(s3)); // 相同输出 0
System.out.println(s1.compareToIgnoreCase(s4)); // 前k个字符完全相同,输出长度差值 -3
}
}
运行结果如下:
1.3 字符串查找
方法 | 功能 |
char charAt(int index)
|
返回
index
位置上字符,如果
index
为负数或者越界,抛出
IndexOutOfBoundsException
异常
|
int indexOf(int ch)
|
返回
ch
第一次出现的位置,没有返回
-1
|
int indexOf(int ch, int
fromIndex)
|
从
fromIndex
位置开始找
ch
第一次出现的位置,没有返回
-1
|
int indexOf(String str)
|
返回
str
第一次出现的位置,没有返回
-1
|
int indexOf(String str, int
fromIndex)
|
从
fromIndex
位置开始找
str
第一次出现的位置,没有返回
-1
|
int lastIndexOf(int ch)
|
从后往前找,返回
ch
第一次出现的位置,没有返回
-1
|
int lastIndexOf(int ch, int
fromIndex)
|
从
fromIndex
位置开始找,从后往前找
ch
第一次出现的位置,没有返
回
-1
|
int lastIndexOf(String str)
|
从后往前找,返回
str
第一次出现的位置,没有返回
-1
|
int lastIndexOf(String str, int
fromIndex)
|
从
fromIndex
位置开始找,从后往前找
str
第一次出现的位置,没有返
回
-1
|
代码示例:
public class test1 {
public static void main(String[] args) {
String s = "aaabbbcccaaabbbccc";
System.out.println(s.charAt(3));
System.out.println(s.indexOf('c'));
System.out.println(s.indexOf('c', 10));
System.out.println(s.indexOf("bbb"));
System.out.println(s.indexOf("bbb", 10));
System.out.println(s.lastIndexOf('c'));
System.out.println(s.lastIndexOf('c', 10));
System.out.println(s.lastIndexOf("bbb"));
System.out.println(s.lastIndexOf("bbb", 10));
}
}
运行结果如下:
1.4 转化
1.4.1 数值和字符串互相转化
代码示例:
public class test1 {
public static void main(String[] args) {
// 数字转字符串
String s1 = String.valueOf(1234);
String s2 = String.valueOf(12.34);
String s3 = String.valueOf(true);
System.out.println(s1);
System.out.println(s2);
System.out.println(s3);
System.out.println("=================================");
// 字符串转数字
int data1 = Integer.parseInt("1234");
double data2 = Double.parseDouble("12.34");
System.out.println(data1);
System.out.println(data2);
}
}
运行结果如下:
1.4.2 字符串大小写转化
代码示例:
public class test1 {
public static void main(String[] args) {
String s1 = "hello";
String s2 = "HELLO";
// 小写转大写
System.out.println(s1.toUpperCase());
// 大写转小写
System.out.println(s2.toLowerCase());
}
}
运行结果如下:
1.4.3 字符串转数组
代码示例:
public class test1 {
public static void main(String[] args) {
String s = "hello";
// 字符串转数组
char[] ch = s.toCharArray();
for (int i = 0; i < ch.length; i++) {
System.out.print(ch[i]);
}
System.out.println();
// 数组转字符串
String s2 = new String(ch);
System.out.println(s2);
}
}
运行结果如下:
1.4.4 格式化
代码示例:
public class test1 {
public static void main(String[] args) {
String s = String.format("%d-%d-%d", 2024, 9,20);
System.out.println(s);
}
}
运行结果如下:
1.5 字符串替换
方法 | 功能 |
String replaceAll(String regex, String replacement)
|
替换所有的指定内容
|
String replaceFirst(String regex, String replacement)
|
替换首个内容
|
代码示例:
public class test1 {
public static void main(String[] args) {
String str = "helloworld" ;
System.out.println(str.replaceAll("l", "_"));
System.out.println(str.replaceFirst("l", "_"));
}
}
运行结果如下:
1.6 字符串拆分
方法 | 功能 |
String[] split(String regex)
|
将字符串全部拆分
|
String[] split(String regex, int limit)
|
将字符串以指定的格式,拆分为
limit
组
|
代码示例1:
public class test1 {
public static void main(String[] args) {
//字符串的拆分
String str1 = "hello world hello man" ;
String[] result1 = str1.split(" ") ; // 按照空格拆分
for(String s: result1) {
System.out.println(s);
}
System.out.println("==========================");
//字符串的部分拆分
String str2 = "hello world hello man" ;
String[] result2 = str2.split(" ",2) ;
for(String s: result2) {
System.out.println(s);
}
}
}
运行结果如下:
public class test1 {
public static void main(String[] args) {
String str = "192.168.1.1" ;
String[] result = str.split("\\.") ;
for(String s: result) {
System.out.println(s);
}
}
}
运行结果如下:
代码示例3:多次拆分
public class test1 {
public static void main(String[] args) {
//方法1
String str1 = "name=zhangsan&age=18" ;
String[] result1 = str1.split("&") ;
for (int i = 0; i < result1.length; i++) {
String[] temp = result1[i].split("=") ;
System.out.println(temp[0]+" "+temp[1]);
}
//方法2
String str2 = "name=zhangsan&age=18" ;
String[] result2 = str2.split("&|=") ;
for (String s:result2) {
System.out.println(s);
}
}
}
运行结果如下:
注意:
1.7 字符串截取
方法 | 功能 |
String substring(int beginIndex)
|
从指定索引截取到结尾
|
String substring(int beginIndex, int endIndex)
|
截取部分内容
|
代码示例:
public class test1 {
public static void main(String[] args) {
String str = "helloworld" ;
System.out.println(str.substring(5));
System.out.println("=======================");
System.out.println(str.substring(0, 4));
}
}
运行结果如下:
1.8 其他操作方法
方法 | 功能 |
String trim()
|
去掉字符串中的左右空格
,
保留中间空格
|
代码示例:
public class test1 {
public static void main(String[] args) {
String str = " hello world " ;
System.out.println("["+str+"]");
System.out.println("["+str.trim()+"]");
}
}
运行结果如下:
注意:trim 会去掉字符串开头和结尾的空白字符(空格, 换行, 制表符等).
2 字符串的不可变性
String是一种不可变对象. 字符串中的内容是不可改变。字符串不可被修改,是因为:
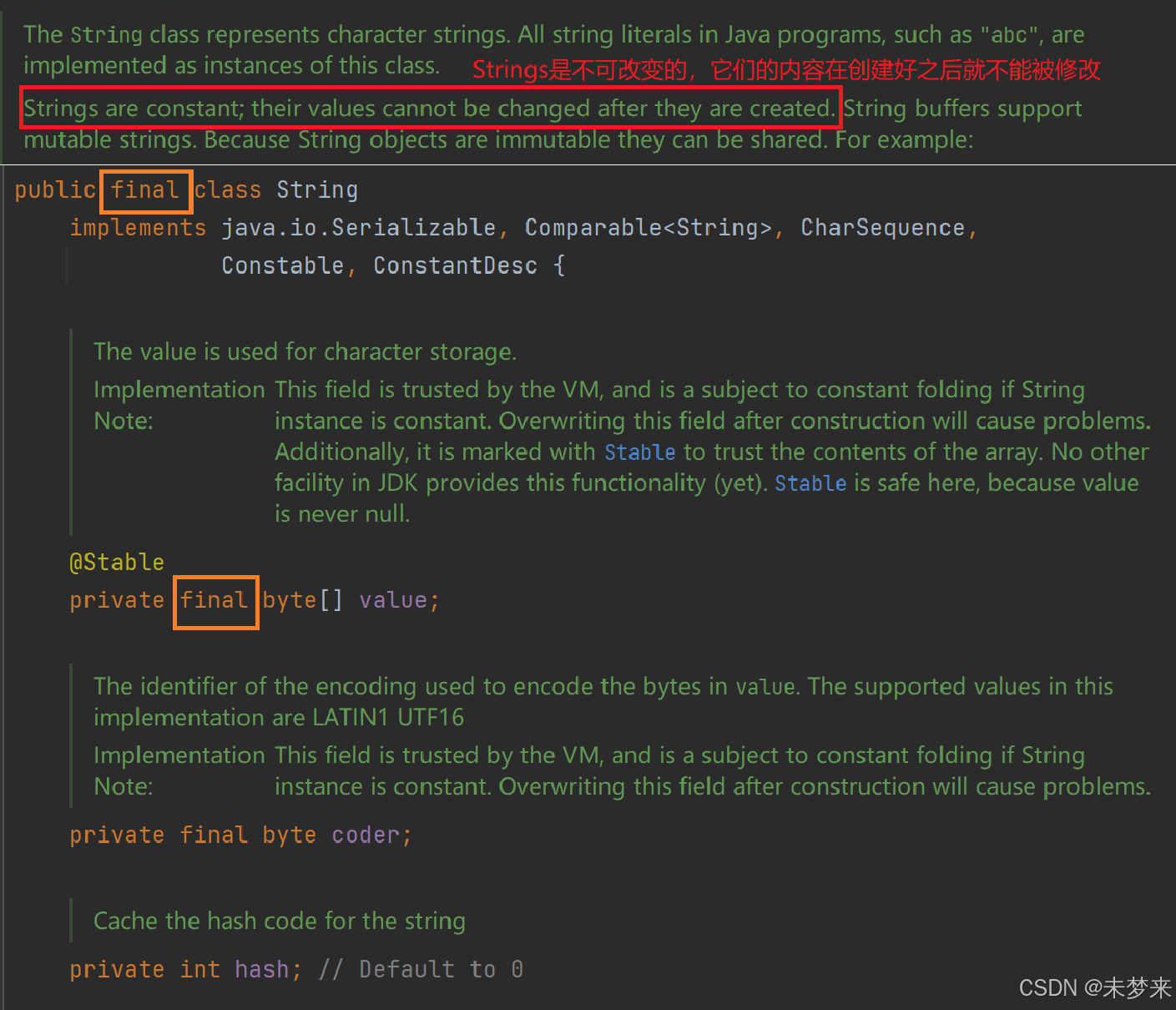
- String类被final修饰,表明该类不能被继承
- value被修饰被final修饰,表明value自身的值不能改变,即不能引用其它字符数组,但是其引用空间中的内容可以修改。
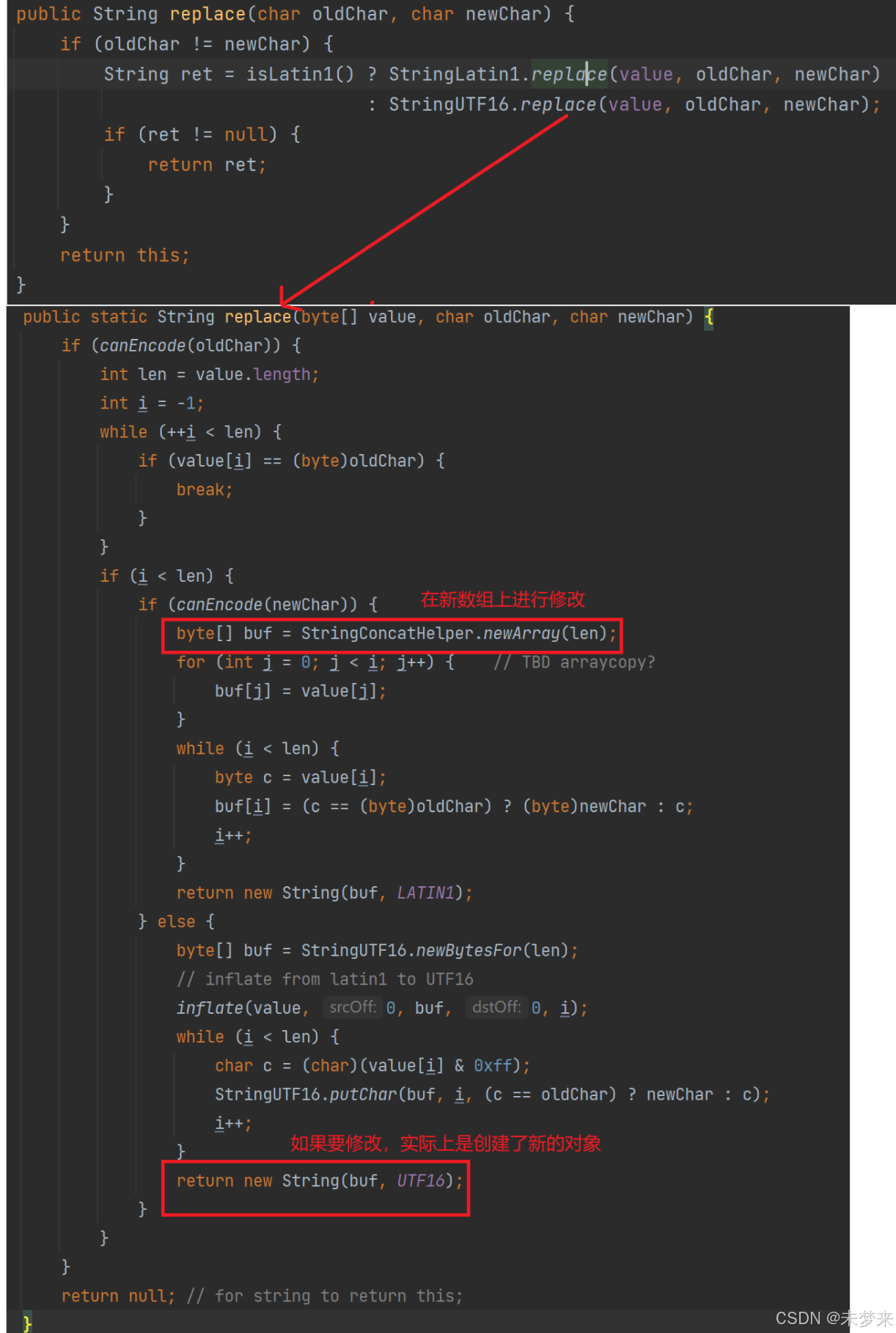
注意:
1. final修饰类表明该类不想被继承,final修饰引用类型表明该引用变量不能引用其他对象,但是其引用对象中的内容是可以修改的。
2. 字符串不可变性的好处
- 方便实现字符串对象池. 如果 String 可变, 那么对象池就需要考虑写时拷贝的问题了.
- 不可变对象是线程安全的.
- 不可变对象更方便缓存 hash code, 作为 key 时可以更高效的保存到 HashMap 中.
3.尽量避免直接对String类型对象进行修改,因为String类是不能修改的,所有的修改都会创建新对象,效率非常低下。
3.StringBuffer 和 StringBuilder
3.1 常用方法
方法 | 功能 |
StringBuff append(String str)
|
在尾部追加,相当于
String
的
+=
,可以追加:
boolean
、
char
、
char[]
、double、
float
、
int
、
long
、
Object
、
String
、
StringBuff
的变量
|
char charAt(int index)
|
获取
index
位置的字符
|
int length()
| 获取字符串的长度 |
int capacity()
| 获取底层保存字符串空间总的大小 |
void ensureCapacity(int
mininmumCapacity)
| 扩容 |
void setCharAt(int index, char ch)
|
将
index
位置的字符设置为
ch
|
int indexOf(String str)
| 返回str第一次出现的位置 |
nt indexOf(String str, int
fromIndex)
| 从fromIndex位置开始查找str第一次出现的位置 |
int lastIndexOf(String str)
|
返回最后一次出现
str
的位置
|
int lastIndexOf(String str, int fromIndex)
|
从
fromIndex
位置开始找
str
最后一次出现的位置
|
StringBuff insert(int
offset, String str)
|
在
offset
位置插入:八种基类类型
& String
类型
& Object
类型数据
|
StringBuffer
deleteCharAt(int index)
|
删除
index
位置字符
|
StringBuffer delete(int
start, int end)
|
删除
[start, end)
区间内的字符
|
StringBuffer replace(int
start, int end, String str)
|
将
[start, end)
位置的字符替换为
str
|
String substring(int start)
|
从
start
开始一直到末尾的字符以
String
的方式返回
|
String substring(int
start
,
int end)
|
将
[start, end)
范围内的字符以
String
的方式返回
|
StringBuffer reverse()
|
反转字符串
|
String toString()
| 将所有字符按照String的方式返回 |
代码示例:
public class test1 {
public static void main(String[] args) {
StringBuilder sb1 = new StringBuilder("hello");
StringBuilder sb2 = sb1;
// 追加:即尾插-->字符、字符串、整形数字
sb1.append(' ');
sb1.append("world");
sb1.append(123);
System.out.println(sb1);
System.out.println(sb1 == sb2);
System.out.println(sb1.charAt(0)); // 获取0号位上的字符 h
System.out.println(sb1.length()); // 获取字符串的有效长度14
System.out.println(sb1.capacity()); // 获取底层数组的总大小
sb1.setCharAt(0, 'H'); // 设置任意位置的字符 Hello world123
sb1.insert(0, "Hello world!!!"); // Hello world!!!Hello world123
System.out.println(sb1);
System.out.println(sb1.indexOf("Hello")); // 获取Hello第一次出现的位置
System.out.println(sb1.lastIndexOf("hello")); // 获取hello最后一次出现的位置
sb1.deleteCharAt(0); // 删除首字符
sb1.delete(0,5); // 删除[0, 5)范围内的字符
String str = sb1.substring(0, 5); // 截取[0, 5)区间中的字符以String的方式返回
System.out.println(str);
sb1.reverse(); // 字符串逆转
str = sb1.toString(); // 将StringBuffer以String的方式返回
System.out.println(str);
}
}
运行结果如下:
3.2 String 、StringBuffer 和 Stringbuilder 的区别
- String的内容不可修改,StringBuffer与StringBuilder的内容可以修改.
- StringBuffer与StringBuilder大部分功能是相似的
- StringBuffer采用同步处理,属于线程安全操作;而StringBuilder未采用同步处理,属于线程不安全操作
本文是作者学习后的总结,如果有什么不恰当的地方,欢迎大佬指正!!!