参考:
https://blog.csdn.net/A_Common_Man/article/details/124601367
App.vue
<template>
<div id="app">
<div class = 'container'>
<h4 style="text-align: center; margin-top: 20px">资产管理</h4>
<table class="table">
<thead>
<tr>
<th>编号</th>
<th>资产名称</th>
<th>价格</th>
<th>创建时间</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr v-for="(item, index) in list" :key="index">
<th>{{ item.id }}</th>
<td>{{ item.name }}</td>
<td>{{ item.price }}</td>
<td>{{ item.time }}</td>
<td><button type="button" class="btn btn-danger" @click="delGoods(index)">删除</button></td>
</tr>
<tr v-if="list.length == 0" style="text-align: center">
<td colspan="5" style="text-align: center;">暂无数据</td>
</tr>
</tbody>
<tfoot v-if="list.length">
<tr>
<td colspan="5" style="text-align: right;">总价值:{{ getSum }} 平均价值:{{ getAvg }}</td>
</tr>
</tfoot>
</table>
<form class="form-inline">
<div class="mb-3">
<input class="form-control" v-model="good.name" placeholder="资产名称">
</div>
<div class="mb-3">
<input class="form-control" v-model="good.price" placeholder="资产价值">
</div>
<div class="mb-3 form-check">
<input type="checkbox" class="form-check-input" id="exampleCheck1">
<label class="form-check-label" for="exampleCheck1">Check me out</label>
</div>
<button type="submit" class="btn btn-primary" @click.prevent="addGoods">添加资产</button>
</form>
</div>
</div>
</template>
<script>
import dayjs from 'dayjs';
import 'bootstrap/dist/css/bootstrap.css'
export default {
data() {
return {
good: {
name: '',
price: '',
},
list: [
{ id: 1, name: "外套", price: 199, time: "2010-08-12" },
{ id: 2, name: "裤子", price: 34, time: "2013-09-01" },
{ id: 3, name: "鞋", price: 25.4, time: "2018-11-22" },
{ id: 4, name: "头发", price: 19900, time: "2020-12-12" },
{ id: 5, name: "帽子", price: 49.99, time: "2012-05-15" },
{ id: 6, name: "衬衫", price: 75, time: "2017-03-21" },
{ id: 7, name: "手套", price: 19.99, time: "2019-11-01" },
{ id: 8, name: "围巾", price: 59.99, time: "2016-12-25" },
{ id: 9, name: "袜子", price: 9.99, time: "2014-08-30" },
{ id: 10, name: "皮带", price: 39.99, time: "2015-07-04" }
]
};
},
methods: {
delGoods(index) {
this.list.splice(index, 1);
for(let i = 0; i < this.list.length; i++) {
this.list[i].id = i+1;
}
},
addGoods() {
const {list, good} = this;
const id = list.length+1;
if(good.name == '' || good.price == '') return alert('输入有空,重新输入')
let object = {
id : id,
name: good.name,
price: good.price,
time: dayjs(new Date()).format('YYYY-MM-DD')
}
list.push(object);
good.name = ''
good.price = ''
}
},
computed: {
getSum() {
let sum = this.list.reduce((sum, item) => sum + item.price, 0)
return parseFloat(sum).toFixed(2)
},
getAvg() {
let avg = this.getSum
avg /= this.list.length
return parseFloat(avg).toFixed(2)
}
}
}
</script>
<style>
</style>
效果预览
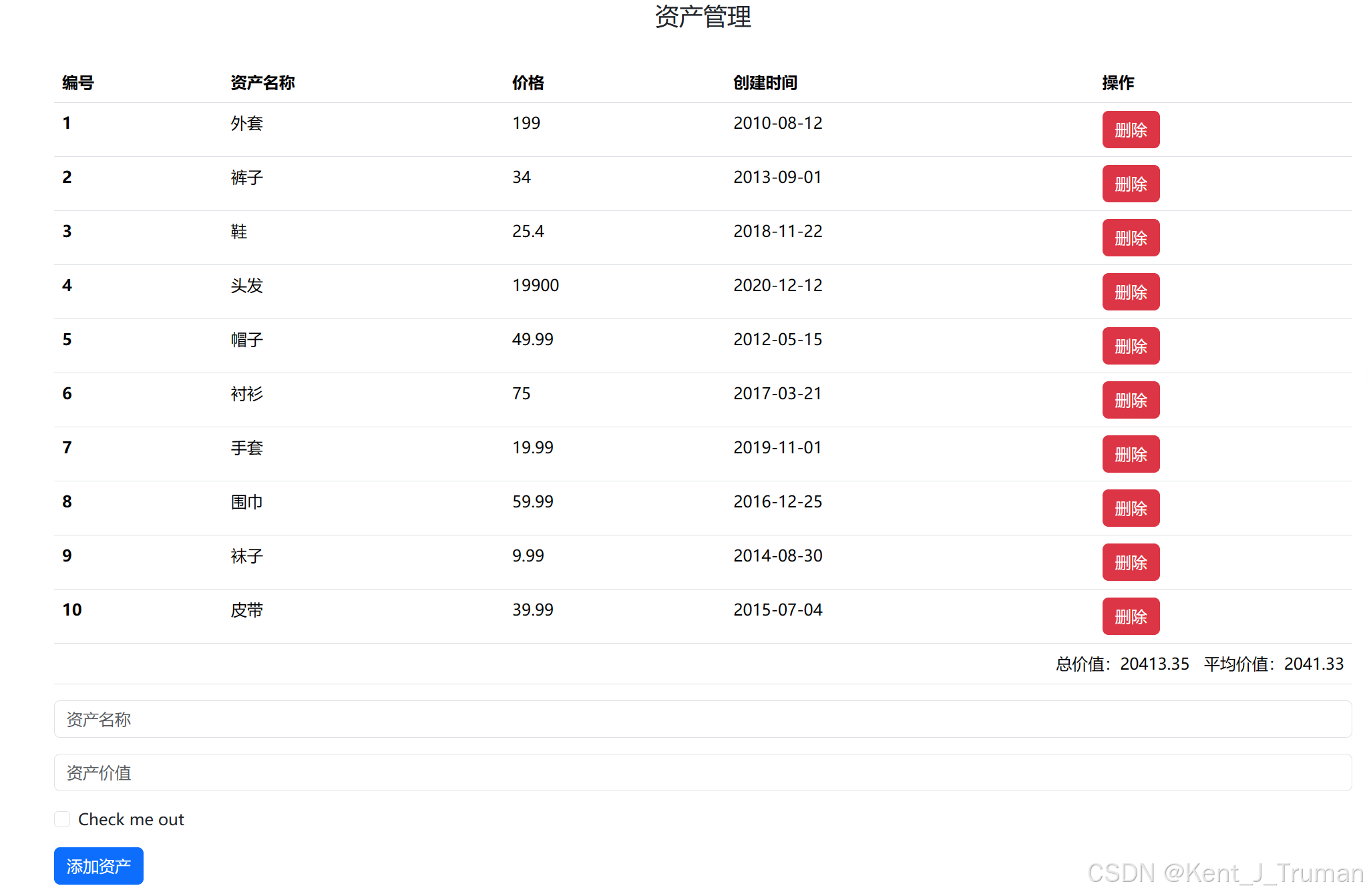
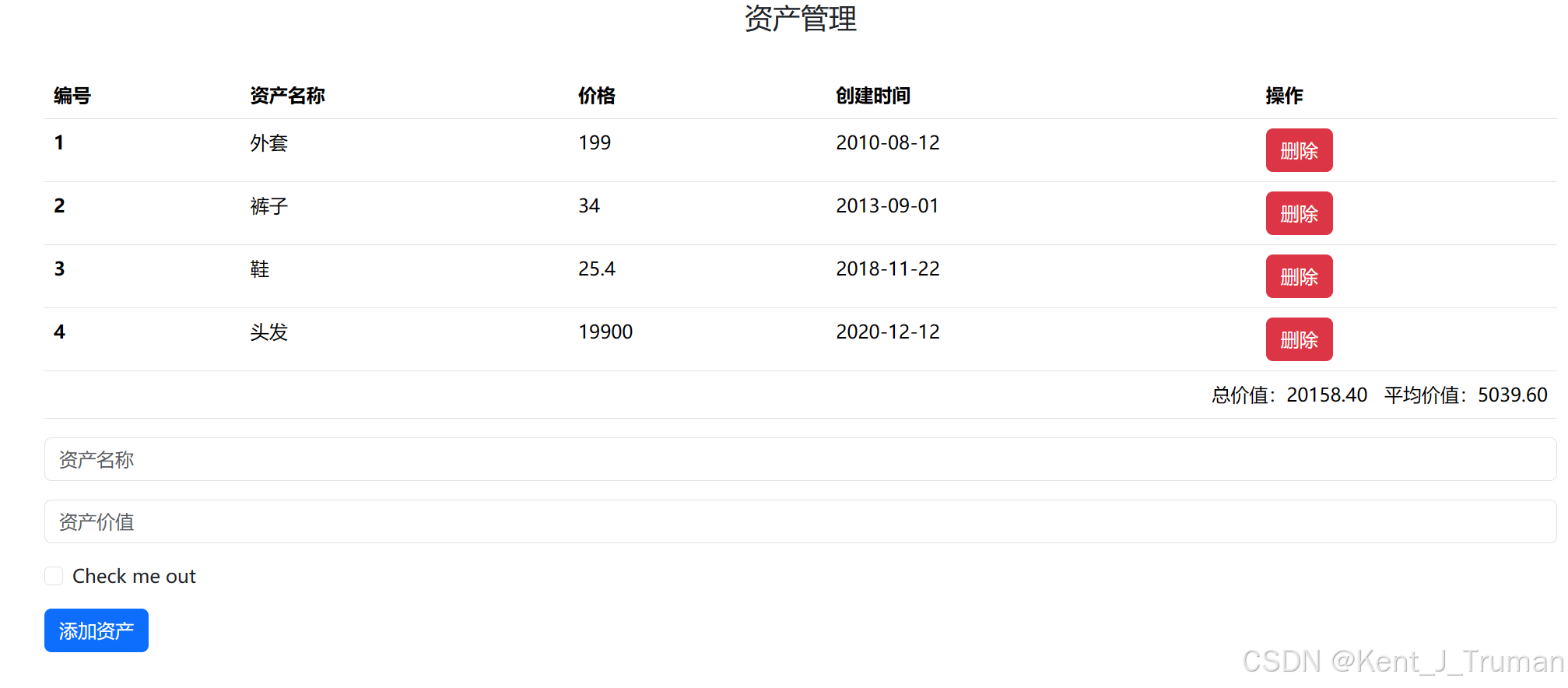
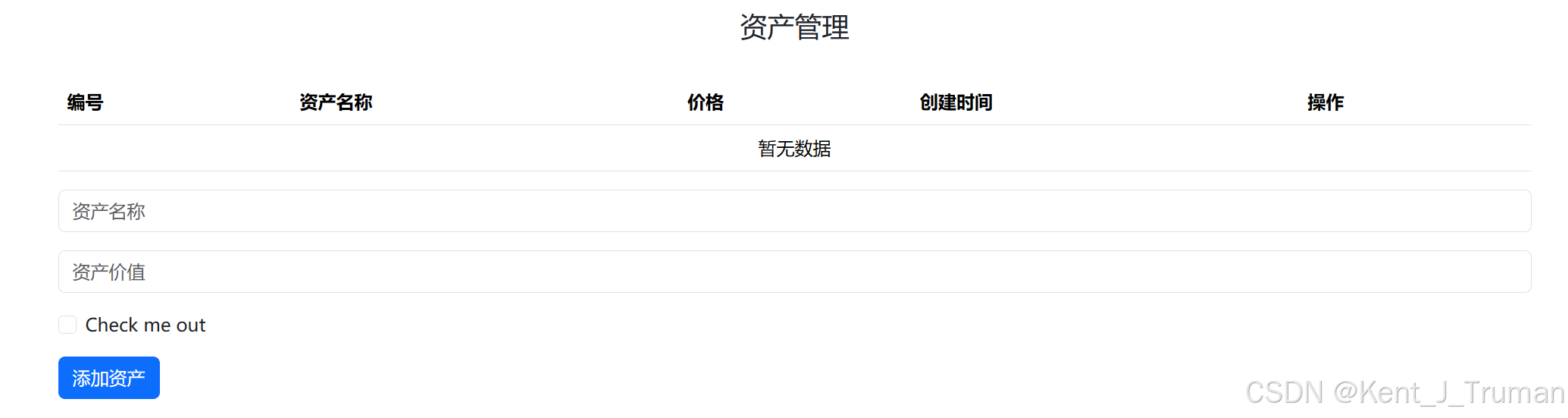
