基础图形的绘制
- paintarea
-
- paintex
-
- main.cpp
- 运行图
paintarea
paintarea.h
#ifndef PAINTAREA_H
#define PAINTAREA_H
#include <QWidget>
#include <QBrush>
#include <QPen>
#include <QPainter>
#include <QPainterPath>
class PaintArea : public QWidget
{
Q_OBJECT
public:
enum Shape{Line,Rectangle,RoundRect,Ellipse,Polygon,PolyLine,Points,Arc,Path,Text,Pixmap};
explicit PaintArea(QWidget *parent = nullptr);
void SetShape(Shape);
void SetPen(QPen);
void SetBrush(QBrush);
void SetFillRule(Qt::FillRule);
void paintEvent(QPaintEvent*);
signals:
private:
Shape shape;
QPen pen;
QBrush brush;
Qt::FillRule FillRule;
};
#endif
paintarea.cpp
#include "paintarea.h"
PaintArea::PaintArea(QWidget *parent)
: QWidget{parent}
{
setPalette(QPalette(Qt::white));
setAutoFillBackground(true);
setMinimumSize(400,400);
}
void PaintArea::SetShape(Shape s)
{
shape =s;
update();
}
void PaintArea::SetPen(QPen p)
{
pen = p;
update();
}
void PaintArea::SetBrush(QBrush b)
{
brush = b;
update();
}
void PaintArea::SetFillRule(Qt::FillRule Rule)
{
FillRule = Rule;
update();
}
void PaintArea::paintEvent(QPaintEvent *)
{
QPainter p(this);
p.setPen(pen);
p.setBrush(brush);
QRect Rect(50,100,300,200);
static const QPoint points[4] =
{
QPoint(150,100),
QPoint(300,150),
QPoint(350,250),
QPoint(100,300)
};
int StartAngle = 30*16;
int SpanAngle = 120*16;
QPainterPath path;
path.addRect(150,150,100,100);
path.moveTo(100,100);
path.cubicTo(300,100,200,200,300,300);
path.cubicTo(100,300,200,200,100,100);
path.setFillRule(FillRule);
switch(shape)
{
case Line:
{
p.drawLine(Rect.topLeft(),Rect.bottomRight());
break;
}
case Rectangle:
{
p.drawRect(Rect);
break;
}
case RoundRect:
{
p.drawRoundedRect(Rect,20,20);
break;
}
case Ellipse:
{
p.drawEllipse(Rect);
break;
}
case Polygon:
{
p.drawPolygon(Rect);
break;
}
case Points:
{
p.drawPoints(points,4);
break;
}
case Arc:
{
p.drawArc(Rect,StartAngle,SpanAngle);
break;
}
case Path:
{
p.drawPath(path);
break;
}
case Text:
{
p.drawText(Rect,Qt::AlignCenter,tr("第一"));
break;
}
case Pixmap:
{
p.drawPixmap(150,150,QPixmap("312.ico"));
break;
}
default:break;
}
}
paintex
paintex.h
#ifndef PAINTEX_H
#define PAINTEX_H
#include <QWidget>
#include "paintarea.h"
#include <QLabel>
#include <QComboBox>
#include <QSpinBox>
#include <QPushButton>
#include <QGridLayout>
#include <QGradient>
class PaintEx : public QWidget
{
Q_OBJECT
public:
PaintEx(QWidget *parent = nullptr);
~PaintEx();
private:
PaintArea* paintArea;
QLabel* ShapeLabel;
QComboBox* shapeComboBox;
QLabel* PenWidthLabel;
QSpinBox* PenWidthSpinBox;
QLabel* PenColorLabel;
QFrame* PenColorFrame;
QPushButton* PenColorBtn;
QLabel* PenStyleLabel;
QComboBox* PenStyleComboBox;
QLabel* PenCapLabel;
QComboBox* PenCapComboBox;
QLabel* PenJoinLabel;
QComboBox* PenJoinComboBox;
QLabel* FillRuleLabel;
QComboBox* FillRuleComboBox;
QLabel* SpreadLabel;
QComboBox* SpreadComboBox;
QGradient::Spread spread;
QLabel* BrushStyleLabel;
QComboBox *BrushStyleComboBox;
QLabel* BrushColorLabel;
QFrame* BrushColorFrame;
QPushButton* BrushColorBtn;
QGridLayout* RightLayout;
protected slots:
void ShowShape(int);
void ShowPenWidth(int);
void ShowPenColor();
void ShowPenStyle(int);
void ShowPenCap(int);
void ShowPenJoin(int);
void ShowSpreadStyle();
void ShowFillRule();
void ShowBrushColor();
void ShowBrush(int);
};
#endif
paintex.cpp
#include "paintex.h"
#include <QColorDialog>
PaintEx::PaintEx(QWidget *parent)
: QWidget(parent)
{
paintArea = new PaintArea;
ShapeLabel = new QLabel(tr("形状:"));
shapeComboBox =new QComboBox;
shapeComboBox->addItem(tr("Line"),PaintArea::Line);
shapeComboBox->addItem(tr("Rectangle"),PaintArea::Rectangle);
shapeComboBox->addItem(tr("RoundedRect"),PaintArea::RoundRect);
shapeComboBox->addItem(tr("Ellipse"),PaintArea::Ellipse);
shapeComboBox->addItem(tr("Polygon"),PaintArea::Polygon);
shapeComboBox->addItem(tr("PolyLine"),PaintArea::PolyLine);
shapeComboBox->addItem(tr("Points"),PaintArea::Points);
shapeComboBox->addItem(tr("Arc"),PaintArea::Arc);
shapeComboBox->addItem(tr("Path"),PaintArea::Path);
shapeComboBox->addItem(tr("Text"),PaintArea::Text);
shapeComboBox->addItem(tr("Pixmap"),PaintArea::Pixmap);
connect(shapeComboBox, static_cast<void (QComboBox::*)(int)>(&QComboBox::activated),
this, &PaintEx::ShowShape);
PenColorLabel = new QLabel("画笔颜色:");
PenColorFrame = new QFrame;
PenColorFrame->setAutoFillBackground(true);
PenColorFrame->setFrameStyle(QFrame::Panel|QFrame::Sunken);
PenColorFrame->setPalette(QPalette(Qt::blue));
PenColorBtn = new QPushButton(tr("更改"));
connect(PenColorBtn,&QPushButton::clicked,this,&PaintEx::ShowPenColor);
PenWidthLabel = new QLabel(tr("画笔线宽|:"));
PenWidthSpinBox = new QSpinBox;
PenWidthSpinBox->setRange(0,20);
connect(PenWidthSpinBox,static_cast<void (QSpinBox::*)(int)>(&QSpinBox::valueChanged),this,&PaintEx::ShowPenWidth);
PenStyleLabel = new QLabel(tr("画笔风格:"));
PenStyleComboBox = new QComboBox;
PenStyleComboBox->addItem(tr("SolidLine"),static_cast<int>(Qt::SolidLine));
PenStyleComboBox->addItem(tr("DashLine"),static_cast<int>(Qt::DashLine));
PenStyleComboBox->addItem(tr("DotLine"),static_cast<int>(Qt::DotLine));
PenStyleComboBox->addItem(tr("DashDotLine"),static_cast<int>(Qt::DashDotDotLine));
PenStyleComboBox->addItem(tr("DashDotDotLine"),static_cast<int>(Qt::DashDotDotLine));
PenStyleComboBox->addItem(tr("CustomDashLine"),static_cast<int>(Qt::CustomDashLine));
connect(PenStyleComboBox, static_cast<void (QComboBox::*)(int)>(&QComboBox::activated),
this, &PaintEx::ShowPenStyle);
PenCapLabel = new QLabel(tr("画笔顶帽:"));
PenCapComboBox = new QComboBox;
PenCapComboBox->addItem(tr("SquareCap"),Qt::SquareCap);
PenCapComboBox->addItem(tr("FlatCap"),Qt::FlatCap);
PenCapComboBox->addItem(tr("RoundCap"),Qt::RoundCap);
connect(PenCapComboBox,static_cast<void (QComboBox::*)(int)>(&QComboBox::activated),
this, &PaintEx::ShowPenCap);
PenJoinLabel = new QLabel(tr("画笔连接点:"));
PenJoinComboBox = new QComboBox;
PenJoinComboBox->addItem(tr("BevelJoin"),Qt::BevelJoin);
PenJoinComboBox->addItem(tr("MiterJoin"),Qt::MiterJoin);
PenJoinComboBox->addItem(tr("RoundJoin"),Qt::RoundJoin);
connect(PenJoinComboBox,static_cast<void (QComboBox::*)(int)>(&QComboBox::activated),
this, &PaintEx::ShowPenJoin);
FillRuleLabel = new QLabel(tr("填充模式:"));
FillRuleComboBox = new QComboBox;
FillRuleComboBox->addItem(tr("Old Even"),Qt::OddEvenFill);
FillRuleComboBox->addItem(tr("Winding"),Qt::WindingFill);
connect(FillRuleComboBox,static_cast<void (QComboBox::*)(int)>(&QComboBox::activated),
this, &PaintEx::ShowFillRule);
SpreadLabel = new QLabel(tr("铺展效果:"));
SpreadComboBox = new QComboBox;
SpreadComboBox->addItem(tr("PadSpread"),QGradient::PadSpread);
SpreadComboBox->addItem(tr("Repeat"),QGradient::RepeatSpread);
SpreadComboBox->addItem(tr("ReflectSpread"),QGradient::ReflectSpread);
connect(SpreadComboBox,static_cast<void (QComboBox::*)(int)>(&QComboBox::activated),
this, &PaintEx::ShowSpreadStyle);
BrushColorLabel = new QLabel(tr("画刷颜色:"));
BrushColorFrame = new QFrame;
BrushColorFrame->setFrameStyle(QFrame::Panel|QFrame::Sunken);
BrushColorFrame->setAutoFillBackground(true);
BrushColorFrame->setPalette(QPalette(Qt::green));
BrushColorBtn = new QPushButton(tr("更改"));
connect(BrushColorBtn,&QPushButton::clicked,this,&PaintEx::ShowBrushColor);
BrushStyleLabel = new QLabel(tr("画刷风格:"));
BrushStyleComboBox = new QComboBox;
BrushStyleComboBox->addItem(tr("SolidPattern"),static_cast<int>(Qt::SolidPattern));
BrushStyleComboBox->addItem(tr("Dense1Pattern"),static_cast<int>(Qt::Dense1Pattern));
BrushStyleComboBox->addItem(tr("Dense2Pattern"),static_cast<int>(Qt::Dense2Pattern));
BrushStyleComboBox->addItem(tr("Dense3Pattern"),static_cast<int>(Qt::Dense3Pattern));
BrushStyleComboBox->addItem(tr("Dense4Pattern"),static_cast<int>(Qt::Dense4Pattern));
BrushStyleComboBox->addItem(tr("Dense5Pattern"),static_cast<int>(Qt::Dense5Pattern));
BrushStyleComboBox->addItem(tr("Dense6Pattern"),static_cast<int>(Qt::Dense6Pattern));
BrushStyleComboBox->addItem(tr("Dense7Pattern"),static_cast<int>(Qt::Dense7Pattern));
BrushStyleComboBox->addItem(tr("HorPattern"),static_cast<int>(Qt::HorPattern));
BrushStyleComboBox->addItem(tr("VerPattern"),static_cast<int>(Qt::VerPattern));
BrushStyleComboBox->addItem(tr("CrossPattern"),static_cast<int>(Qt::CrossPattern));
BrushStyleComboBox->addItem(tr("BDiagPattern"),static_cast<int>(Qt::BDiagPattern));
BrushStyleComboBox->addItem(tr("FDiagPattern"),static_cast<int>(Qt::FDiagPattern));
BrushStyleComboBox->addItem(tr("DiagCrossPattern"),static_cast<int>(Qt::DiagCrossPattern));
BrushStyleComboBox->addItem(tr("LinearGradientPattern"),static_cast<int>(Qt::LinearGradientPattern));
BrushStyleComboBox->addItem(tr("ConicalGradientPattern"),static_cast<int>(Qt::ConicalGradientPattern));
BrushStyleComboBox->addItem(tr("RadialGradientPattern"),static_cast<int>(Qt::RadialGradientPattern));
BrushStyleComboBox->addItem(tr("TexturePattern"),static_cast<int>(Qt::TexturePattern));
connect(BrushStyleComboBox,static_cast<void (QComboBox::*)(int)>(&QComboBox::activated),
this, &PaintEx::ShowBrush);
RightLayout = new QGridLayout;
RightLayout->addWidget(ShapeLabel,0,0);
RightLayout->addWidget(shapeComboBox,0,1);
RightLayout->addWidget(PenColorLabel,1,0);
RightLayout->addWidget(PenColorFrame,1,1);
RightLayout->addWidget(PenColorBtn,1,2);
RightLayout->addWidget(PenWidthLabel,2,0);
RightLayout->addWidget(PenWidthSpinBox,2,1);
RightLayout->addWidget(PenStyleLabel,3,0);
RightLayout->addWidget(PenStyleComboBox,3,1);
RightLayout->addWidget(PenCapLabel,4,0);
RightLayout->addWidget(PenCapComboBox,4,1);
RightLayout->addWidget(PenJoinLabel,5,0);
RightLayout->addWidget(PenJoinComboBox,5,1);
RightLayout->addWidget(FillRuleLabel,6,0);
RightLayout->addWidget(FillRuleComboBox,6,1);
RightLayout->addWidget(SpreadLabel,7,0);
RightLayout->addWidget(SpreadComboBox,7,1);
RightLayout->addWidget(BrushColorLabel,8,0);
RightLayout->addWidget(BrushColorFrame,8,1);
RightLayout->addWidget(BrushColorBtn,8,2);
RightLayout->addWidget(BrushStyleLabel,9,0);
RightLayout->addWidget(BrushStyleComboBox,9,1);
QHBoxLayout* MainLayout = new QHBoxLayout(this);
MainLayout->addWidget(paintArea);
MainLayout->addLayout(RightLayout);
MainLayout->setStretchFactor(paintArea,1);
MainLayout->setStretchFactor(RightLayout,0);
ShowShape(shapeComboBox->currentIndex());
}
PaintEx::~PaintEx() {}
void PaintEx::ShowShape(int value)
{
PaintArea::Shape shape = PaintArea::Shape(shapeComboBox->itemData(value,Qt::UserRole).toInt());
paintArea->SetShape(shape);
}
void PaintEx::ShowPenWidth(int nValue)
{
QColor Color = PenColorFrame->palette().color(QPalette::Window);
Qt::PenStyle Style = Qt::PenStyle(PenStyleComboBox->itemData(PenStyleComboBox->currentIndex(),Qt::UserRole).toInt());
Qt::PenCapStyle Cap = Qt::PenCapStyle(PenCapComboBox->itemData(PenCapComboBox->currentIndex(),Qt::UserRole).toInt());
Qt::PenJoinStyle Join = Qt::PenJoinStyle(PenJoinComboBox->itemData(PenJoinComboBox->currentIndex(),Qt::UserRole).toInt());
paintArea->SetPen(QPen(Color,nValue,Style,Cap,Join));
}
void PaintEx::ShowPenColor()
{
QColor Color = QColorDialog::getColor(static_cast<int>(Qt::blue));
PenColorFrame->setPalette(QPalette(Color));
int nValue = PenWidthSpinBox->value();
Qt::PenStyle Style = Qt::PenStyle(PenStyleComboBox->itemData(PenStyleComboBox->currentIndex(),Qt::UserRole).toInt());
Qt::PenCapStyle Cap = Qt::PenCapStyle(PenCapComboBox->itemData(PenCapComboBox->currentIndex(),Qt::UserRole).toInt());
Qt::PenJoinStyle Join = Qt::PenJoinStyle(PenJoinComboBox->itemData(PenJoinComboBox->currentIndex(),Qt::UserRole).toInt());
paintArea->SetPen(QPen(Color,nValue,Style,Cap,Join));
}
void PaintEx::ShowPenStyle(int StyleValue)
{
QColor Color = PenColorFrame->palette().color(QPalette::Window);
int nValue = PenWidthSpinBox->value();
Qt::PenStyle Style = Qt::PenStyle(PenStyleComboBox->itemData(StyleValue,Qt::UserRole).toInt());
Qt::PenCapStyle Cap = Qt::PenCapStyle(PenCapComboBox->itemData(PenCapComboBox->currentIndex(),Qt::UserRole).toInt());
Qt::PenJoinStyle Join = Qt::PenJoinStyle(PenJoinComboBox->itemData(PenJoinComboBox->currentIndex(),Qt::UserRole).toInt());
paintArea->SetPen(QPen(Color,nValue,Style,Cap,Join));
}
void PaintEx::ShowPenCap(int capValue)
{
QColor Color = PenColorFrame->palette().color(QPalette::Window);
int nValue = PenWidthSpinBox->value();
Qt::PenStyle Style = Qt::PenStyle(PenStyleComboBox->itemData(PenStyleComboBox->currentIndex(),Qt::UserRole).toInt());
Qt::PenCapStyle Cap = Qt::PenCapStyle(PenCapComboBox->itemData(capValue,Qt::UserRole).toInt());
Qt::PenJoinStyle Join = Qt::PenJoinStyle(PenJoinComboBox->itemData(PenJoinComboBox->currentIndex(),Qt::UserRole).toInt());
paintArea->SetPen(QPen(Color,nValue,Style,Cap,Join));
}
void PaintEx::ShowPenJoin(int JoinValue)
{
QColor Color = PenColorFrame->palette().color(QPalette::Window);
int nValue = PenWidthSpinBox->value();
Qt::PenStyle Style = Qt::PenStyle(PenStyleComboBox->itemData(PenStyleComboBox->currentIndex(),Qt::UserRole).toInt());
Qt::PenCapStyle Cap = Qt::PenCapStyle(PenCapComboBox->itemData(PenCapComboBox->currentIndex(),Qt::UserRole).toInt());
Qt::PenJoinStyle Join = Qt::PenJoinStyle(PenJoinComboBox->itemData(JoinValue,Qt::UserRole).toInt());
paintArea->SetPen(QPen(Color,nValue,Style,Cap,Join));
}
void PaintEx::ShowSpreadStyle()
{
spread = QGradient::Spread(SpreadComboBox->itemData(SpreadComboBox->currentIndex(),Qt::UserRole).toInt());
}
void PaintEx::ShowFillRule()
{
Qt::FillRule rule =Qt::FillRule(FillRuleComboBox->itemData(FillRuleComboBox->currentIndex(),Qt::UserRole).toInt());
paintArea->SetFillRule(rule);
}
void PaintEx::ShowBrushColor()
{
QColor Color = QColorDialog::getColor(static_cast<int>(Qt::blue));
BrushColorFrame->setPalette(QPalette(Color));
ShowBrush(BrushStyleComboBox->currentIndex());
}
void PaintEx::ShowBrush(int value)
{
QColor Color = BrushColorFrame->palette().color(QPalette::Window);
Qt::BrushStyle style = Qt::BrushStyle(BrushStyleComboBox->itemData(value,Qt::UserRole).toInt());
if(style == Qt::LinearGradientPattern)
{
QLinearGradient LinearGradient(0,0,400,400);
LinearGradient.setColorAt(0.0,Qt::white);
LinearGradient.setColorAt(0.2,Color);
LinearGradient.setColorAt(1.0,Qt::black);
LinearGradient.setSpread(spread);
paintArea->SetBrush(LinearGradient);
}
else if(style == Qt::RadialGradientPattern)
{
QRadialGradient RadialGradient(200,200,150,150,100);
RadialGradient.setColorAt(0.0,Qt::white);
RadialGradient.setColorAt(0.2,Color);
RadialGradient.setColorAt(1.0,Qt::black);
RadialGradient.setSpread(spread);
paintArea->SetBrush(RadialGradient);
}
else if(style == Qt::ConicalGradientPattern)
{
QConicalGradient conicalGradient(200,200,30);
conicalGradient.setColorAt(0.0,Qt::white);
conicalGradient.setColorAt(0.2,Color);
conicalGradient.setColorAt(1.0,Qt::black);
conicalGradient.setSpread(spread);
paintArea->SetBrush(conicalGradient);
}
else if(style == Qt::TexturePattern)
{
paintArea->SetBrush(QBrush(QPixmap("312.png")));
}
else
{
paintArea->SetBrush(QBrush(Color,style));
}
}
main.cpp
#include "paintex.h"
#include <QApplication>
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
PaintEx w;
w.show();
return a.exec();
}
运行图
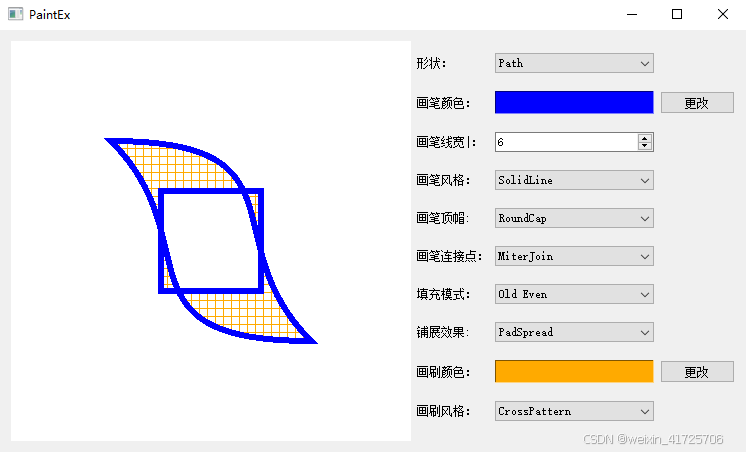