【链表】No. 0148 排序链表【中等】👉力扣对应题目指路
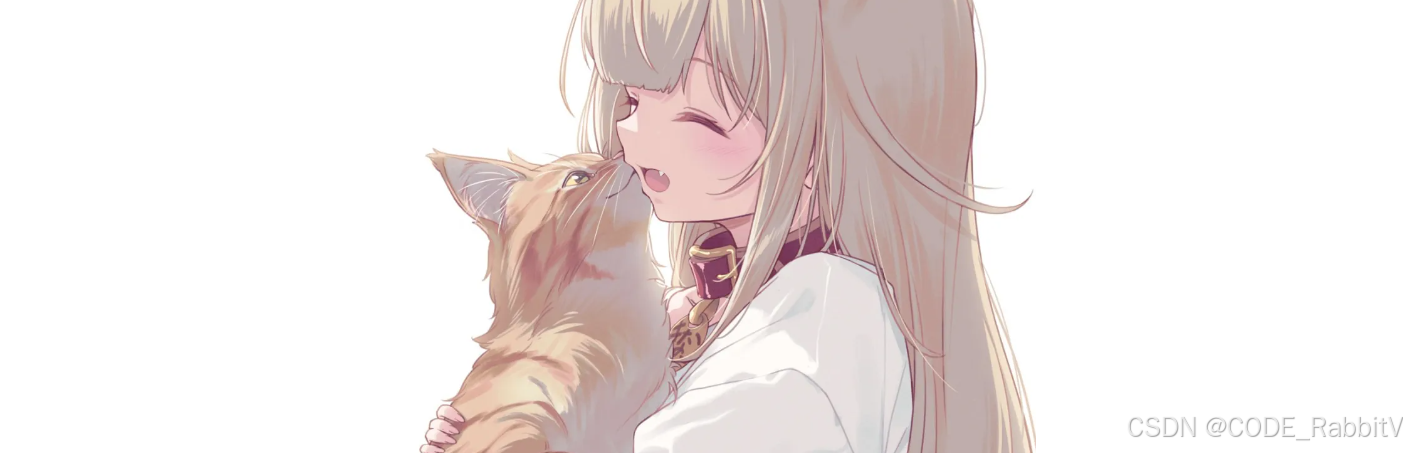
希望对你有帮助呀!!💜💜 如有更好理解的思路,欢迎大家留言补充 ~ 一起加油叭 💦
欢迎关注、订阅专栏 【力扣详解】谢谢你的支持!
⭐ 题目描述:给你链表的头结点 head ,请将其按 升序
排列并返回 排序后的链表
-
【难点🔥】在 O(n log n) 时间复杂度和常数级空间复杂度下,对链表进行排序
-
示例 :
输入:head = [4,2,1,3]
输出:[1,2,3,4]
🔥 思路:归并排序(二分链表,逐层合并)
- 可以参考下图理解 (最上面一行是原链表元素,将两两元素按序合并,最终得到最下面一行的有序链表结果)
- 对于本题目的一般解法是用数组存储链表元素值,排序后存回链表【较容易,本文未给出】
⭐⭐⭐ 题目准备之合并两个有序链表: 一定要先掌握合并两个有序链表 ❗❗❗ 放在最后面啦,供不熟悉的友友参考~
参考如上思路,给出详细步骤如下:
步骤一⭐写出合并两个有序链表
h1 h2
的函数merge
备用
- 创建虚拟头节点
dummy_head
, 逐个往后链接h1 h2
中当前val
更小的节点步骤二⭐用双指针法 (慢指针
slow
, 快指针fast
) 将待排序链表均分为两段
fast
两倍于slow
移动,fast
走到头后,slow
走到中部 (作为第二段 💞 的 head →part_2
)
- 慢指针
slow
每次往前走一步:slow = slow.next
- 快指针
fast
每次往前走两步:fast = fast.next.next
- 为了将两段断开,建立
break_point
记录第二段💞前需要断点位置
- 即用
break_point
追踪slow
的前一位置- 注意:如果当前只有一段 (即当前链表只有一个元素,不够分段),返回当前元素,不再往后继续
- 本文用
break_point
来判断的步骤三⭐递归处理(也就是排序)当前的两段链表:
- 如果两段有序链表都非空,则调用
merge
合并- 否则返回对应非空的那段
# Definition for singly-linked list.
# class ListNode:
# def __init__(self, val=0, next=None):
# self.val = val
# self.next = next
class Solution:
def sortList(self, head: Optional[ListNode]) -> Optional[ListNode]:
def traversal_sort(head):
def merge(h1, h2): ## 【合并两个有序链表】 # ---------------- step 1
dummy_head = ListNode()
current = dummy_head
while h1 or h2:
if not h2 or (h1 and h1.val <= h2.val):
current.next = h1
current = h1
h1 = h1.next
else: # not h1 or (h2 and h2.val < h1.val)
current.next = h2
current = h2
h2 = h2.next
return dummy_head.next
# ------------------------------------------------------- step 2
if not head:
return None
slow = head
fast = head
break_point = None
while fast and fast.next:
break_point = slow # --------------------------- step 2.2.1
slow = slow.next # ----------------------------- step 2.1.1
fast = fast.next.next # ------------------------ step 2.1.2
part_1 = head
part_2 = slow
# -------------------------------------------------- step 2.2.2
if not break_point: return head ## 处理只有一个元素的情况,重要!
break_point.next = None ## 断开
# ------------------------------------------------------- step 3
part_1_result = traversal_sort(part_1)
part_2_result = traversal_sort(part_2)
# ----------------------------------------------------- step 3.1
if part_1_result and part_2_result:
return merge(part_1_result, part_2_result)
# ----------------------------------------------------- step 3.2
if part_1_result: return part_1_result
if part_2_result: return part_2_result
return traversal_sort(head)
⭐⭐⭐ 题目准备之合并两个有序链表:一定要先掌握合并两个有序链表 ❗❗❗
# Definition for singly-linked list.
# class ListNode:
# def __init__(self, val=0, next=None):
# self.val = val
# self.next = next
class Solution:
def mergeTwoLists(self, list1: Optional[ListNode], list2: Optional[ListNode]) -> Optional[ListNode]:
dummy_head = ListNode()
current = dummy_head
h1, h2 = list1, list2 ## 为和本文保持一致,换名为 h1 h2
while h1 or h2:
if not h2 or (h1 and h1.val <= h2.val):
current.next = h1
current = h1
h1 = h1.next
else: # not h1 or (h2 and h2.val < h1.val)
current.next = h2
current = h2
h2 = h2.next
return dummy_head.next
希望对你有帮助呀!!💜💜 如有更好理解的思路,欢迎大家留言补充 ~ 一起加油叭 💦
🔥 LeetCode 热题 HOT 100