Introduction
Euchre (pronounced “YOO-kur”) is a card game popular in Michigan.
The learning goals of this project include Abstract Data Types in C++, Derived Classes, Inheritance, and Polymorphism. You’ll gain practice with C++-style Object Oriented Programming (OOP) with classes and virtual functions.
When you’re done, you’ll have a program that simulates a game of Euchre, supporting a AI player and a Human player.
$
./euchre.exe pack.in noshuffle 3 Ivan Human Judea Simple Kunle Simple Liskov Simple
Hand 0
Ivan deals
Jack of Diamonds turned up
Judea passes
Kunle passes
Liskov passes
Human player Ivan's hand: [0] Nine of Diamonds
Human player Ivan's hand: [1] Ten of Diamonds
Human player Ivan's hand: [2] Jack of Hearts
Human player Ivan's hand: [3] Queen of Hearts
Human player Ivan's hand: [4] Ace of Clubs
Human player Ivan, please enter a suit, or "pass":
Diamonds
Ivan orders up Diamonds
...
Setup
Set up your visual debugger and version control, then submit to the autograder.
Visual debugger
During setup, name your project
p3-euchre
. Use this starter files link:
https://eecs280staff.github.io/p3-euchre/starter-files.tar.gz
VS Code
Visual Studio
Xcode
If you created a
main.cpp
while following the setup tutorial, rename it to
euchre.cpp
. Otherwise, create a new file euchre.cpp
. You should end up with a folder with starter files that looks like this.
You may have already renamed files like
Card.cpp.starter
to
Card.cpp
.
$
ls
Card.cpp.starter Pack_public_tests.cpp euchre_test00.out.correct
Card.hpp Pack_tests.cpp.starter euchre_test01.out.correct
Card_public_tests.cpp Player.hpp euchre_test50.in
Card_tests.cpp.starter Player_public_tests.cpp euchre_test50.out.correct
Makefile Player_tests.cpp.starter pack.in
Pack.hpp euchre.cpp unit_test_framework.hpp
Here’s a short description of each starter file.
File(s)
Description
Card.hpp
Abstraction representing a playing card.
Card.cpp.starter
Starter code for the
Card
.
Card_tests.cpp
Your
Card
unit tests.
Card_public_tests.cpp
Compile check test for
Card.cpp
.
Pack.hpp
Abstraction representing a pack card
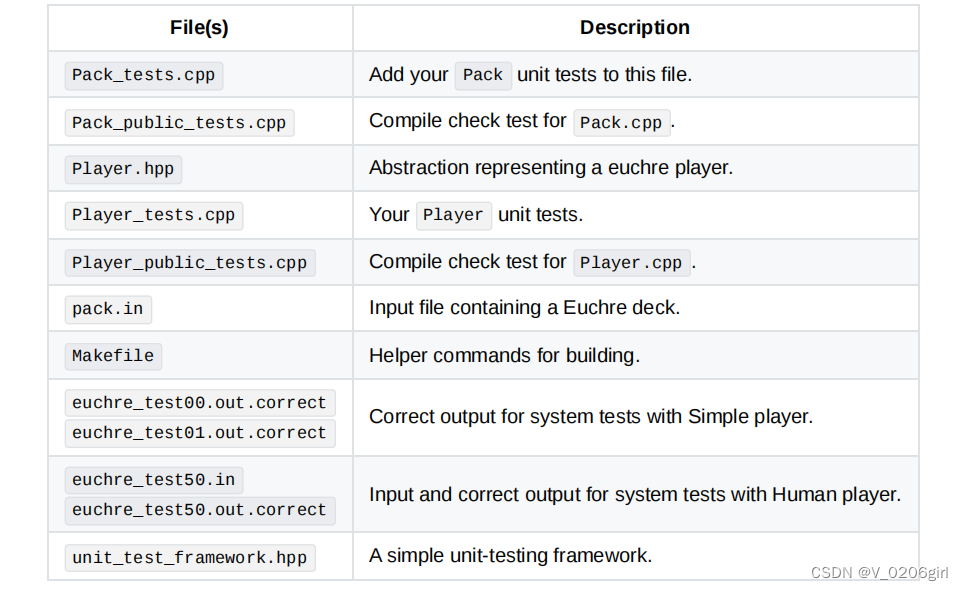