一.vue中的$nextTick
简述与用法:这是一个生命周期钩子
1.语法:this.$nextTick(回调函数)
2.作用:在下一次DOM更新结束后执行其指定的回调
3.什么时候用:当修改数据后,要基于更新后的新dom进行某些操作时,要在nextTick所指定的回调函数中执行
在之前的博客中我们优化了很多次的Todo-List案例,今天我们使用 $nextTick 优化 Todo-List
代码如下:
//src/components/MyItem.vue
<template>
<li>
<label>
<input type="checkbox" :checked="todo.done" @change="handleCheck(todo.id)"/>
<span v-show="!todo.isEdit">{{ todo.title }}</span>
<input type="text" v-show="todo.isEdit" :value="todo.title"
@blur="handleBlur(todo, $event)" ref="inputTitle"/>
</label>
<button class="btn btn-danger" @click="handleDelete(todo.id)">删除</button>
<button v-show="!todo.isEdit" class="btn btn-edit" @click="handleEdit(todo)">
编辑
</button>
</li>
</template>
<script>
export default {
name: "MyItem",
props: ["todo"], // 声明接收todo
methods: {
handleCheck(id) { // 勾选or取消勾选
// 通知App组件将对应的todo对象的done值取反
// this.checkTodo(id)
this.$bus.$emit("checkTodo", id);
},
handleDelete(id) { // 删除
if (confirm("确定删除吗?")) {
// 通知App组件将对应的todo对象删除
// this.deleteTodo(id)
this.$bus.$emit('deleteTodo',id)
}
},
handleEdit(todo) { // 编辑
if (todo.hasOwnProperty("isEdit")) {
todo.isEdit = true;
} else {
this.$set(todo, "isEdit", true);
}
this.$nextTick(function () {
this.$refs.inputTitle.focus();
});
},
handleBlur(todo, e) { // 失去焦点回调(真正执行修改逻辑)
todo.isEdit = false;
if (!e.target.value.trim()) return alert("输入不能为空!");
this.$bus.$emit("updateTodo", todo.id, e.target.value);
},
},
};
</script>
如下图:
二.过渡与动画
1.作用:在插入,更新或移除DOM元素时,在合适的时候给元素添加样式名
如图: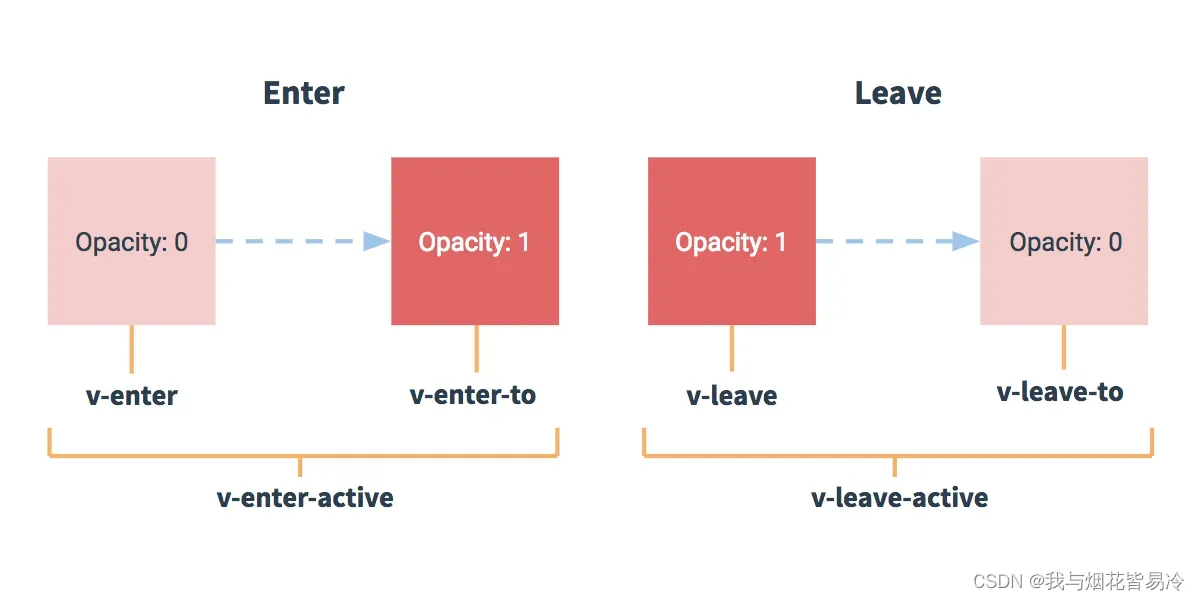
写法:
1.准备好样式
○元素进入的样式
v-enter 进入的起点
v-enter-active 进入过程中
v-enter-to 进入的终点
○ 元素离开的样式
v-leave 离开的起点
v-leave-active 离开过程中
v-leave-to 离开的终点
2.使用<transition>包裹要过度的元素,并配置name属性,此时需要将上面样式名的v换为name
3.要让页面一开始就显示动画,需要添加appear
代码如下:
<transition name="hello" appear>
<h1 v-show="isShow">你好啊!</h1>
</transition>
<style>
.hello-enter-active{
animation: hello 0.5s linear;
}
.hello-leave-active{
animation: hello 0.5s linear reverse;
}
@keyframes hello {
from{
transform: translateX(-100%);
}
to{
transform: translateX(0px);
}
}
</style>
备注:若有多个元素需要过度,则需要使用<transition-group>,且每个元素都要指定key值
<transition-group name="hello" appear>
<h1 v-show="!isShow" key="1">你好啊!</h1>
<h1 v-show="isShow" key="2">尚硅谷!</h1>
</transition-group>
第三方动画库Animate.css
<transition-group appear
name="animate__animated animate__bounce"
enter-active-class="animate__swing"
leave-active-class="animate__backOutUp">
<h1 v-show="!isShow" key="1">你好啊!</h1>
<h1 v-show="isShow" key="2">尚硅谷!</h1>
</transition-group>