步骤:
定义事件,继承ApplicationEvent
定义监听,要么实现ApplicationListener接口,要么在方法上添加@EventListener注解
发布事件,调用ApplicationContext.publishEvent()或者ApplicationEventPublisher.publishEvent();
1、导入maven工程,SpringEvent已经包含在spring-boot-starter-web中
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
2、创建实体类,用于封装操作日志信息
该实体类用来将操作日志中的信息进行数据库的保存
package com.event.domain;
import com.baomidou.mybatisplus.annotation.*;
import java.io.Serializable;
import java.time.LocalDateTime;
import java.util.Date;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import lombok.Data;
/**
*
* 日志操作实体类
*/
@ApiModel(description = "日志操作实体类")
@TableName(value ="pd_auth_event")
@Data
public class Event implements Serializable {
/**
* 操作IP
*/
@ApiModelProperty("操作IP")
@TableField(value = "requestIp")
private String requestIp;
/**
* 日志类型 LogType{OPT:操作类型;EX:异常类型}
*/
@ApiModelProperty("日志类型 LogType{OPT:操作类型;EX:异常类型}")
@TableField(value = "type")
private String type;
/**
* 操作人
*/
@ApiModelProperty("操作人")
@TableField(value = "userName")
private String userName;
/**
* 操作描述
*/
@ApiModelProperty("操作描述")
@TableField(value = "description")
private String description;
/**
* 创建时间
*/
@ApiModelProperty("创建时间")
@TableField(value = "create_time",fill = FieldFill.INSERT)
private LocalDateTime create_time;
@ApiModelProperty(hidden = true)
@TableField(exist = false)
private static final long serialVersionUID = 1L;
}
3、创建事件类
定义系统日志事件,自定义事件需要继承ApplicationEvent
package com.event.event;
import com.event.domain.Event;
import org.springframework.context.ApplicationEvent;
/**
* 定义系统日志事件
*/
public class SysLogEvent extends ApplicationEvent {
public SysLogEvent(Event optLogDTO) {
super(optLogDTO);
}
}
4、创建监听器类
通过@EventListener注解标注对应的事件处理器,处理器会根据参数类型自动识别事件类型的
package com.event.listener;
import com.event.domain.Event;
import com.event.event.SysLogEvent;
import com.event.mapper.EventMapper;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.event.EventListener;
import org.springframework.scheduling.annotation.Async;
import org.springframework.stereotype.Component;
/**
* 事件监听器,监听日志事件
*/
@Component
public class SysLogListener {
@Autowired
private EventMapper eventMapper;
@Async
@EventListener(SysLogEvent.class)
public void saveSysLog(SysLogEvent event) {
Event sysLog = (Event) event.getSource();
long id = Thread.currentThread().getId();
System.out.println("监听到日志操作事件:" + sysLog + " 线程id:" + id);
//将日志信息保存到数据库...
eventMapper.insert(sysLog);
}
}
5、创建Controller,用于发布事件
①实现方式一:
private ApplicationContext applicationContext;
②实现方式二:
private ApplicationEventPublisher applicationEventPublisher;
package com.event.controller;
import com.baomidou.mybatisplus.extension.api.R;
import com.event.domain.Event;
import com.event.event.SysLogEvent;
import io.swagger.annotations.Api;
import io.swagger.annotations.ApiOperation;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.ApplicationEvent;
import org.springframework.context.ApplicationEventPublisher;
import org.springframework.web.bind.annotation.*;
import java.time.LocalDateTime;
/**
* Event日志监听
*/
@Api(value = "/event", tags = {"Event日志监听"})
@RestController
@RequestMapping("/event")
@CrossOrigin
@Slf4j
public class EventController {
@Autowired
private ApplicationEventPublisher applicationEventPublisher;
/**
* 日志监听测试
* @return
*/
@ApiOperation(value = "日志监听测试", notes = "日志监听测试", httpMethod = "GET")
@GetMapping("/test")
@ResponseBody
public R test(){
//构造操作日志信息
Event logInfo = new Event();
logInfo.setRequestIp("127.0.0.1");
logInfo.setUserName("admin");
logInfo.setType("OPT");
logInfo.setDescription("查询用户信息");
//构造事件对象
ApplicationEvent event = new SysLogEvent(logInfo);
//发布事件
applicationEventPublisher.publishEvent(event);
long id = Thread.currentThread().getId();
System.out.println("发布事件,线程id:" + id);
return R.ok("ok").setCode(200);
}
}
6、启动项目并访问Controller可以发现监听器触发了
发布事件,线程id:58
监听到日志操作事件:Event(requestIp=127.0.0.1, type=OPT, userName=admin, description=查询用户信息, create_time=null) 线程id:105
7、查询数据库保存的操作日志
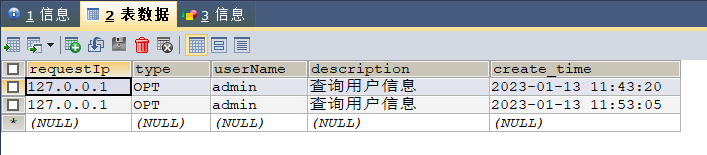
8、项目结构
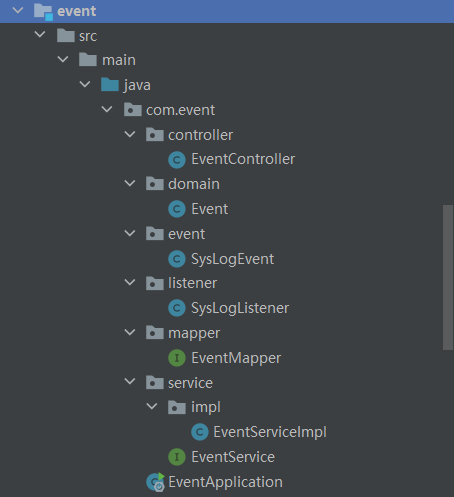