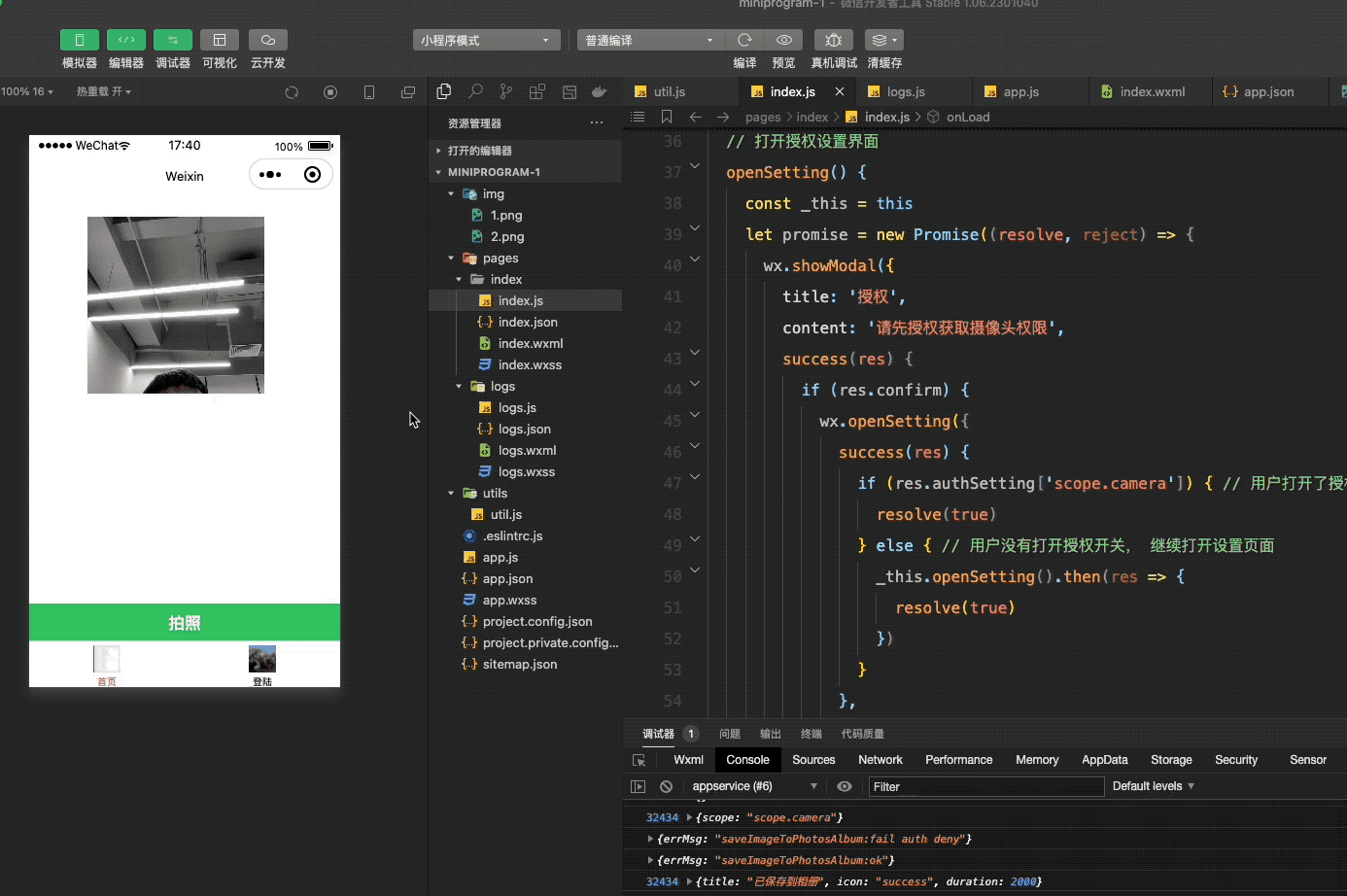
写微信小程序就是要不停的翻阅官方文档查阅所需要的需求。
API的使用说明
wx.getSetting 获取用户的当前设置。返回值中只会出现小程序已经向用户请求过的权限 scope.camera相机
wx.authorize 提前向用户发起授权请求。调用后会立刻弹窗询问用户是否同意授权小程序使用某项功能或获取用户的某些数据,但不会实际调用对应接口。
如果用户之前已经同意授权,则不会出现弹窗,直接返回成功
scope: 'scope.camera' 需要获取权限的 scope: scope.camera 摄像头
wx.showModal
显示模态对话框
wx.openSetting
调起客户端小程序设置界面,返回用户设置的操作结果。设置界面只会出现小程序已经向用户请求过的权限 res.authSetting['scope.camera']表示授权了相机
wx.createCameraContext
创建 camera 上下文 CameraContext 对象。
ctx.takePhoto拍摄照片 ctx.takePhoto({quality: 'high', //quality 成像质量 high 高质量})
wx.previewImage
在新页面中全屏预览图片。预览的过程中用户可以进行保存图片、发送给朋友等操作
wx.downloadFile
下载文件资源到本地。客户端直接发起一个 HTTPS GET 请求,返回文件的本地临时路径 (本地路径),单次下载允许的最大文件为 200MB。使用前请注意阅读相关说明。
注意:请在服务端响应的 header 中指定合理的 Content-Type 字段,以保证客户端正确处理文件类型。
wx.saveImageToPhotosAlbum
保存图片到系统相册。 filePath string 是 图片文件路径,可以是临时文件路径或永久文件路径 (本地路径) ,不支持网络路径
wx.showToast
显示消息提示框
index.js
// index.js
// const { Result } = require("element-ui")
// 获取应用实例
const app = getApp()
Page({
data: {
isAuth: false,
src: '',
modalType: false, //弹框默认不显示
imgUrl: "", //模拟图片
},
async onLoad() {
const _this = this
let res = await app.wx('getSetting')
if (res.authSetting['scope.camera']) {
// 用户已经授权
_this.setData({isAuth: true})
} else {
// 用户还没有授权,向用户发起授权请求
let authorize = await app.wx('authorize', {scope: 'scope.camera' })
if(authorize.msg) {
_this.setData({isAuth: true })
}else {
_this.openSetting().then(res => {_this.setData({isAuth: true})})
}
}
},
// 打开授权设置界面
openSetting() {
const _this = this
let promise = new Promise((resolve, reject) => {
wx.showModal({
title: '授权',
content: '请先授权获取摄像头权限',
success(res) {
if (res.confirm) {
wx.openSetting({
success(res) {
if (res.authSetting['scope.camera']) { // 用户打开了授权开关
resolve(true)
} else { // 用户没有打开授权开关, 继续打开设置页面
_this.openSetting().then(res => {
resolve(true)
})
}
},
fail(res) {
console.log(res)
}
})
} else if (res.cancel) {
_this.openSetting().then(res => {
resolve(true)
})
}
}
})
})
return promise;
},
takePhoto() {
const ctx = wx.createCameraContext()
ctx.takePhoto({
quality: 'high',
success: (res) => {
this.setData({
src: res.tempImagePath
})
wx.previewImage({
current: res.tempImagePath, // 当前显示图片的http链接
urls: [res.tempImagePath] // 需要预览的图片http链接列表
})
this.save(res.tempImagePath)
}
})
},
save(imgUrl) {
let _this = this
let fileName = new Date().valueOf();
wx.downloadFile({
url: imgUrl,
filePath: wx.env.USER_DATA_PATH + '/' + fileName + '.jpg', //自定义临时资源路径
success: (res) => {
let filePath = res.filePath;
wx.saveImageToPhotosAlbum({
filePath,
success(res) {
console.log(res)
app.wx('showToast',{
title: '已保存到相册',
icon: 'success',
duration: 2000,
})
},
fail(e) {
console.log(e)
wx.showToast({
title: '保存失败,请重试',
duration: 2000,
icon: 'none',
})
if(e.errMsg == "saveImageToPhotosAlbum:fail auth deny"){
_this.openSetting().then(res => {_this.setData({isAuth: true})})
}
},
})
}
});
},
})
index.wxml
<view class='camera'>
<!-- <image src="/images/border.png" mode="widthFix"></image> -->
<camera wx:if="{{isAuth}}" device-position="back" flash="off" binderror="error"></camera>
</view>
<button class="takePhoto" type="primary" bindtap="takePhoto">拍照</button>
index.wxss
/**index.wxss**/
.camera {
width: 470rpx;
height: 470rpx;
border-radius: 50%;
margin: 20px auto 0;
position: relative;
}
.camera image {
position: absolute;
width: 100%;
height: 100%;
z-index: 10;
}
.camera camera {
width: 428rpx;
height: 428rpx;
}
button.takePhoto:not([size='mini']) {
position: fixed;
bottom: 0;
left: 0;
width: 100vw;
height: 90rpx;
border-radius: 0;
}
app.js //这里我封装了wx 方法就是为了不使用 success函数和fail 函数,用async 和 await 就可以了。
// app.js
App({
/**
* 本方法就是为了使用能够async和await来获取结果,减少嵌套。
* @attr 微信api的名字
* @params 需要传入的参数
*
* const app = getApp()
* let res = await app.wx('showModal',{title: '授权',content: '43534543',})
console.log(432,res)
* */
wx(attr, params = {}) {
console.log(32434, params )
return new Promise((result, reject) => {
let body = {
success: res => {
console.log(88888,res)
result({...res,msg:attr == 'showModal' ? res.confirm : true})
},
fail: rej => {
console.log(432999,rej)
result({...rej,msg:attr == 'showModal' ? rej.confirm : false})
}
}
for(let k in params){
body[k] = params[k];
}
wx[attr](body)
})
},
globalData: {
userInfo: null
}
})
我是阿豪