文章目录
- 一、处理属性名与字段名不同问题
- 1.通过设置查询别名,使类属性名与字段名(数据库内的名)一致
- 2.设置全局配置,使下划线自动映射为驼峰
- 3.ResultMap
- 二、处理多对一映射问题
- 前提背景
- 1.使用级联来实现
- 2.association 标签实现
- 3.分步查询来实现
- 三、处理一对多问题
-
一、处理属性名与字段名不同问题
1.通过设置查询别名,使类属性名与字段名(数据库内的名)一致
2.设置全局配置,使下划线自动映射为驼峰
<settings>
<setting name="mapUnderscoreToCamelCase" value="true"/>
</settings>
3.ResultMap
<resultMap id="empResultMap" type="com.mybatis.Bean.Emp">
<id property="eid" column="eid" ></id>
<result property="name" column="name"></result>
<result property="age" column="age"></result>
<result property="sex" column="sex"></result>
<result property="email" column="email"></result>
<result property="did" column="did"></result>
</resultMap>
<select id="getEmpt" resultMap="empResultMap">
select * from emp
</select>
二、处理多对一映射问题
前提背景
package com.mybatis.Bean;
public class Dept {
private int did;
private String name;
public int getDid() {
return did;
}
public void setDid(int did) {
this.did = did;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@Override
public String toString() {
return "Dept{" +
"did=" + did +
", name='" + name + '\'' +
'}';
}
}
package com.mybatis.Bean;
public class Emp {
private int eid;
private String name;
private int age;
private char sex;
private String email;
private Dept dept;
public Dept getDept() {
return dept;
}
public void setDept(Dept dept) {
this.dept = dept;
}
@Override
public String toString() {
return "Emp{" +
"eid=" + eid +
", name='" + name + '\'' +
", age=" + age +
", sex=" + sex +
", email='" + email + '\'' +
", dept=" + dept +
'}';
}
public int getEid() {
return eid;
}
public void setEid(int eid) {
this.eid = eid;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public char getSex() {
return sex;
}
public void setSex(char sex) {
this.sex = sex;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
}
1.使用级联来实现
<resultMap id="empResultMap" type="com.mybatis.Bean.Emp">
<id property="eid" column="eid" ></id>
<result property="name" column="name"></result>
<result property="age" column="age"></result>
<result property="sex" column="sex"></result>
<result property="email" column="email"></result>
<result property="dept.did" column="did"></result>
<result property="dept.name" column="dname"></result>
</resultMap>
<select id="getEmpt" resultMap="empResultMap">
select * from emp left join dept
</select>
2.association 标签实现
<resultMap id="empResultMap_" type="com.mybatis.Bean.Emp">
<id property="eid" column="eid" ></id>
<result property="name" column="name"></result>
<result property="age" column="age"></result>
<result property="sex" column="sex"></result>
<result property="email" column="email"></result>
<association property="dept" javaType="com.mybatis.Bean.Dept">
<id property="did" column="did"></id>
<id property="name" column="name"></id>
</association>
</resultMap>
3.分步查询来实现
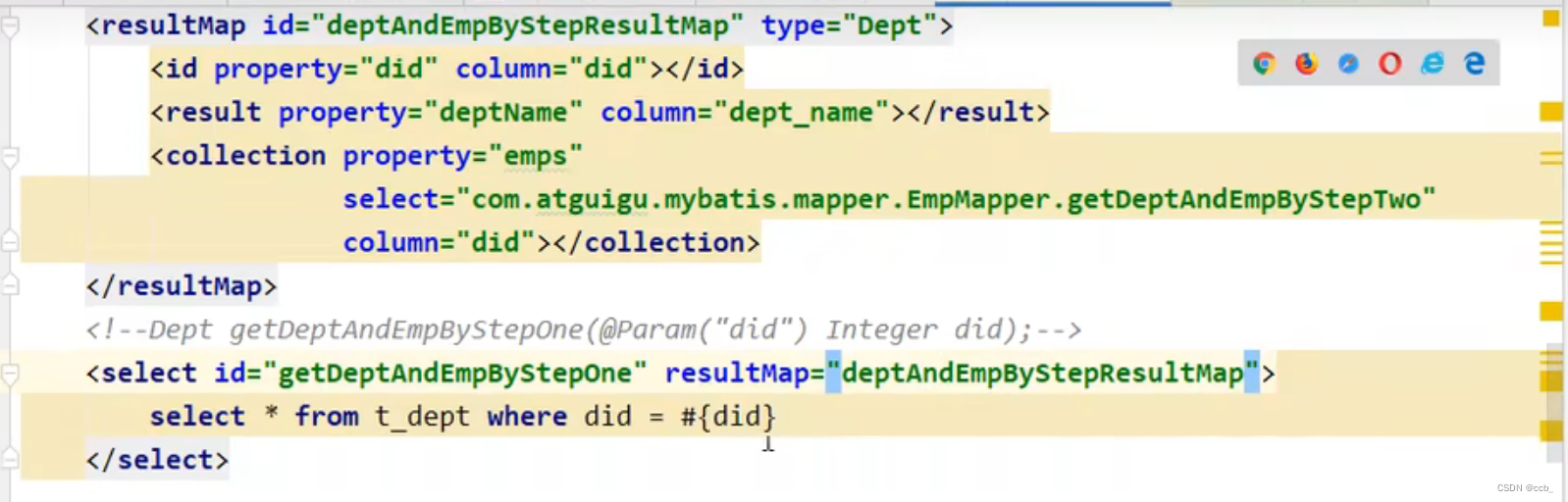
三、处理一对多问题
背景
package com.mybatis.Bean;
import java.util.List;
public class Dept {
private int did;
private String name;
private List<Emp> emps;
public List<Emp> getEmps() {
return emps;
}
@Override
public String toString() {
return "Dept{" +
"did=" + did +
", name='" + name + '\'' +
", emps=" + emps +
'}';
}
public void setEmps(List<Emp> emps) {
this.emps = emps;
}
public int getDid() {
return did;
}
public void setDid(int did) {
this.did = did;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
1.collection实现
<resultMap id="xx" type="com.mybatis.Bean.Dept">
<id property="did" column="did"></id>
<result property="name" column="name"></result>
<collection property="emps" ofType="com.mybatis.Bean.Emp">
<id property="eid" column="eid"></id>
<result property="name" column="name"></result>
</collection>
</resultMap>
<select id="xxx" resultMap="xx">
</select>
2.分步查询
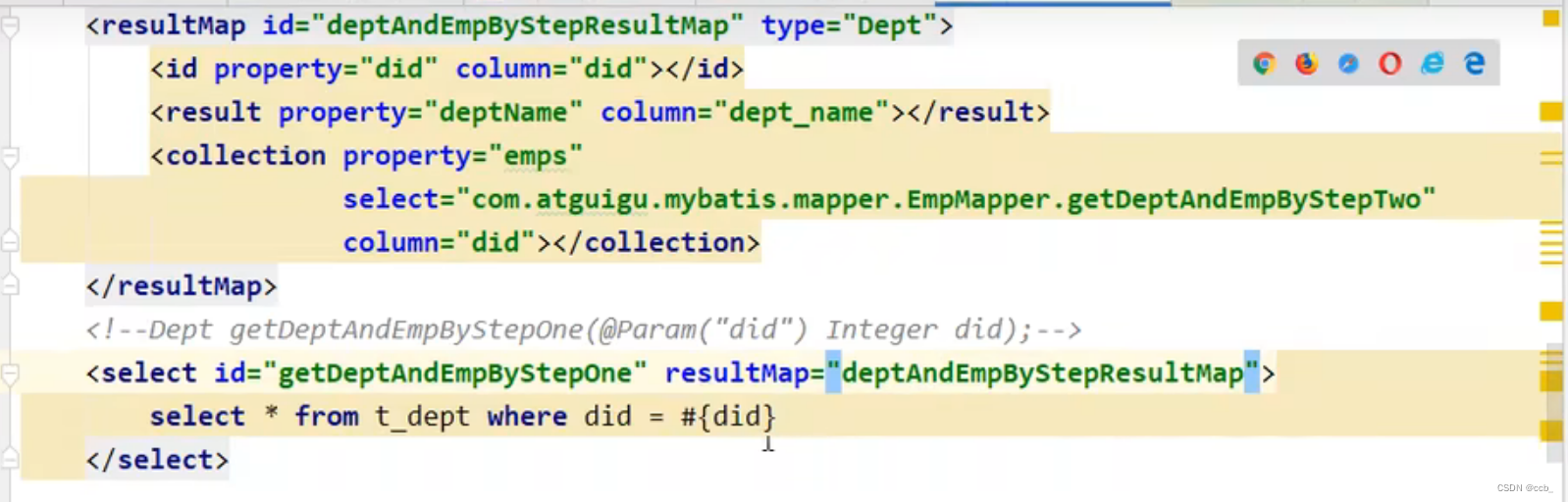